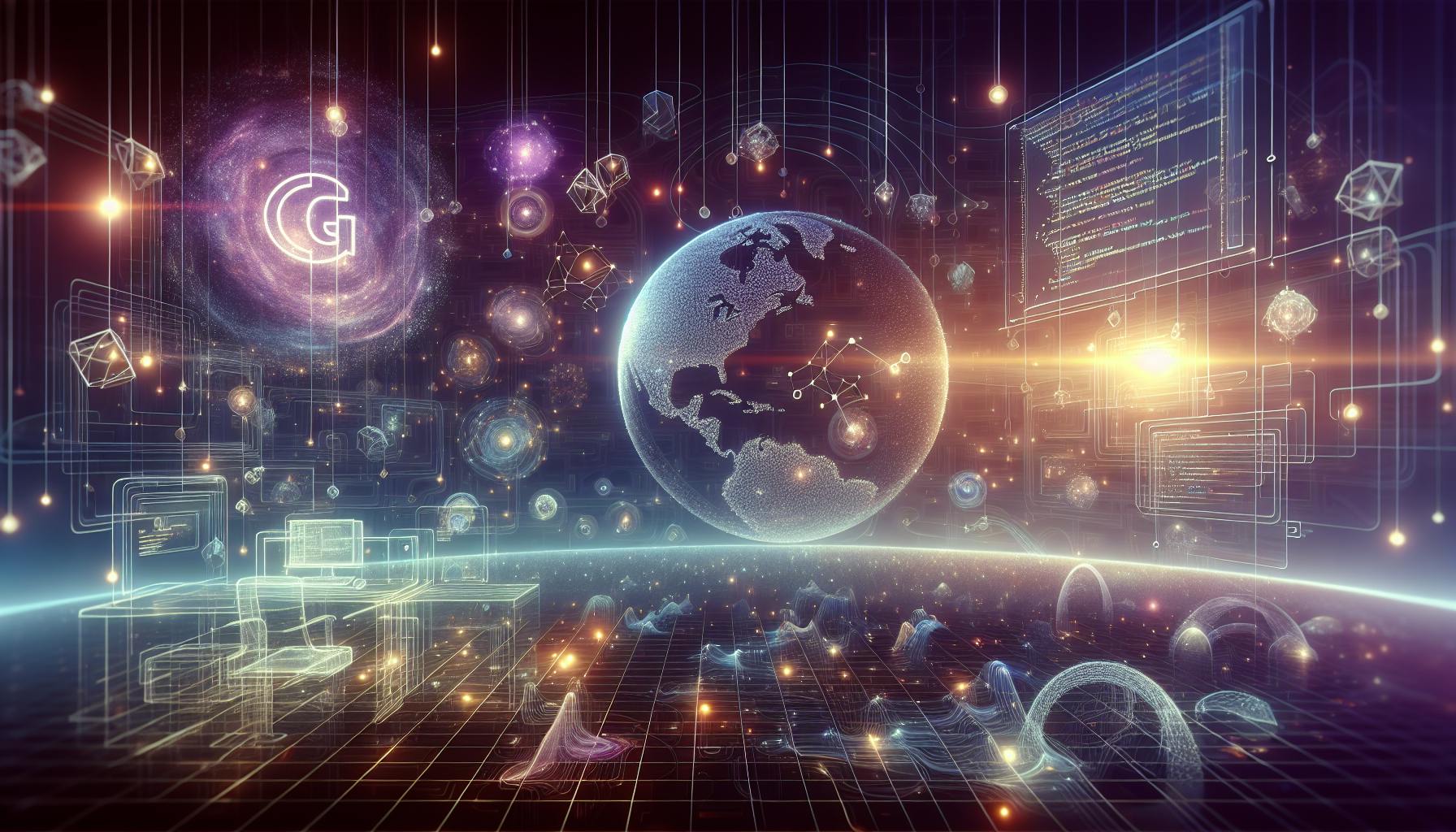
Learn about the basics of Async GraphQL, including increased throughput, simpler code, efficient resource usage, core concepts, setting up your environment, testing strategies, advanced topics, common challenges, and best practices.
Async GraphQL combines the power of GraphQL query language with the efficiency of asynchronous programming, offering a fast, efficient way to fetch data without waiting for one process to finish before starting another. Here's a quick overview:
- Increased Throughput and Faster Response Times: Your server can handle multiple requests simultaneously, allowing for quicker responses to users.
- Simpler, Cleaner Code: Using async/await makes your codebase more readable and less cluttered.
- Efficient Resource Usage: Asynchronous operations require fewer resources, making your applications more scalable.
- Core Concepts: Learn about asynchronous operations, promises, futures, and how they apply to GraphQL.
- Setting Up Your Environment: Guidance on choosing the right runtime, GraphQL library, and additional tools for development.
- Quickstart Guide: Steps to add dependencies, write a schema, execute queries, and integrate with a web server.
- Testing: Strategies for unit, service, resolver, and integration testing of your Async GraphQL applications.
- Advanced Topics: Dive into database integration, authorization, security, and real-time data handling.
- Common Challenges and Best Practices: Tips for performance tuning, debugging, and ensuring security.
Whether you're a developer looking to enhance your app's performance or just curious about Async GraphQL, this guide provides a comprehensive understanding of its basics and how to implement it effectively.
Comparison of Sync vs. Async GraphQL
Metric | Synchronous GraphQL | Asynchronous GraphQL |
---|---|---|
Throughput | Lower (one by one) | Higher (all at once) |
Latency | Higher (waits around) | Lower (quick) |
Code Complexity | Simple | A bit more complex (uses async/await) |
Resource Usage | Needs more | Needs less |
Ecosystem | Well-established | Growing |
In simple terms, traditional GraphQL works one query at a time, which is straightforward but not very fast.
Async GraphQL, on the other hand, can handle many things at once, making it faster and more efficient, even though the code might be a bit trickier.
So, in short, async GraphQL can do a lot more, faster, than the regular kind, even if it means learning a few new tricks. For handling lots of queries, async is definitely the way to go.
Core Concepts of Async GraphQL
Async GraphQL uses some smart tricks to do a lot of tasks at once without getting stuck. Let's dive into these main ideas.
Asynchronous Operations
Imagine you're in a kitchen cooking several dishes at once. You don't just stand there watching water boil. Instead, you multitask - chopping veggies while the pasta cooks. That's what asynchronous operations do. They let different bits of code run at the same time, so your app doesn't just sit there waiting when it could be doing something else.
Here's what makes asynchronous operations special:
- Non-blocking: Your app can keep running other code while it waits for something like data from the internet.
- Concurrent: Lots of tasks can happen at the same time.
- Event-driven: When a task finishes, like getting the data, it tells the app, and the app can move on to the next thing.
- Single-threaded: This all happens in one line of work, so it's like having one really efficient worker instead of a bunch of them getting in each other's way.
In GraphQL, this means that when your app asks for different pieces of data, it doesn't have to wait for one to finish before starting on the next. Everything can happen at once.
Promises and Futures
When you're doing lots of things at once, you need a way to keep track of what's finished and what hasn't. That's where promises and futures come in:
- Promises: These are used in JavaScript. A promise is like saying, "I'll eventually have this data for you." It can be waiting, done, or there might have been a problem. Promises let you deal with each of these outcomes.
- Futures: These are like promises but are used in Rust. A future is something that will have a value later on. You use
.await
to wait for it, which is a bit like saying, "I'll pause here until this task is done."
Both promises and futures make it easier to handle when you're doing a lot of tasks that depend on each other. They help keep things organized so your app can run smoothly without getting bogged down.
By using these tools, GraphQL APIs can handle lots of requests at the same time more efficiently. This is what gives async GraphQL its speed advantage.
Setting Up Your Environment
Getting started with async GraphQL means setting up the right tools and space to work in. Here's a simple guide on what you'll need:
Choose a Runtime
Think of a runtime like the engine that makes async GraphQL go. Here are some good options:
- Node.js - It's been around for a while and has lots of tools for GraphQL and async work. You'll use something called
async/await
here. - Rust - It's a bit more complex but super fast. It uses something called futures and the
.await
syntax. - Go - Known for being fast and good at doing many things at once. It uses goroutines and channels.
Select a GraphQL Library
This is the toolbox that helps you build your GraphQL server, like creating your data blueprint (schema) and figuring out how to get the data (resolving queries). Here are some good picks:
- Apollo Server (Node.js) - It's ready for async out of the box.
- Juniper (Rust) - Built with async in mind.
- gqlgen (Go) - Based on
graphql-go
and supports async.
Choose Helper Libraries
These are like your handy tools that make building easier:
- Validation - Checks if queries match your schema.
- Instrumentation - Helps with logging and keeping track of performance.
- Data Loading - Lets you bundle up requests to save time (DataLoader).
Pick an IDE
A good coding environment can make your work much smoother. Here are some favorites:
- VS Code - Has lots of support for GraphQL and async coding.
- IntelliJ IDEA - Good for Rust and Go coders.
- WebStorm - Great for JavaScript and works well with Apollo tools.
Take some time to play around with different tools and see what works for you. And remember to keep your tools updated! Once you have everything set up, you'll be ready to dive into building your project.
Quickstart Guide to Async GraphQL
Add Dependency Libraries
To kick things off with async GraphQL in Rust, you'll need to grab a few important libraries:
- juniper - This is the main library for working with GraphQL in Rust. Just add it to your Cargo.toml file.
- tokio - This one helps your code do multiple things at once, like a multitasking wizard. Remember to also grab tokio-stream and futures crates.
- warp - A handy tool for managing web requests and making everything work together smoothly.
Once you have these, you're all set to start piecing together an async GraphQL server.
Write a Schema
Your schema is like a blueprint that shows how your data is organized. For instance:
struct User {
id: i32,
name: String,
}
object User {
id: ID!
name: String!
}
Juniper automatically understands how to match your Rust structures to GraphQL thanks to some smart annotations you add to your code.
Now, when someone asks for a User, Juniper knows exactly what to do.
Execute the Query
With your blueprint ready, you can start answering questions (queries):
let schema = Schema::build(Query, Mutation, Subscription).finish();
let query = r#"
query {
user(id: 1) {
id
name
}
}
"#;
let res = schema.execute(query); // returns Result
This will give you a 'promise' (remember those?) that eventually turns into data.
Output as JSON
To turn the data into something readable, like JSON, you'd do:
let json = serde_json::to_string(&res).unwrap();
And there you go, you can send this JSON back to whoever asked for it.
Web Server Integration
To wrap it all up and get your server up and running, you'll do something like:
cargo run --bin server
This starts your server, ready to take on GraphQL requests, process them, and send back answers, all without missing a beat.
Testing Async GraphQL Applications
Testing is a super important step when you're building Async GraphQL apps. It's all about making sure everything works the way it should. Let's dive into the main ways to test your app.
Unit Testing
Unit testing is about checking the small parts of your app to see if they work right on their own. For Async GraphQL, this includes:
- Resolvers - These are the bits that get the data for your queries and mutations. You can pretend to be the schema and context to test them.
- Models - These are your data structures, representing your data. You can use fake data and checks to test them.
- Services - This is the logic part that talks between GraphQL and your models. Here, you pretend the models are real to test the services.
This way, you can quickly find and fix problems in specific spots.
Service Tests
Service tests are a step up from unit tests. They check how different parts of your app work together, like between GraphQL and your database models. You make believe with GraphQL but use real models to:
- Make sure service methods act right under different situations
- Check that the expected database actions happen
These tests confirm that the pieces of your app fit and work together well.
Resolver Tests
Resolvers are key spots where your app connects different parts. Testing them specifically is smart. Here's how:
- Set up a simple GraphQL schema
- Put in some basic query/mutation resolvers
- Think of different situations to test
- Make sure the resolver's answers are what you expect
This checks that resolvers do their job right before you put everything together.
Integration Tests
After checking the small parts, integration tests look at your whole app working together. This is about making sure the real data flow works.
For Async GraphQL, good ways to do this include:
- Fill the database, turn on the GraphQL server, run queries, and see if the API talks to the data layer correctly
- Use tools like Playwright to go through your app's features from start to end, checking if the async parts work right
By mixing these testing methods, you build a strong safety net for your Async GraphQL apps.
sbb-itb-bfaad5b
Advanced Topics in Async GraphQL
Database Integration and Models
When you're creating an Async GraphQL API, connecting it to a database is crucial for storing and retrieving data. Here's how to do it right:
- Pick a library that helps you talk to the database easily. For Node.js, Sequelize or Prisma are good picks. Rust users might like Diesel or MongoDB.
- Match your database models to your GraphQL types. This means if you have a User type in GraphQL, you should have a similar User model for your database. Like this:
type User {
id: ID!
name: String!
}
model User {
id: Integer @id
name: String
}
- Make services for database access. These services will be responsible for getting the data your resolvers need. Keep all the database talk inside these services.
struct UserService {
repo: UserRepository,
}
#[Object]
impl User {
async fn user(id: i32, ctx: &Context) -> User {
let service = ctx.service::<UserService>();
service.get_by_id(id).await
}
}
This setup helps keep your app organized and easy to manage.
Authorization and Security
Keeping your Async GraphQL app safe is super important:
- Use roles to control who can do what.
- Protect sensitive fields with field-level security.
- Stop overly complex or deep queries to keep your server from getting overwhelmed.
- Only let your own frontend talk to your GraphQL API by setting up CORS correctly.
- Check JWT tokens on every request to make sure the user is allowed in.
Don't forget about:
- Encrypting private data
- Checking inputs for errors
- Limiting how often someone can try to access your API
- Using HTTPS for secure communication
Good security practices are essential for a reliable Async GraphQL backend.
Real-Time Data Handling
Async GraphQL can also handle live data updates through subscriptions:
- Clients ask the server to keep them updated with a subscription query.
- Whenever the data changes on the server, it sends the new info to the client.
- This data travels over a websocket, not the usual HTTP.
Here's a Rust example with Warp:
let schema = Schema::build(Query, Mutation, Subscription).finish();
let server = warp::serve(graphql(schema))
.websocket("/graphql")
.run(([127, 0, 0, 1], 3030))
.await;
The .websocket
part sets up a continuous connection.
Other tools like Apollo Server work similarly for subscriptions.
This feature lets Async GraphQL APIs send live updates to clients, making apps feel more responsive and up-to-date.
Common Challenges and Best Practices
Working with async GraphQL can be really helpful, but it also comes with its own set of challenges. Here's a look at some common issues you might run into and some smart ways to handle them:
Performance Tuning
- Too many DB hits - It's important to make sure you're not asking your database for data too often in a way that slows things down. Tools like DataLoader can help by grouping requests together.
- Bloated responses - Keep your schema well-organized and use limits on query sizes to prevent huge chunks of data from clogging up your server.
Testing & Debugging
- Hard to test - Make it easier to check if everything's working by pretending parts of your system are other parts (mocking). Use tests that check how the whole system works together too.
- Tough debugging - Use tools like Apollo Tracing to keep track of where things might be going wrong, like which resolver is taking too long or if there are any errors.
Architecture Choices
- Scaling difficulties - Plan for growing your system by making it easy to add more power when needed. Use different services and databases that can handle more load.
- Complex code - Keep things tidy by organizing code by the type of data it deals with. Make common tasks into services so you can reuse code and keep things simple.
Security Concerns
- Vulnerabilities - Stick to security best practices like limiting how complex queries can be and checking user inputs. Use tokens to check if users are allowed to do what they're trying to do, and protect sensitive data.
- Information leaks - Be careful not to show too much information when things go wrong or by letting everyone see how your data is set up.
Real-Time Apps
- State management - Keep track of data changes in a clear way. Use smart caching to update only what needs to be updated without redoing everything.
- Race conditions - Avoid problems where things don't happen in the order you expect by using version numbers or having a plan for what to do when data conflicts happen.
Getting good at async GraphQL means finding the right balance between speed and keeping things simple, while also making sure to test thoroughly, use tools to help see what's happening, and designing your API carefully. Following smart practices for security, caching, and how you set up your code is key to keeping everything running smoothly.
Conclusion
Using async GraphQL can really speed up your apps and make them handle lots of tasks at once. Here's what you get from it:
- Increased throughput - Your app can take on a lot more requests without getting bogged down.
- Faster response times - Users don't have to wait long to get answers.
- Simpler code - Writing your code with async/await is much cleaner than the old way with lots of callbacks.
- Efficient resource usage - An event-driven approach lets your app do more with less.
But, using async GraphQL also means you have to be careful about a few things. Here are some tips to keep your apps running smoothly:
- Plan for scale - Think about how your app can grow. Break it down into different parts if needed.
- Validate carefully - Always check the data coming in to stop any misuse.
- Use middleware - Adding tools can help you spot problems early.
- Test thoroughly - Make sure to test each part on its own and then all together.
- Monitor closely - Keep an eye on important info like how fast your app is responding and how much it can handle.
- Automate testing - Include tests in your update process to catch any issues early.
By following these steps, you can build strong, ready-for-anything async GraphQL backends.
Async GraphQL is great for making web and mobile apps that need to be fast and handle lots of users. If you build your apps the right way, you can make APIs that are quick, reliable, and ready for anything.
So, why wait? Try out async GraphQL and get your apps ready to handle anything smoothly. The future is all about doing things without having to wait, and now's the time to jump in!
Related Questions
What is GraphQL simply explained?
GraphQL is a way to ask for specific pieces of data from a website or app. Imagine you're at a restaurant with a menu that lets you pick exactly what goes on your plate, rather than choosing a pre-made meal. That's how GraphQL works. You get to ask for the data you want, and you don't get anything extra you didn't ask for.
It's a set of rules for asking for data. GraphQL lets you describe what kind of data you want and how you want it organized. The server then gathers that data from wherever it's stored and gives it back to you in the way you asked.
Why use GraphQL?
Here are some good reasons to use GraphQL:
- Precise data fetching - You only get the data you ask for, nothing more, nothing less. This stops you from getting too much or too little data.
- Powerful developer tools - GraphQL makes coding easier with features like auto-complete and instant documentation.
- Standardization - It gives everyone a common language for getting data, no matter where it's coming from.
- Flexible data access - You can get data from different places all at once, instead of making separate requests.
Overall, GraphQL makes it easier for developers to work and makes apps run faster.
What is the difference between REST and GraphQL?
Here's how REST and GraphQL are different:
- Request format - REST uses different paths like
/users
or/posts
to get data. GraphQL uses one path and you send your data requests there. - Data fetching - REST gives you set packages of data. With GraphQL, you pick exactly what you want.
- Versioning - REST needs new versions when things change. GraphQL just adds new fields without needing versions.
- Tooling - GraphQL has better tools for coding, like helping you write code faster and check your work.
In short, GraphQL lets you ask for just what you need, while REST gives you fixed bundles of data.
How does GraphQL work internally?
When you send a query to GraphQL, here's what happens:
- Parse query - Checks if your request makes sense
- Validate - Makes sure your request fits the rules
- Execute - Finds the data you asked for
- Build response - Puts the data together in the way you wanted
- Send response - Gives you the data back
Resolvers are the workers that find the data you want from databases or other places. The GraphQL server organizes this data to match your request and sends it back to you just the way you asked.