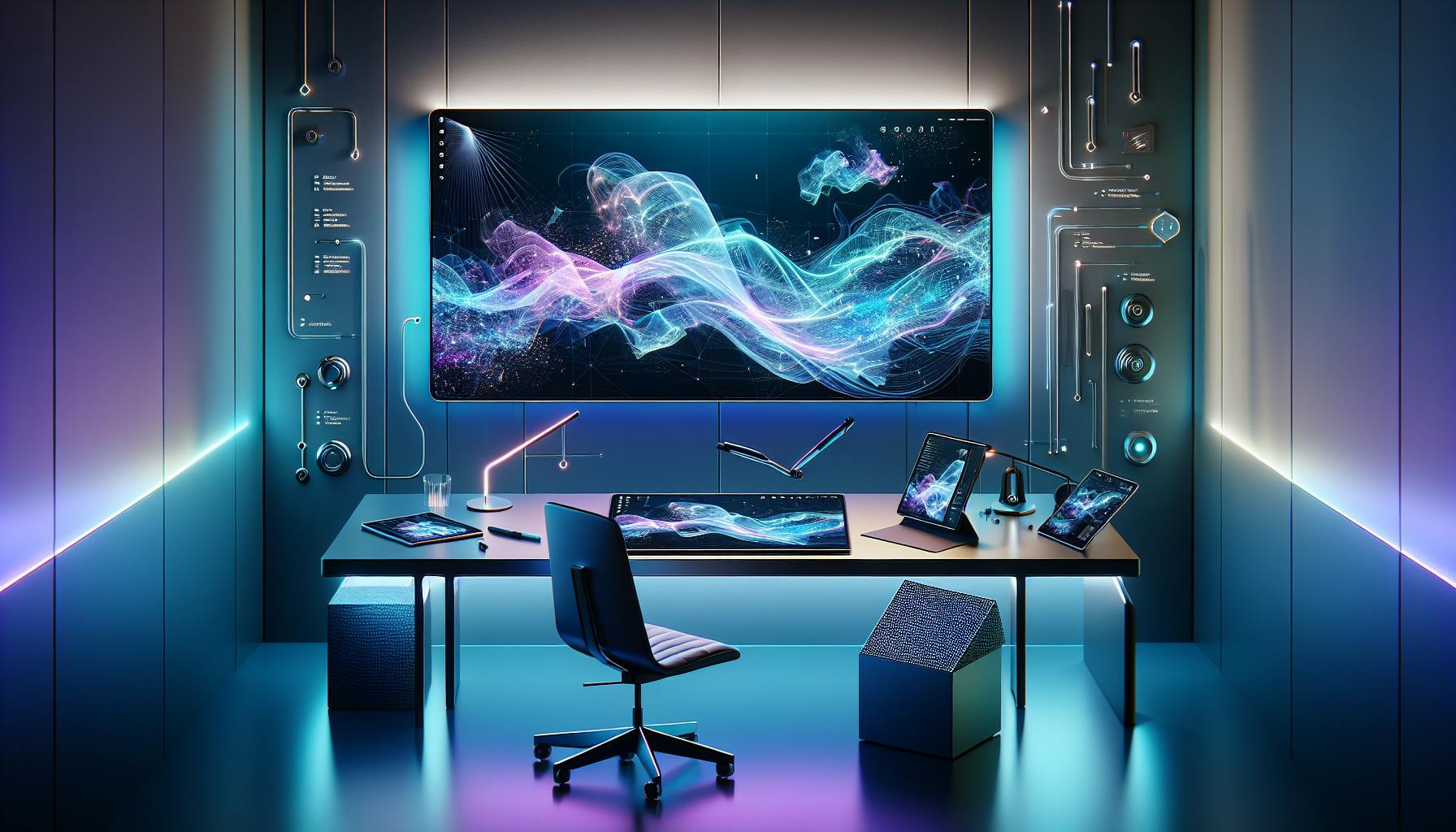
Explore the world of GraphQL extensions with this comprehensive developer's guide. Learn about types of extensions, benefits, implementation techniques, and key features for enhancing your GraphQL setup.
GraphQL extensions are powerful tools designed to enhance your GraphQL setup, making it more efficient and flexible. Here's what you need to know:
- What are GraphQL Extensions? They're add-ons that improve and extend GraphQL's capabilities, including query optimization, code efficiency, and adding new functionalities.
- Types of Extensions: Including schema, query, operation, and type extensions, each serving a different purpose in enhancing your GraphQL server or client.
- Benefits: Extensions offer improved query optimization, enhanced code efficiency, added flexibility, and encourage customization.
- Getting Started: To use extensions, you'll need a basic understanding of GraphQL, Node.js, and access to a GraphQL server setup.
- Implementing Extensions: From schema tweaks to custom directives and operation enhancements, extensions allow for a wide range of improvements.
- Advanced Techniques: Dive deeper with data modeling techniques, creating reusable extension packages, and building custom extensions to fit your specific needs.
- Key Features: Enjoy benefits like intelligent autocomplete, inline errors and warnings, as well as improved navigation and refactoring capabilities.
Whether you're new to GraphQL or looking to enhance your existing setup, GraphQL extensions offer a pathway to building more powerful, efficient, and customized applications.
Understanding GraphQL Extensions
GraphQL extensions are like add-ons that make GraphQL even better. They give you extra tools like making queries run faster, managing data better, and more.
What are GraphQL Extensions?
GraphQL extensions are like extra pieces you can add to your GraphQL setup. They let you do more with your GraphQL server or client, giving you ways to tweak and improve how your app works.
Some examples include:
- Schema extensions - These let you add rules or extra info to your GraphQL setup.
- Operation extensions - These change how queries and updates work on the server.
- Field extensions - These tweak how data fields work.
- Directive extensions - These are custom rules that change how things run.
Extensions are great because they let you add new features without having to redo everything.
Types of Extensions
There are a few main kinds of GraphQL extensions:
- Schema extensions - These add new stuff to the GraphQL language, like marking old fields as outdated.
- Query extensions - These make server queries work better, like by grouping queries together.
- Operation extensions - These change how queries and updates are handled.
- Type extensions - These let you use special data types, like dates or JSON.
You can mix and match these extensions to make your GraphQL server do exactly what you need.
Benefits of Using GraphQL Extensions
Using GraphQL extensions has some big perks:
- Improves query optimization - Extensions that help with caching and grouping queries make your app run faster.
- Enhances code efficiency - Using bits of code again (like fragments) and making custom rules cuts down on repeat work.
- Adds flexibility - Extensions let you update and improve your GraphQL without breaking it.
- Encourages customization - You can make extensions that fit your app's specific needs.
By making your development process smoother and your queries faster, extensions make GraphQL an even better tool for building apps. They fit right into your current setup, giving you new features without a lot of extra hassle.
Setting the Stage
Prerequisites
Before diving into GraphQL extensions, you should have:
- A basic understanding of GraphQLโhow it works and its key terms.
- Some experience with Node.js and using npm for managing packages.
- Access to a GraphQL server setup, like Apollo Server or something similar.
- A favorite code editor, such as VS Code, ready to go.
It's important to be comfortable with setting up a GraphQL schema and writing resolver functions. Also, make sure Node.js is installed on your computer.
Environment Setup
Here's how to get your project ready for adding GraphQL extensions:
-
Start a Node.js project - Type
npm init
in your terminal. -
Get the necessary packages - Install Apollo Server and GraphQL by running
npm install apollo-server graphql
. -
Prepare your schema - Create a
schema.graphql
file where you'll define your data types and the actions you can perform. - Write resolvers - These are JavaScript functions that fetch the data your queries ask for.
- Launch Apollo Server - Get your Apollo Server running with your schema.
-
Add extensions - Find and install any GraphQL extensions with
npm install
. -
Bring in extensions - Use
require
orimport
to add these extensions into your server code. - Set up extensions - Each extension might have its own setup instructions, so follow those to get them working with your server.
Once you have a basic GraphQL server up and running, you can start adding extensions. These usually involve tweaking your schema or how your server operates. Just follow the instructions that come with each extension, and you'll be able to integrate them into your project smoothly.
Implementing GraphQL Extensions
GraphQL extensions let you add cool features to your GraphQL server. Here, we'll show you some key ways to add different kinds of extensions.
Implementing Schema Extensions
Schema extensions are about tweaking your GraphQL setup without a total overhaul. They make adding new stuff or changing things up easier.
Here's how you can do it:
- Add new fields - You can stick new fields onto existing types with something called a directive. It's like saying, "Hey, let's also include this piece of info":
type User @addedField(field: "fullName") {
firstName: String
lastName: String
}
# Now, User also has a fullName field
- Mark deprecated fields - Sometimes, you stop using certain fields. Mark them as outdated with the
@deprecated
directive:
type User {
password: String @deprecated(reason: "Field no longer supported")
}
- Create interface extensions - Add new fields to interfaces, and all types using that interface will get the new field:
interface Searchable @addedField(field: "searchPreview") {
searchText: String!
}
type BlogPost implements Searchable {
title: String!
text: String!
}
# BlogPost now includes searchPreview field
Implementing Directive Extensions
Custom directives let you set up special rules or actions. They can help with:
- Access control - Decide who can see what based on their permissions.
- Data handling - Change or check data in certain ways.
- Instrumentation - Keep track of logs, metrics, and more.
Here's a simple directive to make text uppercase:
directive @uppercase on FIELD_DEFINITION
type Query {
hello: String @uppercase
}
# This will give you "HELLO WORLD"
{
hello
}
Implementing Operation Extensions
Operation extensions are about adding your own logic to queries and updates.
Things you can do include:
- Performance tracking - Keep an eye on how long things take to run.
- Error handling - Manage errors in a smart way.
- Usage analytics - See how often different parts of your app are used.
Here's how to use Apollo Server's tools to track how long requests take:
const { graphql } = require('graphql');
const { GraphQLExtension } = require('graphql-extensions');
class TimingExtension extends GraphQLExtension {
requestDidStart() {
console.time('graphql-request');
}
requestDidEnd() {
console.timeEnd('graphql-request');
}
}
const myGraphQLServer = graphql({
schema,
extensions: [() => new TimingExtension()]
});
sbb-itb-bfaad5b
Advanced GraphQL Extension Techniques
Data Modeling Techniques
When you're setting up a GraphQL API, it's important to organize your data in a way that makes sense and is easy to work with. Here are some strategies:
- Use interfaces for common features - If several objects have the same fields, group them under an interface. This saves you from repeating yourself.
- Use unions for mixed types - When you're not sure what type an object will be, use a union. This lets you handle multiple types more easily.
- Add new fields safely - With schema extensions, you can add new fields to existing types without causing problems. This keeps your API flexible.
- Think ahead - Try to plan for what your API might need in the future. This can save you from having to make big changes later on.
- Be flexible - Consider different scenarios where your API could be used. This helps you design a more versatile system.
By thinking ahead and organizing your data smartly, you can make your GraphQL API more adaptable and easier to update.
Extension Packages & Reuse
To share your custom GraphQL extensions with others:
- Make a package - Turn your extension code into a Node.js package.
- Write clear instructions - Explain how your extension works and how to use it.
- Share on npm - Put your package on npm so others can download it.
- Make key parts available - Make sure to export the important bits of your extension.
- Use in your projects - You can now include your extension in your projects by importing it.
- Set it up - Create an instance of your extension and add it to your GraphQL server settings.
By making your extensions available as npm packages, you make it easy for yourself and others to use them in different projects. Good documentation is key to helping others understand how to integrate them.
Building Custom GraphQL Extensions
If you want to create your own GraphQL extension:
-
Start with the base class - Use the
GraphQLExtension
base class as your starting point. -
Add your own logic - Change methods like
requestDidStart()
to do what you want. -
Work with context - You can access request details using
this.context
inside your methods. - Make it usable - Make sure your extension can be easily added to a GraphQL server.
- Add it to your server - Include your extension when setting up your Apollo Server.
- See it in action - Your extension will now run whenever your server handles requests!
By focusing on specific tasks and using the GraphQLExtension
class, you can create extensions that add useful features to your GraphQL server. Accessing request information through this.context
can give you valuable insights.
Key Features of GraphQL Extensions
GraphQL extensions give developers extra tools to make their GraphQL servers and clients better. Here are some of the top features:
Intelligent Autocomplete
Extensions can help by suggesting what to type next when you're writing GraphQL queries and mutations. They look at your schema, what you've already written, and the context to offer suggestions on fields and arguments you might need.
For instance, the GraphQL extension for VS Code looks through your project for schema definitions and operations you've already written. When you start typing a query, it suggests fields and arguments that make sense to include, based on what you've typed. This makes writing code quicker and helps avoid mistakes.
Inline Errors and Warnings
Extensions can check your queries and mutations as you write them, pointing out any problems right in your code, with clear error messages and warnings.
This means you can spot and fix issues straight away, without needing to run the operation first. It makes coding more efficient because you can correct errors as you go.
Navigation & Refactoring
When working on big GraphQL projects, moving around the project and making changes can be tricky. Extensions make this easier by improving how you navigate and safely change your code.
For example, you can quickly go to or look at the definition of a field, argument, or type by clicking or hovering in your editor. Extensions also make sure that when you rename types, you don't accidentally mess up other parts of your project.
This boosts how much you can get done and makes working with complex code less of a headache. Being able to move around quickly and change things without breaking anything helps keep your code clean and consistent.
Troubleshooting Guide
Schema Conflicts
Sometimes, your GraphQL schema might have parts that clash, like two different things using the same name. Here's how to fix that:
-
Use different prefixes for similar names to avoid mix-ups. For example, you could name types as
User_id
andPost_id
. -
If you find fields or types that clash, rename them to something more specific, like changing
id
touserUuid
orpostId
. - When combining schemas, use tools like Apollo Server to merge them without issues.
- Keep different parts of your schema in separate files or modules. This helps prevent overlap.
- Clearly explain the use of similar fields by adding comments. For instance:
type User {
id: ID! # Unique ID for a user
}
type Post {
id: ID! # Unique ID for a post
}
Type Mismatches
Type mismatches happen when the data type doesn't match what's expected. Here's how to solve this:
- Always double-check that the data types in your schema match what your resolvers return.
- Using TypeScript can help catch these mismatches early on.
- Add checks in your resolvers to ensure data types are correct, and throw errors if not.
- Sometimes, converting data types in your resolvers or using Apollo Server settings can fix mismatches.
For example, checking data types in a resolver:
const isValidDate = date => !isNaN(Date.parse(date));
const resolvers = {
Mutation: {
createPost(parent, args) {
if (!isValidDate(args.publishedOn)) {
throw new Error('publishedOn must be a valid date!');
}
// Create post
}
}
}
Broken Resolvers
If your custom resolvers aren't working right, here's what to do:
- Make sure the resolver functions match what your schema expects.
- Logging arguments and results can help you find where things are going wrong.
- Catch and handle errors properly in your resolvers.
- Test your resolvers one at a time in a tool like GraphQL playground.
- Writing tests for your resolvers can help catch issues before they become bigger problems.
- Debugging in Node.js lets you step through your resolver code to find bugs.
- Always check the input to your resolvers to avoid surprises.
- Comparing working and broken resolvers can help you spot differences.
Example of logging in a resolver:
const resolvers = {
Query: {
user(parent, args, context, info) {
console.log(args); // Logs: { id: '123' }
// Rest of resolver code
}
}
}
Testing Locally
To safely test changes to your GraphQL server or client:
- Work on a separate development branch. This keeps your main project safe.
- Make sure your local setup matches what you have in production.
- After making changes, restart your dev server and test everything.
- Use integration tests to check that everything works as expected.
- Test your changes in a staging environment before going live.
- Once everything's good, merge your changes into the main project.
Here's a simple way to manage testing:
// Start on a dev branch
$ git checkout -b dev
$ // Make your changes
$ npm run dev
$ // Test everything locally
$ // Ready to go live
$ git checkout main
$ git merge dev
$ npm run build
$ npm run start
This approach lets you try new things without risking your main project.
Conclusion
GraphQL extensions are like helpful tools that make your GraphQL setup better and more flexible. They focus on making things like asking for data, managing it, and updating your app smoother.
Here's why GraphQL extensions are so handy:
- They make your work easier by suggesting what to type next and showing mistakes right as you type.
- Your apps run faster because these extensions help with storing data smartly and making fewer requests.
- They cut down on repeated work by letting you reuse parts of your code.
- You get more control over who can see what data and how your app handles information.
- Updating and adding to your GraphQL setup becomes less of a headache.
If you're looking to add new features or just make your current setup better, diving into GraphQL extensions is a great idea. There's a whole bunch of them made by the community, and you can even make your own.
Using these extensions means you can create more powerful backends for your apps without messing up what you've already built. They also work well with tools you might already be using, like Apollo Server and Apollo Client.
So, if you feel like your GraphQL could do more, checking out extensions could be the next step to boost your project!