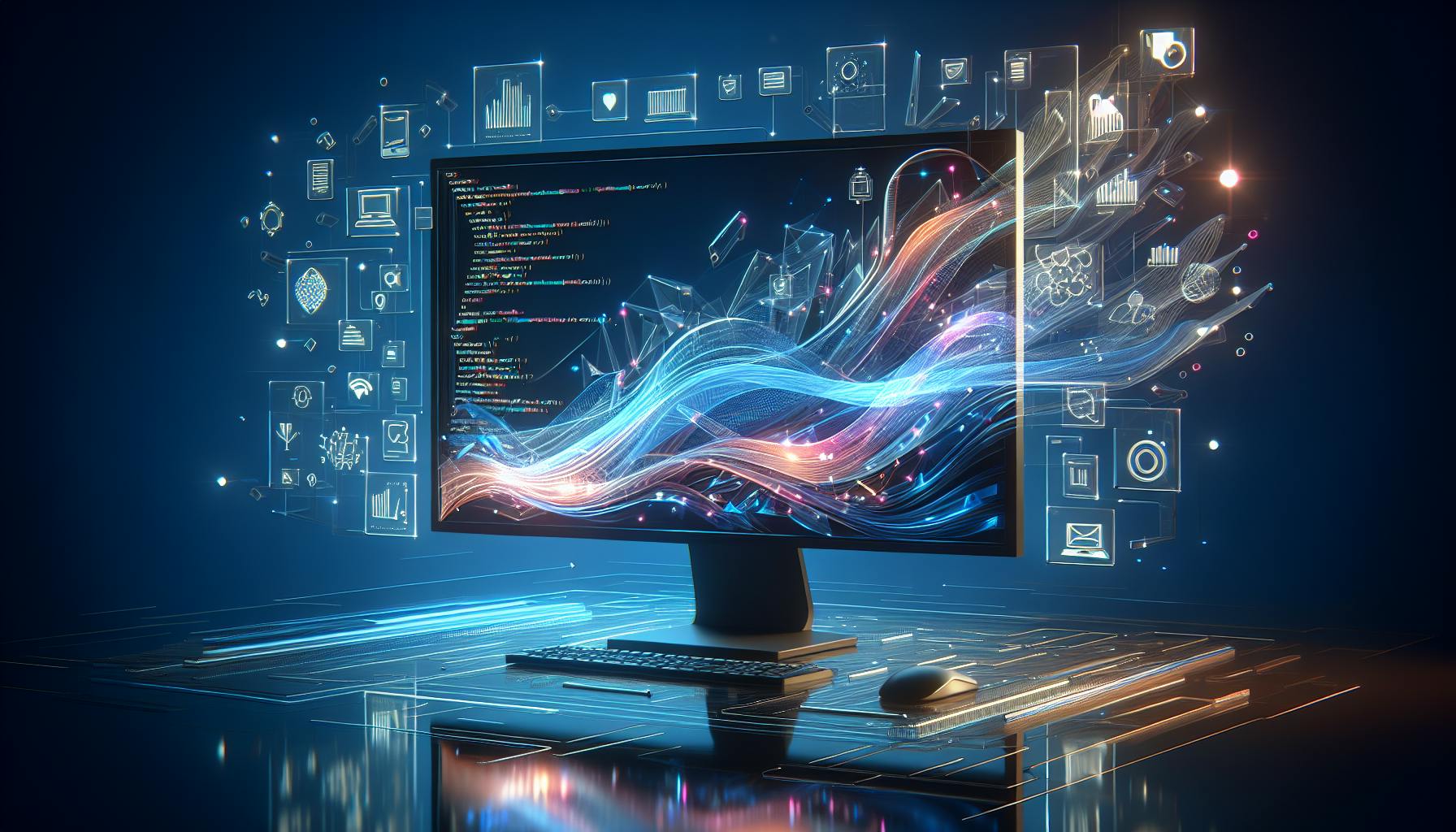
Learn best practices for testing code online, explore popular online IDEs, and discover insights into debugging, unit testing, and advanced techniques to improve your coding skills.
Testing your code online offers a versatile and accessible way to ensure your projects work correctly without the need for complex setups. This guide presents best practices for online code testing, explores popular online IDEs, and provides insights into debugging, unit testing, and advanced testing techniques. Here's a quick overview:
- Why Test Code Online? Easy access, collaboration, cost savings, and the ability to experiment with new technologies.
- Types of Testing: Unit Testing, Integration Testing, Functional Testing.
- Popular Online IDEs: Repl.it, CodeSandbox, IDEOne, and Codeanywhere.
- Best Practices: Follow coding standards, implement version control, prioritize testing and reviews, optimize performance, and secure sensitive data.
- Debugging and Troubleshooting: Use built-in tools, check errors and logs, validate assumptions, leverage collaboration, and take breaks.
- Advanced Techniques: Behavioral Testing, Test Automation & Management, Data Privacy Compliance.
Whether you're a beginner or an experienced coder, these insights will help you test your code more effectively online.
Unit Testing
Unit testing is all about making sure each small part of your code works right on its own. Here's how to do it well when testing online:
- Split your code into small pieces and test them one by one.
- Use tools like Chai or Jest to check if things are working as they should.
- Make sure these tests run fast online.
- Pretend external parts your code talks to (like databases) are there to focus on the piece you're testing.
Integration Testing
This type of testing checks if different parts of your code work well together when they're combined.
Some tips:
- Make your code in pieces that are easy to test together.
- Plan the order of tests to find problems easily.
- Make sure the parts of your code that talk to each other are doing it correctly.
- Look out for problems that could get worse when everything is put together.
Functional Testing
Functional testing makes sure the app does what it's supposed to do, like how a user would see it.
Here's how to do it right online:
- Use tools like Selenium or Cypress for testing how things look in a browser.
- Create tests for the most important things a user would do.
- Check if your app is easy for everyone to use.
- Compare what you expect your app to do vs. what it actually does.
- Test both when things go right and when they don't.
Testing code online makes working together easier and lets you access your work from anywhere. By knowing about different testing types and following these best practices for unit, integration, and functional testing, you can make sure your code is ready to go live.
Choosing the Right Online IDE
Picking the right online space to test your code, known as an IDE (Integrated Development Environment), can really make a difference. Here are some easy-to-understand details about popular options:
Repl.it
Repl.it lets you work with lots of programming languages and makes it easy to work on code with friends or teammates because of its collaboration features.
Key features:
- Works with over 50 languages like JavaScript, Python, and C++
- Lets multiple people code together in real-time
- Has both free and paid versions
- Connects with other tools like GitHub
Repl.it is a good pick for testing different kinds of code online, especially with others.
CodeSandbox
CodeSandbox is great for web projects, especially if you're using JavaScript or TypeScript. It's really good at finding and fixing bugs.
Why choose CodeSandbox:
- Best for JavaScript, TypeScript, and web stuff like React
- Easy to use with web libraries
- Great for fixing bugs with tools like Chrome DevTools
- You can share your work and code with others
- Free, but you can pay for more features
For web development, CodeSandbox is a top choice because of its focus and tools.
IDEOne
IDEOne works with a lot of programming languages and offers some simple ways to find and fix mistakes. It's very straightforward and free.
Key traits of IDEOne:
- Supports common languages like Java, Python, and JavaScript
- Some basic tools to fix mistakes
- Easy to use without any fluff
- No need to sign up
- Completely free
If you need a simple place to test code in popular languages, IDEOne is a solid choice.
Codeanywhere
Codeanywhere is designed for coders and teams, especially in schools. It's good for working together on coding projects.
What Codeanywhere offers:
- Supports many languages in a cloud setup
- Good for learning and teaching coding
- Tools for teams to work together
- You can tweak your coding space
- Free and paid options
For teaching and learning coding together, Codeanywhere is a great option.
Comparison
IDE | Languages | Debugging | Collaboration | Pricing |
---|---|---|---|---|
Repl.it | Many | Decent | Yes | Free tier |
CodeSandbox | JS/TS focus | Excellent | Yes | Freemium |
IDEOne | Most common | Basic | No | Free |
Codeanywhere | Many | Good | Yes | Free & paid tiers |
When picking an online IDE, think about what programming languages you need, if you want tools to find and fix bugs, if you want to work with others, and how much you're willing to spend. These options give you a good range to choose from so you can test your code effectively online.
Best Practices for Testing Code Online
When you test your code online, it's super handy because you can work from anywhere and with anyone. But, you also have to be careful about making sure your code is good and safe. Here are some smart moves to make sure you're doing it right.
Follow Established Coding Standards
It's a good idea to write your code the way most people agree it should be written. This makes it easier for others to read and work with your code. For example, Python has a guide called PEP8, and Java has Google's style guide. Tools like ESLint for JavaScript and Pylint for Python can help check your code automatically.
Implement Version Control
Using tools like Git and GitHub helps you keep track of changes, work on different parts at the same time, and share your work safely. It's like keeping a detailed history book of your code that can help fix things if something goes wrong.
Prioritize Code Testing and Reviews
Testing your code with tools (like Jest for JavaScript) and having others look over it can catch mistakes early. This way, you make sure your code does what it's supposed to do and is easy for others to understand and use.
Optimize Performance
Make your code run faster by doing things like compressing data, remembering results of slow operations, writing efficient code, and checking for memory leaks. This is important so that your code works well even when your team is spread out and using different internet connections.
Secure Sensitive Data
When you're working with private information, make sure it's protected. Encrypt data, check inputs from users carefully, and use tools to find security risks. This keeps your code safe from hackers when you're testing it online.
By keeping these practices in mind, you can make sure your online code testing is top-notch, safe, and works well for everyone involved.
Debugging and Troubleshooting
When you run your code online and hit a snag, figuring out what went wrong and how to fix it is crucial. Here are some simple steps to help you tackle bugs and keep your coding on track.
Use Built-In Debugging Tools
Most places where you can test your code online have tools to help you find bugs, like stopping the code at certain points or going through it step by step. Here’s how they help:
- Stop the code at specific spots to see what’s happening with your data
- Go through your code one line at a time to find where things go wrong
- Keep an eye on data changes to catch issues
Learning to use these tools can save you a lot of guessing and checking.
Check Errors and Stack Traces
When your code doesn’t work, error messages and stack traces can give you hints. They can tell you:
- Where in the code the problem is
- What the code was doing when it stopped working
- What data was being passed around
These clues can lead you back to the bug. If the error messages are confusing, searching them online can help.
Log Key Data
Writing down important information, like what your code is doing or what data it’s working with, can show you where things aren’t going as planned. This can point out:
- If your code is taking a wrong turn
- If the data isn’t what you expected
- Problems when your code talks to other services
Logging is easy and really useful for tricky problems.
Validate Assumptions
If you’re stuck, double-check what you think is true:
- Are the services your code uses working right?
- Are you using APIs correctly?
- Do you have everything you need?
- Is everything set up correctly?
Proving or disproving your guesses can shine a light on the issue.
Leverage Collaboration Tools
Working on bugs with others can be super helpful. Online testing places let you:
- Share your code in real time
- Code together
- Share your screen
- Talk or leave comments
A fresh set of eyes can help you see what you might have missed and get past roadblocks faster.
Take Regular Breaks
If you’re feeling stuck and frustrated, stepping away for a bit can help clear your head. A short break can help you see things differently and check if what you thought was true actually is.
Using these simple steps, the right tools, and asking for help when you need it can make fixing bugs much easier. Remember, it’s okay to ask for help. Working with someone else can help fill in the blanks and get you back on track faster. With practice, you’ll get better at squashing bugs in no time.
sbb-itb-bfaad5b
Unit Testing Best Practices
Naming and Structure
When you're doing unit testing, which is checking small bits of your code to see if they work right, it's key to name and organize your tests clearly. This makes them easy to follow and keep up with.
Test Names
- Give your tests names that tell you what part of the code they're checking and what should happen, like
getUser_ValidUser_ReturnsUserObject
- Keep names straightforward and to the point
Structure (AAA Pattern)
The AAA pattern stands for Arrange, Act, Assert and helps keep things tidy:
- Arrange - Get your test data, inputs, and anything else you need ready
- Act - Run the part of the code you're testing
- Assert - Check to make sure the outcome is what you expected
Using this clear setup makes your tests easier to get.
Avoid Test Pitfalls
There are some common mistakes to steer clear of when testing.
Keep Tests Simple
- Focus on testing one thing at a time
- Use just a few checks (assertions) in each test
- Skip complicated setup steps
Make Tests Focused and Independent
- Use mock-ups for things like databases so your tests don't rely on them
- Make sure tests don't depend on each other
Prevent Brittleness
- Don't make tests too specific on things that don't matter much
- Write tests so they're not affected by small changes in code
Sticking to these best practices helps your tests stay clear, quick, and more robust. This means when you change your code, your tests can still do their job without falling apart.
Advanced Testing Techniques
Behavioral Testing
Behavioral testing is all about seeing how an app works from a user's point of view. It doesn't dive into the technical bits but checks if the app does what it should do. Here's how to do it well:
- Set up tests that act like real users, such as clicking on things and filling out forms. Tools like Selenium are great for this.
- Make sure the whole process, from start to end, works smoothly.
- Test how the app handles both correct and incorrect data.
- Look into unusual situations to see if the app can handle them.
- Keep an eye on how much computer power the app uses to spot any slowdowns.
- Watch for any unexpected changes in the app to make sure it stays stable.
The main idea is to test what the app does without worrying about the technical details behind it.
Test Automation & Management
For big apps, it's key to set up tests that run by themselves and keep them organized:
- Use tools like JUnit, TestNG, or xUnit to write your tests once and then run them whenever you need.
- Run multiple tests at the same time to save time. Tools that help with continuous integration and delivery are useful here.
- Use reports to keep track of what tests are doing over time.
- Stick to a clear plan when writing automated tests to make them easier to handle.
- Make testing a regular part of building your app, like with test-driven development.
- Use version control for your testing stuff so everyone can work together easily.
The goal is to make sure your automated tests are doing their job well and are easy for everyone to work with.
Data Privacy Compliance
When you're testing with real user data, it's important to follow privacy laws like GDPR:
- Hide personal details in the data to keep it safe.
- Sort data by how sensitive it is and treat it carefully.
- Make sure only the right people can see the test data.
- Get rid of test data safely when you're done with it.
- If users say they don't want their data used, make sure the app respects that.
- Keep records to show you're handling data the right way.
It's all about testing in a way that protects people's privacy.
Conclusion
Testing code online helps developers work together better and make their software better, too. By sticking to smart ways of testing, picking the right tools, writing code the right way, and fixing problems smartly, teams can make their work go smoother.
Here's a quick summary of what we've talked about:
- Use different tests like checking small bits (unit testing), seeing if parts work together (integration testing), and making sure the whole app does what it's supposed to (functional testing). Organize your tests well with clear steps.
- Pick an online place to code that fits what you need. Think about if you want to work with others easily, how good the bug-finding tools are, how much it costs, and other things. Some good choices are Repl.it, CodeSandbox, IDEOne, and Codeanywhere.
- Write your code the right way by following rules, using version control, checking each other's work, making your code fast, and keeping private stuff safe. This helps make sure your code is good and doesn't have problems.
- Find and fix bugs using the tools your coding site gives you. Use error messages, write down important info, work with others, and sometimes take a break to figure out tough problems.
- Make testing automatic with tools like Selenium and JUnit to help you check your code all the time. Keep your tests in order and be careful with people's private information.
Testing code online makes it easier and faster for teams to make good software together. By following the tips we've shared, teams can make, improve, and finish their software quickly and safely.
Related Questions
How to test a code online?
To test your code online, you can use these tools:
- JSBin - Great for quick JavaScript tests
- JSFiddle - Good for trying out website code
- CodePen - Useful for front-end coding experiments
- CodeSandbox - Best for larger JavaScript or TypeScript projects
These tools let you write, run, and check your code right from your browser. They're perfect for playing around with code, working together with others, and showing off what you've made.
How can I be a good code tester?
To be good at testing code, try these tips:
- Make sure your tests always give the same result for the same code.
- Focus on one specific thing in each test.
- Check for unusual cases and limits.
- Set up your tests to run automatically when the code changes.
- Look at which parts of your code aren't being tested and test them.
- When a test fails, explain why clearly.
- Work with the people who write the code to make it easier to test.
Being thorough and automating your tests helps you catch problems early.
How do you test quality code?
To check if code is high quality, look at:
- Reliability - Does it work without crashing or making mistakes?
- Complexity - Is it simple enough to understand and change?
- Portability - Can you use it in different projects or on different devices?
- Reusability - How much of the code can you use again in other places?
- Test coverage - How much of your code is checked by tests?
- Tool analysis - What do tools that check your code for problems say?
Using tools that automatically check your code, along with checking it yourself, gives you a good idea of its quality.
What is a code test?
A code test checks that your code does what it's supposed to by:
- Looking at small parts of the code on their own
- Giving these parts specific things to work with
- Seeing if what comes out is what you expected
- Telling you what went wrong if it doesn't work
Good code tests make sure your code is reliable and deals well with different situations. Setting these tests up to run by themselves helps keep your code in good shape as you keep working on it.