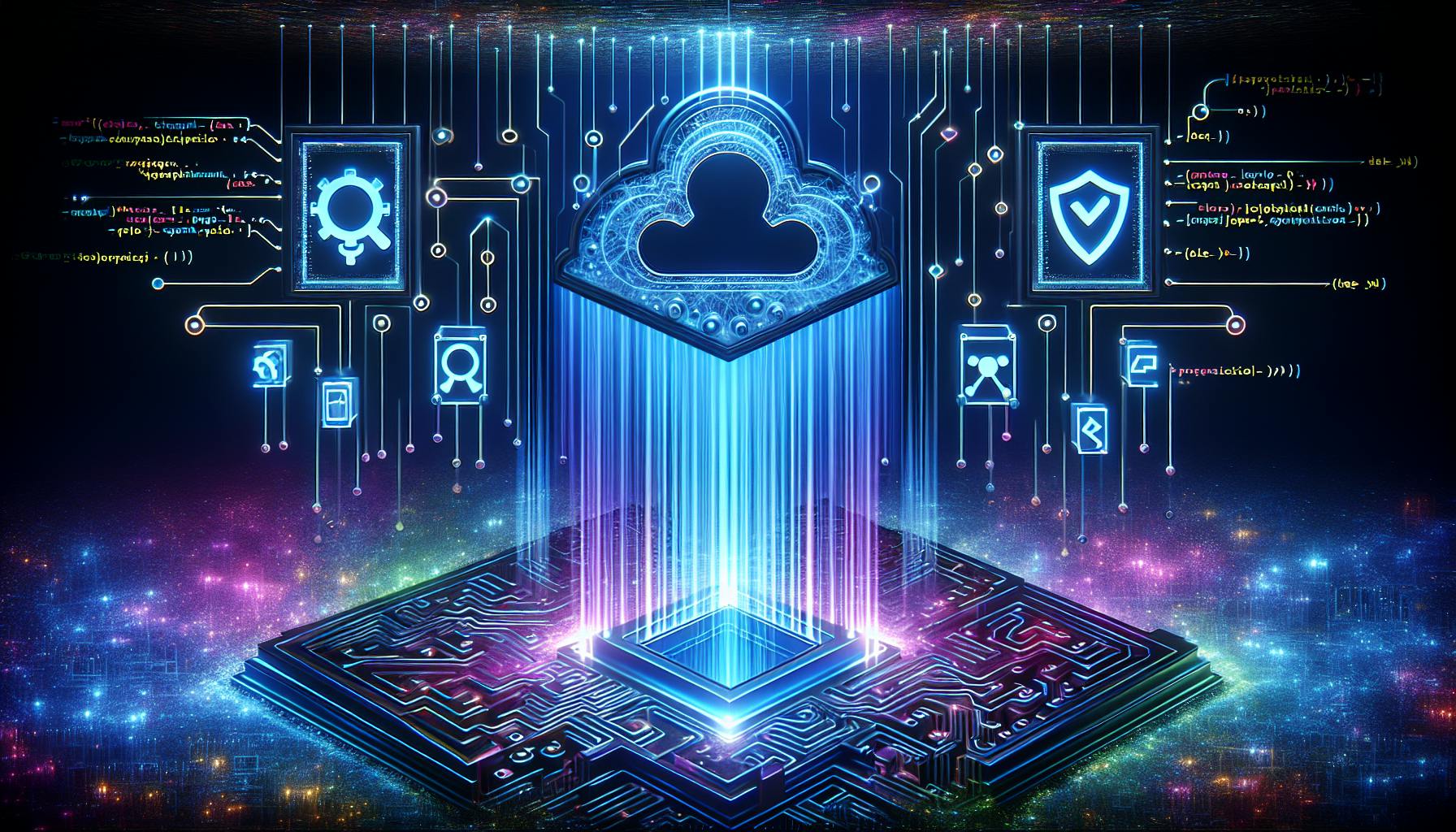
Master GraphQL best practices for efficient APIs. Understand the basics, design schema effectively, optimize queries, handle errors gracefully, secure your API, and more.
GraphQL transforms how we build and interact with APIs by allowing precise data requests. Here's a quick guide to mastering GraphQL for efficient APIs:
- Understand the Basics: Learn what GraphQL is, its core concepts like schema, queries, mutations, and more.
- Design Your Schema Effectively: Use clear names, keep it simple, and design with flexibility and scalability in mind.
- Optimize Your Queries: Avoid common pitfalls like over-fetching, and implement strategies like batching and caching for better performance.
- Handle Errors Gracefully: Provide clear error messages and follow best practices for error handling.
- Secure Your API: Implement authentication, authorization, and input validation to protect your API.
- Best Practices for API Consumption: Write focused queries, implement caching, and handle errors on the client side.
- Evolve and Version Your API: Use strategies for deprecating fields and evolving your schema without breaking existing clients.
- Leverage Tools and Libraries: Utilize development, monitoring, and testing tools to build and maintain your GraphQL API efficiently.
By following these best practices, you can create GraphQL APIs that are fast, flexible, and maintainable, providing a better experience for both developers and users.
What is GraphQL?
GraphQL is a tool that lets you ask for exactly what data you need from a website or app, and not a bit more. It's like being able to customize your meal at a restaurant down to the last detail, rather than picking a set dish from the menu. Facebook made it in 2012, and then shared it with everyone in 2015.
Here’s what makes GraphQL special:
- It has a strongly typed schema which is like a blueprint that both the client (you asking for data) and the server (where the data lives) follow.
- You can ask for specific types of data and get just that.
- It lets you write out your data requests in a special language.
- The server has resolvers that find the data you asked for and send it back to you.
Unlike traditional ways of getting data from the internet, where you get whatever the server sends, GraphQL lets you ask for and get exactly what you need. This saves time and resources.
Some cool things about GraphQL are:
- You can get lots of different things in one go.
- You only get what you asked for, nothing extra.
- It’s easy to see what data you can ask for because of its standardized setup.
- There’s only one version you need to work with, making things simpler.
- It comes with handy tools for developers.
Overall, GraphQL makes it easier for developers to get their work done, helps apps run faster, and lets you build more complicated things.
Core Concepts of GraphQL
Here are the basics of how GraphQL works:
- Schema: This is like a contract or agreement between you and the server about what data you can ask for. It tells you what’s available.
- Queries: These are like questions you ask to get data.
- Mutations: If you want to add, change, or delete data on the server, you use these.
- Subscriptions: These let the server send you updates automatically when something changes.
- Types: The schema talks about different kinds of data models. Basic types like text or numbers help define simple details.
- Fields: These are specific pieces of data you can ask about. You can also give specific instructions to get exactly what you need.
- Resolvers: These are like helpers on the server that find the data for you.
Getting the hang of these basics is important for making, using, and understanding GraphQL APIs well. The schema is your guide, while queries, mutations, and types are tools for working with data. Resolvers are what make it all come together, connecting your requests to the actual data.
Schema Design
Best Practices in Schema Design
When setting up a GraphQL schema, it's key to keep it easy to understand. Here's how to do it right:
- Use clear names: Pick names for types, fields, and so on that tell you what they are. This makes your schema easy to get without needing extra explanations.
- Keep it simple: Don't make your data models too complicated with lots of layers. Try to keep them straightforward.
- Use shared features and mix-and-match types: Interfaces let you show what different types share, and unions let you mix different types in one spot. This makes your schema flexible.
- Use specific types for special data: For things like dates or IDs, create special types. This helps make sure the data fits what you need.
- Allow for missing data: It's okay if some data isn't there. Making fields able to be empty helps avoid errors.
- Break up your data: When showing a lot of data, split it up in a smart way. This helps avoid sending too much at once. Make sure to tell clients when there's more data to see.
- Update carefully: If you need to stop using certain fields, do it slowly. Let people know, move them to new options, then remove the old ones safely.
Advanced Schema Design Techniques
For those looking to go a step further, consider these tips:
- Break it down: Divide your schema into smaller parts that you can put together. This keeps it easy to handle.
- Add smart commands: Use special commands to control what data you get based on certain conditions.
- Save common requests: Use saved queries to make repeated requests quicker and smaller.
- Keep related things together: Put schema types and their resolvers in the same file. This makes it easier to work with.
- Use smart data fetching: Choose a way to fetch data that automatically handles grouping and storing data efficiently.
- Cache responses: Use a cache on the server side to speed up responses for complex queries.
- Use a central gateway for split services: If your schema is spread across different services, use a central point to manage them. This keeps things smooth for the users.
Starting with these best practices in mind helps build GraphQL APIs that can grow and change easily, meeting the needs of users over time.
Query Optimization
This part looks at common issues with GraphQL queries and how to make them work better.
Avoiding Common Pitfalls
GraphQL lets you ask for exactly what you need, which is great. But, if you're not careful, you can run into some problems. For example, if you ask for a list of posts and all their comments, you might end up making way more requests to the server than you expected. This is called the N+1 problem. Imagine asking for 100 posts; you might end up making 101 queries!
Other issues include:
- Over-fetching: This is when you ask for more information than you really need. It's like packing too much for a trip.
- Under-fetching: This happens when you make too many small requests. It's like going back and forth to the store because you forgot items.
Performance Optimization Strategies
Here's how you can avoid these problems:
- Batching: This means grouping your queries together so you don't overload the server. Think of it like doing all your errands in one trip.
- Query complexity analysis: This is a fancy way of saying "make sure your queries aren't too complicated." It helps keep things running smoothly.
- Caching: This is when the server remembers your request, so if you ask for the same thing again, it can answer faster.
- Automatic persisted queries: This is a bit like saving your favorite orders at a restaurant. Next time, you just give a code instead of ordering from scratch.
Other smart moves include grouping related data together (colocating fragments), splitting data into pages (pagination), avoiding asking for the same thing twice (request deduplication), and using fast internet services (CDNs) for stuff that doesn't change.
By optimizing your GraphQL queries, you can get the data you need more quickly and without asking too much from the server.
Error Handling and Security
Effective Error Handling
Dealing with mistakes the right way is super important for a smooth experience and keeping your GraphQL API running well. Here's how to do it:
Be specific with error messages
- Give clear error messages that help fix problems. Include what went wrong and where in the response.
Stick to the rules
- Use the standard error types for common issues, like when something doesn't fit the rules.
Keep an eye on errors
- Log errors and set up warnings for when they happen more than usual. This helps you spot trouble early.
Manage unexpected errors smartly
- Ensure the API can deal with surprises without breaking. Send a simple error message back if something goes wrong.
Explain errors in your docs
- In your documentation, talk about the errors users might run into. Offer tips on how to fix them.
Securing Your GraphQL API
Keeping your API safe is key. Here are the main things to focus on for GraphQL:
Authentication
- Use common methods like OAuth and OpenID for logging in.
Authorization
- Make sure to check if a user is allowed to do something before letting them proceed.
Check inputs carefully
- Always check, clean, and safely handle any data users send in queries.
Limit how much users can do
- Put caps on how many requests a user can make to stop misuse and prevent the system from getting overwhelmed.
Be careful with introspection
- Turn off introspection in the real world to keep your server's details safe.
Keep your server secure
- Stick to best practices for server security, like managing network access, controlling who can do what, keeping track of activities, and more.
Best Practices for API Consumption
Client-Side Considerations
When you're using a GraphQL API from an app, it's smart to keep things running smoothly and quickly. Here's what to keep in mind:
Write focused queries and mutations
- Only ask for the data you really need to keep things light.
- Don't grab extra data you won't use.
- Change up your requests with variables for flexibility.
Implement caching
- Remember the results of your queries to avoid asking again.
- Make sure to refresh your cache when data changes.
- Set up cache rules for certain data when it makes sense.
Use pagination for long lists
- Ask for bits of big data lists at a time to keep your app from slowing down.
- Move through pages with cursor-based navigation.
Handle errors gracefully
- Plan for mistakes and manage them without crashing your app.
- Keep track of errors to understand where things go wrong.
- Show friendly error messages to users.
Optimistic UI updates
- Make your app seem faster by updating the display before confirming with the server.
Batch operations
- Combine several changes into one request to save time.
Persistent queries
- Use saved queries with a unique ID to cut down on the amount of data sent.
Versioning and Evolution
As your GraphQL setup grows, try to make changes without breaking things:
Deprecate fields
- Mark old fields as outdated but keep them around for a while.
Null-safety
- Allow fields and arguments to be optional to avoid errors.
Default values
- Set defaults for arguments to keep things working as they were.
Alias fields
- Introduce new fields by renaming them, then phase out the old ones.
Gate breaking changes
- Let specific clients opt into big changes with a special setting.
Separate schemas
- Keep different versions for old and new clients, directing them as needed.
Maintain change log
- Keep a record of changes so everyone knows what's new and different.
sbb-itb-bfaad5b
Tools and Libraries
This part talks about the must-have tools and libraries if you're working on setting up GraphQL APIs that work well and don't give you a headache.
Essential Tools for Development
Here, we dive into some handy tools for building, testing, and keeping an eye on your GraphQL API:
GraphQL IDEs
- GraphiQL: A simple tool you can use in your web browser to play around with and learn about your GraphQL API. It helps you write queries with suggestions and shows you the structure of your data.
- Playground: Like GraphiQL, but with more bells and whistles. You can tweak the look, work with different settings, and more.
- Apollo Studio: A more advanced tool that lives online. It helps you keep track of your GraphQL API's health, catch errors, and understand how your API is being used.
Server Libraries
These are some go-to libraries for making your server understand and use GraphQL:
- Apollo Server: A package for Node.js that comes with everything you need to get your GraphQL server running smoothly.
- graphql-yoga: Another Node.js server option that's easy to set up and use.
- Graphene: For those using Python, this library helps you build your GraphQL API in a way that feels natural in Python.
Client Libraries
Tools for the apps using your GraphQL API to talk to the server efficiently:
- Apollo Client: A JavaScript library that helps your web or mobile app communicate with GraphQL easily. It handles data caching and updates your app's UI when data changes.
- Relay: Made by Facebook for React apps. It's all about grabbing data in the most efficient way possible.
- Urql: A simpler, lightweight option for JavaScript apps that keeps things straightforward.
Monitoring and Testing
Making sure your GraphQL API is running like a well-oiled machine:
Performance Testing
- k6: A tool for putting your GraphQL API under pressure to see how well it can handle lots of users at once.
- Artillery: Another tool for testing how much load your GraphQL API can take before it starts to slow down or have problems.
Validation
- eslint-plugin-graphql: Helps make sure your GraphQL code is correct and won't cause any issues.
- graphql-validator: Checks queries coming into your server to prevent bad or harmful requests.
Observability
- Apollo Tracing: Lets you see how long it takes for different parts of your GraphQL API to respond, helping you find and fix slow spots.
- GraphQL Metrics: Gives you detailed info on how your GraphQL API is performing, like how fast it's responding and where errors are happening.
Mocking
- Mock Service Worker: Lets you pretend to make real requests to your server without actually hitting the server. Great for testing.
- GraphQL Faker: Creates a fake version of your GraphQL API with made-up data, so you can test without using real data.
By having the right tools in your toolkit, you can make sure your GraphQL API is ready for action, stays fast, and doesn't run into trouble.
Real-world Examples and Case Studies
Case Studies of Efficient GraphQL Implementations
Here are some real-life stories of companies that made their systems better with GraphQL:
Airbnb
- Switched their website and apps to GraphQL.
- Noticed their mobile app worked 40% faster.
- Found it easier and quicker to add new features because of GraphQL’s flexibility.
Netflix
- Chose GraphQL for sending movies and shows to users.
- Used automatic persisted queries to cut down on internet use.
- Added detailed permissions for better security.
PayPal
- Created a GraphQL gateway to connect their different services.
- Their GraphQL setup deals with 500 billion requests every day.
- Relies on GraphQL’s strong typing to make sure everything runs smoothly.
These stories show that GraphQL can make things faster, more flexible, and help teams build stuff quicker. The improvements in speed and the ability to easily change things have been really helpful for these companies.
Common Mistakes and Lessons Learned
Here’s what some experts say about common slip-ups with GraphQL:
Overfetching data
- It’s easy to ask for more info than you need because GraphQL lets you.
- This can make responses bigger and slower.
- Tip: Think carefully about what you ask for and keep it to just what you need.
Not using pagination
- Trying to get long lists without pagination can cause delays.
- It’s also easy to accidentally ask for too much, which can overload servers.
- Tip: Use pagination to break down data into smaller chunks.
Ignoring caching
- If you keep asking for the same things without caching, it wastes resources.
- This puts extra pressure on servers.
- Tip: Set up caching to save time and resources.
Security vulnerabilities
- Letting people look into your GraphQL setup can expose your server.
- Sometimes, people forget to check the data being sent in.
- Tip: Stick to security best practices to keep your data safe.
Avoiding these common mistakes and learning from others can really help when using GraphQL in your projects.
Conclusion
GraphQL lets developers create APIs that are flexible and let clients ask for just what they need. But, to get these good points, it's important to stick to some best practices in how you set up your schema, make your queries better, and handle mistakes.
Key Takeaways
- When you're making your schema, keep it simple and clear. Use special types for certain data, make sure it's okay if some data is missing, and explain any changes clearly.
- Make your queries smarter to avoid asking for too much or too little. Using batching, server-side response cache, and saved queries can make things run smoother.
- When errors happen, be clear about what went wrong and stick to common ways of dealing with them. Keep an eye out for problems and make sure your API is safe.
- On the client side, make sure your queries are to the point, use caching and pagination, and be ready for errors.
- Use the right tools for testing, keeping track of how things are running, and fixing bugs during development.
Following these best practices will help you make GraphQL APIs that are ready for real use, efficient, and can handle a lot of work. Planning how you design your schema, making your queries work better, dealing with errors the right way, and using helpful tools are all key steps to success.
Even though GraphQL makes things easier for developers, it takes careful work to get the most out of it. By using these tips for schema design, making queries better, handling errors, and more, you can make top-notch GraphQL APIs that your users will love.
Related Questions
How can I improve my GraphQL API performance?
To make your GraphQL API faster, especially for big queries, break down the responses into smaller parts using pagination. This method is perfect for queries that end in List
, like articleList
.
Is GraphQL more efficient than REST API?
Yes, GraphQL tends to be quicker because it avoids making multiple network calls, which is common with REST APIs. Also, developing with GraphQL can be faster compared to REST.
How does GraphQL make APIs more maintainable?
GraphQL APIs use a single, evolving version. This means apps can always use new features without much hassle. It also leads to cleaner and easier-to-maintain server code.
What is a typical benefit of a client using a GraphQL API over a REST API?
With REST APIs, you often get back more data than you need. This can slow things down. GraphQL lets you ask for exactly what you want, which can make things run smoother. Also, you can get multiple types of data in one go, which is harder with REST APIs.