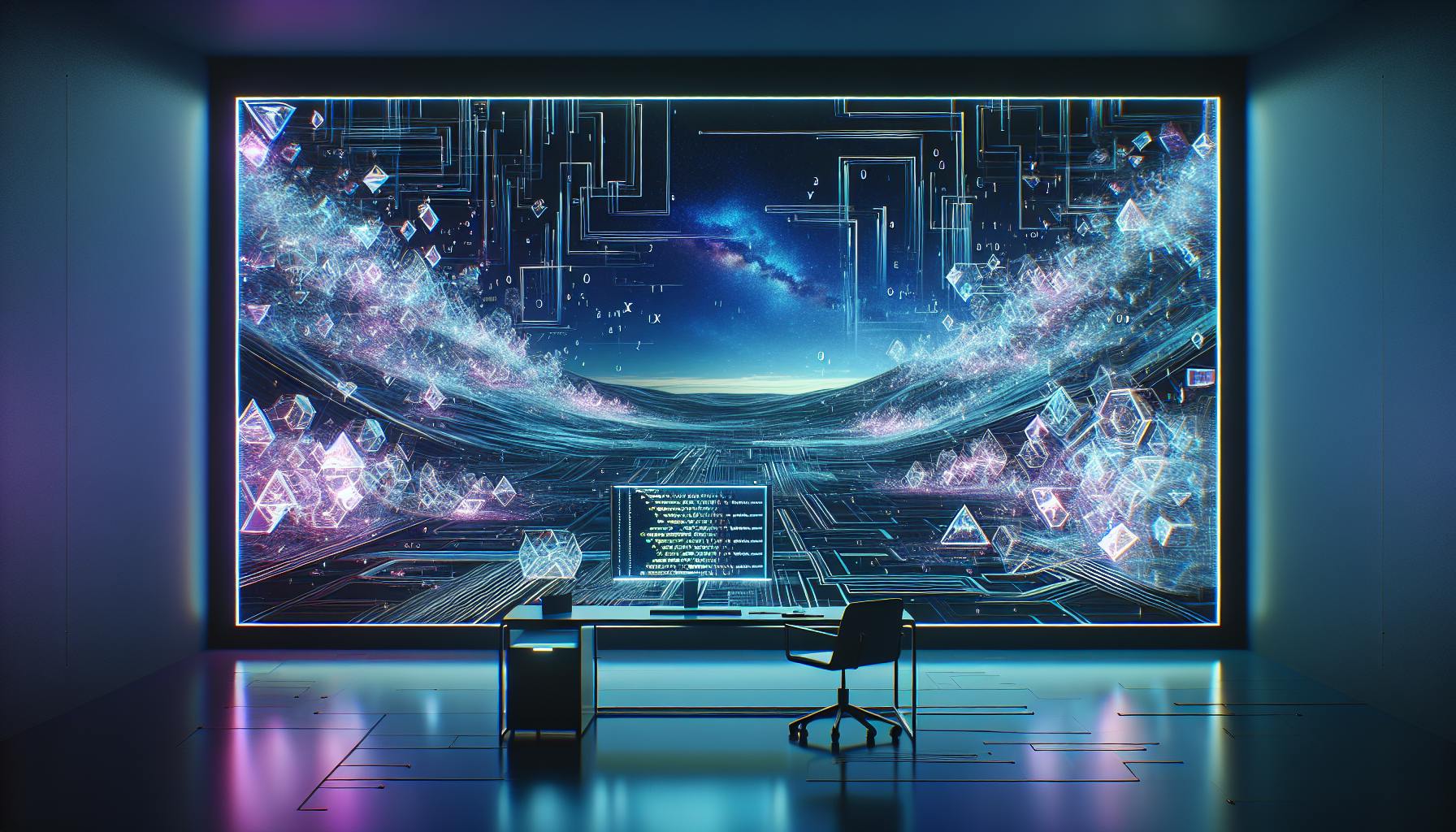
Learn the basics of JavaScript interpreters, core features, how they work, and how to build a simple interpreter. Explore advanced topics like optimizations, performance, and extending functionality.
Understanding how JavaScript interpreters work is crucial for developers looking to make their web pages interactive and engaging. Here's a quick rundown of what you need to know:
- JavaScript interpreters are built into web browsers, allowing them to understand and execute your code.
- They work by reading and executing JavaScript code step-by-step, making it possible to see the effects of code changes in real-time.
- Modern interpreters often use JIT (Just-In-Time) compilation to improve performance, turning JavaScript into optimized code just before execution.
- Essential components of a JavaScript interpreter include the lexer, parser, compiler, and executor, which together process and run your code on the web.
By the end of this guide, you'll have a solid understanding of both the theory behind JavaScript interpreters and practical tips for utilizing them in your web development projects.
What is JavaScript?
JavaScript is a simple yet powerful language used to make websites more fun and interactive. It helps you add things like moving pictures, forms that check your info, and more without having to refresh the page every time.
Here are some basics about JavaScript:
- It works right in your web browser, meaning it can make changes on a webpage in real-time.
- It's one of the main tools for making websites alongside HTML (which creates the content) and CSS (which makes it look nice).
- Even though it looks a bit like the programming language C, JavaScript is easier because the web browser does the hard work of understanding it for you.
- With JavaScript, you can change how a webpage looks or reacts based on what you do, like clicking a button or entering information.
- It's also behind the scenes of more complex websites, working with tools like React or Angular to build big, interactive sites.
To sum it up, JavaScript makes webpages do cool things and is a must-know for making modern, interactive websites.
Core Features of JavaScript
JavaScript lets you do a lot of useful stuff, such as:
- Variables - Keep track of things like numbers, text, lists, and more.
- Operators - Use math and other rules to work with those things.
- Conditionals - Make decisions in your code, like choosing what to do if something is true or false.
- Loops - Do something over and over again without writing it out each time.
- Functions - Group your code into chunks that you can use whenever you need them.
- Events - React to things users do, like clicking or typing.
- DOM Manipulation - Change or add to what's on the webpage on the fly.
- Data Structures - Organize your data in ways that make it easy to work with.
- Browser API - Use special browser features like finding your location or saving data.
Learning these basics helps you build just about anything you can think of for the web.
JavaScript in Action
When you visit a website, the browser gets the HTML (the main content), CSS (the style), and JavaScript (the interactive bits). First, it shows you the page using HTML and CSS. Then, JavaScript makes it come to life by changing things around or adding new stuff without reloading the page.
For example, JavaScript can:
- Put new things on the page, like pictures or text.
- Change how things look or where they are.
- Notice when you do something (like clicking) and react to it.
- Check your info in forms to make sure it's right before sending it off.
- Make cool effects like animations.
JavaScript runs in the order it's written unless you tell it to wait for something, like a button click. You can also tell your browser to load some JavaScript later so it doesn't slow down showing the page.
For safety, JavaScript can't mess with your files or computer directly. But, with your okay, it can do things like see where you are or remember what you did last time.
In short, JavaScript turns basic webpages into interactive experiences. Getting the hang of how it works with the webpage and the browser lets you do some pretty amazing things.
Interpreters and Compilers Explained
The Basics
Think of an interpreter like a live translator who reads a book out loud, translating it into another language one sentence at a time. For programming, this means taking the JavaScript code you wrote and turning it into something the computer understands as it goes along. This is great because you can quickly see how changes you make affect things.
Key points about interpreters:
- They let you see results right away, which is super handy when you're tweaking your code.
- Since they work as they go, they can be a bit slow.
- Some smart interpreters use a trick called JIT (just-in-time) compilation to speed things up. They prepare parts of your code in advance to make everything run smoother.
Now, a compiler is like someone who takes your entire book, translates it all at once, and then gives it back to you. This means your computer can run the translated (compiled) version directly, which is usually faster.
Key points about compilers:
- They make your code run quickly because they do all the hard work before you even start the program.
- You have to wait a bit to see the results of your changes since it translates everything at once.
- Languages like C and Rust use compilers to turn your code into fast-running programs.
In short:
- Interpreters work step-by-step and are great for quick tests but can be slow.
- Compilers get everything ready upfront, making your programs run faster but slowing down the testing process.
Many programming languages use a bit of both to get the best of both worlds.
Pros and Cons
Interpreter Pros:
- Quick feedback on your code changes.
- Works the same across different computers.
- Easy to update with new features.
Interpreter Cons:
- Not as fast as compiled code.
- Hard to make the code run more efficiently.
Compiler Pros:
- Your program runs faster.
- You can fine-tune your code to make it even quicker.
- It's made specifically for the computer it's running on.
Compiler Cons:
- You have to translate your code each time you make changes before you can test it.
- The translated code might only work on certain computers.
- Updating the program means you might have to translate your code all over again.
Overall, interpreters are great for when you want to see changes quickly and don't mind a bit of a slowdown, while compilers are your go-to for making sure your program runs as fast as possible.
The JavaScript Interpreter
How It Works
Think of the JavaScript interpreter as a behind-the-scenes wizard in your web browser that makes your JavaScript code work. Here's a simple breakdown of what happens:
- Lexical Analysis: First up, the interpreter chops your JavaScript code into bite-sized pieces called tokens. This step is all about identifying the different parts of your code, like variables, commands, and symbols.
- Parsing: Next, these tokens get organized into a tree structure that shows how they all fit together. This tree is like a detailed map of your code, helping the interpreter understand what you're trying to do.
- Compilation: Then, the map (or tree) is turned into a special language that the computer's processor can understand. Some interpreters use a smart method called Just-In-Time (JIT) compilation to make this process faster.
- Execution: Finally, this computer-friendly code is run, step-by-step. This is when your code comes to life, making things happen on the webpage, like moving pictures or reacting to clicks.
The most popular JavaScript interpreter is called V8, and it's used in the Chrome browser. V8 is really good at turning JavaScript into fast-running code. It also uses JIT compilation.
Other web browsers have their own interpreters, like Firefox's SpiderMonkey. They all do the same basic job, but each has its own special tweaks.
Key Components
Here are the main parts of a JavaScript interpreter:
- Lexer: This part breaks down the code into tokens by spotting different elements like variable names and symbols.
- Parser: Takes the tokens and arranges them into a tree that shows the structure of your code.
- Abstract syntax tree (AST): A tree that represents the structure of your code in a way the interpreter can understand.
- Compiler: Turns the tree into a language that the computer's processor can run.
- Executor: Takes the computer-friendly code and runs it, making your code do its thing.
- Memory Heap: A place where data is stored while your code runs. It keeps track of things like variables and objects.
- Call Stack: Keeps an eye on which functions are running to make sure everything happens in the right order.
- Event Loop: Makes sure events and callbacks happen at the right time, especially when dealing with code that doesn't run right away.
Together, these parts read, organize, turn into computer language, and manage your JavaScript code, making it work in the blink of an eye.
Building a Simple JavaScript Interpreter
Getting Started
To make a basic JavaScript interpreter, you'll want to start simple. Here's how:
- Pick a few key parts of JavaScript: Start with basics like variables, math, if-else statements, loops, and functions. Trying to handle everything in JavaScript from the start is too complex.
- Make a lexer: This tool looks at your JavaScript code and breaks it down into small pieces, like numbers or plus signs.
- Make a parser: This organizes those small pieces into a tree that shows how your code fits together.
- Make an evaluator: This goes through the tree and follows your code's instructions to do things like calculations or store information.
You can use special tools to help with making the lexer and parser, and it's a good idea to use a known tree format to keep things simple.
Start with easy stuff like math operations and build from there. Test your interpreter with example code to make sure it works right.
Step-by-Step Guide
Here's a simple way to build your interpreter:
1. Define tokens
Break down the code into tokens (basic elements) like numbers, plus signs, minus signs, and so on.
2. Build the lexer
Use a tool or write your own code to turn the JavaScript code into a list of these tokens.
3. Define grammar rules
Set up rules that say how tokens can be put together to make valid code.
4. Build the parser
This checks the tokens against your rules and builds a tree that represents your code.
5. Build an evaluator
Go through the tree, doing what the nodes say, like adding numbers or assigning values to variables.
6. Print output
Show the result of running your code. Later on, you might make it create compiled code instead.
7. Test and debug
Try out different pieces of code, see what the tree and results look like, and fix any problems you find.
It takes some work, but it's a great way to learn. Start with simple tasks and add more complex features as you go.
sbb-itb-bfaad5b
Advanced Topics in JavaScript Interpretation
Optimizations and Performance
JavaScript interpreters use different tricks to make code run faster and smoother:
Just-In-Time (JIT) Compilation
- Turns JavaScript into a special code at the last minute, right before it runs. This makes things quicker because it skips waiting.
- Watches how your code runs and finds slow spots to make them faster.
- This method is in most modern browsers and makes a big difference in speed.
Hot and Cold Code Detection
- Figures out which parts of your code are used a lot (hot) and which aren't (cold).
- It focuses on making the hot parts faster, because not all code needs the same level of attention.
Inline Caching
- Remembers the results of looking up properties or functions, so it's faster the next time around.
- Works well when your code does the same thing over and over.
Hidden Classes
- Groups objects that look alike so they can share information.
- This makes accessing properties quicker and uses less memory.
Code Minification
- Cleans up your code by taking out extra spaces, making variable names shorter, and simplifying things without changing what the code does.
- This makes your code smaller so it's faster for browsers to read and run.
Extending Functionality
JavaScript interpreters can be made to do new things:
User-defined Functions
- Lets you add your own functions that can do special tasks, like interacting with the browser in new ways.
New Data Types
- Allows for different kinds of data structures, making it easier to organize your data for specific tasks.
Language Constructs
- Adds new shortcuts like
for...of
loops orasync/await
to make writing code easier and cleaner.
Type Checking
- Checks the types of your variables to catch mistakes early. Adding optional types can also make your code easier to understand.
Scoped Bindings
Let
andconst
let you declare variables in a way that avoids confusion and errors from unexpected overlaps.
Adding new features to the interpreter means it can understand and run new kinds of code, making your programming more powerful and flexible.
Conclusion
Interpreters are super important for making JavaScript work on web pages. They turn the JavaScript code we write into something the browser can understand, making websites interactive and fun.
In this guide, we talked about:
- How interpreters break down code into small pieces and organize them to figure out what needs to be done
- How they turn this organization into code that the computer can run
- Parts like the lexer, parser, and compiler that help in this process
- Smart ways like JIT compilation that make the whole thing run faster
We also touched on making a basic interpreter yourself, which is a cool way to understand how everything works.
As JavaScript gets updated, so do the interpreters. They get better at making code run fast and can do new things like letting you add your own special functions.
For anyone wanting to know more about how JavaScript works behind the scenes, learning about interpreters is really useful. It can help you write code that runs better and lets you do more creative stuff.
So, if you're curious, start exploring interpreters. It's a great way to dive deeper into JavaScript. Have fun with your coding!
Appendix: Resources for Further Learning
Here are some helpful resources if you want to dive deeper into how JavaScript interpreters work and the basics of creating programming languages:
Tutorials and Courses
- Let's Build a Simple Interpreter - This series helps you make your own interpreter. It's explained in a way that's easy to understand.
- JavaScript Interpreter in JavaScript - A guide to making a basic JavaScript interpreter using JavaScript itself. It's a hands-on way to learn.
- Compilers and Interpreters - A free course on Udacity that covers the important parts of how compilers and interpreters work.
Documentation
- V8 Docs - Learn about Google's V8 engine, which is a key part of how Chrome understands JavaScript.
- SpiderMonkey - Info on Mozilla's JavaScript engine for Firefox.
- JavaScript Engine Fundamentals - A deep dive into how JavaScript engines work under the hood.
Books
- Writing An Interpreter In Go - A book that guides you through building an interpreter, with examples in Go.
- Engineering a Compiler - A detailed textbook on how to design compilers.
These resources are great for getting a better understanding of how programming languages work, especially JavaScript. They'll help you see behind the scenes of how your code runs, making you a better programmer.
Related Questions
How to write an interpreter in JavaScript?
To make your own interpreter in JavaScript, follow these steps:
- Build a lexer: This part looks at your code and breaks it down into smaller pieces, like numbers or symbols.
- Create a parser: This organizes those small pieces into a structure (called an abstract syntax tree) that shows how your code fits together.
- Make an interpreter: This goes through the structure and follows the instructions, doing things like calculations or saving information.
Here are some things you'll need to do:
- Decide on the tokens (pieces of code) you want to recognize.
- Use tools like regular expressions to help build the lexer.
- Set up rules for how tokens can be combined.
- Use a parser generator to create the parser based on your rules.
- Go through the structure to interpret and run the code.
It's a bit of work, but it's a great way to really understand how interpreters work. Start with the basics and add more as you go.
What interpreter does JavaScript use?
JavaScript runs on interpreters found in web browsers like Chrome and Firefox. The most well-known one is Google's V8 engine in Chrome. V8 breaks down JavaScript code, turns parts of it into optimized machine code using a technique called just-in-time compilation, and then runs it fast.
Do you need an interpreter for JavaScript?
Yes, you do need an interpreter for JavaScript. When you run JavaScript code, the browser's built-in interpreter takes care of:
- Breaking the code into smaller pieces
- Organizing it into a structure
- Turning parts of it into machine code
- Running the code step by step
This process lets JavaScript run right away, without needing to be compiled first.
Is JavaScript one of the 3 languages all web developers must learn?
Yes, JavaScript is one of the three main languages every web developer should know, along with HTML and CSS. HTML is for content and structure, CSS for style and presentation, and JavaScript for making things interactive. Together, they let you create dynamic web pages and full web applications. Learning JavaScript is crucial for anyone wanting to work in web development.