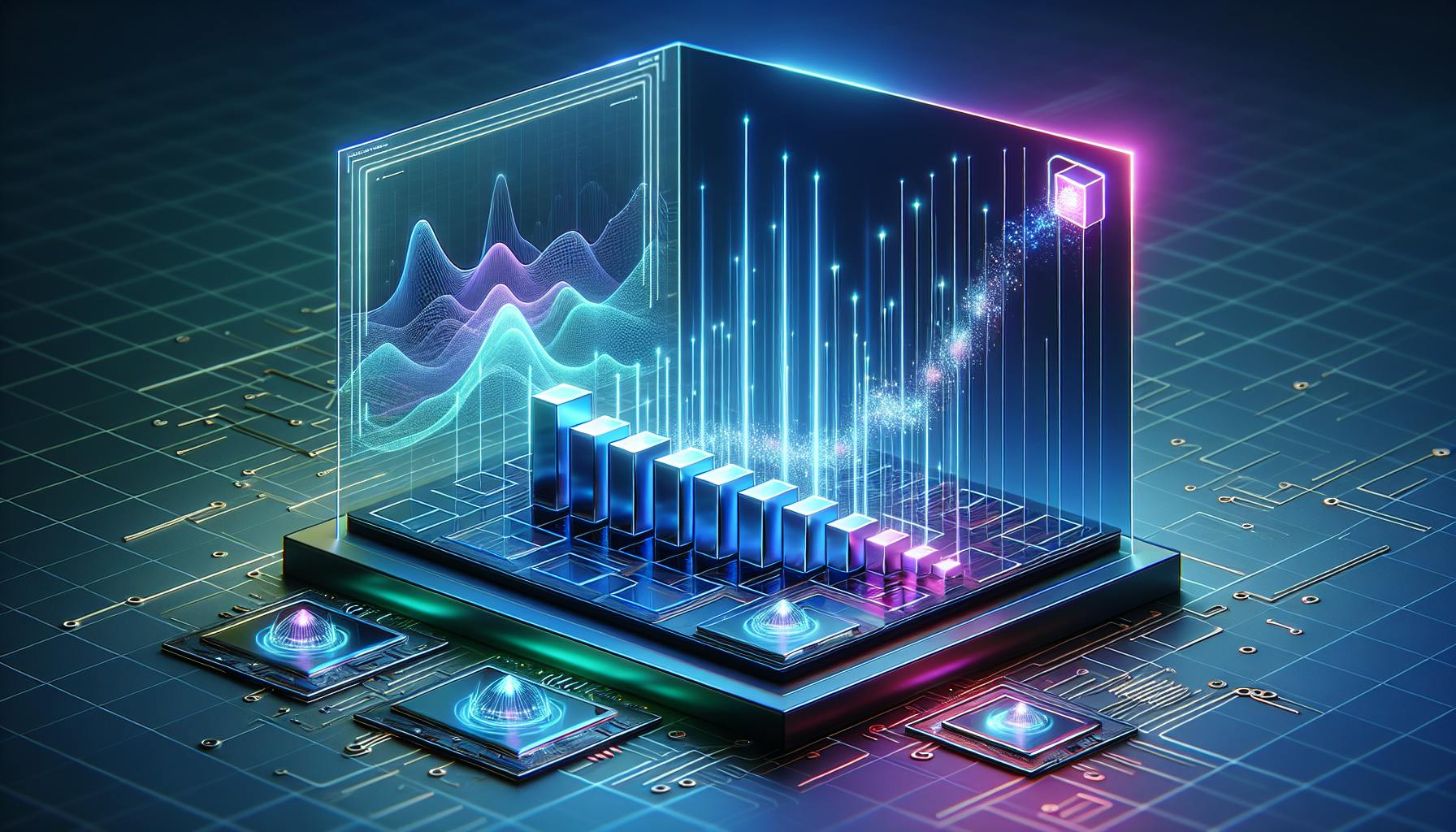
Learn how to manipulate arrays with pop() and push() methods in JavaScript. Discover the basics of arrays, accessing elements, properties, and methods.
Understanding how to manipulate arrays with pop()
and push()
methods in JavaScript is crucial for managing data effectively. These methods allow you to add or remove items from the end of an array, playing a pivotal role in data structure manipulation such as implementing stacks and queues. Here's a quick overview:
push()
Method: Adds one or more elements to the end of an array and returns the new length of the array.pop()
Method: Removes the last element from an array and returns that element.
These methods not only help in straightforward addition and removal of elements but also in more complex operations like transferring items between arrays or maintaining logs. For operations at the start of an array, JavaScript offers the shift()
and unshift()
methods, which work similarly to pop()
and push()
but affect the first element of the array.
This introduction aims to provide a concise explanation of the pop()
and push()
methods in JavaScript, showcasing how they can be used to manipulate array data effectively.
Declaring Arrays
Creating an array in JavaScript is as simple as putting square brackets []
. Here's what it looks like:
let fruits = []; // This is an empty array
let numbers = [1, 2, 3]; // Here's an array with some numbers in it
If you want to mix things up, you can put different types of items in your array:
let mix = [1, "hello", true];
And if you need an array within an array, you can do that too:
let matrix = [[1,2], [3,4]];
Accessing Array Elements
Getting to the items in your array is easy. Just use the item's number in the line, starting with 0:
let fruits = ['apple', 'banana', 'orange'];
let first = fruits[0]; // This gets you 'apple'
let second = fruits[1]; // And this gets you 'banana'
let last = fruits[2]; // Finally, this gets you 'orange'
You can also change items by giving them a new value:
fruits[1] = 'mango'; // Now your fruits array looks like ['apple', 'mango', 'orange']
Array Properties and Methods
Finding out how many items are in your array is super easy with the .length
property:
let nums = [1, 2, 3, 4];
nums.length; // This tells you there are 4 items
Here are some handy tools (methods) for working with arrays:
push()
- adds an item to the endpop()
- takes the last item offunshift()
- puts an item at the startshift()
- removes the first item
These tools change the original array when you use them.
The Push Method
The push()
method in JavaScript lets you add one or more items to the end of a list (array) and tells you how long the list is after adding them.
Syntax
Here's how you use push()
:
array.push(element1, element2, ..., elementN)
array
is your list's nameelement1
,element2
, etc., are the items you want to add
You can add anything like numbers, words, or even other lists.
Return Value
push()
gives back the new length of the list after adding the items.
For example:
let fruits = ['apple', 'banana'];
let length = fruits.push('orange');
// length = 3
Here, push()
says the list is now 3 items long.
Adding Single and Multiple Elements
You can put in one item:
let nums = [1, 2, 3];
nums.push(4);
// nums is now [1, 2, 3, 4]
Or add several items at once:
let languages = ['Python', 'JavaScript'];
languages.push('Java', 'C++');
// languages is now ['Python', 'JavaScript', 'Java', 'C++']
When you use push()
, it changes the original list by adding the new items to the end.
The Pop Method
The pop()
method in JavaScript is like the opposite of push()
. It takes the last item out of your array.
Syntax
Here's how you write it:
array.pop()
You just use .pop()
with your array. It doesn't need anything else to work.
Return Value
When you use pop()
, it gives you back the item it just took out.
For example:
let fruits = ['apple', 'banana', 'orange'];
let removedFruit = fruits.pop();
// removedFruit is now 'orange'
So, pop()
took out 'orange' from the end and told us what it removed.
Repeatedly Popping Elements
You can keep using pop()
to take out one item after another until your array is empty:
let stack = [1, 2, 3, 4];
while(stack.length > 0) {
let removed = stack.pop();
console.log(removed);
// It will show 4, 3, 2, 1 one by one
}
This is a handy way to clear out an array or deal with items one at a time.
Using pop()
with push()
lets you manage your array in a neat way, where you can add items to the end and take them off from the end. This is perfect for situations where you only need to work with the last item you added.
Using Pop and Push Together
The pop()
and push()
methods in JavaScript are like two sides of the same coin. They work together perfectly to help you manage lists in your programs. Let's dive into how you can use them in smart ways.
Implementing a Stack
Think of a stack like a stack of books. You add books to the top and also take from the top. Here's how you can do that with arrays:
let stack = [];
stack.push(1); // Stack now has 1
stack.push(2); // Stack now has 1, 2
stack.push(3); // Stack now has 1, 2, 3
let x = stack.pop(); // x is 3, stack is back to 1, 2
Using push()
to add and pop()
to remove keeps things in order. The last thing you added is the first to go.
Creating a Queue
A queue is like a line at a store. The first person in line is the first to leave. Here's how to set up a queue with arrays:
let queue = [];
queue.push(1); // Queue now has 1
queue.push(2); // Queue now has 1, 2
let y = queue.shift(); // y is 1, queue is now just 2
So, you use push()
to add to the end, and shift()
(a cousin of pop()
) to remove from the start.
Going Back and Forth
Sometimes, you might need to take something out of an array and then put it back. Like this:
let items = [1, 2, 3];
let x = items.pop(); // x is 3
items.push(x); // items is back to 1, 2, 3
This can be useful when you need to work with part of an array for a bit, then return it to its original state.
Other Ways to Use Pop and Push
Here are some simple examples:
- Keeping a log:
let log = [];
log.push('Logged in'); // Add a log entry
log.push('Performed action'); // Add another
- Moving items between arrays:
let first = [1, 2];
let second = [3];
second.push(first.pop()); // Moves 2 from first to second
- Getting the last few items:
let numbers = [1, 2, 3, 4];
let last2 = numbers.splice(-2); // Gets the last 2 items
numbers.push(...last2); // Adds them back
So there you have it. pop()
and push()
are simple but powerful tools for working with arrays in JavaScript. Whether you're building a stack, a queue, or just need to manipulate list items, these methods are essential for coding and development.
sbb-itb-bfaad5b
Alternatives
While push()
and pop()
are great for adding and taking away items at the end of your list, there are two other methods, shift()
and unshift()
, that let you do similar things but at the start of your list.
The Shift Method
array.shift()
is like taking the first item out of your list and giving it to you. It's similar to pop()
, but it works on the first item, not the last.
For example:
let nums = [1, 2, 3];
let first = nums.shift(); // first = 1, nums is now [2, 3]
The Unshift Method
array.unshift()
is the opposite of shift()
. It adds items to the start of your list and tells you how many items are in the list now.
Here's an example:
let nums = [2, 3];
let length = nums.unshift(1); // length = 3, nums is now [1, 2, 3]
So, unshift()
puts items at the start of the list, just like push()
does at the end.
Use Cases
shift()
and unshift()
are useful when you need to work with the first items in your list. You might use them for:
- Handling a line of tasks or people
- Turning a list backward
- Working with the first item for specific needs
While push()
and pop()
are perfect for stacks and some lines, unshift()
and shift()
let you manage your list from both ends.
Conclusion
Let's wrap up what we've learned about using pop and push in JavaScript:
array.push()
helps you add items to the end of an array and tells you the new size. It's a straightforward way to add things without needing to know the last position.array.pop()
takes away the last item from an array and gives it back to you. This is useful when you want to remove items one by one, starting with the most recent addition.
Using these two methods, you can manage your arrays effectively, whether you're adding entries, moving items around, setting up stacks and queues, or just organizing data.
Remember, if you need to work with the beginning of an array, you can use shift and unshift. They're like push and pop but for the front of your array.
In short, these methods help you:
- Easily add or remove items
- Create stacks where the last item added is the first to be removed
- Make queues where the first item added is the first to go
- Transfer items between arrays
- Handle lists in various ways
For most tasks involving adding or taking away elements in arrays, push and pop are your best friends. They're essential for anyone getting into coding & development, especially in JavaScript. Make sure to practice using them!
Related Questions
What is the alternative to pop array in JavaScript?
If you don't want to use pop()
to remove the last item from an array, you can use shift()
instead. The big difference is that shift()
takes away the first item in the array, not the last one. Both pop()
and shift()
are about removing items, but from different ends of the array.
What does the functions pop and push do to an array?
push()
- puts new items at the end of an arraypop()
- takes away the last item from an arrayshift()
- removes the first item from an arrayunshift()
- puts new items at the beginning of an array
How do you push elements into an array in JavaScript?
To add an element to the end of an array, you use the push()
method. It tells you how many items are now in the array and can add several elements at once:
let fruits = ['apple', 'banana'];
fruits.push('orange', 'grapes'); // Now, fruits has ['apple', 'banana', 'orange', 'grapes']
What is the opposite of push array in JavaScript?
The opposite of push()
is pop()
. While push()
adds an item to the end of an array, pop()
removes the last item and gives it back to you:
let fruits = ['apple', 'banana', 'orange'];
let removed = fruits.pop(); // removed is 'orange', and now fruits has ['apple', 'banana']