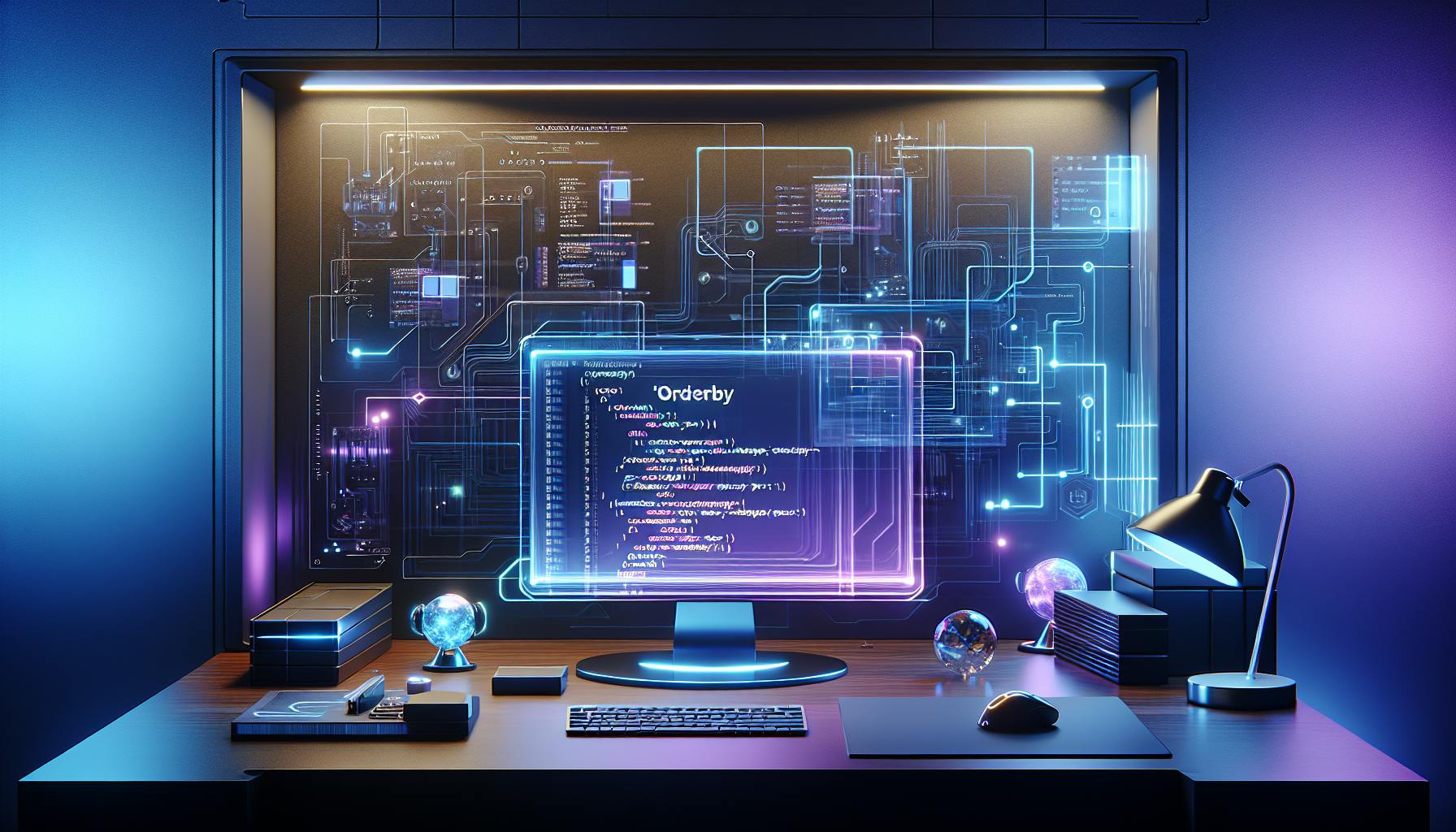
Learn how to effectively sort data in GraphQL using the `orderBy` argument. Enhance your apps with dynamic sorting, nested connections, and handling null values. Get started with a beginner's guide!
Sorting your data in GraphQL using the orderBy
argument can significantly enhance the usability and efficiency of your applications. Here's a quick guide to get you started:
- Why Sort Data: Organizing data helps users process information faster, understand context and relationships, and improves overall app performance.
- How to Use
orderBy
: Add theorderBy
argument in your GraphQL queries to sort data by specific fields, in ascending or descending order. - Sorting Different Types of Data: Whether it's scalar fields like titles and numbers, object fields like author names, or even computed fields like word counts, GraphQL allows for flexible sorting options.
- Setting Up Your Schema: Ensure your GraphQL schema includes
BookOrder
andSortingOrder
inputs to define sortable fields and their order direction. - Querying and Resolvers: When querying, specify your sorting preferences. On the backend, resolvers will handle the sorting logic based on your query.
Common Challenges and Solutions
- Ensure your schema correctly defines sortable fields.
- Only attempt to sort by fields that exist in your schema.
- Update resolvers to properly handle sorting logic.
- Watch out for performance issues, especially with large datasets or complex sorting criteria.
Advanced Tips
- Dynamic Sorting: Allow users to choose sort criteria at runtime for more dynamic data presentation.
- Nested Sorting: Sort data at multiple levels, like sorting authors by name, then their books by title and chapters by order.
- Handling Null Values: Decide how to treat null values in sorting, ensuring they don't disrupt the data order.
Sorting data effectively can make your GraphQL-powered apps more intuitive and responsive, enhancing the user experience.
Why Ordering Data Matters
Sorting your data right can make your app nicer to use in many ways:
- Scanning and Processing: People usually look at websites and apps from top to bottom and left to right. When your data is in a logical order, it's easier for them to find what they need.
- Context and Relationships: When you sort things like dates or names in order, it's easier to see how they relate to each other. This helps users understand the information better.
- Consistency and Predictability: If your app always shows data in the same order, it's easier for people to use. They know where to find things, which makes the app feel more reliable.
- Performance: If your database knows how to find sorted data quickly, your app can work faster.
Using orderBy
in GraphQL
To sort your data in GraphQL, you use the orderBy
argument in your query. Here's a simple example:
{
books(orderBy: TITLE_ASC) {
title
publishDate
}
}
This sorts books by their title from A to Z.
You tell GraphQL how to sort your data with a special setup in your schema:
input BookOrder {
title: SortingOrder
publishDate: SortingOrder
}
enum SortingOrder {
ASC
DESC
}
Here, you can choose to sort things in ascending order (ASC
) or descending order (DESC
).
You can sort by more than one thing to be more precise, like first by title, then by date.
Ordering by Scalar Fields
Sorting simple things like titles or numbers is easy:
{
books(orderBy: {title: ASC}) {
title
}
}
This works for basic data types in your schema, like dates or IDs.
Ordering by Object Fields
You can also sort by details inside other details, like this:
{
books(orderBy: { author: { name: ASC } }) {
title
author {
name
}
}
}
This sorts books by the author's name.
Ordering by Computed Fields
Sometimes, you can sort by things that are calculated, not just stored directly:
type Book {
title: String
wordCount: Int! @derivedFrom(field: content)
}
Then, you can sort books by how many words they have:
{
books(orderBy: { wordCount: DESC }) {
title
wordCount
}
}
This lets you sort in many flexible ways, making your app better for everyone using it.
Prerequisites
Before you start sorting your data with GraphQL queries, it's good to know a few basics:
GraphQL Schema Basics
Think of your GraphQL schema like a blueprint of your data. It shows what types of data you have, like books, their details (title, publish date, author), and how they connect. When setting up sorting, this blueprint tells you where you can apply it.
For instance, a Book
might look like this in your schema:
type Book {
title: String
publishDate: Date
author: Author
}
And you can decide to sort any of these fields.
Query Arguments
When you ask GraphQL for data, you can tweak your request with special instructions, called arguments, like orderBy
for sorting. Here’s a simple way to ask for books sorted by publish date:
query {
books(orderBy: PUBLISH_DATE_ASC) {
title
publishDate
}
}
Your schema must know these arguments are allowed.
Resolvers
Resolvers are like the backstage crew for your GraphQL queries. They find and organize the data you asked for. If you want your books sorted, the resolver makes sure they’re handed to you in the right order.
Here’s a peek at what a resolver might do:
Book: {
books: (parent, args) => {
return db.books.find().sort(args.orderBy)
}
}
So, knowing a bit about how your data is structured (schema), how to ask for what you want (query arguments), and how data is fetched and sorted (resolvers) is key to getting your data in order.
Step-by-Step Guide
Defining Your Schema
First, let's set up our schema to allow sorting. Imagine we're dealing with books. Here's how you might write it:
type Book {
title: String
publishDate: Date
author: Author
}
input BookOrder {
title: SortingOrder
publishDate: SortingOrder
}
enum SortingOrder {
ASC
DESC
}
What we're doing here is:
- Creating a
BookOrder
input type to say which fields we can sort by and how (ascending or descending). - Using
SortingOrder
to define the direction of sorting. - Making sure our fields like
title
andpublishDate
are ready to be sorted.
To sort by something more specific, like an author's name, you'd set it up like this:
input BookOrder {
author: AuthorOrder
}
input AuthorOrder {
name: SortingOrder
}
Now, we can sort books by who wrote them.
Adding 'Order By' to Queries
Here's how you'd ask for books sorted by title:
{
books(orderBy: {title: ASC}) {
title
}
}
We're telling the server to give us books in alphabetical order by their title.
If you want to sort a list of links by URL and then description, you'd write:
{
links(orderBy: {url: ASC, description: DESC}) {
url
description
}
}
This sorts links by URL first, then flips the order for the description.
Handling Multiple Criteria
When you sort by more than one thing, GraphQL looks at your first criteria, then uses the next one if there's a tie. For instance, if you're sorting books by author and then title, it first arranges them by author. If two books have the same author, then it looks at the titles.
Backend Considerations
On the backend, your server and databases need to work out how to sort things the way you asked. For books, a database command might look like this:
SELECT * FROM Books
ORDER BY author, title ASC
The resolver turns your query into this command.
Keep in mind:
- Sorting makes your server do more work.
- Using indexes on your database can make sorting faster.
- The more things you sort by, the slower it might get.
So, make sure you check how fast your queries run and try to make them as efficient as possible.
Common Pitfalls
When you're sorting data in GraphQL, there are a few common mistakes you might run into. Here's how to spot and fix them, so everything runs smoothly.
Not Setting Up Your Schema Properly
To sort data, your schema needs to be set up right:
- Make sure the fields you want to sort are included.
- You have to have
SortOrder
andBookOrder
ready to go.
If you miss this, sorting won't work because GraphQL won't understand what you're asking for.
Fix: Always double-check your schema before you start sorting.
Attempting to Sort by Non-Existent Fields
Sometimes, you might try to sort by fields that don't exist:
{
books(orderBy: {pages: ASC}) {
title
}
}
If there's no pages
field for Book
, this query won't do anything.
Fix: Make sure you're only trying to sort fields that actually exist in your schema.
Forgetting to Update Resolvers
Even if your schema is set, you need to make sure your server knows how to sort the data. If you don't update your resolvers, nothing will change.
Fix: Always remember to adjust your resolvers when you add sorting.
Performance Issues
Sorting can make your server work harder, especially if you have a lot of data. If not done carefully, it can slow things down.
Fix: Keep an eye on how fast your queries run. Use database indexes for fields you sort a lot. Try to sort only when you really need to.
Over-Sorting Data
Sorting by a lot of different things is useful but can also make things slow and complicated.
Fix: Stick to sorting by only the most important fields. Try not to sort by more than 2-3 fields in the same query.
By paying attention to these details and testing your queries, you can make sure your apps work well with orderBy
.
sbb-itb-bfaad5b
Advanced Tips
Dynamically Sorting Data
Let's say you're not sure how you want to organize your data until the last minute. GraphQL is cool because it lets you decide how to sort your data when you actually need it.
For instance, you might let your app users choose whether they want to see books sorted by their title or the author's name:
const orderBy = getUserSortingPreference() // 'title' or 'author'
{
books(orderBy: { [orderBy]: ASC }) {
title
author
}
}
This way, by using variables, you can sort your data however you want at any time.
Sorting Nested Connections
When you're dealing with complex data, you might need to sort different parts of it carefully:
type Author {
name: String
books: [Book]
}
type Book {
title: String
chapters: [Chapter]
}
{
authors(orderBy: {name: ASC}) {
name
books(orderBy: {title: ASC}) {
title
chapters(orderBy: {order: ASC}) {
title
order
}
}
}
}
In this case, we're doing three things:
- Sorting authors by their names
- Sorting their books by titles
- Ordering the chapters in each book
This method keeps everything organized at every level.
Dealing with Null Values
Sometimes, you might find some data missing (null values) when you're sorting:
{
books(orderBy: {title: ASC}) {
title // some titles might be missing
}
}
To keep these missing titles together, you can do something like this:
{
books(orderBy: {title: ASC_NULLS_LAST}) {
title
}
}
This way, any missing titles are put at the end instead of being scattered throughout your list.
The trick to sorting complex data is really understanding how your data is set up. Make sure your schema is well-thought-out and use resolvers to manage tricky situations like ordering, missing data, sorting different parts of your data, and changing how you sort on the go.
With a bit of practice, you can manage even the trickiest data sorting needs.
Conclusion
Sorting your data with orderBy
in GraphQL is a super useful trick. By this point, you've learned the basics of how it works and when you might want to use it.
Here are a few important points to keep in mind:
- Organizing your data makes it simpler to look through and understand. It's really helpful for making things clear and keeping them consistent.
- In your schema, you set up the rules for sorting with things like
BookOrder
andSortingOrder
. - When you write queries, you use
orderBy
to tell GraphQL how you want your data arranged. - The heavy lifting of sorting based on your query is done by resolvers on the server side.
- Be on the lookout for common snags like not setting up your schema correctly, trying to sort fields that don't exist, and running into slow performance.
- You can decide how to sort your data right when you're making the query, which gives you lots of flexibility.
- For data that's a bit more complicated, make sure to sort both the main items and their related parts carefully.
Now that you've got the basics down, why not try using orderBy
in some of your GraphQL projects? See how sorting can make your apps more user-friendly.
Here are a few project ideas to start with:
- Arrange blog posts by when they were published and their title.
- List products by price and how popular they are.
- Sort comments based on how many likes they have and when they were posted.
Have fun organizing your data! With orderBy
, you can explore all sorts of ways to make your data do exactly what you need.
Related Questions
How do you use order by in GraphQL query?
To sort the results in your GraphQL query, you can use the order_by
argument. This lets you organize your data by certain fields, either from top to bottom or vice versa. Here’s how:
{
books(order_by: {name: Desc}) {
name
author {
name(order_by: {age: Asc})
}
}
}
In this example, books are sorted by name in reverse order, while authors are sorted by age from youngest to oldest.
Is GraphQL an overkill?
GraphQL might seem like too much for simple needs, like if you're always using the same data in the same way. But for apps that need to get different pieces of data in various ways, GraphQL is really helpful. It stops you from getting too much or too little data. So, for bigger or more complex apps, GraphQL is just right.
How do I organize my GraphQL files?
It’s a good idea to split your GraphQL stuff—like types and resolvers—into separate files based on what they're about. For instance, put everything about "books" in a books.js file. Tools like graphql-modules
can help you combine these files into one big schema. This way, your code is neat and organized.
Is GraphQL harder than REST?
At first, learning GraphQL can seem harder than REST because there's more to know, like schemas, resolvers, and the query language. But, for apps that are a bit complex, GraphQL can make things faster and smoother since it solves common problems you run into with REST. So, even though it might take a bit more effort to learn, it's worth it in the long run.