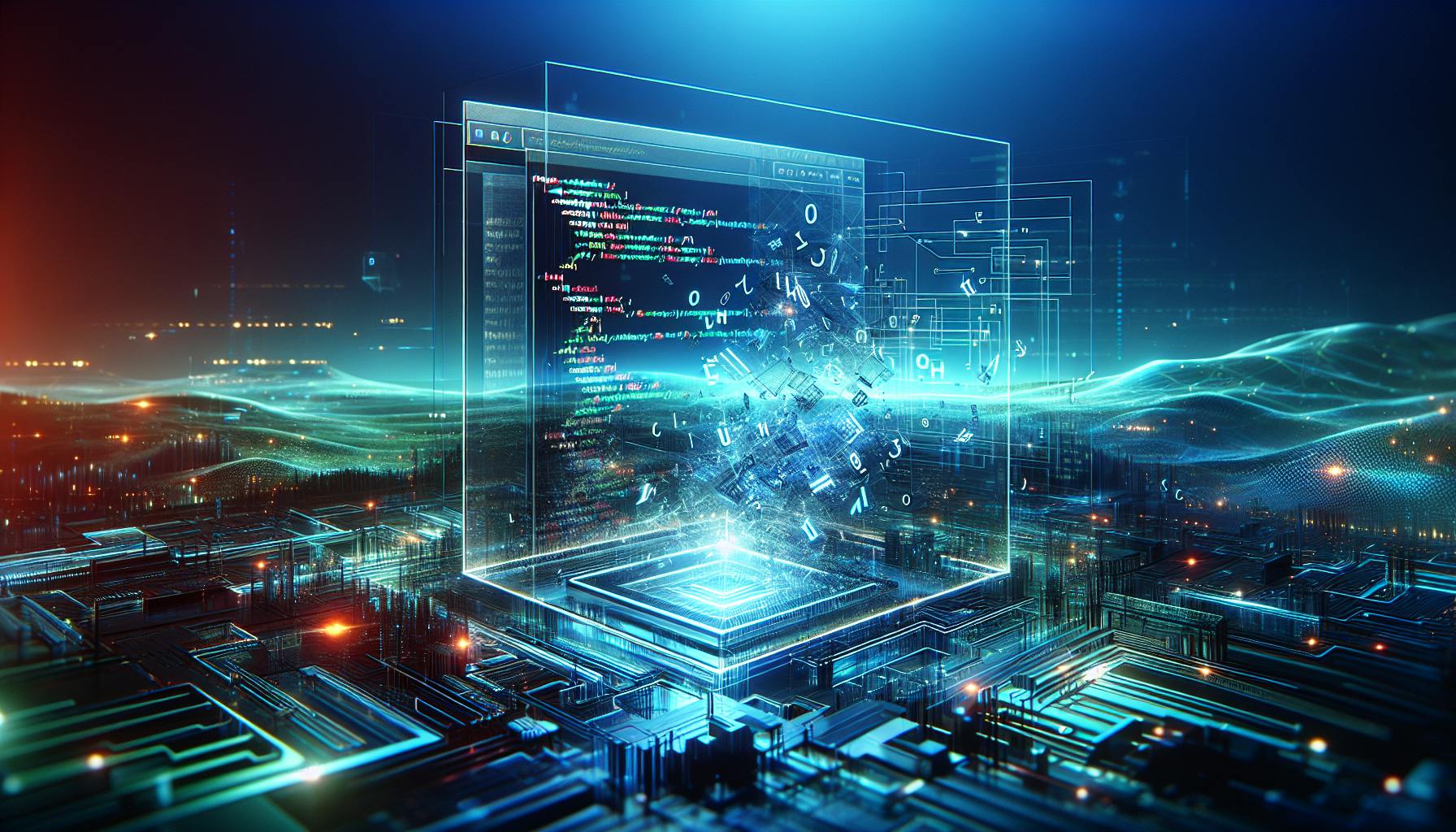
Learn the basics of HTML documents and how to use Chrome Developer Tools for web development. Explore essential HTML elements, DevTools features, and practical tips.
Creating and refining websites in Chrome involves understanding HTML documents and leveraging Chrome's Developer Tools (DevTools). Here's a quick guide to get you started:
- Understanding HTML: Learn the basics of HTML structure, including elements like
<head>
,<body>
, and<title>
, which help browsers display your page correctly. - Using Chrome DevTools: Access DevTools using keyboard shortcuts or the Chrome menu to inspect and modify HTML in real-time, debug issues, and test responsive designs.
- Practical HTML Development: Edit HTML directly in the Elements panel, debug common problems, and ensure your site works well on all devices.
- Advanced Features: Explore HTML5 APIs for enhanced interactivity and use DevTools for performance optimization and accessibility testing.
This guide is designed to help new developers understand the essentials of creating and optimizing web content with HTML and Chrome's powerful tools.
Anatomy of an HTML Document
Here's what a simple HTML page looks like:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<!-- page content goes here -->
</body>
</html>
<!DOCTYPE html>
- This line tells the browser that this is a modern HTML5 document.<html>
- This is the main container that holds everything in your webpage.<head>
- This part has stuff like the webpage's title, links to stylesheets, and scripts.<title>
- This sets the name you see on the browser tab.<body>
- This is where all the stuff that people actually see on your page goes.
This setup helps the browser understand and show your page correctly.
Common HTML Elements
Here are some basic parts of HTML you'll use a lot:
Text Elements
<p>
- For paragraphs of text<h1>
to<h6>
- For titles and subtitles
Structural Elements
<div>
- A box for grouping stuff together<span>
- A small container, usually for a bit of text
Interactive Elements
<a>
- For links<form>
- To make forms for users to fill out<input>
- Lets users enter data
These parts let you organize text, make sections on your page, and add things like links and forms.
HTML Attributes
HTML parts can have extra settings called attributes:
class
- Lets you group elements for stylingid
- A unique identifier for styling or scriptingstyle
- Directly add styles to an elementsrc
- Tells where to find images or videoshref
- Sets the destination for a link
Attributes help you customize how parts of your page look or act.
Chapter 2: Chrome Developer Tools (DevTools) Introduction
Accessing DevTools
There are a couple of simple ways to open the DevTools in Chrome:
- Keyboard shortcut:
- For Mac users: Option + Command + J
- For Windows/Linux users: Control + Shift + J
- Right-click on any part of a webpage and choose "Inspect"
- Go to the Chrome menu, select More Tools, and then click on Developer Tools
Once you do that, the DevTools window pops up. You can attach it to the side of your browser so it's easy to see.
Essential Panels for HTML Development
Here are some handy parts of DevTools when you're working with websites:
Elements Panel
This panel shows you the webpage's HTML and CSS. Here's what you can do:
- Look at and change HTML elements and their attributes
- Try out CSS changes on the spot to see what happens
- Fix problems with how things look on your page
It's like a live peek into your webpage's code.
Console Panel
The Console lets you:
- Run JavaScript right there on your webpage
- See errors or messages from your JavaScript code
- Work with JavaScript objects that are part of your page
It's great for quick JavaScript tests without reloading your page.
Sources Panel
This part deals with the JavaScript and CSS files your page uses. You can:
- Stop JavaScript in its tracks to check out what's happening (this is called setting breakpoints)
- Walk through your code step by step
- Change scripts on the fly
- Look at network requests to see how fast things are loading
These panels are super useful for tweaking your HTML, CSS, and JavaScript. They're a big help in making sure your webpage looks and works just right.
Chapter 3: Practical HTML Development with Chrome DevTools
Editing HTML in Real-Time
The Elements panel in Chrome DevTools lets you change the HTML of a webpage while you're looking at it. This is super handy for making quick adjustments.
- Click on any HTML tag in the Elements panel to see where it shows up on the page. It's a good way to understand how your code turns into what people see.
- Double click on text to change it right away, like updating a headline or paragraph.
- You can add, delete, or move HTML elements around in the panel to change how the page is put together.
- Change attributes like
id
,class
,src
to see how they affect the look and actions of your page. - Right-clicking on elements gives you more options, like adding CSS styles or setting up JavaScript breakpoints.
This instant editing lets you try out changes quickly without having to refresh the page, making it easier to get your layout just right.
Debugging HTML Issues
DevTools also helps you find and fix common HTML problems:
- The Console will show warnings or errors if there's something wrong with your HTML.
- You can check the padding, margins, and sizes of elements to sort out layout issues.
- It can point out things like missing image alt text or wrong ARIA roles.
- You can force elements to show in states like hover or focus to make sure they work right.
- Use breakpoints in JavaScript that interacts with your page elements to figure out problems with things that move or change.
Fixing these issues early on makes your website better and saves you trouble later.
Responsive Design Testing
The Device Mode toolbar lets you see how your site looks on different devices, like phones or tablets:
- Pick from devices like iPhone, Pixel, or Galaxy to check compatibility.
- Slow down the CPU and internet speed to mimic real-life use.
- Switch between portrait and landscape to see if your page adjusts.
- You can also set your own screen sizes for specific tests.
Making sure your site looks good on all screens is key, and this tool helps you test everything before your site goes live.
Chapter 4: Advanced HTML & Chrome DevTools Features
Working with HTML5 APIs
HTML5 brought us some cool tools that let websites do more stuff. Here are a few:
- Geolocation API - This tool can figure out where you are. Websites can use it to show places or things near you.
- Web Storage API - Lets websites save information on your computer or phone. This way, they can remember things about you without having to ask all the time.
- Web Sockets - These make it possible for websites to chat back and forth with the server without waiting. It's great for things like live chat.
- Web Workers - This lets websites do heavy work in the background without making the site slow or freeze.
- WebRTC - Allows video calls to happen right in your browser without needing extra software.
Chrome DevTools can help you work with these tools by:
- Checking out API data in the Console.
- Pausing code to see what's happening, step by step.
- Pretending to be in different places to test location features.
- Watching how these features affect your website's speed.
Using these tools can make your website do more interesting things.
Performance Optimization
How fast your site loads is super important. If it's slow, people might leave.
Chrome DevTools has a Performance panel that helps you make your site faster:
- It shows you what's taking so long to load.
- Finds code or pictures that aren't needed.
- Points out when your site does too much work, making it slow.
- Helps you make pictures smaller so they load faster.
Even making your site a little bit faster can help keep people around.
Accessibility Features
Everyone should be able to use your website, even if they have disabilities. HTML helps with this.
Chrome DevTools has tools to check if your site is easy for everyone to use:
- The Color Picker checks if your text is easy to read on its background.
- The Screen Reader tells you what your site sounds like to people who can't see it.
- It points out things you might have missed, like labels for buttons.
- You can check if people can use your site without a mouse.
- And, you can see how your site looks to people with different kinds of vision problems.
Making sure your site works for everyone is not just nice, it's important. It makes your site better for all users.
sbb-itb-bfaad5b
Conclusion
HTML documents and tools like Chrome's DevTools are really helpful for people who make websites. By knowing the basic parts of HTML and how to put them together, you can make websites that are easy and fun to use. DevTools makes it even better by letting you change things as you go, find and fix mistakes, and make your site faster and more welcoming to everyone.
Here are some simple points to remember:
- HTML documents are like the backbone for websites. Parts like
<head>
,<body>
, and<title>
set up the basic structure. - You can use different HTML elements to add text, pictures, links, and forms to your site. Attributes help you customize these elements.
- Chrome DevTools has sections like Elements, Console, and Sources that let you change your site's code and check it in real time.
- Being able to edit HTML with DevTools on the spot helps you quickly see how changes look and work.
- Tools for finding and fixing code or performance issues help you solve problems fast.
- Features that make your site run faster and be open to everyone are really important.
By getting the hang of HTML basics and using DevTools to change and test your site, you can make really great websites that people enjoy using.
Try playing around with HTML parts and using DevTools to see what you can do. As you get more comfortable with the basics, you might want to explore more advanced stuff like HTML5 APIs for adding cool interactive features. The web is always changing, so using these tools can help you keep up and make awesome websites.
Appendix: Additional Resources
Here are some helpful links if you want to dive deeper into HTML and how to use Chrome DevTools:
HTML References
- MDN Web Docs - HTML - A great place to look up HTML stuff.
- W3Schools - HTML - Easy to follow tutorials and info on HTML.
- HTML Living Standard - The newest rules and features for HTML5.
Chrome DevTools
- DevTools Documentation - The official guide to using DevTools.
- Udacity Course on DevTools - A free video course on how to use DevTools.
- DevTips YouTube Channel - Handy video tips for working with DevTools.
HTML Books
- HTML & CSS: Design and Build Websites by Jon Duckett - A great book for beginners on HTML and CSS.
- HTML5 for Web Designers by Jeremy Keith - Focuses on the latest HTML5 features.
Accessibility Resources
- WebAIM - Info and resources on making your site accessible to everyone.
- The A11Y Project - Guides from the community on accessibility.
Performance Optimization
- web.dev/fast - Tips on making your website load faster.
- DebugBear - Keeps an eye on your website's speed.
HTML Newsletters
- Really Good Emails - Get inspired by these design ideas for HTML emails.
- Mailchimp - Guides on email marketing.
I hope these links help you learn more about creating better websites with HTML and Chrome DevTools! Feel free to reach out if you have more questions.
Related Questions
What are the basic HTML document?
An HTML document is a simple text file that ends with .html or .htm. It's filled with text and special codes known as HTML tags (like <html>
, <head>
, <body>
) that tell web browsers how to show the webpage. A basic HTML document needs:
<!DOCTYPE html>
- Tells the browser it's an HTML5 document.<html>
- The main container for everything in the document.<head>
- Holds stuff like the webpage title and links to styles or scripts.<body>
- Where all the stuff you see on the webpage goes.<title>
- Sets the webpage's title (what you see on the browser tab).
These elements help the browser display the webpage correctly.
How to create HTML document in web development?
To make an HTML document:
- Use a basic text editor (like Notepad or TextEdit).
- Start with the
<!DOCTYPE html>
declaration. - Add the
<html>
,<head>
, and<body>
tags. - Put a
<title>
tag inside the head section. - Use other tags like
<p>
,<h1>
,<img>
inside the body to add content. - Save the file with an .html or .htm extension.
- Open it in a web browser to see your webpage.
This is how you set up a simple webpage that browsers can understand.
What are the basic rules in creating an HTML document?
When making an HTML document, remember:
- HTML elements need opening and closing tags with content in the middle.
- Use attributes inside opening tags to give more details.
- Block elements start on a new line; inline elements don't.
- Put elements inside each other correctly, like a family tree.
- Use specific elements (like
<header>
,<nav>
) for different parts of your page. - Make sure your HTML is tidy and follows web standards.
Following these rules helps make your webpage work better.
What should every HTML document have?
Every HTML document must have:
- A
<!DOCTYPE html>
declaration. - An
<html>
root element. - A
<head>
element with a<title>
. - A
<body>
element for all the stuff you see on the page. - A
<title>
element for the webpage's name.
The title is really important because it's used for the page name in browser tabs and bookmarks. Having these basics makes sure your webpage shows up right in browsers.