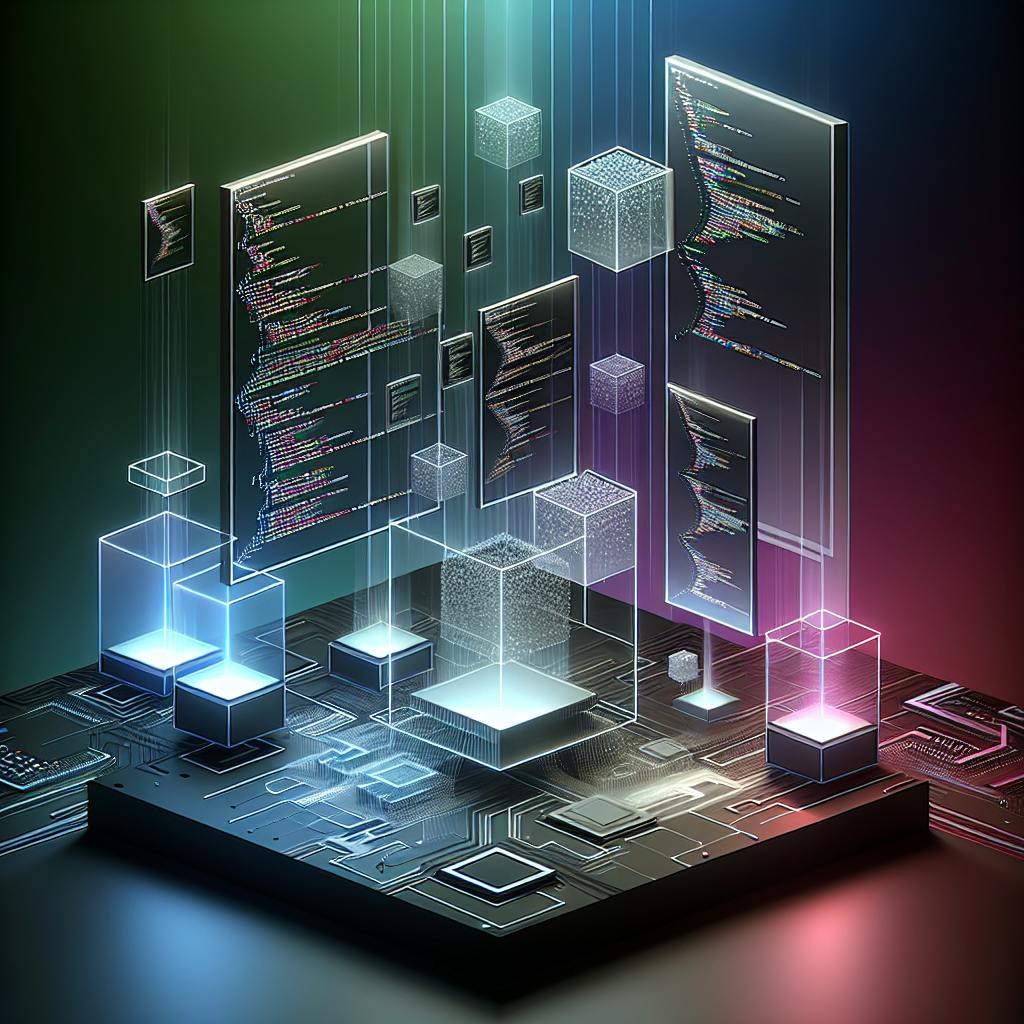
Explore the importance of Objective-C for maintaining legacy iOS apps, including setup, basics, best practices, and transitioning to modern Swift development.
Objective-C remains crucial for maintaining and updating legacy iOS applications, despite the introduction of Swift. This guide covers:
-
Why Objective-C Still Matters: Objective-C's historical significance and continued relevance for working with existing iOS projects.
-
Setting Up for Objective-C Work: Configuring the development environment, including Xcode, for Objective-C development.
-
Objective-C Basics: Understanding Objective-C syntax, data types, control flow, and functions.
-
Advanced Objective-C Topics: Exploring classes, objects, inheritance, protocols, categories, blocks, memory management, and essential frameworks.
-
Writing Good Objective-C Code: Best practices for coding standards, memory management, debugging, error handling, and performance optimization.
-
Moving to Modern iOS Development: Strategies for using Objective-C and Swift together, converting to Swift, and choosing a language for new features.
By mastering Objective-C, you'll be equipped to maintain and update your legacy iOS apps while transitioning to modern iOS development practices.
Quick Comparison: Objective-C vs. Swift
Criteria | Objective-C | Swift |
---|---|---|
Language Type | Object-Oriented | Multi-Paradigm |
Memory Management | Manual (ARC) | Automatic Reference Counting (ARC) |
Syntax | Verbose | Concise |
Performance | Faster | Slightly Slower |
Interoperability | Native | Requires Bridging |
Adoption | Legacy | Modern |
Setting Up for Objective-C Work
To maintain and update legacy iOS applications, you need to set up a development environment for Objective-C work. This section will guide you through the process of configuring your tools and environment to work efficiently with Objective-C.
Getting Xcode Ready for Objective-C
To start developing with Objective-C, you need to have Xcode installed on your Mac. If you haven't already, download and install Xcode from the App Store. Once installed, launch Xcode and proceed with the initial setup.
Understanding the Xcode Interface
Familiarize yourself with the Xcode interface to develop efficiently. The Xcode interface is divided into several areas:
Area | Description |
---|---|
Project Navigator | Find your project files and folders here. |
Editor Area | Write your code here. |
Utilities | Get additional information and tools to help with your project. |
Creating a New Objective-C Project
To create a new Objective-C project in Xcode, follow these steps:
1. Create a New Xcode Project: Select Create a New Xcode Project from the Welcome Screen or go to File > New > New Project... from the menu.
2. Select a Template: In the Mac OS X section, select Application.
3. Choose a Project Type: In the upper-right pane, select Command-Line Tool followed by Next.
4. Enter Project Details: Enter a Product Name, Organization Name, and Company Identifier.
5. Configure Project Settings: Make sure the project Type is set to Foundation and the checkbox for Use Automatic Reference Counting is selected.
6. Save Your Project: Choose a location to save your project and click Create.
By following these steps, you'll have a new Objective-C project set up and ready for development.
Objective-C Basics
This section covers the fundamental concepts and syntax of Objective-C, providing a solid foundation for understanding legacy codebases.
Objective-C Syntax and Structure
Objective-C is built on top of the C programming language and adds object-oriented features. It uses a unique syntax for messaging, where objects communicate by sending messages to each other. A basic Objective-C program consists of classes, objects, and methods.
Here's an example of a basic Objective-C class:
@interface Person : NSObject
@property (nonatomic, copy) NSString *name;
- (void)sayHello;
@end
@implementation Person
- (void)sayHello {
NSLog(@"Hello, my name is %@!", self.name);
}
@end
Data Types, Variables, and Constants
Objective-C provides various data types, including:
Data Type | Description |
---|---|
int |
Whole numbers |
float |
Decimal numbers |
char |
Single characters |
NSString |
Strings of characters |
NSArray |
Arrays of objects |
Variables are declared using the type variableName;
syntax, and constants are declared using the const type variableName;
syntax.
Control Flow: Loops and Conditions
Objective-C provides various control flow structures, including:
Control Flow | Description |
---|---|
if-else |
Conditional statements |
for |
Loops that iterate over a sequence |
while |
Loops that repeat until a condition is met |
switch |
Statements that execute different blocks of code based on a value |
Here's an example of an if-else
statement:
if (age > 18) {
NSLog(@"You are eligible to vote.");
} else {
NSLog(@"You are not eligible to vote.");
}
Defining and Calling Functions
Functions in Objective-C are defined using the returnType functionName(parameters);
syntax, and called using the functionName(parameters);
syntax.
Here's an example of defining and calling a function:
- (void)sayHello:(NSString *)name {
NSLog(@"Hello, my name is %@!", name);
}
[sayHello:@"John"]; // calling the function
By mastering these basic concepts, you'll be well on your way to understanding and working with legacy Objective-C codebases.
Advanced Objective-C Topics
This section covers more complex aspects of Objective-C that are essential for understanding and improving legacy applications.
Classes, Objects, and Inheritance
Classes, Objects, and Inheritance
Objective-C implements object-oriented programming concepts such as classes, objects, inheritance, and polymorphism. A class defines the properties and methods of an object. Classes can inherit behavior from parent classes, allowing for code reuse and a more hierarchical organization of code. Polymorphism enables objects of different classes to be treated interchangeably, providing flexibility and code reuse.
For example, imagine creating a music app with a Song
class that defines properties like title, artist, and duration. Each song in the app would be an object belonging to this class!
Protocols and Categories
Protocols and Categories
Protocols define a set of methods that a class can implement to adhere to a particular behavior. Categories allow you to add custom behavior to existing classes without creating a subclass.
For example, let's say we have a NSString
class and we want to add a method that counts the number of vowels in a string. Instead of creating a new subclass, we can just create a category on NSString
and add our method there.
Category | Description |
---|---|
NSString (VowelCounting) |
Adds a method to count the number of vowels in a string |
@interface NSString (VowelCounting)
- (int)vowelCount;
@end
@implementation NSString (VowelCounting)
- (int)vowelCount {
int vowelCount = 0;
for(int i=0; i<[self length]; i++) {
char c = [self characterAtIndex:i];
if(c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u') {
vowelCount++;
}
}
return vowelCount;
}
@end
Now any instance of NSString
has access to this vowelCount
method!
Blocks and Memory Management
Blocks and Memory Management
Blocks are a powerful feature in Objective-C that allow you to create closures, which are functions that have access to their own scope and can capture variables from that scope. Blocks are commonly used for asynchronous programming, where you need to execute a block of code after a certain operation has completed.
Memory management in Objective-C is crucial to avoid memory leaks and crashes. You need to manually manage memory using retain, release, and autorelease, which can be error-prone. However, with the introduction of Automatic Reference Counting (ARC), memory management has become much simpler.
Foundation and UIKit Frameworks
Foundation and UIKit Frameworks
The Foundation framework provides a set of classes and protocols that are essential for building iOS apps, including NSString
, NSArray
, and NSDictionary
. The UIKit framework provides a set of classes and protocols for building user interfaces, including UIViewController
, UIView
, and UITableView
.
By mastering these advanced Objective-C topics, you'll be well on your way to building robust and efficient legacy iOS applications.
sbb-itb-bfaad5b
Writing Good Objective-C Code
When developing legacy iOS applications, it's essential to follow best practices for writing efficient, maintainable, and high-quality Objective-C code. This section shares guidelines and techniques to help you achieve this goal.
Coding Standards and Conventions
Coding Standards and Conventions
To ensure consistency and readability in your Objective-C code, follow these coding standards and conventions:
-
Use descriptive names for variables, such as
userName
oruserAge
. -
Organize your code in a logical manner.
-
Use whitespace effectively to make your code easy to read.
Managing Memory Effectively
Managing Memory Effectively
Effective memory management is critical in Objective-C to avoid memory leaks and crashes. Here are some guidelines to manage memory effectively:
Memory Management Technique | Description |
---|---|
Automatic Reference Counting (ARC) | Automatically manages memory for you. |
Retain and Release | Manually manage memory using retain and release. |
Autorelease Pools | Manage temporary objects using autorelease pools. |
Debugging and Error Handling
Debugging and Error Handling
Debugging and error handling are essential skills for any developer. Here are some useful debugging techniques:
-
Use
NSLog
to print messages to the console. -
Set breakpoints to halt execution at specific points in your code.
-
Inspect variables and memory using Xcode's debugging tools.
Optimizing Performance
Optimizing Performance
Optimizing the performance of your Objective-C code is critical for a smooth user experience. Here are some strategies to optimize performance:
Performance Optimization Technique | Description |
---|---|
Minimize Memory Allocations | Reduce memory allocations and deallocations. |
Caching | Reduce computation time by caching results. |
Algorithm Optimization | Optimize algorithms for performance. |
Profiling | Use Instruments to identify performance bottlenecks. |
By following these guidelines and techniques, you'll be able to write high-quality, efficient, and maintainable Objective-C code for your legacy iOS applications.
Moving to Modern iOS Development
Moving to modern iOS development is essential for developers working on legacy iOS applications. This section guides you on how to transition from legacy Objective-C codebases to modern iOS development practices, including Swift.
Using Objective-C and Swift Together
When working on a project that involves both Objective-C and Swift, you need to understand how to maintain interoperability between the two languages. One approach is to use a bridging header file, which allows you to expose Objective-C code to Swift.
Here's how to use a bridging header file:
Step | Description |
---|---|
1 | Add Objective-C code to a Swift project or vice versa. |
2 | Xcode automatically generates a bridging header file. |
3 | Use the bridging header file to expose Objective-C code to Swift. |
Another important file to consider is the {project-name}-swift.h
header file, which exposes Swift code to Objective-C.
Converting to Swift
Converting your Objective-C codebase to Swift can be a challenging task. Here are some strategies to make the transition smoother:
Strategy | Description |
---|---|
Start small | Convert small portions of your codebase, such as a single feature or module. |
Understand differences | Learn about the differences between Objective-C and Swift, such as memory management and syntax. |
Choosing a Language for New Work
When deciding which language to use for new features in your legacy app, consider the following factors:
Factor | Description |
---|---|
Complexity | If you're working on a complex feature, consider using Objective-C or Swift depending on your needs. |
Team expertise | Choose a language based on your team's expertise. |
Future-proofing | Consider using Swift for new features to future-proof your app. |
By considering these factors, you can make an informed decision about which language to use for new features in your legacy app.
Wrapping Up
Key Takeaways
In this guide, we've covered the essential aspects of using Objective-C for legacy iOS app development. You now have a solid foundation to maintain and update your legacy iOS applications.
Remember:
-
Understand the syntax, data types, and control flow of Objective-C to write efficient and reliable code.
-
Follow best practices and coding standards to ensure your codebase remains maintainable and scalable.
Learning Resources
To further enhance your Objective-C skills, explore the following resources:
Resource | Description |
---|---|
"Programming in Objective-C" by Stephen Kochan | A comprehensive book covering advanced Objective-C topics. |
Apple Developer Documentation | Official documentation providing in-depth information on iOS frameworks, libraries, and best practices. |
Online Communities and Tutorials | Join iOS developer communities, such as Stack Overflow and GitHub, to connect with other developers and access tutorials and code samples. |
Keep Learning and Growing
Working with legacy code can be challenging, but it's an essential aspect of iOS development history. By mastering Objective-C, you'll not only be able to maintain and update your legacy apps but also develop a deeper understanding of the iOS ecosystem.
Stay ahead in iOS development by:
-
Embracing the challenges of working with legacy code.
-
Continuously learning and growing to stay up-to-date with the latest developments.
FAQs
Why use Objective-C instead of Swift?
If you're working with C or C++ third-party frameworks, Objective-C might be a better choice. This is because Swift needs a separate wrapper to work with these frameworks, which can be problematic. This is especially important when working with legacy codebases or projects that heavily rely on these frameworks.
Should I learn Objective-C before Swift?
While it's recommended that new developers start with Swift, Objective-C has its advantages. It has a superior runtime compared to Swift, making it a better choice for projects that use powerful SDKs. Additionally, if you're working with foundation APIs like CoreFoundation, AVFoundation, or CoreAnimation, Objective-C is a better language to use. However, for future-proof projects, Swift is the way to go.
Language | When to Use |
---|---|
Objective-C | When working with C or C++ third-party frameworks, or with foundation APIs like CoreFoundation, AVFoundation, or CoreAnimation. |
Swift | For future-proof projects, or when you don't need to work with C or C++ third-party frameworks. |