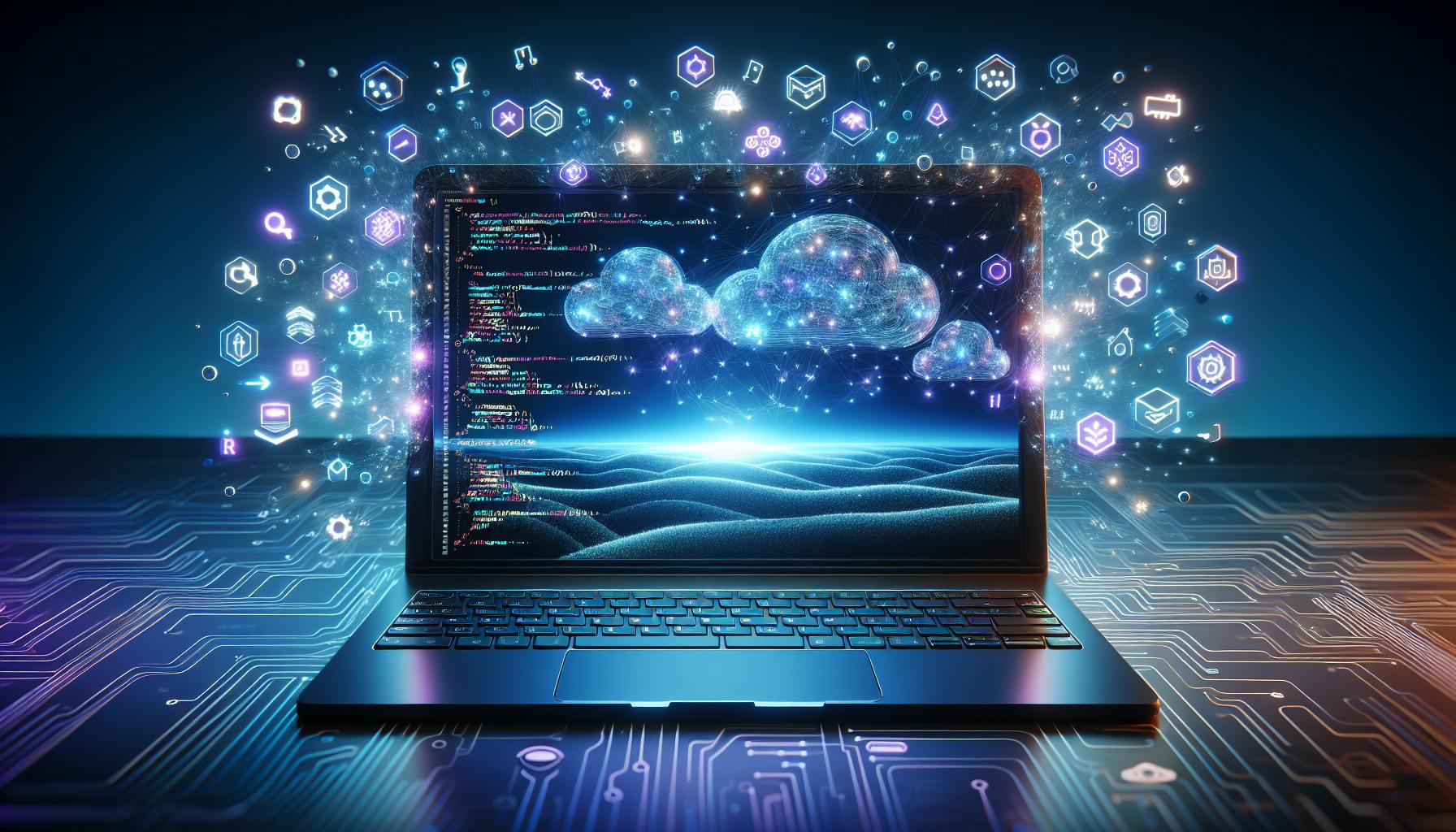
Learn about the importance of test cases in React JS projects, types of tests, setting up a testing environment, writing test cases, testing component interactions, handling asynchronous operations, advanced testing techniques, best practices, and common pitfalls in testing React apps.
When diving into React JS, testing your components is crucial for ensuring they work as intended. This guide simplifies the testing process, providing you with the essentials to get started:
- Understanding Test Cases: These are checks to ensure your app behaves correctly under various conditions.
- Importance of Testing: Testing aids in catching bugs early, making changes with confidence, and speeding up development.
- Types of Tests: Learn about unit, integration, and end-to-end tests.
- Setting Up Your Testing Environment: Steps to install testing libraries and configure your test scripts.
- Writing Your First Test Case: A practical example using a
<Button>
component. - Testing Component Interactions: How to simulate user actions like clicks and form submissions.
- Testing Asynchronous Operations: Techniques for handling operations that involve waiting for data.
- Advanced Testing Techniques: Insights into testing custom hooks, context, and using snapshot testing.
- Best Practices and Common Pitfalls: Tips to enhance your testing strategy and avoid typical mistakes.
Whether you're new to React or looking to improve your testing skills, this guide offers a practical approach to creating test cases for your React JS projects.
What are Test Cases?
Test cases are like a checklist for making sure your app does what it's supposed to do. You'll have a list of things to check off, like:
- Making sure a part of your app shows up right when you change something
- Checking if forms work when you fill them out
- Seeing if error messages pop up when they should
- Making sure you can move around in the app without problems
- Testing if parts of your app that need to wait for something (like info from the internet) work as expected
By having these checks, you can be sure that every part of your app works fine, both the simple stuff and the tricky bits.
Why are Test Cases Important?
Here's why having these checks is a big deal for making apps with React:
- Stops bugs: These tests find problems early, so they don't bother your users. This includes things like parts of the app not showing up right, problems talking to the internet, or the app not keeping track of things correctly.
- Makes changes easier: When you have tests, you can change stuff without worrying about breaking things. This means you can add new features or tidy up the code with less stress.
- Shows how things should work: Good tests explain how parts of your app should work. They're like a guide for other people who work on your app.
- Speeds up making the app: Testing on your own computer means you find and fix problems faster than if you wait for someone else to test it. This makes the whole process of making the app quicker.
Having lots of tests is key for making sure your React app is solid and works well.
Types of Tests
When you're testing, there are a few main types you'll hear about:
Unit Tests
Unit tests focus on one small part of your app at a time. They make sure that each little bit (like a button or a message) works right on its own. Tools like Jest and React Testing Library are great for this.
Integration Tests
Integration tests look at how different parts of your app work together. They're about making sure that when you put several parts together, they still work right. This is good for checking more complex stuff.
End-to-End Tests
End-to-end tests are like pretending you're a user and walking through everything they would do. It's about making sure the whole app works from start to finish, just like it should for a real user. Tools like Cypress and Selenium are used for these tests.
Choosing the right type of test depends on what part of the app you're looking at and how big of a picture you want to get. It's a good idea to use a mix of these tests to make sure everything in your app is working great.
Setting Up Your Testing Environment
Getting your React project ready for testing means you need to get some tools and set things up so you can write and run tests. Here's how to do it step by step:
Install Testing Libraries
For testing React components, these are the go-to libraries:
- Jest - This tool finds and runs your test files. It's often paired with:
- React Testing Library - Helps you test React components in a way that's closer to how users interact with them.
If you used create-react-app
to start your project, you're all set. If not, you can add them like this:
npm install --save-dev jest @testing-library/react
Create Test Files
Test files end with .test.js
or .spec.js
. People usually keep them in a __tests__
folder.
Name your test files after the component they're testing, like Button.test.js
for testing Button.js
.
Configure Test Script
To make running tests easy, add a test command in your package.json
file:
"scripts": {
"test": "jest"
}
Now, typing npm test
will run all your tests.
Import Dependencies
In your test files, start by bringing in what you need:
import React from 'react';
import { render } from '@testing-library/react';
And don't forget the component you're testing:
import Button from './Button';
Write Test Cases
To test something, you use it()
blocks. Here's an example:
it('matches snapshot', () => {
const {asFragment} = render(<Button>Click</Button>)
expect(asFragment()).toMatchSnapshot();
});
This test checks if the button looks like it's supposed to.
Run Tests
When you run npm test
, it'll check all your tests and tell you how they did.
With these steps, you're ready to start testing your React components with Jest and React Testing Library!
Writing Your First Test Case
Let's start with a simple test case to make sure a component shows up right. We'll use a <Button>
component as an example.
Set Up the Test File
First off, make a Button.test.js
file and bring in what we need:
import React from 'react';
import { render } from '@testing-library/react';
import Button from './Button';
Render the Component
Next, we use the render
method from React Testing Library to show the component:
const { getByText } = render(<Button>Click Me</Button>);
This makes the button appear in a virtual space we can check.
Write the Test Case
Now let's write our test case with Jest's it()
method:
it('shows the button with the right text', () => {
getByText('Click Me');
});
The getByText()
method looks for the "Click Me" text in the component. If it can't find that text, our test won't pass.
Expand the Test
To make our test better, we could also check things like if the button has the right look:
it('shows the button with the right text and look', () => {
const button = getByText('Click Me');
expect(button).toHaveClass('btn');
expect(button).toHaveStyle('background: blue');
});
Here we grab the button and use Jest matchers to check its class and style.
Run the Test
Lastly, we run npm test
and Jest will run the test case on the component. We can see if what we expected is true or not.
This is the basic way to check if a component shows up right! From here, we can test more things like how it reacts to clicks, gets data, and more.
Testing Component Interactions
Interacting with websites is something we all do. You click on things, type in your details, and expect everything to work smoothly. Testing these actions in your React apps is crucial to ensure they respond correctly.
Here's a simple guide to testing user actions like clicks, typing, and form submissions in React:
Simulating User Events
React Testing Library lets us pretend to do things like clicking or typing in a component. Here are some basic actions you might want to check:
Clicks
// Pretend to click a buttonireEvent.click(getByText('Submit'));
Text Inputs
// Pretend to type into a text box
fireEvent.change(getByPlaceholderText('Name'), {
target: {value: 'Mary'}
});
Form Submission
// Pretend to submit a form
fireEvent.submit(getByTestId('checkout-form'));
You can find more ways to simulate actions in the docs.
Mocking Event Handlers
When you simulate an action, it might trigger a function. We can create a fake version of this function (mock it) to see if it works as expected:
// Create a fake handleClick function
jest.fn(handleClick = jest.fn());
// Check if handleClick was called after a click
fireEvent.click(getByText(/Click/i));
expect(handleClick).toHaveBeenCalledTimes(1);
Asserting Interaction Results
We also want to make sure that when someone interacts with a component, the right things happen:
// Check if clicking changes what's shown
fireEvent.click(getByText('Show More'));
expect(getByTestId('extra-content')).toBeInTheDocument();
Testing Conditional Rendering
If your component shows different things under different conditions, you can test that too:
// Check what's shown before and after a click
expect(getByLabelText(/off/i)).toBeInTheDocument();
userEvent.click(getByLabelText(/toggle/i));
expect(getByLabelText(/on/i)).toBeInTheDocument();
Common Examples
Here are some simple tests you might write:
- Clicking a button hides or shows more info
- Sending a form shows a 'thank you' note
- Typing in a box updates something else on the page
- Clicking an icon changes how it looks or where it is
- Moving items around changes their order
Testing these interactions helps make sure your app does what your users expect. It's all about making sure everything works well and feels right when people use your app.
Testing Asynchronous Operations
In React apps, you often have to deal with operations that wait for something to happen, like getting data from the internet. Testing these parts is crucial to make sure they work right. Here's a simple way to test these wait-and-see functions in React components:
Mocking API Calls
Instead of actually calling the internet during tests, we pretend by using a fake fetch
function that immediately gives us some made-up data:
jest.spyOn(window, 'fetch').mockImplementation(() =>
Promise.resolve({
json: () => Promise.resolve({
data: mockData
})
})
);
This way, fetch
will give us our fake data right away, without reaching out to the internet.
Using Async/Await
Since fetching data can take time, we use async/await to write our test as if it was happening step by step:
test('displays fetched data', async () => {
// Show component
const { findByText } = render(<MyComponent />);
// Wait for the fake fetch to finish
await findByText(mockData.message);
});
The findByText
function waits until it can find the text we're looking for.
Asserting Async Behavior
We check to make sure the component:
- Shows a 'loading' message
- Displays the data once it's fetched
- Shows an error if something goes wrong
test('handles fetch correctly', async () => {
// Check for 'loading'
expect(screen.getByText(/loading/i)).toBeInTheDocument();
// Wait for data and check it shows up
await screen.findByText(mockData.message);
// Simulate an error and check for an error message
fetch.mockReject(() => {
expect(screen.getByText(/error/i)).toBeInTheDocument();
});
});
Mocking Unresolved Promises
Sometimes, we might want to see what happens if the data never comes. We can do this by pretending fetch
doesn't finish:
fetch.mockImplementation(() => new Promise(() => {}));
Then we check to make sure the component keeps showing the 'loading' message.
By testing these wait-and-see operations, we make sure our React app can correctly show data from the internet. Using a fake fetch lets us control the test without real internet calls.
sbb-itb-bfaad5b
Advanced Testing Techniques
When your React app starts to get bigger, testing it can become more complicated. Here are some smarter ways to deal with those tougher spots.
Testing Custom Hooks
Custom hooks let you reuse code across your React app. To test them:
- Pretend any outside parts they talk to (like context or APIs) are something you control
- Try giving them different inputs to see if they react the way you expect
- See if they cause the screen to update when they should
For instance:
const mockDispatch = jest.fn();
test('useData hook requests data', () => {
const {result} = renderHook(() =>
useData(mockDispatch)
);
expect(mockDispatch).toHaveBeenCalledWith({type: 'FETCH_DATA'});
expect(result.current).toEqual({data: null, loading: true});
});
Here, we're pretending the reducer dispatch function and checking how the hook behaves.
Testing Context
Components that use React context need the right setup to work.
To check these components:
- Surround them with fake providers
- Change the providers to test how components react in different situations
Like this:
const UserContext = React.createContext();
const mockUser = {name: 'Mary'};
test('Profile shows user name from context', () => {
render(
<UserContext.Provider value={mockUser}>
<Profile />
</UserContext.Provider>
);
expect(screen.getByText(mockUser.name)).toBeInTheDocument();
});
We're making sure the component can get the name from context.
Snapshot Testing
Snapshot tests take a picture of your component and later check if anything has changed. They're good for catching unexpected changes after updates.
But remember:
- They're not great if your component changes a lot
- They can get messy with big components
- They don't test how things work, just how they look
Snapshots are best for parts of your app that don't change much, like:
- Common elements that look the same everywhere
- Components that display data from outside
- Basic utility components
Mix snapshot tests with other kinds for a complete overview.
Testing Error Handling
Making sure your app can show errors correctly is key.
Here are some things to check:
- A backup plan if a part of your app breaks
- The right error messages for different problems
- Special components that handle errors
For example:
jest.spyOn(console, 'error');
test('shows error message on failure', () => {
render(<BuggyComponent />);
expect(console.error).toHaveBeenCalled();
expect(screen.getByRole('alert')).toHaveTextContent(/went wrong/i);
});
Here, we're checking if the error message shows up when there's a problem.
Getting ready for these complex situations helps us test our React apps more deeply as they grow. Using a mix of different test types is the best way to be sure everything works as it should.
Best Practices
When you're writing tests for your React apps, it's smart to follow some rules to make sure your tests are really helping. Here's what to keep in mind:
Write Tests Early On
- Start testing as soon as you begin making something new. This stops problems from building up.
- If you need to, change your code so it's easier to test. You might need to make some parts simpler or use fake versions of things your code talks to.
Test One Thing Per Test
- Make sure each test looks at just one thing. If a test fails, you'll know exactly what went wrong.
- Don't mix different checks in one test. It makes it harder to figure out what the issue is.
Use Descriptive Names
- Name your tests in a way that tells you what they do. For example, 'shows error message if form is empty'.
- Mention the specific parts, actions, and results you're testing.
Isolate Components
- Use fake versions of other parts of your app, like data fetching, to test each piece by itself.
- This keeps your tests focused and fast.
Test Key User Journeys
- Focus on testing the most important parts of your app that users will interact with.
- If you're short on time, test the big stuff first.
Use Realistic Data
- Test with data that looks like what users would actually enter, both good and bad.
- This makes sure your tests cover what happens in real life.
Avoid Implementation Details
- Test what your app does from the outside, like what users see and do, not how it's built inside.
- If you change how something works but it still does the job, your tests should still pass.
Follow Testing Pyramid
- Have lots of small tests for individual parts and fewer big tests for how things work together.
- Big tests can be tricky and slow, so use them wisely.
By sticking to these tips, your tests will be more useful, easier to manage, and really help make sure your React app works well.
Common Pitfalls
When you're writing tests for your React apps, it's easy to slip up in a few common ways. Knowing what these mistakes are and how to dodge them can make your tests stronger and easier to work with.
Testing Implementation Details
Sometimes, we get too caught up in how a component is put together rather than focusing on what it shows and how it acts for users. Like checking if certain functions are called, instead of looking at what gets displayed.
// Not great
test('calls componentDidMount', () => {
const spy = jest.spyOn(MyComponent.prototype, 'componentDidMount');
render(<MyComponent />);
expect(spy).toHaveBeenCalledTimes(1);
});
// Better
test('shows welcome message', () => {
const { getByText } = render(<MyComponent />);
getByText('Welcome!');
});
How to fix it: Keep your tests centered on what the component does and shows, not on the inner workings.
Overmocking Dependencies
Mocking everything can make your tests break easily. If you mock too much, you'll have to redo tests a lot when you change how things work.
How to fix it: Only mock the parts that make testing hard or unreliable.
Testing Multiple Things
Trying to check a bunch of different things in one test can make it tough to figure out why it failed.
How to fix it: Stick to testing one thing at a time.
Ignoring Error Cases
If you don't check how components act when things go wrong, you're missing out on important checks.
How to fix it: Test with bad inputs or simulate errors to see how your component handles them.
Interdependent Tests
When tests rely on other tests to pass, it can lead to shaky and unreliable results.
How to fix it: Make sure to reset things before and after each test so they don't depend on each other.
Too Many Snapshots
Using too many snapshot tests can be a problem because they break easily and don't really test how things work.
How to fix it: Use snapshots carefully, mainly for things that don't change much. Test actions and interactions in other ways.
By sticking to these tips, you can avoid common testing traps and build tests that are quicker, easier to manage, and more effective at finding issues early on. Focus on how the app works from a user's view, test one thing at a time, and get ready for things to go wrong sometimes.
Conclusion
Making sure your React parts work well is super important for creating apps that people enjoy using. By checking that parts show up right, respond to clicks and types correctly, and handle data and errors smoothly, you can be sure your app will do what it's supposed to do.
If you're new to React, testing might seem a bit daunting at first. But starting with easy checks and slowly adding more tests can help you get the hang of it. Tools like React Testing Library and Jest make writing tests easier by doing a lot of the work for you.
As your app gets bigger and more complicated, using tricks like pretending for parts your app talks to, checking custom hooks, and planning for when things don't go as expected can keep your tests helpful and reliable. Even though you might run into issues like pretending too much or focusing too much on the small details, keeping these common mistakes in mind can guide you towards better testing habits.
By starting to test early and keeping up with testing the most important parts of your app, you can make your app stronger and ready for future changes and improvements. Testing lets you make changes and add new things without worrying, because you know your app will still work right.
Additional Resources
If you're looking to dive deeper into testing your React apps, here are some helpful links to check out:
- React Testing Library Docs - This is a go-to resource for learning how to use React Testing Library.
- Jest Docs - Here, you'll find everything you need to know about using Jest for testing.
- Testing Implementation Details - A useful article on why it's important not to get too caught up in the tiny details when testing.
- Common Testing Mistakes - This post talks about mistakes people often make when testing and how to avoid them.
- How to test custom React hooks - A guide on how to test the custom hooks you create in React.
- Simplifying Test Setup - Some tips from the React documentation on making your test setup easier.
- Testing Pyramid - An explanation of different types of tests and how they fit into what's called a 'testing pyramid'.
These resources offer more examples and advice on testing React components and apps using tools like Jest and React Testing Library. They cover topics such as avoiding common testing errors, making your test setup simpler, and how to test your custom hooks.