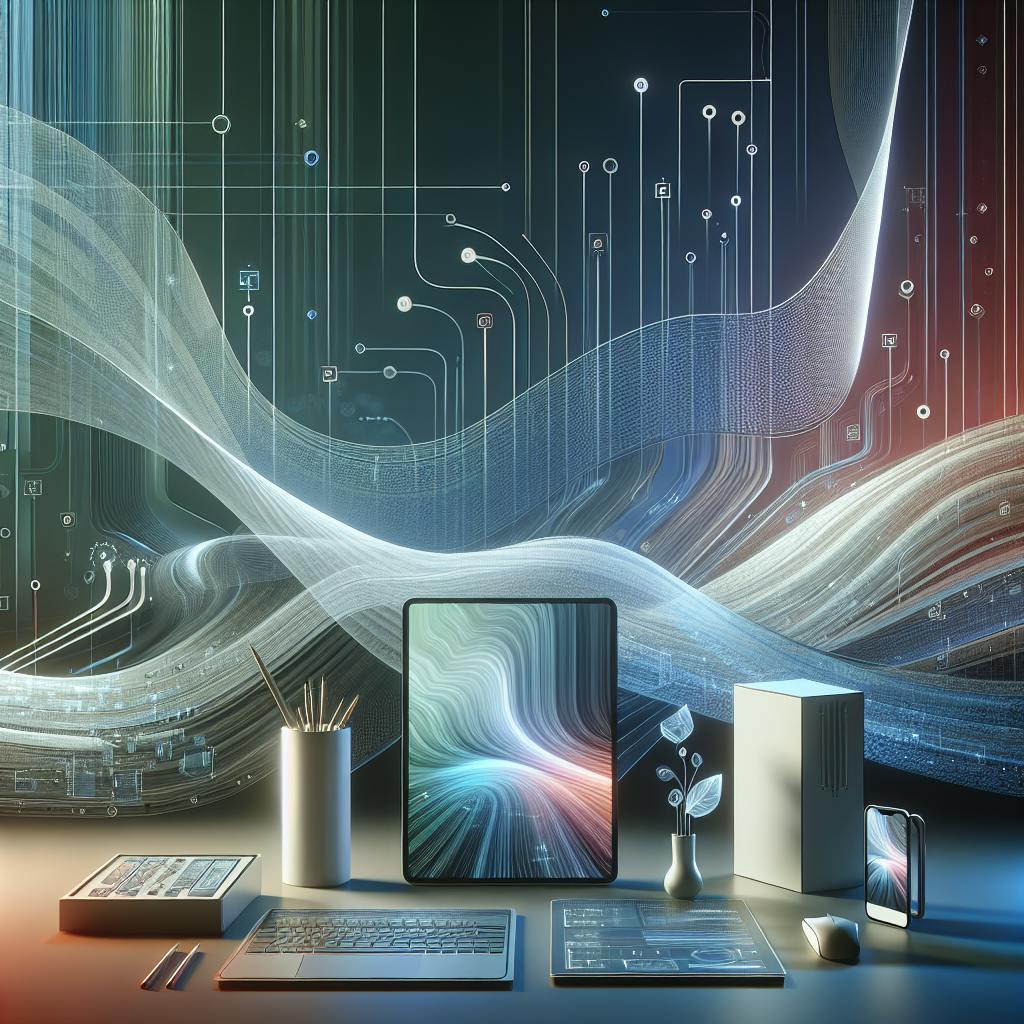
Discover how Svelte's compiler optimizes performance with build-time compilation, efficient reactivity, and smaller bundle sizes.
Svelte's compiler is what sets it apart. Here's the key stuff:
- Compiles components at build time, not runtime
- Creates lean JavaScript that updates the DOM directly
- No virtual DOM - better performance, less overhead
- Smaller bundles, faster-loading apps
The big benefits:
- Tiny bundle sizes (7kB vs 45kB for React starters)
- Faster initial loads
- Minimal runtime overhead
- Efficient reactivity with less code
The process:
- Parse Svelte code into an Abstract Syntax Tree (AST)
- Analyze AST to optimize
- Transform into efficient JavaScript
Tips for using Svelte's compiler:
- Use reactive declarations for dynamic values
- Keep components small and focused
- Leverage Svelte's built-in directives and animations
Quick comparison:
Feature | Svelte | React |
---|---|---|
Compilation | Build-time | Runtime |
Bundle Size | Smaller | Larger |
DOM Updates | Direct | Virtual DOM |
Performance | Faster | Slower |
Learning Curve | Easier | Steeper |
Svelte's build-time approach offers major performance gains, making it great for speed-critical projects.
Related video from YouTube
How Svelte Compiles Code
Let's break down Svelte's compilation process:
Parsing: Creating the AST
First, Svelte parses .svelte
files:
- Breaks down into HTML, CSS, and JavaScript
- Creates Abstract Syntax Trees (ASTs) for each part
- Handles Svelte-specific syntax
The result looks like:
{
html: { type, start, end, children },
css: { type, start, end, attributes, children, content },
instance: { type, start, end, context, content },
module: { type, start, end, context, content }
}
Analysis: Extracting Information
Next, Svelte analyzes the AST:
- Tracks variables
- Identifies dependencies
- Detects reactive statements and event handlers
- Determines variable scopes
This info goes into a Component
instance.
Transformation: Generating Output
Finally, Svelte transforms the analyzed code:
- Creates code for server-side and client-side rendering
- Generates a
create_fragment
function for efficient DOM updates - Produces template literals for server-side rendering
It also handles CSS:
- Generates unique class names to prevent conflicts
- Optimizes CSS for performance
The result? Lean JavaScript that updates the DOM directly, no virtual DOM needed.
Main Parts of the Svelte Compiler
The Svelte compiler has three key components:
Parser: Building the AST
The parser:
- Breaks down
.svelte
files - Handles Svelte-specific syntax
- Uses
acorn
for JavaScript andcss-tree
for CSS parsing
The AST looks like:
{
html: { type: 'Fragment', children: [...] },
css: { ... },
instance: { context: 'default', content: {...} },
module: { context: 'context', content: {...} },
}
Analyzer: Managing Dependencies
The analyzer:
- Tracks variables
- Identifies dependencies
- Detects reactive statements and event handlers
- Determines variable scopes
It creates a Component
instance with all this info.
Code Generator: Creating JavaScript
The code generator:
- Creates code for server-side and client-side rendering
- Generates a
create_fragment
function for DOM updates - Produces template literals for server-side rendering
- Optimizes CSS
The result? Efficient JavaScript that updates the DOM directly.
How Svelte Improves Code
Svelte makes your code better in several ways:
Making CSS Better
Svelte's compiler does clever things with CSS:
- Scopes styles to components
- Removes unused CSS
Here's how it scopes CSS:
<style>
p { color: blue; }
</style>
<p>This text will be blue</p>
Compiles to:
<style>
p.svelte-xyz123 { color: blue; }
</style>
<p class="svelte-xyz123">This text will be blue</p>
This keeps styles contained and CSS lean.
Efficient Reactivity
Svelte's reactivity system is built-in and efficient:
- Figures out dependencies at compile time
- Only updates parts of the DOM that change
Example:
<script>
let count = 0;
function increment() {
count += 1;
}
</script>
<button on:click={increment}>
Clicked {count} {count === 1 ? 'time' : 'times'}
</button>
Svelte compiles this to efficient JavaScript that updates only what changes.
To make the most of Svelte's reactivity:
- Use
$:
for reactive declarations - Keep components focused
- Use Svelte's built-in directives
Svelte vs. Other Frameworks
Svelte takes a unique approach compared to React and Vue:
Aspect | Svelte | React/Vue |
---|---|---|
Processing Time | Compile-time | Runtime |
DOM Updates | Direct | Virtual DOM |
Bundle Size | Smaller | Larger |
Performance | Faster | Slower |
Learning Curve | Easier | Steeper |
Svelte compiles at build time, offering:
- Smaller bundles
- Faster performance
- Better battery life on mobile
Rich Harris, Svelte's creator, says:
"Svelte is a compiler that knows at build time how things could change in your app, rather than waiting to do the work at run time."
Real-world examples show Svelte's benefits:
- QuickBudget: Faster load times, better UX
- Decathlon: Better performance on low-power devices
Trade-offs to consider:
- Smaller ecosystem than React or Vue
- Always needs a build step
Choose Svelte for:
- Performance-sensitive apps
- Static web apps
- Mobile-first projects
Consider React or Vue for:
- Large, complex apps
- Projects needing lots of third-party libraries
- Teams familiar with these frameworks
sbb-itb-bfaad5b
How to Check Compiled Output
To see how Svelte compiles your code:
-
Use the Svelte REPL:
- Go to the Svelte REPL website
- Write or paste your code
- Click "JS output"
-
Compile locally:
- Install Svelte:
npm install svelte
- Run:
npx svelte compile MyComponent.svelte > MyComponent.js
- Install Svelte:
- Use a build tool like Rollup:
import svelte from 'rollup-plugin-svelte';
import resolve from '@rollup/plugin-node-resolve';
import commonjs from '@rollup/plugin-commonjs';
import { terser } from 'rollup-plugin-terser';
export default {
input: 'src/main.js',
output: {
format: 'iife',
file: 'public/bundle.js'
},
plugins: [
svelte(),
resolve(),
commonjs(),
terser()
]
};
Run rollup -c
to compile.
When analyzing output, look for:
- How reactive statements are handled
- DOM creation and updates
- Event listener attachments
Example:
Svelte component:
<script>
export let name = 'world';
</script>
<h1>Hello {name}!</h1>
Compiled JavaScript (simplified):
export default function Component(options) {
let { name = 'world' } = options.props || {};
let h1;
let t1;
return {
c() {
h1 = element("h1");
t1 = text("Hello ");
t2 = text(name);
t3 = text("!");
},
m(target, anchor) {
insert(target, h1, anchor);
append(h1, t1);
append(h1, t2);
append(h1, t3);
},
p(changed, ctx) {
if (changed.name) set_data(t2, ctx.name);
},
d(detaching) {
if (detaching) detach(h1);
}
};
}
This shows how Svelte creates and updates DOM elements based on your component's state.
Common Compiler Settings
Key Svelte compiler options:
Option | Type | Default | Description |
---|---|---|---|
name |
string | 'Component' | JavaScript class name |
filename |
string | null | For debugging and sourcemaps |
generate |
string | 'dom' | Output type: 'dom', 'ssr', or false |
dev |
boolean | false | Adds extra checks and debug info |
css |
string | 'injected' | How styles are handled |
customElement |
boolean | false | Creates a custom element |
Set these in svelte.config.js
:
module.exports = {
compilerOptions: {
dev: true,
css: 'external'
}
};
Key points:
dev: true
adds checks but slows down your appcss
option changes style processingcustomElement: true
for web componentsgenerate
for SSR or client-side components
Rich Harris explains:
"The compiler settings give you fine-grained control over how Svelte generates code. This is crucial for optimizing performance and adapting to different use cases."
For browser-only code, use:
if (!building) {
// Browser-only code
}
Fixing Common Compiler Problems
When you hit compiler issues:
- Check the console for error messages
- Review your code for syntax mistakes
- Inspect the compiled output in dev tools
Common errors and fixes:
Error | Cause | Solution |
---|---|---|
ReferenceError | Undefined variable | Check spelling and declarations |
Syntax Error | Incorrect Svelte syntax | Review Svelte docs |
Type Error | Mismatched data types | Verify types and conversions |
Event Handling Error | Incorrect event binding | Check event listener syntax |
Transition Error | Animation code issues | Review transition directives |
Rich Harris advises:
"The key to efficient debugging in Svelte is understanding the relationship between your source code and the compiled output. Often, what looks like a runtime error is actually a compile-time issue."
For complex issues:
- Use the Svelte REPL to isolate problems
- Enable source maps in your build
- Try a linter like ESLint with a Svelte plugin
Remember, Svelte serves the last working version if it hits a syntax error. Clear your cache and rebuild after fixes.
Wrap-up
Svelte's compiler is a game-changer:
-
Build-time optimization:
- Smaller bundles
- Faster loads
- Less runtime overhead
-
No virtual DOM:
- Direct DOM updates
- Less memory use
- Faster rendering
-
Efficient reactivity:
- Automatic updates
- Less boilerplate
- Better performance
The numbers:
Metric | Svelte | React |
---|---|---|
Bundle size (starter) | 7kB | ~45kB |
Runtime overhead | Minimal | Higher |
Initial load time | Faster | Slower |
Rich Harris explains:
"By shifting the work to compile-time, we can deliver better performance without sacrificing developer experience."
Real-world impact: The New York Times used Svelte for real-time COVID-19 charts, benefiting from small bundles and efficient updates.
To maximize Svelte's compiler:
- Use reactive declarations
- Keep components small
- Use Svelte's built-in features
FAQs
What is Svelte's compilation process?
- Parsing: Creates an Abstract Syntax Tree (AST)
- Analysis: Identifies dependencies and references
- Transformation: Generates optimized JavaScript and CSS
This happens at build time, resulting in:
- Faster app loading
- Smaller bundle size
- Less browser work
Comparison:
Framework | Compilation | Bundle Size | Runtime Overhead |
---|---|---|---|
Svelte | Build-time | Smaller | Minimal |
React | Runtime | Larger | Higher |
Rich Harris notes:
"By shifting the work to compile-time, we can deliver better performance without sacrificing developer experience."
This approach lets Svelte create efficient code tailored to your app, optimizing for performance.