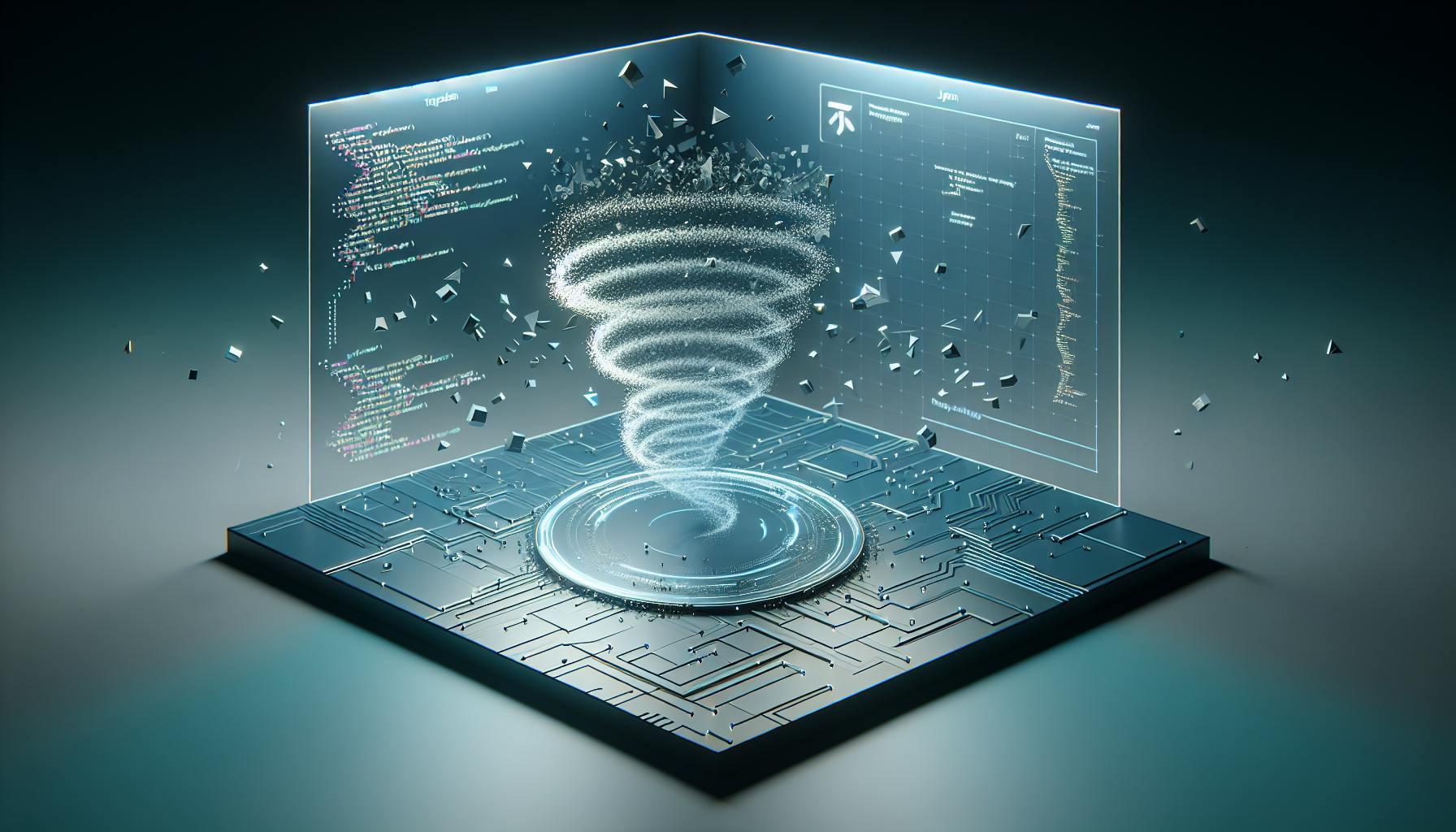
Learn about npm and tsc essentials for developers working with TypeScript. Set up your project, configure tsconfig.json, use the TypeScript compiler, and follow best practices for efficient development.
If you're diving into the world of TypeScript, understanding how to use npm (Node Package Manager) and tsc (TypeScript Compiler) is crucial. Here's a quick overview to get you started:
- npm is used to manage dependencies in your project, including installing TypeScript itself.
- tsc converts TypeScript code into JavaScript, making it understandable for web browsers and servers.
To set up your TypeScript project, you’ll first need Node.js and npm. Then, you can create a project folder, initialize npm, and install TypeScript. The tsconfig.json
file is key for configuring how the TypeScript compiler works for your project. When it comes to writing code, choosing the right editor (like Visual Studio Code or WebStorm) can significantly enhance your development experience by providing helpful features such as code suggestions and built-in debugging tools.
Here are some best practices to follow:
- Manage your dependencies wisely.
- Automate repetitive tasks with npm scripts.
- Keep your TypeScript compiler (
tsc
) running in watch mode for automatic recompilation. - Write tests to ensure your code works as expected and aim for high code coverage.
Remember, staying updated with the latest TypeScript features and community best practices can make your development process more efficient and enjoyable.
Installing Node.js and npm
To start using TypeScript, first, you need Node.js and npm on your computer. Here's how to do it:
- Visit nodejs.org and download the LTS (Long Term Support) version of Node.js for your system. This step also installs npm for you.
- After installation, open your terminal (or command prompt) and check if Node.js and npm are correctly installed by typing:
node -v
npm -v
- If you don't see the version numbers, try restarting your terminal. Then, check again.
Now you have Node.js and npm ready, and you can begin working on a TypeScript project.
Setting Up a TypeScript Project
- First, make a new folder for your project and open your terminal in that folder.
- Start by setting up npm in your project:
npm init -y
- Next, add TypeScript to your project by installing it:
npm install typescript --save-dev
- To get ready for TypeScript, create a config file by running:
npx tsc --init
- Now, open the
tsconfig.json
file that was created. Here, you can adjust settings like which version of JavaScript you want your TypeScript to be turned into, how modules work, and more.
You're all set to write and compile TypeScript code in your new environment!
Using the TypeScript Compiler (tsc)
The TypeScript compiler, or tsc, is a tool that changes TypeScript code into regular JavaScript. This means you can write your code in TypeScript, which adds extra rules to help catch errors, but still run it anywhere that JavaScript works.
Installing tsc globally
You can set up tsc on your computer for use in any project by running:
npm install -g typescript
This command makes the TypeScript compiler and the tsc command available everywhere on your system.
Compiling TypeScript Code
To turn a TypeScript file into a JavaScript file, you use the tsc command like this:
tsc hello.ts
This command creates a file named hello.js with the JavaScript version of your code.
If you're working on a bigger project, you can compile everything at once by telling tsc to look at your tsconfig.json file:
tsc -p tsconfig.json
tsc Compiler Options
Here are some useful tsc commands to know:
-t TARGET
: This lets you pick which version of JavaScript you want your TypeScript to turn into (for example, ES5).--module SYSTEM
: This tells tsc how to organize your code into modules (like CommonJS).-w
: This keeps tsc running and automatically recompiles your code when it changes.--sourcemap
: This creates a sourcemap, which is a way to easily debug your code by showing you where the original TypeScript code is in the compiled JavaScript.
For more details on these options, you can check out the tsc compiler options documentation.
Configuring tsconfig.json
Setting up your tsconfig.json file is a key step when you're using TypeScript. This file helps you tell the TypeScript compiler (tsc) exactly how to turn your TypeScript code into JavaScript code. It's like giving directions on how to get from point A (TypeScript) to point B (JavaScript).
Creating a tsconfig.json
You can get a tsconfig.json file going in two ways:
- You can create a tsconfig.json file yourself and put it in your project's main folder.
- Or, you can open your terminal, go to your project's folder, and type
tsc --init
. This command asks tsc to make a basic tsconfig.json file for you.
Key tsconfig.json Options
Here are some important settings you might want to tweak:
- compilerOptions - This is where you set up how tsc changes your TypeScript into JavaScript. Options here include:
- target - Decides what version of JavaScript you end up with (like ES5 or ES6).
- module - Picks the system for organizing your code (like CommonJS or ES6).
- outDir - Tells tsc where to put the JavaScript files it makes.
- include - Lists the files or folders you want tsc to work on.
- exclude - Lists the files or folders you don't want tsc to touch.
- extends - Lets you use settings from another tsconfig.json file.
Sample tsconfig.json
Here's a simple example of what your tsconfig.json might look like:
{
"compilerOptions": {
"target": "ES6",
"module": "commonjs",
"outDir": "dist"
},
"include": [
"src/**/*.ts"
],
"exclude": [
"node_modules"
]
}
With these settings, you're asking tsc to:
- Change your TypeScript into ES6 JavaScript
- Use CommonJS for organizing your code
- Put the resulting JavaScript in a folder named
dist
- Work on TypeScript files in the
src
folder - Ignore anything in
node_modules
Getting your tsconfig.json file right helps make sure tsc does exactly what you want when turning your TypeScript code into JavaScript.
TypeScript Development Environment
Editor/IDE Support
When you're coding with TypeScript, you can use many different code editors or IDEs (which are like super-powered editors). These tools make your coding life easier by helping with things like suggesting code as you type and finding mistakes.
Visual Studio Code is a free editor that's great for TypeScript. It helps you with:
- Suggesting code and fixes
- Letting you debug code right in the editor
- Making it easy to change and improve your code
- Having a built-in place to type commands
- Setting up custom tasks for building your code
WebStorm is another tool that's all about TypeScript. It gives you:
- Suggestions for finishing your code
- Instant checks for errors
- Tips about types and parameters when you hover over code
- Easy ways to find and go to code definitions
- Tools for changing your code
- Testing tools
Both VS Code and WebStorm use TypeScript's type system to give you better coding suggestions. Setting up your editor to use these features well is key to coding faster and smarter.
Debugging TypeScript
Fixing bugs in TypeScript can be made easier by:
- Turning on sourcemaps in your
tsconfig.json
. This links your JavaScript code back to your original TypeScript, making it easier to see where things go wrong. - Using the debugging tools in your editor that work with sourcemaps. VS Code and WebStorm are both good at this.
- Setting breakpoints right in your TypeScript code, so you can pause there and see what's happening.
With sourcemaps, you can debug as if you were working directly in TypeScript, avoiding the confusion of switching between TS and JS files. Using your editor's debugging tools along with this makes for a powerful setup to catch and fix bugs early.
sbb-itb-bfaad5b
Best Practices with npm and tsc
When working with TypeScript, it's important to manage your project smartly to make your coding life easier. Here's a straightforward guide on how to do just that:
Dependency Management
- When adding libraries to your project, use a special format (like
~1.2.3
) in yourpackage.json
file. This way, you automatically get small updates that fix bugs. - Regularly check for security issues in the libraries you're using by running
npm audit
. - Only add libraries you really need to your project. If you find you're not using one, go ahead and remove it.
Task Automation
- You can make repetitive tasks like building your code or checking its style easier by setting up
npm scripts
. - Keep your code compiling automatically as you work by using
tsc -w
. This saves you from having to manually compile every time you make a change. - Before you commit your code, use tools like Husky to automatically check that your code builds and passes tests.
Testing
- Make sure your code works as expected by writing tests. You can use tools like Jest, Mocha, or Jasmine for this.
- Run your tests automatically with
npm test
. - Use coverage tools to see how much of your code is tested. Try to cover at least 80% of it.
Staying Current
- Keep up with new updates by reading the TypeScript release notes.
- Follow blogs and watch videos about TypeScript to learn new tips and tricks.
- Try out new project templates and tools as they come out.
- Join TypeScript forums and talk with other developers to learn from them.
Following these simple steps will help you write better TypeScript code, reduce errors, and save time on manual tasks. Plus, keeping up with the latest in TypeScript can make coding more fun and help you improve your skills.
Conclusion
Understanding npm and tsc is super important if you're working with TypeScript. They help you manage your project smoothly and let you focus on coding rather than fixing problems.
Here's what you need to remember:
- npm takes care of your project's needs - It's like a helper that gets you TypeScript and any other tools or libraries your project needs. This makes setting up your project a lot easier.
- tsc turns your TypeScript into JavaScript - Since browsers and servers use JavaScript, not TypeScript, you need tsc to translate your code. Learning how to use tsc is crucial.
- Set up your tsconfig.json carefully - This file tells tsc how to do its job. Setting it up right from the start can save you trouble later on.
- Use the right editor/IDE - A good code editor that understands TypeScript can make your work much faster and easier. It can also help you find and fix errors quickly. Remember to use source maps for easier debugging.
- Stick to best practices for managing your project - Keep your project organized by managing dependencies, automating tasks, and testing your code properly. This keeps your work efficient and helps you avoid problems.
Learning about npm and tsc is definitely worth it. Once you get the hang of it, you'll be able to work faster, make fewer mistakes, and handle bigger projects with confidence. Getting these basics down is key to making the most out of TypeScript.
Related Questions
What is TSC in NPM?
npm lets you install TypeScript on your computer so you can use the tsc command in your terminal. To do this, just type:
npm install -g typescript
This installs the latest version of TypeScript (right now, that's version 5.3). The tsc command helps turn your TypeScript code into JavaScript. So, by using npm, you can easily set up tsc on your computer to work on TypeScript projects.
What is TS toolbelt?
TS toolbelt is a package full of tools to help you work with TypeScript types more easily. Here are some things it can help you do:
- Work with different types of data (like objects, unions, functions, and literals) in advanced ways
- Make software components that are easy to use again, flexible, and safe to work with
- Make complicated type changes simpler
- Make your code safer by checking and organizing types better
Think of it like having a belt full of handy tools that make dealing with TypeScript types much simpler and more efficient.