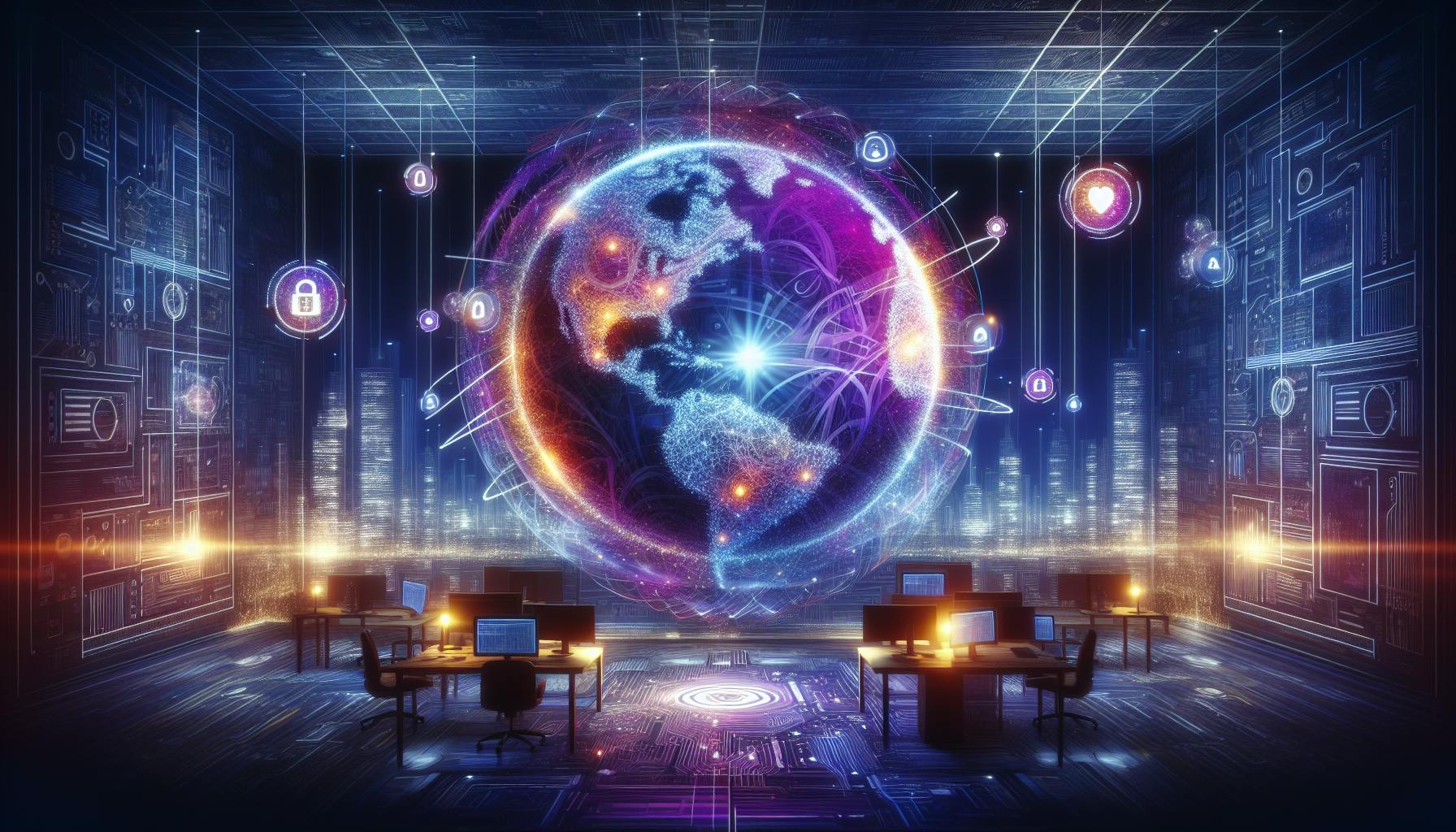
Learn how to set up and use the HTTPS Everywhere Chrome extension to enhance online security. Develop a similar extension with key features, testing tips, and publishing guidelines.
The HTTPS Everywhere Chrome Extension plays a crucial role in enhancing online security by automating the switch from HTTP to HTTPS, ensuring a secure browsing experience. This guide provides a comprehensive overview of how to set up and use the extension, along with insights into its importance for web security. Here's what you'll learn:
- What HTTPS Everywhere is: A tool developed by the EFF to secure your web connections.
- The role of HTTPS: It encrypts your data, authenticates sites, and ensures data integrity.
- How to develop a similar Chrome extension: Including setting up your environment, coding with HTML, CSS, and JavaScript, and understanding Chrome's extension architecture.
- Key features for your extension: Like using manifest.json, service workers, content scripts, and the DeclarativeNetRequest API for enhanced security.
- Testing and debugging tips: Strategies to ensure your extension works smoothly and securely.
- Publishing on the Chrome Web Store: A step-by-step guide to making your extension available to users.
Also, it addresses common questions such as the discontinuation of HTTPS Everywhere, installing the extension, and enabling developer mode in Chrome. This guide is designed to be accessible, providing you with the knowledge to either use or develop your own security-focused extensions effectively.
What is HTTPS Everywhere?
HTTPS Everywhere is a tool you can add to your browser, made by a group called the Electronic Frontier Foundation (EFF). It helps make sure that when you visit websites, your connection to them is secure. Basically, if a website can use a secure connection (HTTPS) but doesn't always do that, HTTPS Everywhere steps in and fixes the URL so you're using HTTPS instead. This happens automatically, so once you've got it installed, you don't have to do anything else.
Here's what you need to know about it:
- It keeps a list of websites that can use HTTPS and makes sure you connect to them securely.
- You don't have to change how you browse the web; it works without you needing to do anything.
- It's made by EFF and some volunteers, and anyone can check its code to make sure it's safe.
- You can use it on most popular browsers like Chrome and Firefox, and it's also part of some privacy-focused browsers.
- If a website already uses HTTPS by default, the extension won't mess with it.
By taking care of HTTPS for you, HTTPS Everywhere makes your online time safer and keeps your private stuff private, without getting in your way.
The role of HTTPS Everywhere in enhancing web security
HTTPS Everywhere helps keep you safe online in several ways:
Encryption - It changes sites to use HTTPS, which means everything you do on those sites is encrypted. This stops people from snooping on what you're doing.
Authentication - It checks that you're actually visiting the website you think you are. This helps stop hackers from tricking you into visiting fake sites.
Integrity - Because it encrypts your data, HTTPS Everywhere also stops hackers from changing the information sent between you and the website. So, what you see is exactly what the website sent.
HSTS Support - It tells browsers to only use secure connections with certain websites, reducing the chance of being redirected to an insecure site.
Mixed Content Blocking - The tool stops insecure items like images or scripts from loading on secure pages, which helps keep your information safe.
In simple terms, by making sure you're using secure HTTPS connections, HTTPS Everywhere helps protect your online activities from eavesdroppers, impersonators, and tamperers. The EFF says that by 2021, HTTPS Everywhere has made over 1 trillion web requests safer. They keep updating it to cover more websites that support HTTPS but don't automatically use it.
Setting Up Your Development Environment
Prerequisites for Chrome extension development
Before you start making a Chrome extension like HTTPS Everywhere, you need a few things:
- A program for writing code, such as Visual Studio Code
- Node.js and npm for handling parts of your project
- Git and GitHub for keeping track of changes
- Chrome browser for trying out your extension
- A good grasp of JavaScript, HTML, and CSS
- Some understanding of how websites talk to each other securely and how to use web tools like storage and tabs
Make sure you have these ready to go before diving into creating your extension.
Tools and technologies needed (HTML, CSS, JavaScript)
Making a Chrome extension uses the same stuff that websites do: HTML, CSS, and JavaScript. Here's a quick rundown:
HTML is what you use to set up what your extension looks like and where things go. It's the foundation.
CSS makes everything look nice. It lets you pick colors, sizes, and how things are laid out.
JavaScript makes things work. It lets your extension do stuff, like react when someone clicks a button.
Together, these three let you build an extension that not only looks the way you want but also does what you want. You'll also use JavaScript for working with web tools that let your extension save data, open new tabs, or check web requests.
Getting comfy with HTML, CSS, and JavaScript is key, so make sure you're solid on these before jumping into making extensions.
Creating Your First HTTPS Everywhere Extension
Step 1: Manifest.json File
Overview of the manifest.json file and its significance
The manifest.json file is like a passport for your Chrome extension. It tells Chrome all the important stuff about your extension, like its name, what it does, and what special permissions it needs. For an HTTPS Everywhere extension, this file is crucial because it helps set up secure connections and tells the browser how to keep your web browsing safe.
Key elements to include in your manifest.json for HTTPS Everywhere
Your manifest file should have:
- "permissions": ["webRequest", "webRequestBlocking"] - To let your extension check and change web requests.
- "background": {"service_worker": "background.js"} - For tasks that run in the background.
- "content_scripts": [...] - For scripts that work directly on web pages.
- "declarative_net_request": {"rule_resources": [...] } - For rules on how to change website addresses to use HTTPS.
Adding permissions like "privacy" and "storage" can also help manage your extension better.
Step 2: Implementing Service Workers
Role of service workers in Chrome extensions
Service workers are like little helpers that work behind the scenes. They can do things like changing web requests to make sure they're secure, even when no web page is open. This is perfect for HTTPS Everywhere because it's all about keeping your browsing secure.
How to use service workers for HTTPS Everywhere functionalities
You can use service workers to automatically change website addresses from HTTP to HTTPS, making sure your connection is always secure. Here's a simple way to do it:
chrome.webRequest.onBeforeRequest.addListener(details => {
let newUrl = details.url.replace("http:", "https:");
return {redirectUrl: newUrl};
}, {urls: ["<all_urls>"]});
Step 3: Content Scripts
Explanation of content scripts
Content scripts let you run JavaScript right on web pages. They can change what you see on a page or how it behaves, which is useful for making sure all parts of a website use HTTPS.
Using content scripts to enforce HTTPS on web pages
You can use content scripts to find and fix parts of a website that aren't secure, like images or links that use HTTP. Here's how:
let images = document.querySelectorAll('img[src^="http:"]');
for (let img of images) {
img.src = img.src.replace("http://", "https://");
}
Step 4: Adding UI Elements
Integrating toolbar actions and side panels
You can add buttons or panels to Chrome that let users interact with your extension. This can be a great way to let people customize how the extension works for them.
Customizing the UI for better user interaction
When making your UI, keep it simple and user-friendly. Use clear designs and easy-to-understand controls. Testing your UI with real users can help make it even better.
Step 5: Utilizing DeclarativeNetRequest API
How DeclarativeNetRequest enhances web security
The DeclarativeNetRequest API lets you set rules for how web requests are handled without needing extra scripts. This can make your extension faster and more secure.
Implementing network request interception for HTTPS enforcement
Here's an example of a simple rule to change a website from HTTP to HTTPS:
{
"id": 1,
"priority": 1,
"action": {"type": "redirect", "redirect": {"url": "https://example.org"}},
"condition": {"urls": ["http://example.org"]}
}
Using DeclarativeNetRequest makes it easier to keep your browsing secure by automatically switching to HTTPS.
Designing a High-Quality Extension
Importance of a single, easily understood purpose
When making an extension, it's best to focus on doing one thing really well. If you try to add too many features, it can get confusing and hard to use.
For instance, the HTTPS Everywhere Chrome extension does one thing: it switches your browser to use HTTPS, which is a more secure way of browsing the web. This makes it super clear what the extension is for and why it's useful.
Sticking to one main feature means you can make that feature work really well, which is what users prefer over having lots of features that don't work as they should.
Ensuring compliance with Chrome Web Store policies
If you want your extension to be available on the Chrome Web Store, it has to follow certain rules. These rules are about keeping things safe, private, and running smoothly. Here's a quick look at some of these rules:
Policy | Description |
---|---|
Permissions | Only ask for the access you really need. Explain why if you need more. |
Privacy | Take good care of user's private info and be clear about what you do with it. |
Security | Be smart about how you deal with websites and user data. |
Performance | Make sure your extension doesn't slow down the browser too much. |
User Experience | Make it easy and pleasant to use your extension. |
Following these rules helps make sure your extension gets approved and that people feel safe using it. You can find more details on what's required in the Chrome Developer docs.
sbb-itb-bfaad5b
Testing and Debugging
Strategies for effective testing of your HTTPS Everywhere extension
Testing your HTTPS Everywhere Chrome extension is super important to make sure it works well and keeps your web browsing safe. Here's how to do it right:
Unit testing
- Check each part of your extension to make sure it does what it's supposed to.
- Use tools like Jest, Mocha, or QUnit to help automate these checks.
- Don't forget to test unusual situations to catch any hidden issues.
Integration testing
- After checking the small parts, make sure they all work together as expected.
- Test how different parts of your extension interact.
- Use mock-ups for services your extension depends on.
UI testing
- Go through all the buttons and screens manually to check they work right.
- You can also use tools like Selenium or Puppeteer to automate this.
- Make sure your extension looks good and works well on different browsers and devices.
Security testing
- Try to break into your own extension to find weak spots.
- Look out for common web security issues like XSS and CSRF.
- Make sure your extension uses secure connections and follows web security best practices.
Performance testing
- See how your extension handles a lot of users at once.
- Look for parts that slow down your extension and fix them.
- Cut down on unnecessary internet requests.
Beta testing
- Let a small group of real users try out your extension before everyone else.
- Fix any problems they find.
- Use their feedback to make your extension better.
Debugging common issues encountered during development
When you're building your HTTPS Everywhere extension, you might run into some problems. Here's how to fix them:
CORS issues
- Make sure your backend services are set up right for CORS.
- You can use special tools or browser add-ons for CORS when you're just testing things out.
Messaging errors
- Double-check the messages you're sending and receiving are structured right.
- Make sure you have a plan for handling messages that don't have a matching handler.
Script injection problems
- Use
CSP
to only allow scripts from places you trust. - Always clean up inputs from outside before you use them on your web page.
Certificate errors
- If you're inspecting traffic, make sure to add your certificates correctly.
- Check your certificates are up-to-date and valid.
Compatibility problems
- Use the browser console to look for errors on all the browsers you want your extension to work on.
- If some browsers don't support certain features, you might need to add extra code (polyfills) to make them work.
Performance problems
- Use browser tools to find out where you can make your extension faster.
- For things that happen a lot, like scrolling, try to make them less demanding.
Authorization issues
- Make sure your extension asks for the right permissions in its setup file.
- Check if users are logged in properly for parts of your extension that need it.
Keeping these tips in mind can help you avoid common issues and make a secure, user-friendly HTTPS Everywhere Chrome extension.
Publishing Your Extension
Preparing your extension for the Chrome Web Store
Before you can share your HTTPS Everywhere extension with the world through the Chrome Web Store, you need to get a few things ready. Here's what to do:
Packaging
- Put all your extension's files and pictures into a
.zip
file, just like Chrome wants. - Check that you've included everything needed, like your manifest file, icons, and code.
- Test the zip file by loading it into Chrome to make sure it works right.
Documentation
- Write clear instructions on how to install and use your extension for your store page.
- Add a
README
file that explains what your extension does and how it works. - Say thank you to any third-party tools or images you used.
Branding
- Create a nice icon and banners for your page in the store.
- Make sure all images fit the Chrome Web Store's size requirements.
- Pick a name for your extension that's easy to remember and explains what it does.
Testing
- Check your extension for any bugs, security issues, or slow spots.
- Ensure it works well on all versions of Chrome that are supported.
- Set up a way to keep an eye on any crashes, errors, or feedback from users.
Doing these steps will help make sure your extension is ready to go live without any hitches!
The submission process: A step-by-step guide
Here's how to get your extension into the Chrome Web Store:
1. Sign up as a Chrome Developer
- Visit the Chrome Developer Dashboard.
- Use your Google Account to sign in.
2. Click "Add new item" and upload your extension's .zip
package.
3. Fill out the listing details like:
- The title, description, pictures, and categories.
- Your privacy policy and how you rate your content.
- If you're selling your extension, set the price and choose the countries it'll be available in.
4. Pay the one-time developer fee of $5 USD.
5. Make sure your listing follows all Store policies.
6. Click Publish
to send your extension in for review.
7. Wait for the review process to finish. The team will check if your extension meets all the rules. This might take anywhere from 1 to 7 days.
8. Once approved, your extension will be live for people to find and use!
After your extension is out there, remember to keep it updated, help users if they have questions, and keep an eye on how it's doing. If you need help or have questions, you can always talk to the Chrome Web Store team.
Conclusion
The HTTPS Everywhere Chrome extension is super important for both people who make websites and those who visit them. It helps keep your online visits safe by making sure you're always using a secure connection. This stops others from sneaking a peek at what you're doing or trying to trick you. As more websites start using HTTPS, this extension adds an extra layer of safety.
In this guide, we talked about how to make a similar extension for Chrome. We went through the steps like setting up the right permissions, using background helpers, and changing website addresses to be secure. We also touched on how to make your extension easy for people to use and follow the rules for the Chrome Web Store.
Before you share your extension with the world, testing it well is super important. This makes sure it works great and doesn't cause any trouble while browsing.
Now, you know how to make your own secure browsing tool. You could even add more cool features like fixing mixed content or checking if a website's security certificate is legit. Making an extension like this helps everyone browse the web safely.
HTTPS Everywhere is a project that anyone can help make better. Whether you're adding to it or making something new, focusing on keeping things private online is a big deal. It's all about making sure that using the web safely is easy for everyone.
Related Questions
Is HTTPS Everywhere discontinued?
Yes, the folks who made HTTPS Everywhere, the Electronic Frontier Foundation (EFF), said in 2021 that they're not going to update it anymore. That's because browsers like Chrome, Firefox, and Edge now make sure websites use HTTPS automatically. The EFF suggests using your browser's own HTTPS settings for safer surfing. While HTTPS Everywhere will still work for a bit, it won't be updated to keep up with new browser changes.
How do I install HTTPS Everywhere in Chrome?
To get HTTPS Everywhere on Chrome:
- Head over to the Chrome Web Store
- Click "Add to Chrome"
- Confirm you want to add the extension
Once it's added, HTTPS Everywhere will automatically switch insecure HTTP sites to more secure HTTPS ones when possible.
Do I still need HTTPS Everywhere?
Nowadays, you don't really need HTTPS Everywhere because browsers like Chrome, Firefox, and Edge can force websites to use HTTPS on their own. Here's how to turn it on:
- Chrome: Go to
Settings > Privacy and Security
and turn onUse secure DNS
- Firefox: Go to
Settings > General > Browsing
and tickAutomatically use HTTPS
- Edge: Go to
Settings > Privacy, search and services > Security
and turn onUse secure DNS
Turning this on makes sure all your website visits are over HTTPS, so you don't need an extra extension.
How do I enable Chrome extension developer mode?
If you're trying to test an extension in Chrome that's not in the web store, do this:
- Open Chrome and go to
chrome://extensions
- At the top right, switch on
Developer mode
- Click
Load unpacked
and pick your extension's folder
Developer mode lets you try out extensions before they're officially out. Just remember to turn it off when you're done testing to keep your browser safe.