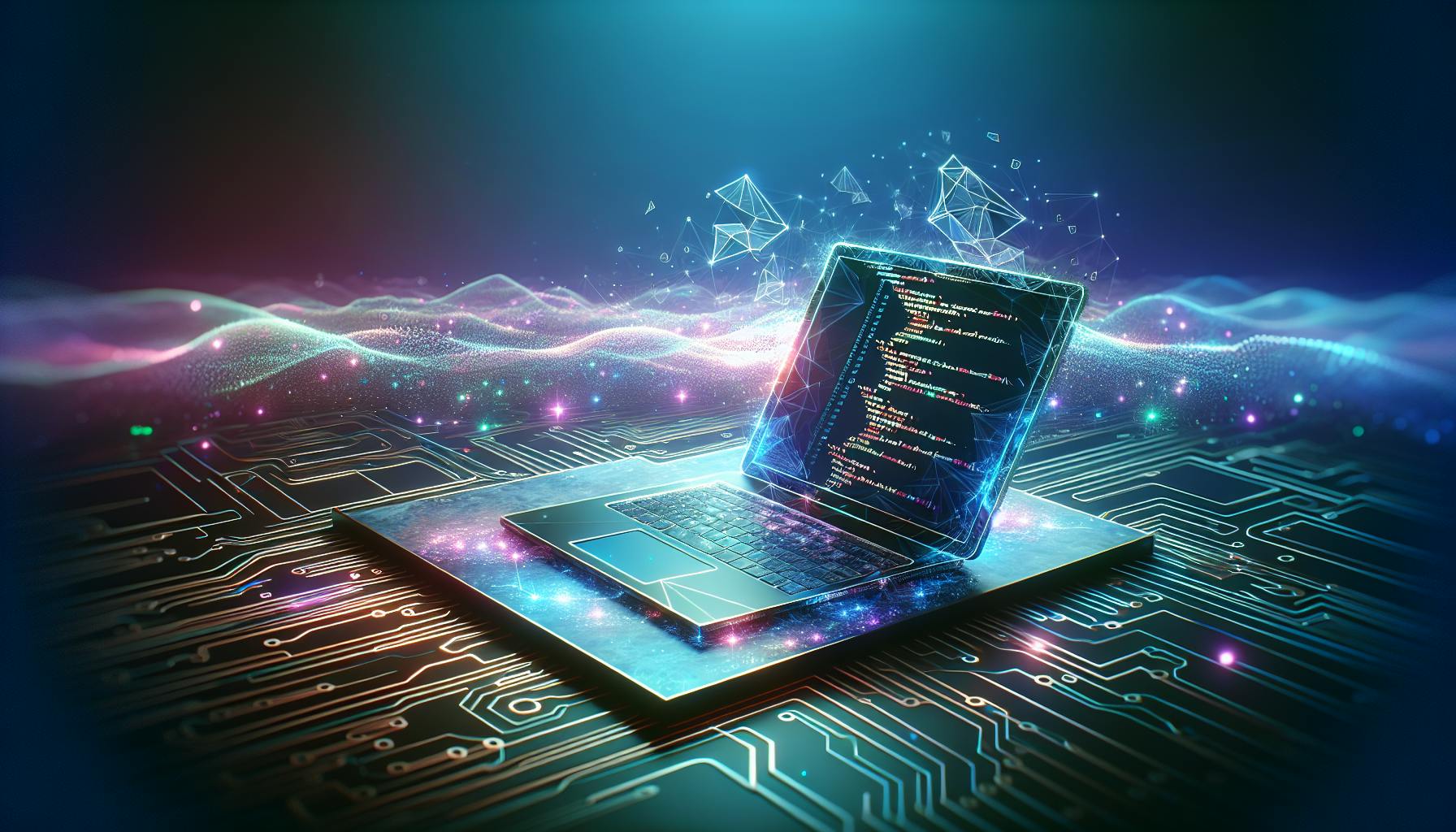
Learn how to create and use arrays of objects in JavaScript, enhance your data management skills, and make your web projects more dynamic and user-friendly.
In this beginner's guide, we'll dive into how to create and use arrays of objects in JavaScript, a fundamental skill for web development. Here's a quick overview:
- Basics of JavaScript Objects: Objects are like containers with labeled info, making data easy to organize and access.
- Understanding Arrays: Arrays let you store a list of items, including objects, in a specific order.
- Creating an Array of Objects: Learn to group objects within an array for organized data management.
- Adding Objects to Arrays: Techniques for adding objects at different positions within an array.
- Manipulating Arrays of Objects: Find, filter, transform, and sort objects in arrays to manage your data effectively.
- Advanced Techniques: Explore loops,
Array.map()
,Array.reduce()
, andObject.fromEntries()
for complex data manipulation.
By mastering arrays of objects, you'll enhance your ability to handle complex data structures, making your web projects much more dynamic and user-friendly.
Key-Value Pairs Explained
Think of a JavaScript object as a list where each item has a name and some info attached to it. For example:
const person = {
name: "John",
age: 30
};
In this example, "name" and "age" are the labels (keys), and "John" and 30 are the pieces of info (values) attached to them. You can use these labels to get the info you want.
Objects can hold many pieces of info, and the info can be different types, like text, numbers, true/false values, lists, or even other objects.
Examples of Simple Objects
Here's how you can create and use objects in JavaScript:
Object with different types of info
const person = {
name: "Sara",
age: 28,
developer: true,
skills: ["JavaScript", "React"]
};
console.log(person.name); // "Sara"
console.log(person.skills[0]); // "JavaScript"
Object that can do something
const dog = {
name: "Rex",
breed: "Labrador",
bark: function() {
console.log("Woof woof!");
}
}
dog.bark(); // "Woof woof!"
Objects let us keep all sorts of data, including actions (like the dog's bark), in a neat and easy-to-use way. This is why they're super handy in JavaScript.
Understanding JavaScript Arrays
Think of JavaScript arrays as a shopping list where you can jot down multiple items on a single piece of paper. Here's what makes them special:
- You can put different kinds of things on the list – like fruits, a number, or even another smaller list.
- Each thing on your list has a number that shows its order, starting with 0.
- Arrays come with handy tools (methods) to add or remove things, find something specific, or do a quick check of everything on the list.
For instance:
const fruits = ["apple", "banana", true, {name: "orange"}];
Here, we have a mix: two fruits by name, a true/false value, and an object (think of it as a box with more info inside).
To pick something from the list, use its number:
fruits[0]; // "apple"
fruits[1]; // "banana"
fruits[2]; // true
fruits[3]; // {name: "orange"}
Some tools (methods) you might find useful are:
.length
- tells you how many items are on the list.push()
- adds a new item to the end.pop()
- takes the last item off.unshift()
- puts a new item at the start.shift()
- removes the first item
Learning about arrays is like learning to organize your shopping list better. They can hold all sorts of things, even lists within lists! This makes them a key part of using JavaScript.
Creating an Array of Objects
First, let's start by making an empty list where our cars will go:
const cars = [];
Now, let's add some cars to our list. Each car is like a mini-box with details inside it:
cars.push({
make: "Toyota",
model: "Corolla",
year: 2022
});
cars.push({
make: "Tesla",
model: "Model 3",
year: 2021
});
Here, we're using a method called push()
to add new cars to our list. Each car has details like make
, model
, and year
.
You can also start your list with cars already in it like this:
const cars = [
{
make: "Toyota",
model: "Corolla",
year: 2022
},
{
make: "Tesla",
model: "Model 3",
year: 2021
}
];
To look at a car's details, we use something called dot notation:
const firstCar = cars[0];
console.log(firstCar.make); // "Toyota"
console.log(firstCar.year); // 2022
And if we want to see every car's model in the list, we can loop through like this:
for (let i = 0; i < cars.length; i++) {
console.log(cars[i].model);
}
This goes through each car and tells us the model.
So, to wrap it up, we make a list, fill it with cars (each having its own set of details), and then we can look at or use those details. It's a neat way to keep all the car info organized.
Adding Objects to an Array
Add a New Object at the Start
To put a new object at the beginning of an array, you can use unshift()
. Here's how it works:
const cars = [
{make: "Toyota", model: "Corolla"},
{make: "Tesla", model: "Model 3"}
];
cars.unshift({make: "Ford", model: "F-150"});
console.log(cars);
// [
// {make: "Ford", model: "F-150"},
// {make: "Toyota", model: "Corolla"},
// {make: "Tesla", model: "Model 3"}
// ]
This method puts the Ford object right at the start of our cars list.
Add a New Object at the End
To add an object to the back of an array, you use push()
like this:
const cars = [
{make: "Toyota", model: "Corolla"},
{make: "Tesla", model: "Model 3"}
];
cars.push({make: "Honda", model: "Civic"});
console.log(cars);
// [
// {make: "Toyota", model: "Corolla"},
// {make: "Tesla", model: "Model 3"},
// {make: "Honda", model: "Civic"}
// ]
The Honda object is added to the end of the list.
Add a New Object in the Middle
If you want to insert an object somewhere in the middle, splice()
is your go-to. It needs 3 things:
- Where to start adding
- How many items to take out (put 0 if you're just adding)
- What to add
Check out this example:
const cars = [
{make: "Toyota", model: "Corolla"},
{make: "Tesla", model: "Model 3"}
];
cars.splice(1, 0, {make: "Ford", model: "Mustang"});
console.log(cars);
// [
// {make: "Toyota", model: "Corolla"},
// {make: "Ford", model: "Mustang"},
// {make: "Tesla", model: "Model 3"}
// ]
This method places the Ford object at the second spot, without getting rid of anything.
sbb-itb-bfaad5b
Manipulating Arrays of Objects
Finding Objects
You can use the Array.find()
method to quickly find the first object in an array that fits what you're looking for. This comes in handy when you're trying to find something specific, like a car of a certain model.
For example:
const cars = [
{make: "Toyota", model: "Corolla", year: 2022},
{make: "Tesla", model: "Model 3", year: 2021},
{make: "Ford", model: "Mustang", year: 1969}
];
const mustang = cars.find(car => car.model === "Mustang");
console.log(mustang);
// {make: "Ford", model: "Mustang", year: 1969}
Here, we're looking for a car that's a Mustang and showing its details when we find it.
Filtering Objects
The Array.filter()
method is great for picking out all the objects that meet a certain condition. It gives you a new array filled with just those objects.
For example, to find all cars made after 2000:
const recentCars = cars.filter(car => car.year > 2000);
console.log(recentCars);
// [
// {make: "Toyota", model: "Corolla", year: 2022},
// {make: "Tesla", model: "Model 3", year: 2021}
// ]
This way, we only get cars that are newer than 2000.
Transforming Objects
You can change objects in an array with the Array.map()
method. This can be for updating values or adding new info.
For example, adding a full name for each car:
const carsWithNames = cars.map(car => {
car.fullName = `${car.make} ${car.model}`;
return car;
});
console.log(carsWithNames[0].fullName); // "Toyota Corolla"
This method goes through each car, adds a full name, and then gives us back the updated list.
Adding Properties to Objects
To add a new feature to every object in an array, you can use Array.forEach()
:
cars.forEach(car => {
car.type = "sedan";
});
console.log(cars[1].type); // "sedan"
This adds a 'type' feature with the value 'sedan' to every car.
Sorting Objects
You can organize an array of objects by a certain feature with Array.sort()
:
cars.sort((a, b) => a.year - b.year);
console.log(cars[0].year); // 1969
We're arranging the cars by their year, from oldest to newest.
Condition Checking
Array.every()
lets you check if all objects meet a certain condition. Array.includes()
sees if a certain value is in the array.
For example:
const allModern = cars.every(car => car.year > 2000); // false
const hasTesla = cars.includes(model => model === "Tesla"); // true
These methods are useful for checking things like if all cars are modern or if there's a Tesla among them.
Advanced Techniques
Sometimes, you might want to mix and match data from different lists into a neat package of objects in JavaScript. Here are some cool tricks to do just that:
Using Loops
Imagine you have two lists: one with labels (like 'name', 'age') and another with the actual info ('John', 30). You can loop through these lists to pair each label with its info in an object.
const labels = ["name", "age"];
const info = ["John", 30];
const data = [];
for (let i = 0; i < labels.length; i++) {
data.push({
[labels[i]]: info[i]
});
}
console.log(data);
// [{name: "John"}, {age: 30}]
This creates a list where each item is a mini-box (object) with a label and info paired up.
Using Array.map()
You can also use Array.map()
to quickly make these mini-boxes:
const labels = ["name", "age"];
const info = ["John", 30];
const data = labels.map((label, i) => ({
[label]: info[i]
}));
console.log(data);
// [{name: "John"}, {age: 30}]
This method goes through each label and pairs it with the corresponding info.
Using Array.reduce()
Or, turn the lists into a single array of mini-boxes with Array.reduce()
:
const labels = ["name", "age"];
const info = ["John", 30];
const data = labels.reduce((acc, label, i) => {
acc.push({
[label]: info[i]
});
return acc;
}, []);
console.log(data);
// [{name: "John"}, {age: 30}]
Here, we're building up our list one mini-box at a time.
Using Object.fromEntries()
Lastly, you can create a single object with all the labels and info using Object.fromEntries()
:
const labels = ["name", "age"];
const info = ["John", 30];
const entries = labels.map((label, i) => [label, info[i]]);
const data = Object.fromEntries(entries);
console.log(data);
// {name: "John", age: 30}
This method turns our pairs of labels and info into a complete object, like a big box holding everything together.
Conclusion
Let's wrap up what we've learned about making and using arrays of objects in JavaScript:
- Objects are like labeled boxes for keeping your data organized.
- Arrays are like lists where you can store lots of different things, including those labeled boxes (objects).
- You can add objects to arrays with simple actions like
.push()
(to add at the end),.unshift()
(to add at the beginning), and.splice()
(to add somewhere in the middle). - Some handy tools for working with arrays of objects are:
.find()
- helps you find the first object that matches what you're looking for.filter()
- lets you get a list of objects that meet certain criteria.map()
- allows you to update data in your objects.sort()
- helps you put your objects in order- For more complex tasks, you can use methods like
.forEach()
and.map()
together with loops or.reduce()
to create objects from lists.
Practicing these skills will help you manage data better in your web projects. You could start by creating an array of objects for something you like, such as video games, books, or desserts. Play around with the information you store and use the methods we talked about to interact with your data.
Getting comfortable with arrays and objects will make a big difference in your JavaScript projects. Have fun experimenting!