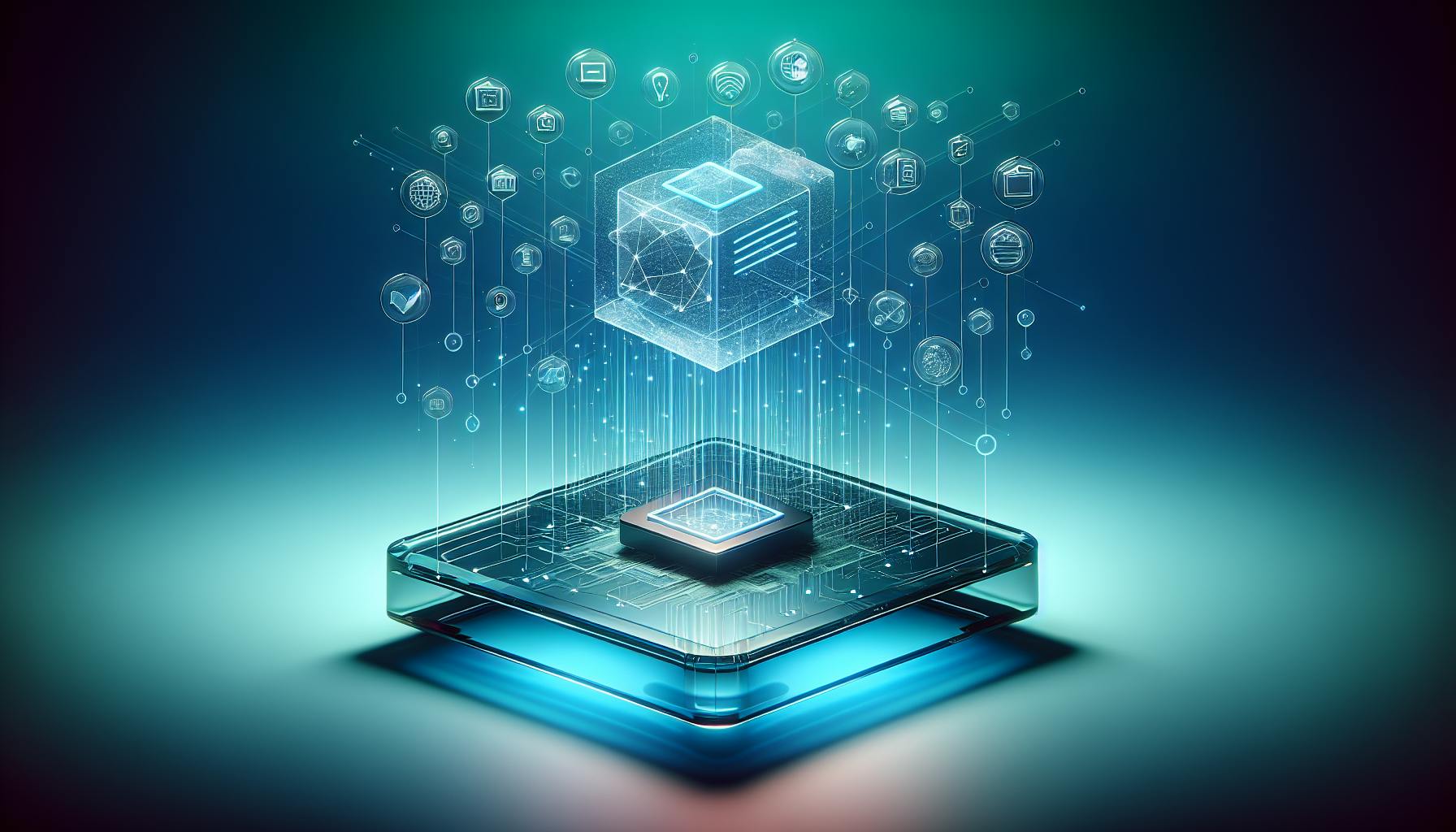
A comprehensive guide to understanding and using Webpack for web development projects. Learn about core concepts, setting up Webpack, advanced techniques, common challenges, and best practices.
Webpack is a powerful tool that simplifies the way web developers bundle and optimize their websites. Here's a quick guide to understanding and using Webpack:
- Webpack combines JavaScript files into fewer packages, making your website faster and more efficient.
- It handles not just JavaScript, but also CSS, images, and more, transforming them into a format that's ready for the web.
- Core concepts include Entry Points, Output, Loaders, Plugins, and Modes to manage how your files are bundled.
- Setting up Webpack involves organizing your project, installing Webpack, and configuring it according to your needs.
- Advanced techniques like code splitting and asset management can further optimize your site.
- Common challenges may arise, but understanding the basics can help you troubleshoot.
- Follow best practices for organization, performance optimization, and build analysis to make the most out of Webpack.
This guide aims to equip you with the knowledge to get started with Webpack, enhancing your web development projects with better efficiency and control over your code.
What is Webpack?
Webpack is like a big machine that takes all your website's pieces—like your JavaScript, CSS, and images—puts them together in a smart way, and makes them ready for the web. Here's how it does it:
- Entry Point: This is where Webpack starts. Think of it as the main door. It's the first file Webpack looks at, and from there, it figures out which other files are needed.
- Output: After Webpack has looked at all the files, it bundles them up into a few files that are easier for browsers to read.
- Loaders: Normally, Webpack only understands JavaScript. Loaders are like translators that let Webpack work with other types of files, like CSS or images, and turn them into JavaScript or something that works with JavaScript.
- Plugins: These are tools that do a bunch of different tasks, like making files smaller, organizing your files better, or adding some settings to your project.
- Mode: This tells Webpack whether you're building your site to test it out (development) or to put it live on the web (production). Each mode has its own settings to make sure your site runs its best.
Basically, Webpack takes all your files, figures out how they connect, changes them if needed, and bundles them into a smaller number of files that are faster for browsers to use.
Why Use Webpack?
Using Webpack is a smart move because:
- It's efficient: It bundles your code into fewer files. This means browsers load your site faster because they don't have to grab as many files.
- It makes your site faster: When you're ready to show your site to the world, Webpack makes your files as small as possible, so your site loads quickly. It also gets rid of any code you're not using.
- It handles more than just JavaScript: Webpack can deal with your images, CSS, and more, making it easier to get your site ready to go live.
- It makes coding nicer: With tools like the webpack-dev-server, you can see changes to your site right away without refreshing the page. Plus, there are loads of plugins to help you do all sorts of things more easily.
In short, Webpack makes building websites faster, more efficient, and a bit easier, especially when you have a lot of files and code to manage.
Core Concepts
Webpack's setup revolves around a few main ideas that help it package your website's files for the internet. Getting to grips with these concepts will make it easier to use Webpack for your projects.
Entry
Think of the entry point as Webpack's starting line. It's usually your main JavaScript file, like index.js, where you list all the things your website needs.
Webpack looks at this file first and then follows all the connections to other files and assets, putting together a big map of everything it needs to bundle. You can tell Webpack to start with just one file, or you can give it several starting points.
Output
The output is Webpack telling you where it's going to put the bundled files and what it will call them. You decide on a folder (like dist/) for the bundled files and how to name them.
Common naming patterns include using [name].js for the bundle's name, [hash] for a unique build number, or [contenthash] for a file-specific unique number.
Loaders
By default, Webpack can only understand JavaScript and JSON files. Loaders are like adapters that let Webpack handle other types of files (CSS, images, fonts, etc.) and turn them into modules that can be included in the bundle.
This means you can use a CSS file in your JavaScript code, for example. Some well-known loaders are css-loader and file-loader.
Plugins
Plugins are like helpers that work on the whole bundling process, not just individual files. They can do a lot of different things, like optimizing your bundle, managing assets, or adding environment variables.
Plugins give you more control over how Webpack bundles your files. Examples of helpful plugins are TerserPlugin for optimizing, HtmlWebpackPlugin for managing HTML files, and CleanWebpackPlugin for cleaning up your output directory.
Mode
Webpack lets you set a mode to tell it what you're preparing your bundle for: development, production, or none. Each mode has settings optimized for that situation.
For instance, development mode makes debugging easier, while production mode makes your code as small and fast as possible. These settings help make setting up Webpack a bit simpler.
Setting up a Webpack Project
Project Structure
When you start a project with Webpack, it's a good idea to keep your files organized in src
and dist
folders:
src
- This is where all your original code goes before Webpack bundles it. This includes your JavaScript, CSS, images, fonts, and more.dist
- After Webpack does its magic, it puts the finished, optimized code here. This is what you'll put on the web.
Keeping your files like this helps make sure you're only working with the code you need and not mixing it up with the finished product.
A common setup might look something like this:
my-app
|- src
| |- index.html
| |- index.js
| |- styles.css
|- dist
|- webpack.config.js
Installing Webpack
To get Webpack ready, start by setting up a new project with npm init
and then add Webpack:
npm init -y
npm install webpack webpack-cli --save-dev
The Webpack CLI lets you run Webpack from the command line.
Next, you'll want to pick up some tools Webpack uses, like:
- css-loader
- file-loader
- html-webpack-plugin
npm install --save-dev css-loader file-loader html-webpack-plugin
Configuring Webpack
Webpack uses a file called webpack.config.js
for its settings. Here, you tell it things like:
- Where to start bundling
- Where to put the finished files
- Which extra tools (like loaders and plugins) to use
Here's a simple webpack.config.js
to start with:
const path = require('path');
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
entry: './src/index.js',
output: {
filename: 'main.js',
path: path.resolve(__dirname, 'dist'),
},
module: {
rules: [
{
test: /\.css$/,
use: ['style-loader', 'css-loader'],
},
],
},
plugins: [
new HtmlWebpackPlugin({
template: './src/index.html'
}),
],
};
This setup:
- Starts with
index.js
- Puts the bundled code in
/dist/main.js
- Works with CSS files
- Creates an HTML file from a template
You can always add more tools and settings to match what your project needs.
Advanced Webpack
Let's dive deeper to make your code and work process even better.
Code Splitting
Code splitting is about breaking up your code into smaller pieces. This way, your website loads faster because the browser only grabs the bits it needs right away.
How to do it:
- Dynamic imports - You can pull in parts of your code only when they're needed by using a special command like
import('./myModule')
. This splits off that part into its own little package. - Entry points - You can set up different starting points for your code, which helps break it up into separate packages from the get-go.
Asset Management
This is all about handling stuff like pictures, fonts, and stylesheets. Webpack uses helpers called loaders to do this:
file-loader
- Helps bundle up images and fonts.css-loader
- Lets you include CSS in your JavaScript.style-loader
- Puts CSS right into the webpage.
Using these tools, you can make sure all your website's visuals get bundled up correctly.
Optimizing Performance
Making your website run faster and smoother is key. Here are some tricks:
- Tree shaking - This gets rid of code you're not using to make everything lighter and faster.
- Production mode - This is a special setting that makes your code as small and fast as possible for when your site goes live.
- Code splitting - As mentioned, splitting your code into chunks means the browser doesn't load unnecessary stuff.
- Compression - You can shrink your files down so they're quicker to download.
- Caching - This is a way to tell browsers to remember certain files, so they don't have to reload them every time.
By focusing on these areas, you can make your website load quicker and give visitors a smoother experience.
sbb-itb-bfaad5b
Common Challenges
Webpack is super useful but can be a bit tough to get the hang of at first. Here are some typical problems you might run into and how to fix them:
Loaders Not Working
Loaders let Webpack handle files that aren't JavaScript. If they're not doing their job, make sure:
- You've installed the loader right
- You've set up the loader test correctly for the kinds of files you're working with
- Your webpack config file actually uses the loader in the module rules
Plugins Misconfigured
Plugins help tweak how Webpack does its thing. Common mistakes include:
- Forgetting to
require()
the plugin or not adding it correctly - Giving the plugin the wrong options
- Using plugins that don't play nice with each other
Complicated Config
The Webpack config file can get really complex. To keep it manageable:
- Break your config into smaller files by function
- Use
webpack-merge
to put these smaller configs together - Comment your code to explain what's going on
Slow Builds
As your project gets bigger, Webpack might start to slow down. You can speed things up by:
- Cutting out code you don't need
- Using tools to see what's making your bundles big
- Turning on caching to save time
Getting Overwhelmed
Webpack has a lot of parts, which can feel overwhelming. Keep these tips in mind:
- Start with the basics and slowly add more as you learn
- Look at the docs if you're stuck
- Focus on understanding the main ideas before diving into more complex stuff
Take it one step at a time, build a strong foundation, and lean on the community and resources available to solve tricky issues.
Best Practices
Here are some simple ways to make your Webpack setup better and ready for showing off your website:
Keep Things Organized
- Break your settings into smaller pieces, like one for loaders and another for plugins. Use a tool called
webpack-merge
to put them back together. - Don't shy away from writing notes in your config to help you remember why you did something.
- Give your folders and files clear names so you know where everything is.
Optimize Performance
- Switch to
production
mode to make your code smaller and faster. - Turn on compression to make files smaller so they load quicker.
- Get rid of code you're not using with something called tree shaking.
- Keep code for libraries separate from your main code to speed things up.
- Use hashes in your file names (like
[name].[hash].js
) so browsers don't have to reload them if they haven't changed.
Analyze Your Build
- Find out what's making your files big with a tool called webpack-bundle-analyzer.
- Keep an eye on how long it takes to build your project and how big the files are with Speed Measure Plugin.
Cache Intelligently
- Store parts of your site that don't change much, like libraries or styles, so they load faster next time.
- Use
cache-loader
when you have transformations that take a lot of time, like using Babel.
Code Split Carefully
- Break your code into pieces based on different parts of your site or different components instead of just random chunks.
- Load parts of your site only when needed with something called dynamic imports.
- Only split your code if it actually makes your site faster to load.
Watch Mode
- Use
webpack --watch
to make small updates quickly during development. - For live updates as you code, use
webpack-dev-server
.
Following these simple tips will help you make a Webpack setup that's neat and fast for when you're ready to show your site to the world. It's all about keeping things tidy, making your site quick, remembering what changes you made, and making sure everything updates smoothly.
Additional Resources
If you're looking to get more familiar with Webpack, here are some easy-to-follow resources:
Official Documentation
- Webpack documentation - This is the go-to place to understand everything about Webpack. It's detailed and covers everything from start to finish.
Tutorials
- Webpack Academy - Offers free, step-by-step lessons on how to use Webpack. Perfect for those just starting out.
- Using Webpack in Vue JS - Shows you how to set up Webpack if you're working with Vue.
Tools
- webpack-bundle-analyzer - Helps you see and improve the size of your Webpack files.
- Speed Measure Plugin - Lets you check how fast your build is and how to make it faster.
Discussion Forums
- Webpack GitHub Discussions - A place to talk about Webpack, ask questions, and get help.
- Webpack StackOverflow - A community where people discuss their Webpack problems and solutions.
These resources are great for learning more, solving problems, and finding out how to make your Webpack setup better. Connecting with other Webpack users can also give you new ideas and tips.