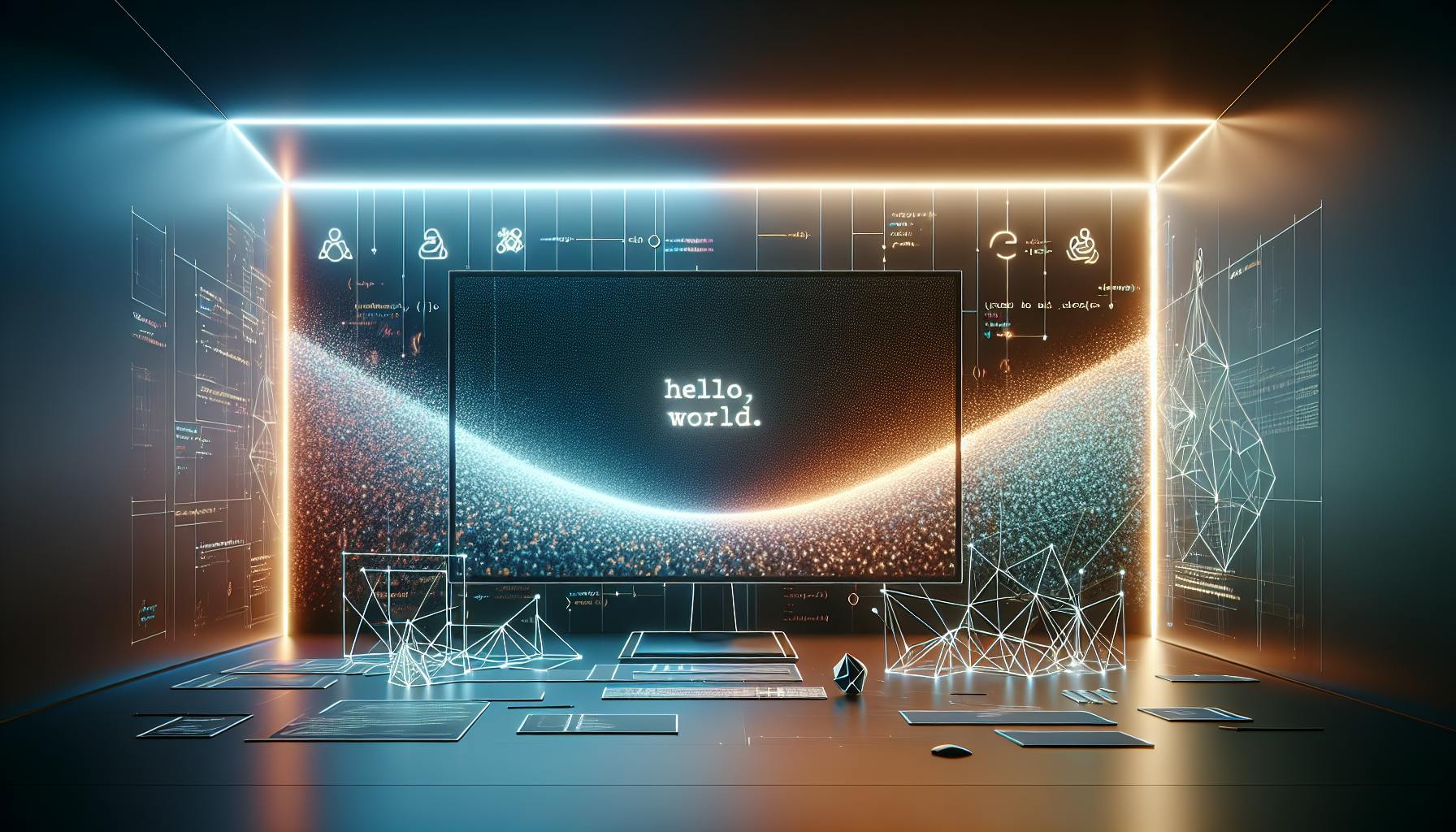
Learn how to start programming as a beginner with this guide on choosing your first language, understanding basic concepts, setting up your development environment, and more.
Starting programming as a beginner can feel overwhelming, but it's an exciting journey into a world of creating and problem-solving. Here's a straightforward guide to get you off the ground:
- Choose Your First Programming Language: Python and JavaScript are highly recommended for their simplicity and versatility.
- Understand Basic Concepts: Familiarize yourself with variables, loops, conditional logic, and functionsโ the building blocks of coding.
- Set Up Your Development Environment: Install a code editor like Visual Studio Code or Atom, and learn to use Git for version control.
- Start Small: Write simple programs, such as a 'Hello World' script or a basic calculator, to practice your skills.
- Learn and Practice: Utilize free online resources and platforms like freeCodeCamp, Codecademy, or edX for structured learning.
- Join a Community: Engage with other learners on platforms like GitHub, Dev.to, or Reddit to share knowledge and get support.
Remember, the key to becoming proficient is consistent practice and not being afraid to make mistakes. Every programmer starts somewhere, and with patience and persistence, you'll see your skills grow.
Breaking Down Programming Basics
When you're starting out with programming, understanding a few basic ideas can really clear up how coding works. It's like learning the alphabet before you write a story.
Key Ideas for New Programmers
Let's go over some simple concepts that all coding languages use:
Variables
Think of variables as boxes where you keep information for your program. You name each box, and you can change what's inside it anytime. Boxes can hold different kinds of stuff - numbers, words, or even yes/no answers.
Data Types
Data types tell you what kind of stuff you can put in your variable boxes. Here are some examples:
- Integers - These are whole numbers like 1, 20, or 45.
- Strings - These are pieces of text, like "Hi there!". You put them in quotes.
- Booleans - These are just yes (true) or no (false) values. They're great for making decisions in your code.
Conditional Logic
This is how your program makes choices. It's like saying, "If it's sunny, wear sunglasses. If not, don't." Based on what's true, your program will do different things.
Loops
Loops are a way to do something over and over again without writing it out each time. If you have a list of items, you can use a loop to go through each one.
Functions
Functions are like little helpers in your code. You set up a function to do a task, and then you just call it by name whenever you need that task done. It keeps your code neat and makes tasks reusable.
Starting with the Basics
When you're just learning how to start coding as a beginner, play around with these concepts. Try changing what's in your variables, use conditional logic to make decisions, loop through lists, and organize your code with functions. Getting comfortable with these basic building blocks will make moving on to more complicated stuff a lot easier.
Roadmap to Starting Your Programming Journey
Starting to learn coding can feel like a lot at first. Here's a simple guide to help you begin:
Choose Your First Language
It's best to start with just one language. Python and JavaScript are great first choices:
- Python - It's easy to understand and can be used for many things like web apps and AI.
- JavaScript - It makes websites and web apps work and has lots of users to help you out.
Java, C#, and Ruby are also good for starters. Pick one that seems interesting to you.
Set Up Your Coding Environment
You need a place to write your code, like the text editors Visual Studio Code or Atom. Download and set up the one you like, along with any extras it needs for your language.
Learn to use Git for keeping track of your code changes and GitHub for sharing your projects.
Learn Key Programming Concepts
Understand the basics like:
- Variables for keeping data
- Conditional logic for making choices
- Loops for doing things over and over
- Functions for organizing and reusing code
There are lots of free courses online that can teach you these important parts.
Write Your First Program
Begin with something simple, like showing "Hello World!" or making a basic calculator. It's okay if it's not perfect right away. Keep trying, watch tutorials, and ask for help. Learning to code needs time and patience.
Keep practicing, and you'll start to get the hang of it. This is your first step into a world full of coding possibilities.
Choosing Your First Programming Language
Why Python is a Top Choice for Beginners
Python is often the first language people learn because it's simple and easy to read. Here's why it's good for starters:
- Easy to read and understand - Python's code looks a bit like English, which makes it easier for beginners to understand what's going on.
- High-level abstraction - Python does a lot of the complicated stuff for you, so you can focus on learning how to program without getting too bogged down in details.
- Rapid testing - You can quickly test out your Python code, which helps you learn faster because you can try things and see results right away.
- Versatile - You can use Python for all sorts of projects, from websites to data analysis. This keeps learning fun and interesting.
JavaScript: The Gateway to Web Development
If you want to make websites or web apps, JavaScript is a great place to start. Here's why:
- It's what makes websites interactive and fun to use. Knowing JavaScript is key if you want to work on website design.
- There's a big community of people who use JavaScript, so you can find lots of help and resources.
- Knowing JavaScript can help you get a job since a lot of companies need people who can work with it.
Java: A Versatile Language for Backend and Mobile
Java is also a good choice for beginners, especially because:
- Portability - Java works on all kinds of devices, which means you can write code that runs everywhere.
- Back-end web - Java is used a lot for the parts of websites that you don't see but that make everything work.
- Android - Most apps on Android phones are made with Java, so learning it means you can make mobile apps.
- Promotes good practices - Java helps you learn to code in a disciplined way, which is a good habit.
Which Programming Language Should I Learn First as a Beginner?
There's no single "best" language to start with. Think about what you want to make, then look at what languages can help you do that. Also, consider how easy it is to find learning resources and if knowing the language can help you get a job. Some good ones to think about first are:
- Python
- JavaScript
- Java
- C#
- Ruby
The key is to pick one and start practicing. The basic ideas you learn will help you no matter what language you try next.
Setting Up Your Development Environment
Installing Python and Exploring IDLE
To start coding in Python, you first need to put it on your computer. Here's how to do it:
- Visit python.org and grab the latest version for your computer. Remember to let Python be part of your system's PATH when you're setting it up.
- Open the IDLE program that comes with Python. It's a basic place where you can write and run Python code.
- Try typing
print("Hello World!")
and run it. Congratulations, you've just made your first Python program!
IDLE is simple, but it's good for getting the hang of Python.
Choosing the Right Code Editor for Your Needs
IDLE is okay to start, but most people who write code prefer tools with more features, like:
- Highlighting code in different colors
- Helping you finish lines of code
- Letting you change its look
- Adding extra tools to make coding easier
Some good choices are:
- Visual Studio Code: It's free and you can change it a lot. Great for Python.
- PyCharm: Has everything you need for Python, but it's a bit more advanced.
- Atom: Made by GitHub, you can change a lot about it. Lots of extra tools.
- Sublime Text: Fast and good at handling text in smart ways.
Try some out and see which one feels right for you.
Essential Tools for Modern Programming
Besides a text editor, here are some tools that can help:
- Git: Keeps track of changes in your code. Very important for coders.
- npm: If you're making websites, this helps manage JavaScript tools.
- Make: Helps automate the process of building programs.
Knowing these tools makes you a better programmer.
Creating a Productive Coding Environment
To make coding easier:
- Pick an editor you like and set it up to help you work better.
- Keep your project files organized.
- Add tools to your editor that help check and fix your code.
- Keep guides for your coding language close by.
- Make sure your desk and computer setup is comfortable.
- Don't forget to take breaks and drink water.
Small things like these can make coding a lot more enjoyable.
sbb-itb-bfaad5b
Understanding Programming Fundamentals
This part talks about the basic ideas in programming like variables, data types, making choices with code, and doing things over and over again.
Variables and Comments: The Building Blocks of Code
Think of variables as little containers for keeping information. You name each one, and you can change what's inside whenever you want. Here's a simple example in Python:
my_name = "Ada" # This is a note to yourself
print(my_name)
Notes in your code, called comments, help you remember what your code is supposed to do. They're not part of the running code.
Data Types: Strings, Integers, and Beyond
Data types are like labels that tell the computer what kind of data you're working with. Some common ones include:
- Integers - Whole numbers without decimals.
- Floats - Numbers with decimals.
- Strings - Text. Always in quotes.
- Booleans - Just true or false. Great for making choices.
Choosing the right data type is key for your code to work right.
Making Decisions with Conditional Statements
Conditional statements let your code do different things based on whether something is true or not. Here's how you can use an if
statement in Python:
age = 20
if age >= 18:
print("You can vote!")
And you can use else
for when the if
part isn't true:
age = 16
if age >= 18:
print("You can vote!")
else:
print("You cannot vote yet.")
Looping Structures: Repeating Actions in Code
Loops are super handy for running the same code many times. A for
loop goes through a list one by one:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
A while
loop keeps going as long as something is true:
count = 0
while count < 5:
print(count)
count += 1
You can use break
and continue
to have more control over your loops.
Crafting Your First Coding Project
Planning Your Project: From Idea to Execution
When you're ready to start your first coding project, keep it simple. Think about a simple program you want to make. This could be a game where you guess numbers, a basic calculator, or a story game you play by choosing options. Write down your ideas and what you want your program to do. Start small, with just one file, and you can make it more complex later. Having a clear plan and knowing what you want to make will help you succeed.
Designing Program Logic with Pseudocode
Before you start coding, it's a good idea to plan out your program. You can do this with pseudocode, which is like a draft of your code written in simple language. For example:
Set a secret number to a random number between 1 and 100
Keep asking for the user's guess
If the guess is right:
Say "You win!"
If the guess is too high:
Say "Too high, try again"
If the guess is too low:
Say "Too low, try again"
This helps you think about how your program will work. Drawing diagrams can also help you see how different parts connect.
Implementing Your First Python Application
Now, using your pseudocode as a guide, you can start writing your first Python program. Let's make a simple game where the player guesses a number:
- Use the random library to pick a number
- Use a loop to keep asking for guesses
- Give hints if the guess is too high or too low
- Congratulate the user if they guess correctly
Test your code often to make sure it works. If you're stuck, look for help online.
Debugging and Refining Your Program
Try your program with different inputs to find any mistakes. If something doesn't work:
- Use
print
to see what's happening in your code - Look up any error messages you get
- Check your code step by step to find where things go wrong
After your program works, you can make it better by cleaning up your code. This means making it run faster, easier to read, or more efficient. This is a great way to learn and improve.
With some hard work and patience, you'll be able to make a complete program from scratch!
Expanding Your Programming Knowledge
Once you've got the hang of the basics in coding, there's a bunch of free stuff online to help you get even better. Here's where you can look:
Online Learning Platforms
There are websites that let you learn more about coding for free:
- freeCodeCamp: Offers courses on things like making websites and analyzing data. You can also get certificates.
- Codecademy: Has interactive courses on more than 10 programming languages. You can pick what you want to learn.
- edX: Offers college-level courses in computer science and programming from big-name schools.
- Coursera: Focuses on specific skills and offers certificates in coding.
Coding Challenge Websites
Get better at coding by solving challenges and puzzles:
- Codewars: Solve problems, see how others did it, and move up levels.
- HackerRank: Practice coding and get ready for job interviews.
- Project Euler: Tackle math and computing problems with your coding skills.
Developer Communities
Meet other coders to ask questions, work together, and learn:
- GitHub Discussions: A place to ask questions and get help on GitHub.
- Dev.to: Read blog posts and talk with other developers.
- Hashnode: A blogging platform for developers to share and learn.
- Reddit: Subreddits like r/learnprogramming are good for coding advice.
The cool thing about coding is there's always something new to learn! Joining a community can also help you keep growing.
Conclusion and Next Steps in Your Programming Adventure
Recapping the Journey: From Basics to First Program
We've gone through the basics of how to start programming as a beginner, including:
- Picking your first programming language like Python or JavaScript
- Getting your computer ready with the right tools and editors
- Understanding key ideas such as variables, loops, and how to make decisions in your code
- Making a simple program by planning it out first
- Fixing any mistakes and making your code better
Finishing your first small project is a big deal. It shows you can stick with it and solve problems.
Continuing Education: Pathways to Advanced Learning
To get even better at coding:
- Keep practicing by doing online courses, tackling coding puzzles, and working on your own projects
- Hang out online where other coders are, to ask questions and share what you know
- Show off your work in a portfolio
- Think about more formal education like a computer science degree or a coding bootcamp
Make a habit of coding a little every day. It's more effective than cramming all at once.
Becoming a Good Programmer: Practice and Persistence
Becoming great at coding is a long road that needs:
- Not giving up when things get tough
- Breaking down big challenges into smaller steps
- Always looking to learn more and get better
- Being patient, creative, and logical
- Asking for help when you need it and using resources like forums or documentation
- Looking back at your code to see if you can improve it
Keep at it because you enjoy solving puzzles and building things. Skills will come with time and practice. Remember to help others along the way and have fun learning.
Related Questions
What is the first step to learn coding for beginners?
The first thing to do when you're new to coding is to figure out why you want to learn it. Knowing your motivation helps keep you going. Start with easy-to-use tools and programming languages like Python or JavaScript. Then, try making a simple project to get the hang of basic coding stuff like variables, loops, and functions. Going step by step and having clear goals will help you get better at coding.
What should a beginner coder start with?
Python is a great choice for beginners because its syntax is easy to read, almost like English. This makes it less intimidating for new coders to grasp programming concepts. Plus, Python is flexible for various projects and enjoyable to work with.
What is the first step to becoming a programmer?
The first big step is usually getting a proper education, like a bachelor's degree in computer science or a related field. While it's possible to become a programmer without a degree, having one can make you more attractive to employers and provide you with important basic knowledge.
Can I teach myself to code?
Absolutely, you can teach yourself to code using online courses, YouTube tutorials, programming blogs/books, or other learning resources that fit your learning style. Make sure to practice coding regularly and work on projects to strengthen what you've learned. Keep pushing through the tough spots when learning on your own.