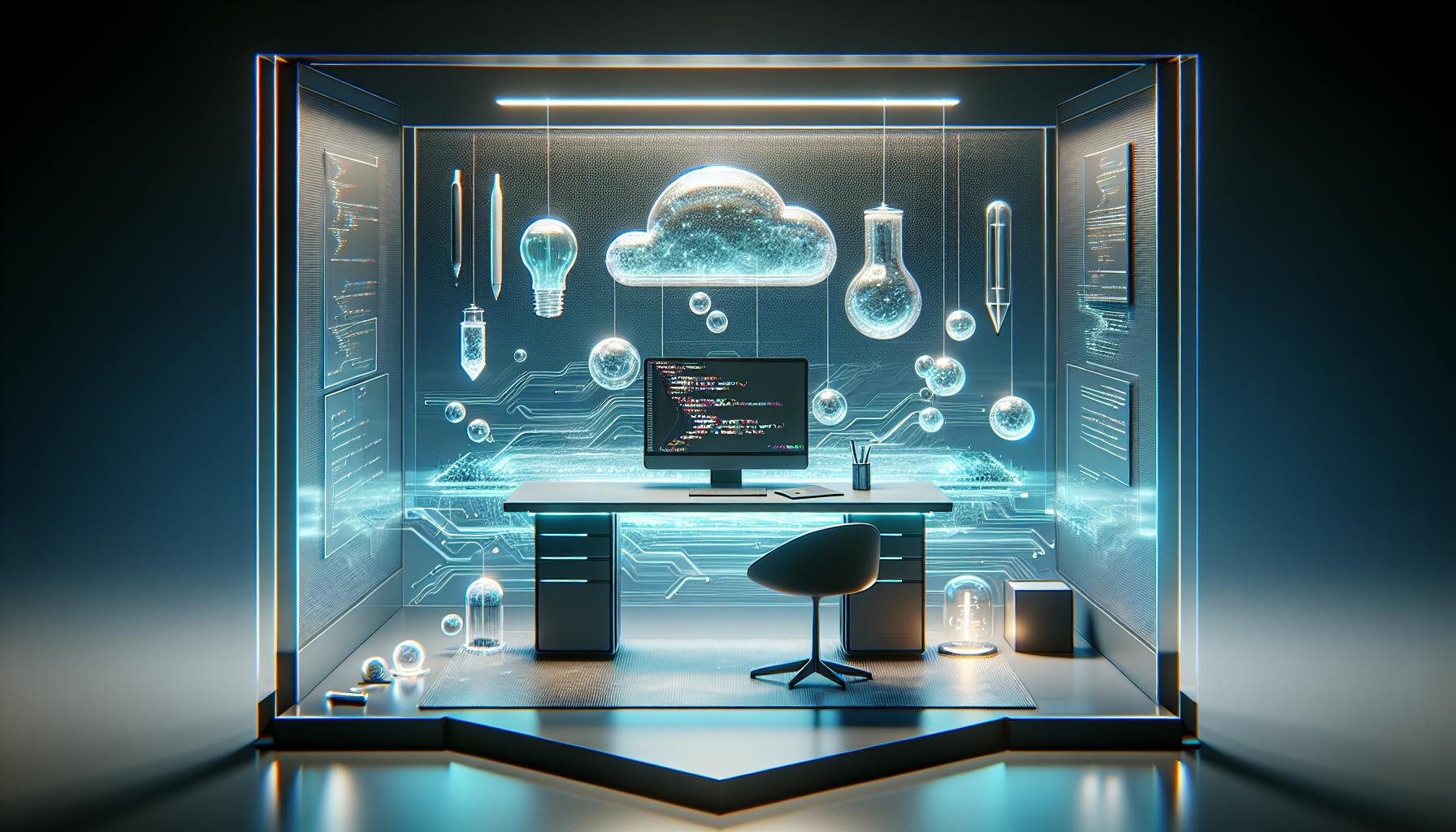
Learn how to improve as a JavaScript writer by following code conventions, readability tips, and performance optimization techniques. Master debugging and adopt a test-driven mindset for better code quality.
Becoming a better JavaScript writer means focusing on clarity, efficiency, and constant improvement. Here's what you need to know:
- Follow Standard Code Conventions: Use guides like the Airbnb JavaScript Style Guide for cleaner, more understandable code.
- Improve Readability with Comments: Use comments to explain the 'why' behind code sections, especially complex ones.
- Refactor Frequently: Regularly revisit and clean up your code to maintain its efficiency and readability.
- Write Performant JavaScript Code: Optimize your code for speed by avoiding scope pollution, leveraging browser APIs efficiently, and optimizing loops and recursion.
- Master Debugging Techniques: Use strict mode, leverage source maps, and log data effectively to find and fix errors quickly.
- Adopt a Test-Driven Mindset: Emphasize testing to ensure your code works as intended, utilizing tools like Jest for unit testing and automating your testing workflows.
Improving as a JavaScript writer involves not just understanding the language's syntax but also adopting best practices for code quality, performance, and maintainability. Whether you're a beginner or an experienced developer, these strategies will help you write JavaScript that's both powerful and easy to manage.
Follow Standard Code Conventions
Using common coding rules and guides like the Airbnb JavaScript Style Guide is helpful because:
- It makes your code easier for others to read. Names for things in your code will make more sense.
- Your code will look neat, making it quicker to understand.
- Getting used to these rules means you're ready to work with others.
- Tools like ESLint can automatically check your code for mistakes, saving you trouble.
Sticking to these rules keeps your code tidy and easy to read, no matter how complex it gets. It pushes you to write JavaScript that's clean and easy to understand.
Improve Readability with Comments
Comments should help explain why and what your code does, rather than how it does it. Don't overdo it - if your code is clear, you won't need many comments. But, a few well-placed ones can help explain:
- Why a part of your code is there, especially if it's tricky
- What you were thinking when you wrote it
- Any important background info
Also, comments are great for:
- Breaking up your code into clear sections
- Highlighting parts that aren't finished yet
- Pointing out where you might make things better later on
Good comments tell people why you did something, not just how. Keep them short, useful, and up-to-date.
Refactor Frequently
Going back to improve your code regularly is important. It keeps your code clean and up-to-date. Here's why it's good:
- Splitting big functions into smaller ones makes them easier to use again and test.
- Using the latest JavaScript features can make your code better.
- Changing names that don't make sense to something clearer.
- Combining similar bits of code into one place.
- Putting related functions together so they're easier to find.
Keep looking at your code to find ways to make it better. This means your code gets more organized and easier to work with as time goes on. Think of your code as something that's always changing and getting better.
Write Performant JavaScript Code
Making your JavaScript code run fast is key to giving people a smooth experience on your website or app. If things load slowly or feel sluggish, people might not want to stick around. Here's how to keep your code speedy and efficient.
Limit Scope Pollution
Scope pollution happens when you have too many variables floating around together, which can make things confusing and could cause mix-ups in your code.
It's better to use const
and let
for declaring variables since they are limited to where they're used, unlike var
which is more wide-reaching:
// Not the best choice
var a = 1;
var b = 2;
// A better approach
const a = 1;
let b = 2;
You can also wrap parts of your code in functions that run right away to keep variables from being seen everywhere:
(function() {
// Your variables go here
const a = 1;
// This part can use a
// But outside this function, a isn't available
})();
Doing this helps your code use memory better and stops you from accidentally changing values you didn't mean to.
Leverage Browser APIs Efficiently
The browser comes with tools built-in that can help your code run faster.
For example, IntersectionObserver
is great for loading images or parts of your page only when they're about to be seen:
const observer = new IntersectionObserver(callback);
observer.observe(target); // This function runs when the target is visible
And for animations, requestAnimationFrame()
is smoother than setInterval()
:
function animate() {
// Your animation code goes here
requestAnimationFrame(animate);
}
requestAnimationFrame(animate);
This makes sure your animations are in sync with how the browser refreshes the screen, making them look better.
Optimize Loops and Recursion
Using for
loops is usually quicker than forEach
:
// This is faster
for (let i = 0; i < arr.length; i++) {}
// This is slower
arr.forEach(el => {})
Remembering results with recursive functions:
// Save the result of fib(n) so we don't have to do it again
const cache = {};
function fib(n) {
if (n in cache) {
return cache[n];
} else {
cache[n] = fib(n-1) + fib(n-2);
return cache[n];
}
}
This stops your function from doing the same work over and over, which can really speed things up.
By following these tips, your JavaScript code will be more efficient and make your site or app feel faster. Use tools like DevTools in your browser to check your code's performance before and after making changes to see the difference.
Master Debugging Techniques
Finding and fixing errors in your JavaScript code can seem tough, but knowing the right steps can make it a lot easier. Here are some handy tips to help you out when you're stuck with a bug.
Activate Strict Mode
By adding "use strict"; at the beginning of your JavaScript files, you turn on strict mode. This helps by pointing out common mistakes as you code.
For instance:
"use strict";
function test() {
// Your code here
}
Strict mode is great because it:
- Makes sure you declare variables before using them, so you avoid mistakes
- Stops you from accidentally creating global variables which can mess things up
- Changes how 'this' works in functions, making it safer
- Stops you from adding new properties to objects that shouldn't change
In short, strict mode helps your code be more secure and error-free by being strict about the rules.
Leverage Source Maps
When your JavaScript is made smaller for websites (minified), it's hard to find errors because everything is squished together. Source maps help by connecting your minified code back to the original, making it easier to figure out problems.
To do this:
- Create a source map when you make your code smaller
- Use the map to find errors in your minified code
For example, with tools like webpack:
devtool: 'source-map',
Using source maps means you can debug in your editor, not in the squished code.
Log Data Effectively
Writing down what your app is doing at certain times (logging) helps you understand what's happening inside.
Mark your log messages so you know what you're looking at:
console.log("User data", userData);
Use different log types like warn()
and error()
to show how important something is:
console.warn("File not found");
console.error("Could not connect");
And change how much you log to control how much detail you see:
console.log("DB connected"); // For debugging
console.info("User signed in"); // For general info
By logging smartly, you can get a clearer picture of what your app is doing, making it easier to fix problems.
sbb-itb-bfaad5b
Adopt a Test-Driven Mindset
Testing is super important when you're writing JavaScript. It's like making sure everything works before you show it to others. By writing tests first, you catch problems early and make sure your code does what it's supposed to do.
Unit Test Critical Functions
Testing one piece of your code at a time helps avoid future headaches. Here's how to do it right:
- Try to test without relying on other parts of your app.
- Check for weird or unexpected inputs to see if your code can handle them.
- Use fake versions of things your code talks to, like databases or web services.
For example, if you're testing a simple sum()
function, you'd write tests like this:
test('sums numbers', () => {
expect(sum(1, 2)).toBe(3);
expect(sum(-1, 1)).toBe(0);
expect(sum(5, 0)).toBe(5);
});
This way, you can change your code later and quickly know if something breaks.
Integrate Testing Tools
Tools like Jest and Mocha help you write and run tests easily. Using ESLint with Jest helps catch common mistakes and makes your tests better.
Automate Testing Workflows
Automating your tests means they run by themselves whenever you change your code. This stops bugs from sneaking in.
Using something like GitHub Actions, you can set up your tests to run every time you update your code:
on: push
jobs:
test:
runs-on: ubuntu
steps:
- uses: actions/checkout@v2
- run: npm install
- run: npm test
If a test fails, you can't move forward, which encourages you to write tests from the start. Automated testing makes your code stronger and gives you peace of mind.
Conclusion
Becoming a better JavaScript developer means you're always learning and getting better at what you do. It's about making sure your code is easy to read, works fast, and doesn't have mistakes. Here's a quick summary of what we talked about:
- Use common rules and tips like the ones from Airbnb to make your code look the same everywhere. This makes it easier for you and others to understand and work on. Remember to use comments to explain why you did something, but only when you really need to.
- Keep improving your code by organizing and updating it. This makes it easier to work with later on.
- Be smart about using variables to avoid mix-ups. Use the tools your browser gives you like
IntersectionObserver
andrequestAnimationFrame()
to make your site run smoother. - Make loops and recursive functions faster by choosing the right way to do things. Remember to save results from functions so you don't do the same work twice.
- Turn on strict mode to catch mistakes early. Use source maps to figure out where errors are coming from in your code. Log what's happening in a way that makes sense, so you can find and fix problems faster.
- Test the important parts of your code to make sure changes don't break anything. Use tools like Jest to help you test easier. Make testing automatic so it happens every time you make changes, catching problems right away.
Following these tips will help you write JavaScript that's better and easier to maintain. The key is to keep doing these things regularly. Learning JavaScript is a long journey, but adding these habits to your daily work will help you get better bit by bit.
Related Questions
How to write better JavaScript?
Here are some tips for writing clearer JavaScript code:
- Name your variables clearly using camelCase to help others understand what they do.
- Prefer
const
andlet
overvar
to avoid problems with variables being seen everywhere. - Keep your code neat by grouping related code together in functions or modules.
- When looping, try not to do heavy tasks inside as it can make your code slow.
- Arrow functions are great because they make your code shorter and avoid some common mistakes.
- Use default values in functions for times when no value is given.
- Breaking down objects and arrays into simpler parts can make your code easier to read.
- Write comments to explain tricky parts of your code, but only where needed.
How can I become a better JavaScript developer?
To get better at JavaScript:
- Make sure you understand the basics well.
- Learn about how objects and functions can work together.
- Keep up with new ways to write JavaScript like using arrow functions, promises, and async/await.
- Practice by building things and solving coding problems.
- Read up on the latest best practices and learn from experts.
- Always aim for clean and well-organized code.
- Get to know the tools developers use to find and fix problems in their code.
How can I improve my JavaScript?
Improving your JavaScript skills takes regular effort:
- Try building something new often.
- Work on coding challenges online.
- Join in on open source projects.
- Read books and blogs about JavaScript.
- Follow JavaScript news and learn from others.
- Teach yourself by watching videos and going to meetups.
- Try coding with others to learn from them.
The more you code, the better you'll get.
Why is JavaScript so hard for me?
JavaScript might feel tough because:
- It does things differently when it comes to running code in order.
- Some ideas like closures and callbacks can be tricky at first.
- Getting started with all the tools and setups can be overwhelming.
- Differences in how browsers work can be confusing.
- Errors can be hard to figure out.
- There's always something new to learn.
- It's easy to start with habits that might not be the best.
Don't worry! Focus on the basics, practice a lot, ask for help, and consider using TypeScript to add some rules that might help.