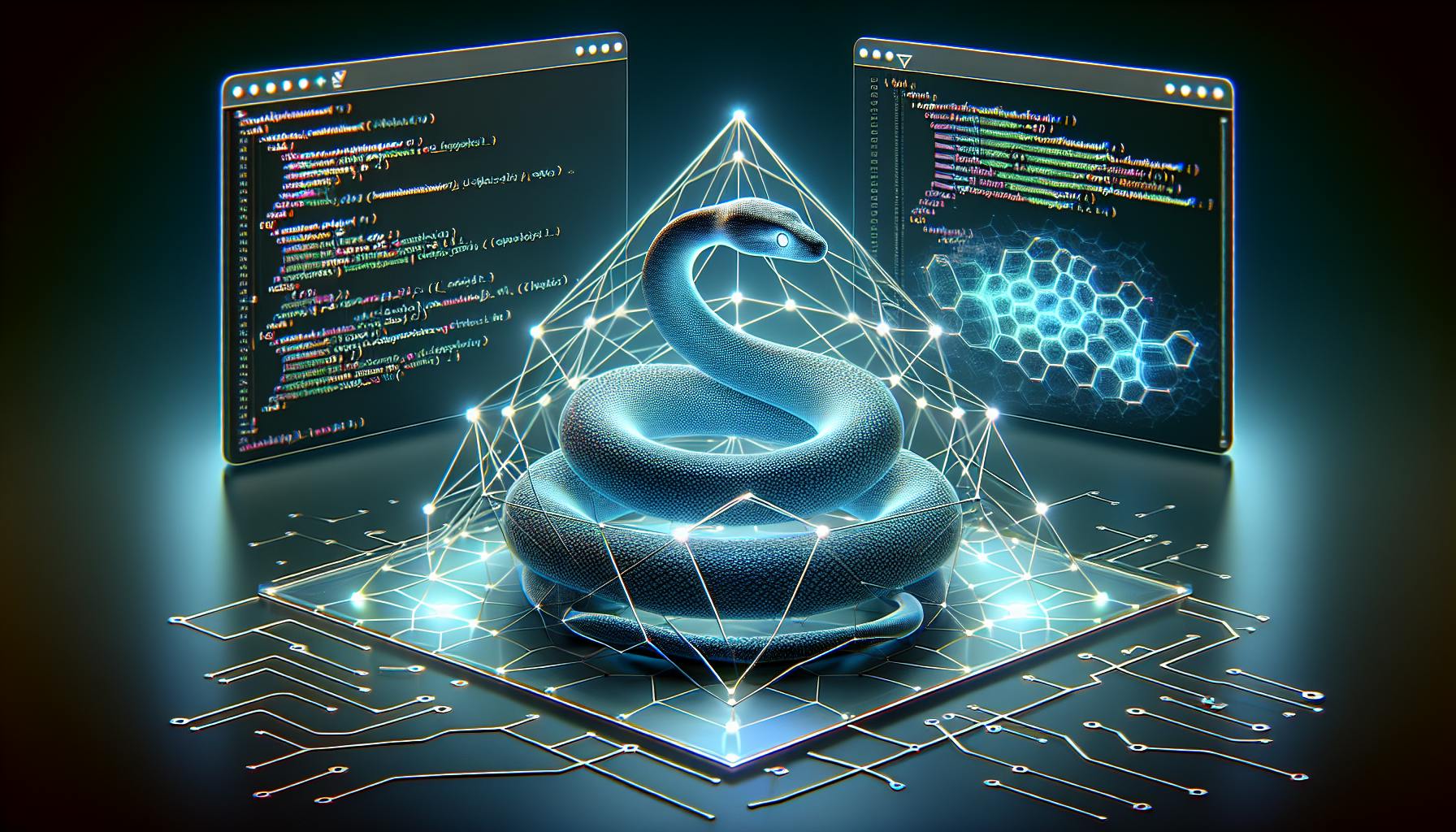
Learn how to integrate Graphene with Django to build efficient GraphQL APIs using Python. Follow a step-by-step guide to set up Graphene-Django in your Django project and start building your first schema.
Integrating Graphene with Django lets you build efficient GraphQL APIs using Python. Here's a quick guide to get you started:
- Install Graphene-Django: First, set up a virtual environment and install Graphene-Django in your Django project.
- Build Your First Schema: Create a Django model, define a GraphQL type for it, and set up queries to interact with your data.
- Executing Queries: Use GraphiQL to test your GraphQL queries, fetching or mutating data as needed.
- Advanced Features: Explore advanced integrations like Relay for pagination, Django forms for data validation, and authentication to secure your API.
Whether you're new to GraphQL or looking to integrate it with your Django project, this guide outlines the steps and concepts to help you start building powerful APIs. For a detailed walkthrough, including code examples and tips on best practices, keep reading.
Installation
Here's a step-by-step guide to get Graphene-Django ready in your Django project.
1. Create a Virtual Environment
It's smart to use a separate space on your computer for each project to avoid mixing up files. That's what a virtual environment does for Python projects.
Here's how to set one up:
- Open your terminal and go to your Django project's folder.
- Type
python3 -m venv env
to make a new virtual environment calledenv
. - Activate it by typing
source env/bin/activate
on Linux or macOS, orenv\Scripts\activate
on Windows.
You'll know it's working because you'll see env
before the prompt in your terminal.
2. Install Graphene-Django
With your virtual environment running, add Graphene-Django by typing:
pip install graphene-django==2.15
This command grabs the version 2.15, which is stable and works well. To find the latest version, check the Graphene-Django releases page.
3. Add to Installed Apps
Next, let Django know about Graphene-Django. Open your settings.py
file and add graphene_django
to the INSTALLED_APPS
section like this:
INSTALLED_APPS = [
# other apps
"graphene_django",
]
This step makes sure Django can work with Graphene-Django properly.
And that's it! You've got Graphene-Django installed and you're all set to start building your GraphQL API.
Building Your First Schema
1. Create a Django Model
First, we need some data to work with. Let's create a simple Django model called Author
:
from django.db import models
class Author(models.Model):
name = models.CharField(max_length=50)
This model is straightforward with just a name
field. After creating it, remember to update your database by running:
python manage.py makemigrations
python manage.py migrate
Then, add a few authors either through Django's admin site or the Python shell for testing.
2. Define a GraphQL Type
Now, let's create a GraphQL type for our Author
model. In a new file called schema.py
, write:
import graphene
from graphene_django import DjangoObjectType
from .models import Author
class AuthorType(DjangoObjectType):
class Meta:
model = Author
fields = ("id", "name")
This code makes our Django model work with GraphQL by turning it into a GraphQL type.
3. Create the Query Class
Next, we decide what kind of data we can ask for. We do this in the Query
class:
class Query(graphene.ObjectType):
all_authors = graphene.List(AuthorType)
def resolve_all_authors(root, info):
return Author.objects.all()
Here, we're saying we want to be able to get all authors. The resolve
function is where we get the data from our database.
4. Set Up the Schema
Lastly, we tie everything together into a GraphQL schema:
schema = graphene.Schema(query=Query)
With this schema, we can now use GraphQL to ask for data about all authors!
Executing Queries
To use your setup to ask for and get data, follow these easy steps:
Set Up GraphiQL
GraphiQL is a tool that lets you try out your data requests in a user-friendly way. To get it ready in Django:
- First, add an extra tool called
graphene-django-extras
pip install graphene-django-extras
- Then, in your
schema.py
file, bring in GraphiQL and set it up:
from graphene_django_extras import GraphiQLPage
urlpatterns = [
# other urls
path("graphiql", GraphiQLPage.as_view(graphiql=True)),
]
- Now, if you go to
localhost:8000/graphiql
on your web browser, you'll see the GraphiQL page.
Write Your First Query
With GraphiQL, you can test out asking for data. Let's try to get a list of all authors:
{
allAuthors {
id
name
}
}
This asks for the id
and name
of each author from the all_authors
part we set up before.
Query Variables
You can make your queries more specific by using variables:
query GetAuthor($id: ID!) {
author(id: $id) {
name
}
}
To test it, use the Query Variables section to give the id
you want to look up.
Fetch Relationships
To get data that's connected, just include it in your query:
{
author(id: 1) {
name
posts {
title
}
}
}
This gets the name and all posts for the author with ID 1.
Perform Mutations
Mutations are how you change data on the server. You set them up like queries:
class CreateAuthor(graphene.Mutation):
# Mutation setup
pass
class Mutation(graphene.ObjectType):
create_author = CreateAuthor.Field()
Then, you can run these mutations from GraphiQL just like you do with queries.
That's a quick look at how to use your Graphene Django setup to ask for and change data! If you have questions, feel free to drop them in the comments.
sbb-itb-bfaad5b
Mutations
Mutations in GraphQL let you change data on the server, like adding, updating, or deleting things. It's a more organized way to make changes than the old-school methods.
1. Create Mutations
To start making changes with Graphene-Django, you need to set up a special kind of command called a mutation. Here's how you do it:
import graphene
class AuthorMutation(graphene.Mutation):
class Arguments:
# Details for the mutation go here
# What you get back after making the change
name = graphene.String()
def mutate(self, info, name):
# The change-making logic goes here
return AuthorMutation(name=name)
class Mutation(graphene.ObjectType):
create_author = AuthorMutation.Field()
This example shows how to add a new author by giving a name. The mutate
part does the actual work, and AuthorMutation
tells you what you'll get back.
You can have many mutations in the Mutation
class for different things like updating or deleting.
2. Execute Mutations
To make these changes happen, you send a special request to the GraphQL spot with something like:
mutation {
createAuthor(name:"John Doe") {
name
}
}
You can also use variables in your requests:
mutation CreateAuthor($name: String!) {
createAuthor(name: $name) {
name
}
}
Just remember to include the variable's value when you send it.
Other examples you might use include:
mutation UpdateAuthor($id: ID!, $name: String!) {
updateAuthor(id: $id, name: $name) {
name
}
}
mutation DeleteAuthor($id: ID!) {
deleteAuthor(id: $id)
}
This setup lets you do more specific things than traditional ways, with clear rules on what's allowed.
Advanced Integrations
Relay Support
Graphene-Django has a feature that helps with organizing big lists of data using something called Relay, which is a way to ask for data in chunks. This is great for loading pages faster because you only get a small part of the data at a time.
To use this, you change a bit of code to ask for data in a special way that understands these chunks:
from graphene_django.filter import DjangoFilterConnectionField
class Query(graphene.ObjectType):
all_books = DjangoFilterConnectionField(BookNode)
When you ask for data, you can now say how much you want at once and get information on whether there's more:
{
allBooks(first: 10) {
pageInfo {
hasNextPage
}
edges {
cursor
node {
id
title
}
}
}
}
This way, you don't have to load everything at once, making your app faster.
Django Forms
You can use Django's forms in your GraphQL setup to check if data is correct before saving it. This is handy because Django forms are good at checking data.
Here's an example:
from graphene_django import DjangoModelFormMutation
class AuthorForm(forms.ModelForm):
class Meta:
model = Author
fields = ("name", "birth_date")
class CreateAuthor(DjangoModelFormMutation):
class Meta:
form_class = AuthorForm
class Mutation(graphene.ObjectType):
create_author = CreateAuthor.Field()
This code makes sure your data is okay before adding a new author, using forms you're maybe already familiar with.
Authentication
To make sure only the right people can see or change data, you can use login checks and permissions.
For example, to make a part of your data private:
from graphql_jwt.decorators import login_required
@login_required
class PrivateQuery(graphene.ObjectType):
my_data = graphene.String()
You can also check if someone has the right permissions to see data:
from graphene_django.filter import DjangoFilterConnectionField
class Query(graphene.ObjectType):
all_books = DjangoFilterConnectionField(
BookNode,
permission_classes=[IsAdminUser],
)
For logging in and staying logged in, there's a tool that works with GraphQL to handle logins and keeping users authenticated.
Overall, these features help keep your data safe and make sure only the right people can access or change it.
Conclusion
Mixing Graphene with Django lets you create cool GraphQL APIs using Python. Django's tools and features, like checking who can do what and handling data safely, help you make a strong GraphQL service quickly.
Here's what we've covered:
- Graphene-Django makes it easy to work with Django's data models in GraphQL.
- You can ask for data and make changes to it using queries and mutations.
- Features like breaking data into pages, filtering, and making sure only the right people can access certain data make your API strong and useful.
- Using Django forms, you can check if the data is okay before saving it.
- GraphiQL is a handy tool for testing and playing with your API.
In short, Graphene helps you build GraphQL APIs in Python easily, and Django gives you a bunch of tested tools and features. They work great together for making flexible and quick services.
Next, you might want to put your API out there for others to use and look into using frontend frameworks like React to display your GraphQL data. The Graphene documentation has lots of extra examples and tips to help you build on what you've learned.
Happy coding with Graphene and Django!