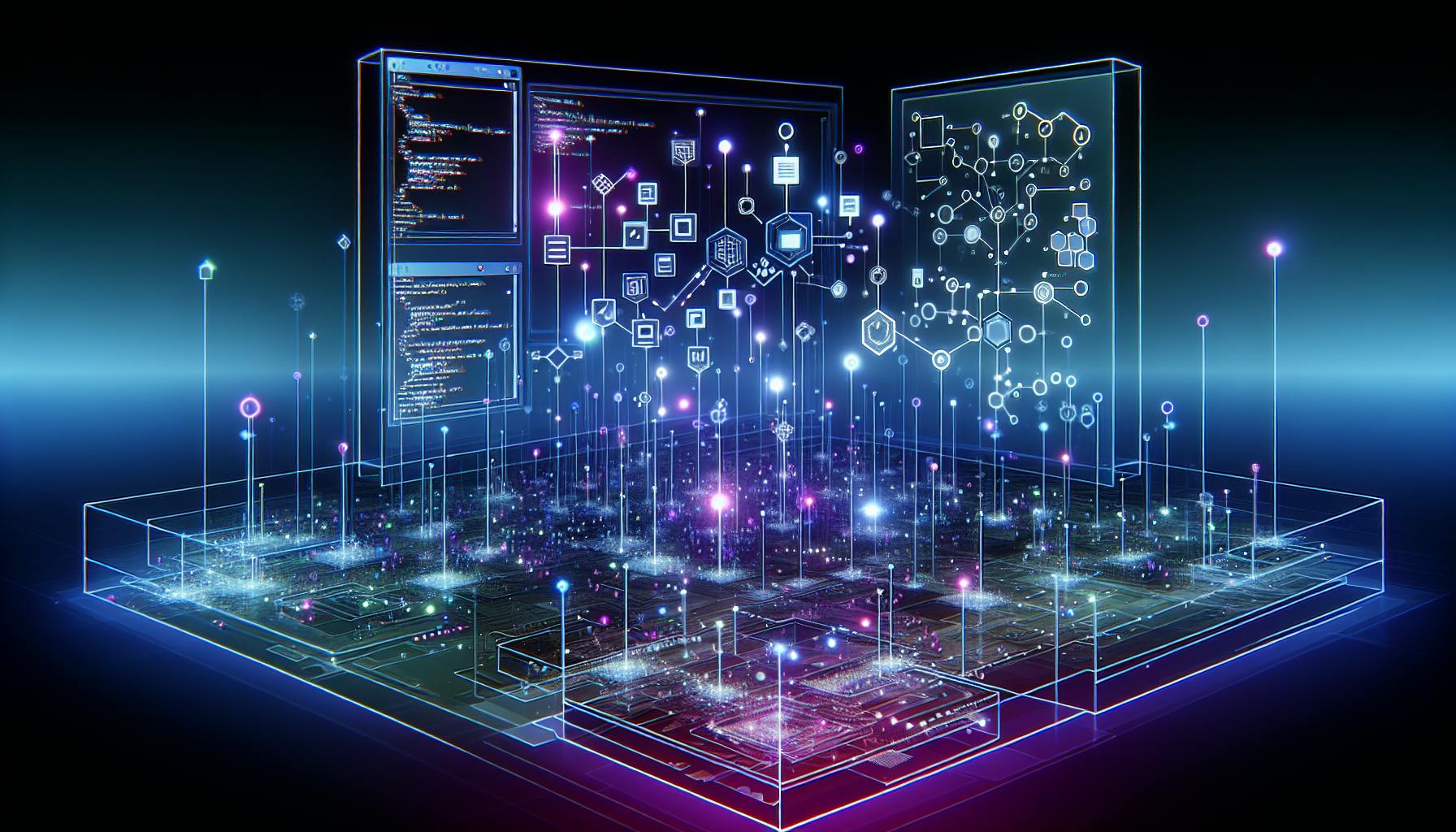
Discover how React Hook Form's useForm simplifies form management, improves performance, and integrates with UI libraries. Learn about managing form state, handling submissions, and more.
If you're diving into the world of React and looking to manage forms efficiently, useForm
from React Hook Form is a game-changer. This community guide provides a comprehensive overview of how useForm
can streamline your form management in React applications. Here's what you need to know in a nutshell:
- Simplifies Form Management: Automates repetitive tasks like input validation, state tracking, and form submission handling.
- Improves Performance: Reduces unnecessary re-renders, enhancing your app's speed.
- Versatile: Compatible with various design systems for aesthetically pleasing forms.
- Customizable Validation: Allows for custom rules for input validation.
- Easy Integration: Works well with UI libraries like Material UI and Ant Design.
By addressing common form-related challenges, useForm
lets you focus on creating user-friendly interfaces. Whether you're dealing with simple or complex forms, this guide walks you through setting up useForm
, managing form state, handling submissions, validating inputs, and integrating with UI libraries. Plus, it explores advanced techniques and real-world examples to get you up and running in no time.
Prerequisites
Before diving into React Hook Form, make sure you have:
- Node.js and npm on your computer to get a React project going
- React version 16.8.0 or newer since it supports hooks
- A basic React project ready, maybe one you made with
create-react-app
Installation
You can add react-hook-form to your project with npm:
npm install react-hook-form
Importing and Setup
First, bring useForm into your React component:
import { useForm } from "react-hook-form";
To start a form, just call useForm()
:
const { register, handleSubmit } = useForm();
- Use
register
to connect input fields handleSubmit
deals with what happens when the form is sent
Here's a simple example to show how it works:
function App() {
const { register, handleSubmit } = useForm();
function onSubmit(data) {
console.log(data);
}
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register("firstName")} />
<button>Submit</button>
</form>
);
}
This is the basic info you need to start using useForm! Next, we'll explore how to make sure inputs are filled out correctly, show error messages, and handle more complicated situations.
Managing Form State
Initializing Form State
To start your form with certain values already filled in, you can set up defaults like this:
const { register, handleSubmit, formState: { values } } = useForm({
firstName: 'John',
lastName: 'Doe'
});
This way, the form begins with firstName
as "John" and lastName
as "Doe". You can look at these starting values anytime with formState.values
.
Updating Form State
To make sure the form updates when someone types in it, use the register
function on your inputs:
<input {...register("firstName")} />
This links the input to your form so it updates automatically as it's filled out.
If you want to manage changes more directly, you can make a function like this:
function handleInputChange(event) {
setValue(event.target.name, event.target.value);
}
Then, add this function to the onChange
part of your input to have more control:
<input
{...register("firstName")}
onChange={handleInputChange}
/>
Accessing Form State
When you need to see what's currently in the form, just check formState.values
:
const { formState: { values } } = useForm();
console.log(values); // Shows current values
You can also show these values on your webpage:
<p>
First Name: {formState.values.firstName}
</p>
This lets you keep track of what's going on with your form at any time using the formState
object.
Handling Form Submission
When you use handleSubmit
from React Hook Form, it makes it super easy to deal with forms when they're sent in. Think of handleSubmit
as a helper that takes care of the form data once the user hits the submit button.
Setting up handleSubmit
First, you need to get handleSubmit
ready in your component:
const { handleSubmit } = useForm();
Next, make a function called onSubmit
that will do something with the form data:
function onSubmit(data) {
// What to do with the data
}
Link this function with handleSubmit
in your form setup like this:
<form onSubmit={handleSubmit(onSubmit)}>
{/* places for users to enter data */}
</form>
Now, whenever the form is submitted, the onSubmit
function will run and handle the data.
Submitting Form Data
The onSubmit
function gets the data automatically. Here's how to see it:
function onSubmit(data) {
console.log(data);
}
To show just the first name from the form, for example:
function onSubmit(data) {
console.log(data.firstName);
}
You could also send this data over to a server:
function onSubmit(data) {
fetch('/api/users', {
method: 'POST',
body: JSON.stringify(data)
});
}
Processing Submissions
Inside your onSubmit
function, you can do whatever you need to after the form is sent in.
Some things you might do:
- Show a message saying everything went well
- Send out emails
- Update information in a database
- Take the user to a new page
For instance:
function onSubmit(data) {
addUserToDatabase(data);
showSuccessMessage();
redirectToHomePage();
}
Using handleSubmit
and onSubmit
helps you keep your code neat and focused, making sure the form does its job without mixing it up with the rest of your app's code.
Form Validation
Defining Validation Rules
To make sure your form inputs are filled out correctly, you can set rules using the register()
function. For example, you can make an email field that checks if the input is actually an email:
const { register } = useForm();
<input
{...register("email", {
required: true,
pattern: /^\\S+@\\S+$/
})}
/>
This code makes sure the email is needed and follows a certain pattern (like name@domain.com).
There are other rules you can use like min
, max
, minLength
, maxLength
, and validate
. Check out the docs to learn more.
Displaying Validation Errors
You can see if there are any mistakes with your inputs by looking at the errors
object from useForm:
const { errors } = useForm();
<input
{...register("email", {required: true})}
/>
{errors.email && <p>Email required</p>}
If there's a problem, like the email field is empty, it'll show a message saying "Email required".
Custom Validation
For rules that are specific to your form, use the validate
option:
{...register("username", {
validate: value =>
value.length > 5 || "Must be longer than 5 chars"
})}
This example checks that the username is more than 5 characters long. The validate
function returns a message if there's an issue or nothing if it's all good.
You can get as detailed as you need with these rules to make sure your form works just right.
Integrating with UI Libraries
React Hook Form works really well with other UI libraries like Material UI and Ant Design, thanks to a special part called the Controller component. This means you can use these libraries to make your forms look great and still manage everything easily with React Hook Form.
Use with Material UI
For Material UI components like TextField and Checkbox, you can use the Controller component to make sure they work well with useForm.
import { Controller } from "react-hook-form";
import { TextField } from "@material-ui/core";
function App() {
const { control } = useForm();
return (
<Controller
name="firstName"
control={control}
defaultValue=""
render={({ field }) => <TextField {...field} />}
/>
);
}
Here are more examples:
Use with Ant Design
Ant Design components can also be used with the Controller in a similar way:
import { Controller } from "react-hook-form";
import { Input } from "antd";
function App() {
const { control } = useForm();
return (
<Controller
name="lastName"
control={control}
render={({ field }) => <Input {...field} />}
/>
);
}
More examples include:
The Controller part makes it easy to connect these UI libraries with React Hook Form, so everything works smoothly together.
sbb-itb-bfaad5b
Advanced Techniques
Watching Field Values
watch()
is a handy tool in useForm that lets you keep an eye on what's happening in your form fields. You can use it for things like:
- Instantly showing messages if something's not right
- Changing what's shown on the form based on what the user picks
- Adding up values from different fields
Here's a simple way to use watch()
to show a warning if a password isn't long enough:
const { register, watch } = useForm();
const password = watch("password");
return (
<>
<input {...register("password")} />
{password && password.length < 8 && (
<p>Password must be at least 8 characters</p>
)}
</>
);
You can also keep track of more than one field at a time:
const { watch } = useForm();
const [firstName, lastName] = watch(["firstName", "lastName"]);
Every time the fields you're watching change, watch()
will update.
Managing Form Arrays
For fields where you might have a bunch of values, like a list of emails, useForm lets you use arrays.
Start by setting up your field as an array:
useForm({
emails: []
});
Then, you can add or remove items with append
and remove
:
const { register, control } = useForm({
emails: []
});
<input {...register("emails.0")} />
<Controller
name="emails"
control={control}
render={({ field }) =>
<Button onClick={() => append(field.name)}>
Add Email
</Button>}
/>
To show all the emails, just loop through them like this:
{emails.map((email, index) => (
<div key={index}>
<input {...register(`emails.${index}`)} />
</div>
))}
This way, you can easily handle a list of items, adding, changing, or removing them as needed.
Real-World Examples
Sample Project Walkthrough
Let's dive into how React Hook Form is used in a real project. We'll look at the code from this GitHub repository for an eCommerce web app.
First, we import React Hook Form and set it up:
import { useForm } from "react-hook-form";
const {
register,
handleSubmit,
formState: { errors },
} = useForm();
Then, we create rules for checking the input fields:
<input
{...register("firstName", {
required: true,
minLength: 3
})}
/>
If there's a mistake, like the name being too short, we show a message:
{errors.firstName && (
<p>First name required and minimum 3 chars</p>
)}
When the form is sent, the handleSubmit
function gathers the data:
<form onSubmit={handleSubmit(onSubmit)}>
</form>
function onSubmit(data) {
console.log(data);
}
This example shows us how to manage forms in a React app using React Hook Form. It covers setting up the form, checking inputs, showing error messages, and collecting data when the form is submitted.
Community Showcase
The React community has made some cool projects with React Hook Form:
- A signup form that checks user information using Material UI
- A multi-step form wizard that breaks down long forms into smaller parts
- An application form with complex rules, made with Ant Design
These projects show that React Hook Form can handle everything from simple to complex forms. The examples from the community demonstrate the different ways you can use this tool to build forms in React.
Conclusion
React Hook Form makes managing forms in React apps a lot easier. It takes care of the usual tasks like checking input fields, keeping track of changes, and handling errors, so you can focus on making your app user-friendly.
Here are some main points to remember:
- You can connect input fields and deal with form submissions using
register
andhandleSubmit
- It lets you check fields for errors with ready-made rules and your own checks
- It works well with UI libraries like Material UI and Ant Design, making your forms look nice
- You can keep an eye on what users type and handle lists of inputs for more interactive forms
- It's possible to build all sorts of forms, from simple sign-in pages to detailed multi-step forms
React Hook Form can do a lot more than what we've talked about here. As you work on more projects, you might want to look into other features like:
- Checking inputs in more detail with asynchronous validation
- Understanding the difference between controlled and uncontrolled inputs
- Working with nested field values
- Using form context to share data across your app
- Making your forms run faster and smoother
React Hook Form is designed to meet almost any need you might have for forms. It aims to make form management less of a headache, allowing you to create the forms you envision, whether they're simple or complex, bare-bones or feature-rich. Try it out in your next React project and see how it goes!
Related Questions
Why do we use useForm () in React?
The useForm()
hook in React makes managing forms easier by giving us tools like register
and handleSubmit
. These tools help us link up inputs, check entries, keep track of what's being typed, and handle form submissions without writing a lot of code. Basically, it does the heavy lifting so we can focus on making forms that are easy for people to use.
Is React hook form worth using?
Yes, React Hook Form is a great tool for managing forms. It's light but powerful, making form tasks like state management, validation, and submissions simpler with a straightforward approach. Many developers appreciate how it makes their work easier and faster, plus it's flexible and doesn't slow down your app.
What are the downsides of Formik?
Formik is a bit bigger in size compared to some other options and might take a bit more time to learn at first. It also doesn't have some of the advanced validation features that you might find in libraries like React Hook Form. However, it's still a solid choice for many projects, depending on what you need.
What is the best form validation for React?
There isn't a single "best" form validation library for React. Popular ones like Formik, React Final Form, and React Hook Form each have their own strengths. Many people like React Hook Form because it's efficient and comes with a lot of features. But if you're already comfortable with Formik, you might prefer to stick with it. It's all about what works best for your project.