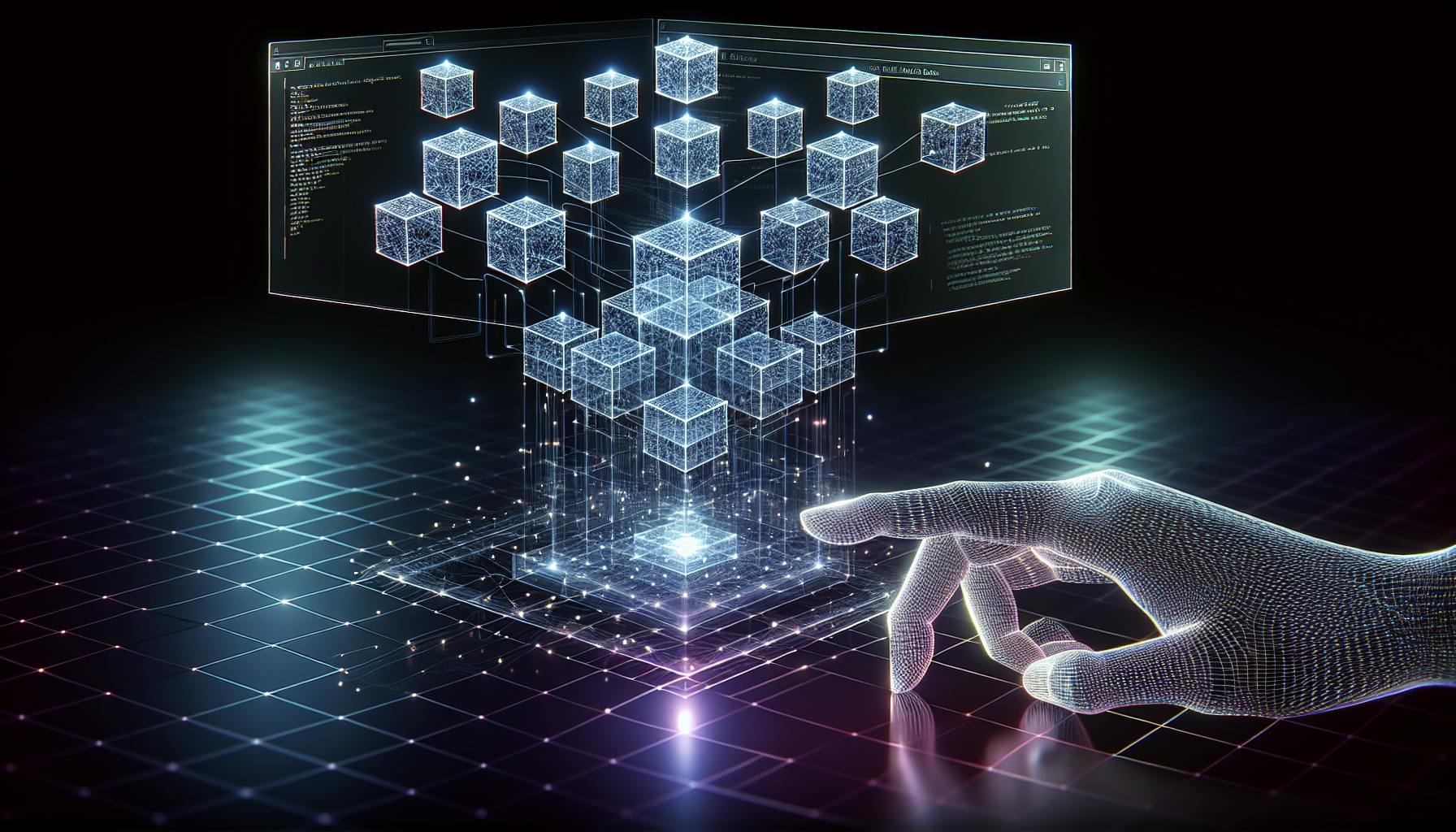
Learn the basics of array objects, including creation, manipulation, and iteration. Discover how array objects can help you organize and manage data efficiently in your coding projects.
Array objects are like versatile toolboxes for managing data efficiently in your coding projects. Here's a quick guide on what you need to know about array objects:
- What Are Array Objects? They're containers that store multiple pieces of information, making it easier to organize and manage data.
- Creating Arrays: Use square brackets
[]
to create an array with items likeconst fruits = ["apple", "banana", "orange"];
. - Accessing and Modifying: Access items using their index, starting at 0, and modify them directly or with methods like
.push()
and.pop()
. - Special Features: Arrays come with methods that allow you to perform operations like adding, removing, changing items, and more.
- Iterating Over Arrays: Use
.forEach()
,.map()
, and.filter()
to work with each item, transform them, or select specific ones based on criteria.
By understanding these basics, you'll be well-equipped to handle and manipulate data effectively in your programming endeavors.
Array Object Definition
An array object lets you put many pieces of info into one container, a bit like putting different types of socks in one drawer. Each piece of info sits in its own spot, starting from spot number 0.
For instance, if you have a bunch of names of products, you can keep them all in one array instead of having to remember a separate spot for each one. This makes it easier to find and manage them.
Some key things to know about arrays:
- They keep things in order - every item has its own number spot.
- You can change them - add new items, take some out, or swap things around.
- They can have the same item more than once.
Arrays can hold all kinds of things - numbers, words, yes/no values, and even other arrays.
Advantages of Array Objects
Arrays are great for a few reasons:
- They keep related things together, which is neater and easier to work with.
- They can do a lot with less effort - like finding or sorting items quickly.
- They're flexible - you can easily change what's inside them.
- You can go through them one by one to do something with each item.
- They're easy to move around - you can copy all the stuff in one array to another.
Arrays come with special tools (methods) that make common tasks simple, like putting things in order or mixing them up.
Array Object Use Cases
You can use arrays for lots of different things:
- Keeping a list of contacts - names, numbers, emails all together.
- Managing a shopping cart - what's being bought and how much of it.
- Tracking scores - who's winning and by how much.
- Organizing events - when they are and what they're about.
- Making playlists - all your favorite songs in one place.
Whenever you need to keep and use many pieces of info together, arrays are a handy tool. They help keep things organized and make working with lots of data easier.
Array Object Syntax and Properties
Array Literal Syntax
Creating an array is simple - just use square brackets []
.
For example:
const fruits = ["apple", "banana", "orange"];
This line makes an array named fruits
that holds 3 types of fruit.
To get a specific fruit out, you just use the fruit's number in square brackets:
const firstFruit = fruits[0]; // "apple"
Remember, arrays start counting at 0, not 1. So, the first item is 0, the second is 1, and so on.
You can fill an array with any kind of data you want:
const numbers = [1, 5, 7];
const booleans = [true, false, true];
The array keeps the order of the items just as you put them in.
Length Property
The length
property tells you how many items are in the array:
const fruits = ["apple", "banana", "orange"];
fruits.length; // 3
You can also change the length
to cut off items or make the array longer.
For example, if you change the length to 2, the last item gets removed:
fruits.length = 2;
fruits; // ["apple", "banana"]
This is handy for clearing an array or setting a certain size.
Indexing in Arrays
Arrays are numbered starting with 0. This means the first item is 0, the second is 1, and so on.
Index: 0 1 2
Array: ["a", "b", "c"]
Use the item's number in square brackets to access or change it:
const letters = ["a", "b", "c"];
letters[1]; // "b" - get the 2nd item
letters[2] = "z"; // change the 3rd item
letters; // ["a", "b", "z"]
If you try to use a number that doesn't exist, like -1, you won't get anything back.
The way arrays are numbered, along with their length, makes it easy to go through each item one by one.
Common Array Object Operations
Arrays have some built-in tricks that help you add, take away, and tweak items easily. These tricks, or methods, make working with arrays a lot smoother.
Array.push() and Array.pop()
The .push()
method lets you tack on one or more items to the end of an array:
let fruits = ["apple", "banana"];
fruits.push("orange");
fruits; // ["apple", "banana", "orange"]
You can keep adding items with .push()
and they'll line up at the end.
The .pop()
method takes off the last item from an array:
fruits.pop();
fruits; // ["apple", "banana"]
Using .push()
and .pop()
, you can add new stuff to the end and remove old stuff from the back, kind of like a line.
Array.unshift() and Array.shift()
.unshift()
is similar to .push()
, but it adds items to the beginning instead:
fruits.unshift("strawberry");
fruits; // ["strawberry", "apple", "banana"]
The .shift()
method takes away the first item, just like .pop()
does with the last one:
fruits.shift();
fruits; // ["apple", "banana"]
Array.splice() Method
The .splice()
method is for cutting and adding items wherever you want in an array.
It needs 3 things:
- Where to start
- How many items to take out
- What items to put in (this last part is optional)
For example, to take out 1 item from the second spot:
fruits.splice(2, 1);
To add an item at the first spot:
fruits.splice(1, 0, "lemon");
And you can do both at the same time:
fruits.splice(2, 1, "pineapple", "kiwi");
This means you take 1 item out from the second spot, and then put in pineapple and kiwi. The .splice()
method is great for making specific changes to arrays.
sbb-itb-bfaad5b
Iterating, Mapping and Filtering
Array.forEach()
The .forEach()
method is like telling your computer to go through each item in an array and do something with it. It's like going through a list and checking off each item as you go.
Here's how you use it:
const fruits = ["apple", "banana", "orange"];
fruits.forEach(function(fruit, index) {
console.log(fruit, index);
});
// This will show:
// apple 0
// banana 1
// orange 2
Important points about .forEach()
:
- It doesn't give you anything back.
- You can't skip over items like in a for loop.
- It's great for when you just need to use each item in some way.
Array.map()
The .map()
method changes each item in an array into something else based on a rule you give it.
For turning all fruit names to uppercase:
const fruits = ["apple", "banana", "orange"];
const uppercased = fruits.map(fruit => {
return fruit.toUpperCase();
});
// Results in: ["APPLE", "BANANA", "ORANGE"]
Things to remember about .map()
:
- It creates a new array with the updated items.
- The new array has the same number of items.
- If the rule is simple, you don't need the word 'return'.
Array.filter()
The .filter()
method gives you a new array with only the items that pass a test you set.
To get just the even numbers:
const numbers = [1, 2, 3, 4];
const evens = numbers.filter(n => {
return n % 2 === 0;
});
// You get: [2, 4]
Key points about .filter()
:
- It gives you a smaller array based on your test.
- It doesn't change the items, just picks some based on your criteria.
Conclusion
Think of array objects like a toolbox for keeping your data tidy and in order. Here's a quick recap of what we've learned about them:
- Arrays are like lists that keep different bits of info in a line, starting with the number 0. This setup makes finding and using your data straightforward.
- They have a special count, called a length, that tells you how many items are in the list. You can even change this count to make the list longer or shorter.
- Arrays have some cool tricks (methods) like
.push()
,.pop()
,.shift()
, and.splice()
for adding, removing, or changing items in your list. - You can use
.forEach()
to go through your list and do something with each item. - With
.map()
and.filter()
, you can make new lists either by changing items in the original list or picking out certain items that meet specific criteria.
Now that you've got the basics down, you're ready to use arrays to organize data in your coding projects. Arrays are super useful and can make dealing with lots of data much simpler. The more you use them, the better you'll get at managing and manipulating information in your code.