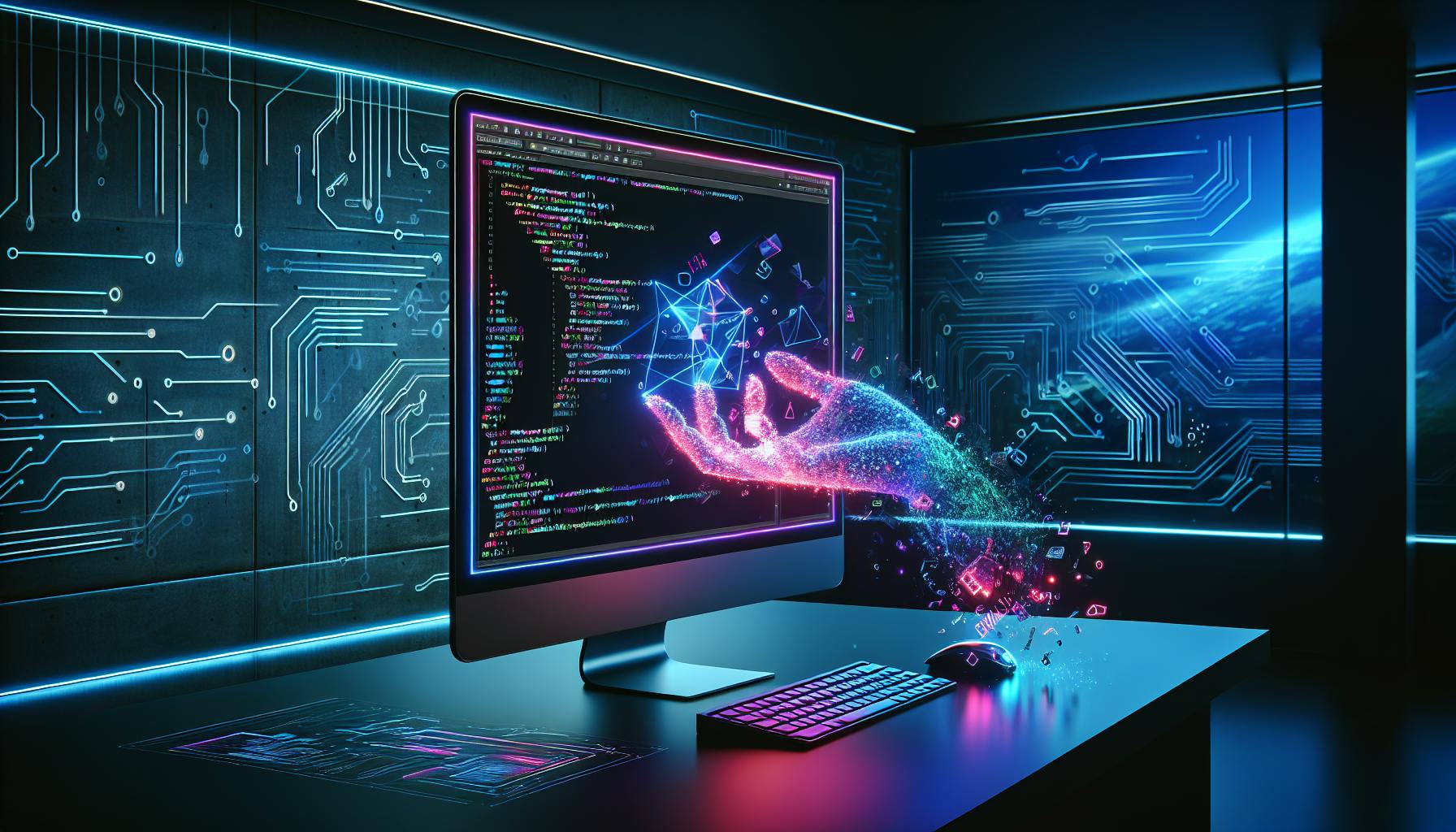
Learn how to use GraphQL mutations to change data on your website or app. Understand the basics, setup, error handling, and common use cases.
If you're curious about how to change data on a website or app using GraphQL, you've come to the right place. Here's a quick guide to understanding and using GraphQL mutations:
- Mutations are for making changes: Unlike queries that fetch data, mutations let you add, update, or delete data.
- Simple setup: Each mutation requires a name, some inputs, and specifies what data it returns.
- Use of variables: Variables add flexibility, allowing the same mutation to be used for different data changes without rewriting it.
- Error handling: Strategies like validation, try/catch blocks, and user-friendly error messages ensure robust mutations.
- Common uses: Creating new records, updating existing ones, and deleting data are standard mutation tasks.
This beginner's guide will walk you through everything from the basics of setting up mutations to dealing with errors and testing your changes. Whether you're adding a new blog post or updating user information, mutations make these tasks straightforward and efficient.
Prerequisites
Before diving into GraphQL mutations, let’s make sure you have everything you need:
GraphQL Server Setup
- You should already have a GraphQL server up and running. This guide won’t cover how to set one up from scratch.
- Your server needs to be ready to handle mutations with the right schema and resolver logic.
Understanding of GraphQL Concepts
- You should know how to fetch data using GraphQL queries.
- Understand the difference between queries (for reading data) and mutations (for changing data).
- Be familiar with how GraphQL schema and types work.
Development Environment
- Make sure Node.js and npm are installed on your computer.
- It’s good to use a code editor like VS Code to work on examples.
- Have git ready for downloading code examples.
- A terminal is necessary for running commands and starting your GraphQL server.
Testing GraphQL Mutations
- Tools like GraphQL Playground are great for trying out mutations.
- You can also use curl, Postman, or even write some client code to test them.
With these prerequisites in place, you’re all set to start learning about mutations in GraphQL. We’ll use these basics in the next parts to walk you through some examples. Let me know if there’s anything you’re not clear on!
Understanding GraphQL Mutations
Mutations in GraphQL let you change data on a server. They're different from queries, which get data without changing anything.
GraphQL Mutation Structure
Here's what a simple mutation looks like:
mutation {
updateUser(id: "1", name: "Jane") {
id
name
}
}
It includes:
- The
mutation
keyword - A name for the action, like
updateUser
- Details like the user
id
andname
you want to change - The data you want back, like
id
andname
, to see the update
The server uses the details you give to change the data, then sends back the new data in one go.
Comparing Queries and Mutations
Here's how queries and mutations are different:
- Purpose: Queries get data, mutations change data
- Side Effects: Mutations make changes, queries don't
- Return Values: Queries give back data that's already there, mutations give back the changed data
- Endpoints: They usually talk to different parts of the server
The big difference is that mutations change things, while queries just look at things without changing them.
So, remember, use queries when you want to look at data, and mutations when you need to update or change data. Both are important tools in GraphQL for handling data in a smart way.
Writing Your First Mutation
Let's try adding a feature to our GraphQL API that lets users change data on the server. We'll go through a simple example where we add a new blog post.
Adding a Blog Post
First up, we need to tell our GraphQL setup that we want to add new blog posts. Here's how you do it:
type Mutation {
createPost(title: String!, content: String!, author: ID!): Post
}
This line of code means we're setting up a new action, called createPost
, that needs a title, some content, and an author's ID. When it works, it gives back a new Post.
Now, we need to make this action actually do something. Here's the code that makes it work:
Mutation: {
createPost(_, { title, content, author }) {
const newPost = {
id: nextPostId++,
title,
content,
author
}
posts.push(newPost)
return newPost
}
}
This part is about creating a new blog post and adding it to our list of posts. It also makes sure the new post is sent back to whoever asked for it, so they get the new post's details.
Testing with GraphQL Playground
Testing your new mutation is easy with tools like GraphQL Playground.
Try out this mutation to add a new post:
mutation {
createPost(
title: "My new post"
content: "Here is my awesome content!"
author: "123"
) {
id
title
}
}
If everything's set up right, you'll see your new post pop up!
Testing like this is a good step to make sure everything works before you add it to a real app.
sbb-itb-bfaad5b
Using Variables in Mutations
Variables make your mutations flexible and powerful. Instead of writing the same details over and over in your mutations, you can use variables. This way, you can change the details each time you use the mutation without rewriting it.
Here’s why variables are so handy:
-
Reusability - You can use the same mutation for different tasks by changing the variables. For example, the
createPost
mutation can be used many times with different titles and content. - Safety - By separating the data from the mutation, you avoid accidentally changing the wrong data.
- Flexibility - You can update the variables without touching the mutation itself. This keeps your mutations easy to manage.
Declaring Variables
Here’s how you set up variables for your mutation:
mutation CreatePost($title: String!, $authorId: ID!) {
createPost(title: $title, author: $authorId) {
id
}
}
Think of variables like placeholders in your mutation. You list them at the start, and then you can use them inside the mutation.
Note: The !
next to the variable type means that it’s a must-have.
Passing Variables
When you run the mutation, you give the variables in a separate list:
{
"title": "My new post",
"authorId": "123"
}
This lets you use different values without changing the mutation.
Accessing Updated Data
You can also ask for data back using the new variables like this:
mutation CreatePost($title: String!, $authorId: ID!) {
createPost(title: $title, author: $authorId) {
id
title
author {
id
name
}
}
}
Using variables means you can keep using the same mutations in different situations. It’s a smart way to keep your GraphQL API clean and efficient.
Error Handling Strategies
When you're working with GraphQL mutations, it's super important to deal with errors the right way. Here are some tips to help you do just that:
Validation
Before you even start with a mutation, check the data you're about to use. This helps catch any mistakes early on:
function createPost(input) {
// Check the input
if (!input.title) {
throw new Error("A title is needed.");
}
// If all's good, create the post
}
This step stops problems before they start.
Try/Catch Blocks
Use try/catch to manage your mutations safely:
try {
// Try running the mutation
} catch (error) {
// If there's an error, handle it here
}
This way, you can deal with errors smoothly.
Return User-Friendly Messages
Make sure to give back messages that people can understand, not code jargon:
{
"errors": [
{
"message": "Sorry, there was a problem creating your post. Try again later."
}
]
}
Log Errors
Keep a record of errors to help fix any issues:
console.error(error);
Logging makes troubleshooting easier.
Global Error Handling
Have one place to catch and handle all errors:
app.use((error, req, res, next) => {
// Deal with errors for all routes here
})
This approach keeps your code clean and efficient.
By paying attention to how you handle errors, you make your app stronger and keep your users happy. These strategies for mutations ensure a smoother experience for everyone using your GraphQL API.
Common Mutation Examples
Mutations let you update the information on your server. Here are a few typical examples to show you how it's done.
Creating New Records
A common task with mutations is to add new information, like a new user or a blog post.
Here's a simple way to add a new user:
mutation {
createUser(name:"John", email:"john@example.com") {
id
name
email
}
}
To try this out:
- Use the mutation in GraphQL Playground
- Check your backend (like your database) to make sure the new user is there
And that's pretty much it!
Updating Existing Records
Mutations are also great for changing info you already have, like a user's email:
mutation {
updateUser(id: "123", email: "john@newemail.com") {
id
email
}
}
After you run this mutation:
- Make sure user 123's email is updated in your backend
- Use a query to fetch the user's data again and check the update
Deleting Records
You can also use mutations to delete information, like removing a user:
mutation {
deleteUser(id: "123") {
id
}
}
After doing this:
- Verify that the user is gone from your backend
- Do a query for all users to see that user 123 is no longer there
These examples show how mutations simplify changing data. You just define the actions you want, like adding, updating, or deleting information.
Conclusion
Think of GraphQL mutations as a super tool for changing data on your website or app. Here’s what we’ve learned:
- Mutations are for changes - They’re different from queries because they let you add, change, or remove data.
- They’re simple to set up - Each mutation has a name, some inputs, and tells you what it gives back.
- Variables make them flexible - By using variables, you can use the same mutation for many different tasks without rewriting it.
- Dealing with mistakes - Using strategies like try/catch, logging errors, checking inputs first, and having a one-stop error handler makes your mutations strong and reliable.
- What they’re used for - Common tasks include creating new records, updating existing ones, and deleting things you don’t need anymore.
Now you know the basics of using mutations in GraphQL to change data. This is just the start. As you get more comfortable, you can dive into more advanced topics like how to manage user logins, speed things up with batching, or keep your data fresh with caching.
Just remember, starting with the basics is always best. This guide has given you a solid foundation to get going with mutations and make your app more interactive.