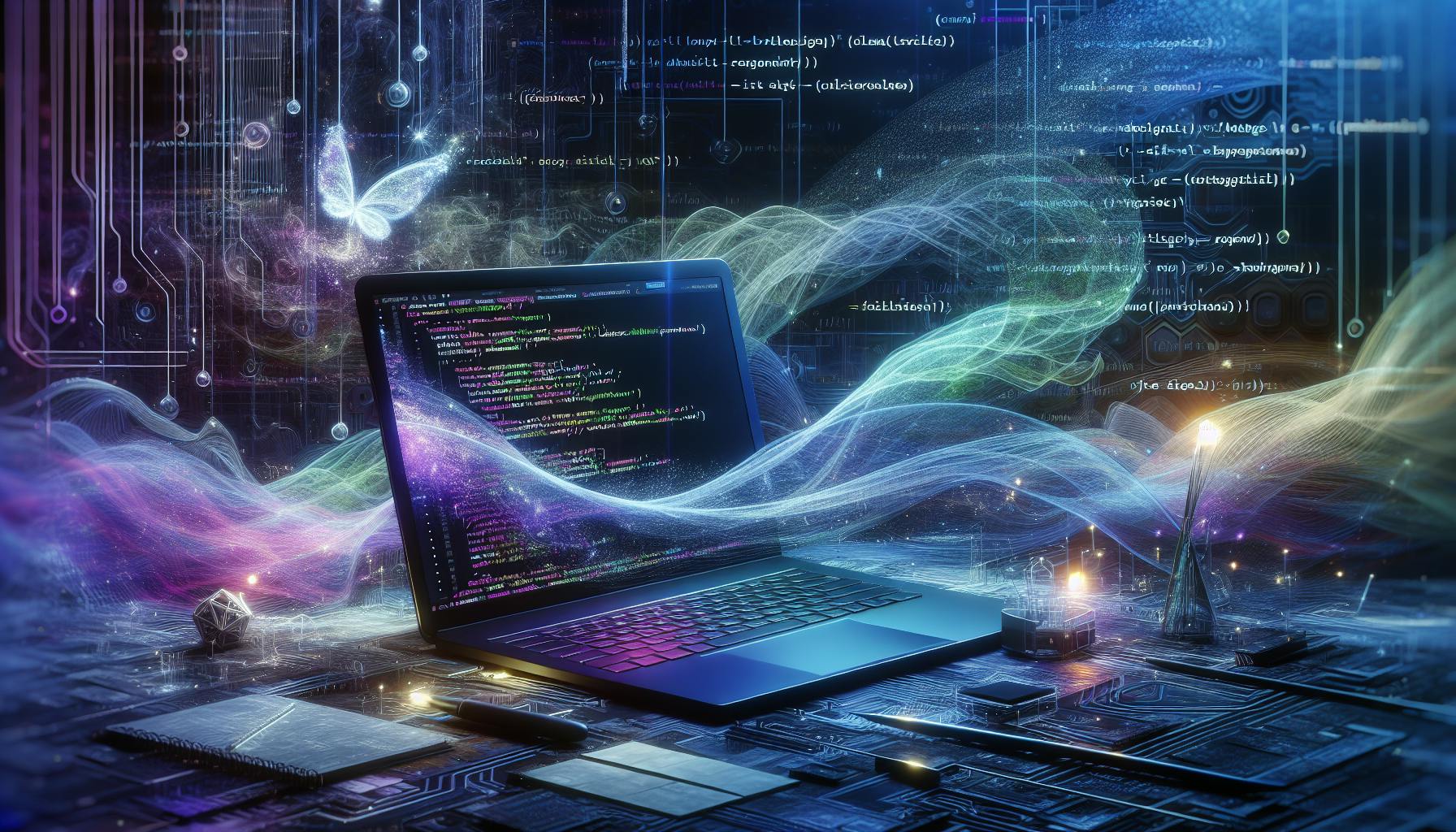
Learn how to integrate HTML and JavaScript to create interactive web pages. Understand the <script> tag, implement functions, include libraries like jQuery, and ensure accessibility. Start making your website more engaging!
Integrating HTML and JavaScript transforms static web pages into interactive experiences. This guide simplifies the process, covering the essentials on how to make your web pages come alive with JavaScript. Here's what you'll learn:
- Understanding the
<script>
Tag: Learn to include JavaScript in your HTML using the<script>
tag either by embedding code directly or linking to an external file. - Implementing JavaScript Functions: Discover how to create and invoke functions to perform tasks like displaying the current time or validating email input.
- Including JavaScript Libraries: Enhance your pages by incorporating libraries such as jQuery for additional functionality.
- JavaScript and Accessibility: Ensure your website is accessible to all users by following best practices for navigation, text alternatives, and more.
By the end of this guide, you'll have a solid foundation for making your web pages interactive using HTML and JavaScript, while also ensuring they are accessible to a broad audience.
Understanding the <script>
Tag
The <script>
tag is a way to include JavaScript code on a webpage. This lets the browser run JavaScript code, which can make web pages interactive and dynamic.
You can use the <script>
tag in two main ways:
- Directly write JavaScript code between the opening and closing
<script>
tags. Like this:
<script>
console.log('Hello World!');
</script>
- Link to an external JavaScript file with the
src
attribute. Like this:
<script src="script.js"></script>
It's a good idea to put <script>
tags in the <head>
or at the beginning of the <body>
to help your page load and run smoothly.
Adding JavaScript Code Examples
Here's how you can write JavaScript right inside <script>
tags to show the current time:
<script>
let date = new Date();
let time = date.toLocaleTimeString();
document.getElementById("time").innerText = time;
</script>
And here's how you link to an external .js
file:
<script src="time.js"></script>
In the time.js
file, you would have:
let date = new Date();
let time = date.toLocaleTimeString();
document.getElementById("time").innerText = time;
This approach keeps your JavaScript separate from the HTML, making things neater and easier to manage.
Implementing JavaScript Functions
JavaScript functions are like handy toolboxes that let you group together pieces of code to do a specific job. Think of them as mini-programs that you can run over and over again.
To make a function in JavaScript, you do this:
function functionName() {
// code to do something
}
And when you want to use your function, you just call it like this:
functionName();
Functions are great for making web pages interactive. For instance, if you want something to happen when a user clicks a button, you can use a function like this:
<button onclick="buttonClicked()">Click me</button>
function buttonClicked() {
alert('You clicked the button!');
}
Let's see a real-life example - checking if an email address looks right when someone fills out a form:
<form onsubmit="return validateEmail()">
<input type="email" id="email">
<button type="submit">Submit</button>
</form>
function validateEmail() {
let email = document.getElementById('email').value;
if (!email.includes('@') || !email.includes('.')) {
alert('Please enter a valid email address');
return false;
}
}
This code looks at the email when the form is submitted. If it doesn't have an '@' or a '.', it tells the user the email isn't right and stops the form from sending.
Including JavaScript Libraries
JavaScript libraries like jQuery give you extra tools to use in your code. To add one from a CDN (a place on the internet where you can get code):
- Put a
<script>
tag with the CDN link in your HTML:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
- Now you can use jQuery in your code:
$('button').click(function(){
alert('You clicked a jQuery button!');
});
Libraries like jQuery have lots of ready-to-use functions that can make coding easier and faster.
sbb-itb-bfaad5b
JavaScript and Accessibility Tips
When you're mixing JavaScript with your website, it's really important to make sure everyone can use it easily. Here's how to keep your site friendly for all users:
Make sure everyone can navigate
Some people can't use a mouse, so your site should work well with just a keyboard. Here's what you can do:
- Use
tabindex
to help with moving around the page with the tab key - Make your own controls easy to use without a mouse
- Check that forms and buttons work with the
tab
andenter
keys
Add text for images and videos
People who use screen readers need text to understand what's on your site:
- Put
alt
text on images - Offer text versions for audio or video content
- Use
aria-label
for parts of your site that are complicated
Let users zoom in
For those who need to see things up close, make sure your site doesn't break when they zoom in. Stay away from fixed sizes that could mess up the layout.
Look for accessibility issues
Checking your site can help you find and fix problems. Some tools that can help are:
- Lighthouse - checks for accessibility problems
- aXe - spots things that might cause trouble
- NVDA - a screen reader that lets you experience your site as someone with visual impairments might
Stick to web standards
Using the right HTML elements and ARIA roles helps screen readers understand and talk about your content the right way.
Making your JavaScript work for everyone is about paying attention and testing. If you do it right, you'll make a site that's great for all users.
Conclusion
Mixing JavaScript with HTML is key to making websites that do cool stuff. Here's a simple way to put them together:
- Use the
<script>
tag to either write JavaScript right on your page or to link to a JavaScript file. - Make functions in JavaScript to do things like reacting when someone clicks a button.
- You can also use tools like jQuery to make your job easier when changing stuff on your page.
- And don't forget, it's super important to make sure your website is easy for everyone to use.
With these basics, you're ready to start making your web pages way more interesting with JavaScript. You can add things like moving pictures, checking forms to make sure they're filled out right, and lots more.
As you keep learning, focus on simple but important ideas like:
- The Document Object Model (DOM) API, which lets you change page elements
- Listening for things like mouse clicks to run your code
- Using data attributes and storage to keep information
- Learning about promises and how to make your page work smoothly without waiting around
And remember, looking up how to do stuff online and practicing is a great way to get better. With some time and effort, you'll be able to make websites that are not only fun but also really useful.
HTML and JavaScript working together is what makes today's web so cool. Getting good at using both will let you turn your creative ideas into reality, one step at a time.
Related Questions
How do you integrate JavaScript with HTML?
To mix JavaScript with HTML, you can use the <script>
tag. Place it in either the head or body part of your HTML document. Here's how it looks:
<script>
// Put your JavaScript code here
</script>
Or, you can link to a JavaScript file from your HTML using the src
attribute like this:
<script src="script.js"></script>
This method keeps your JavaScript code separate from your HTML, making your code cleaner.
How is HTML and JavaScript connected?
HTML and JavaScript are linked through something called DOM (Document Object Model) manipulation. HTML sets up the page's structure and content. JavaScript can change the DOM to update the page content without needing to reload it.
For instance, if you press a button, it might activate a JavaScript function that hides a part of the page. This is how HTML content and JavaScript actions are connected.
Can HTML and JavaScript be used together?
Absolutely, HTML and JavaScript are often used together. HTML lays out the page, while JavaScript makes it interactive. Modern websites use both to:
- Change content without reloading
- Check forms for errors
- Make menus and buttons interactive
- Create apps that run in your browser
- Connect to services over the internet
- Animate page elements
Basically, for anything on a website that moves or changes, HTML and JavaScript are working together.
How to interact JavaScript with HTML?
JavaScript interacts with HTML in several key ways:
- It uses the DOM API to get, update, and play with HTML elements
- It can handle events, like clicks, directly in the HTML with things like
onclick
- It selects elements using
document.getElementById
orquerySelector
- It can change HTML content with
innerHTML
Here are some simple examples:
// Change the text of a paragraph
document.getElementById("p1").innerHTML = "New text!";
// Make something happen when a button is clicked
button.onclick = displayAlert;
// Get the value from an input box
let name = document.querySelector("#name").value;
With JavaScript, you can make your website do a lot of cool things by manipulating the HTML.