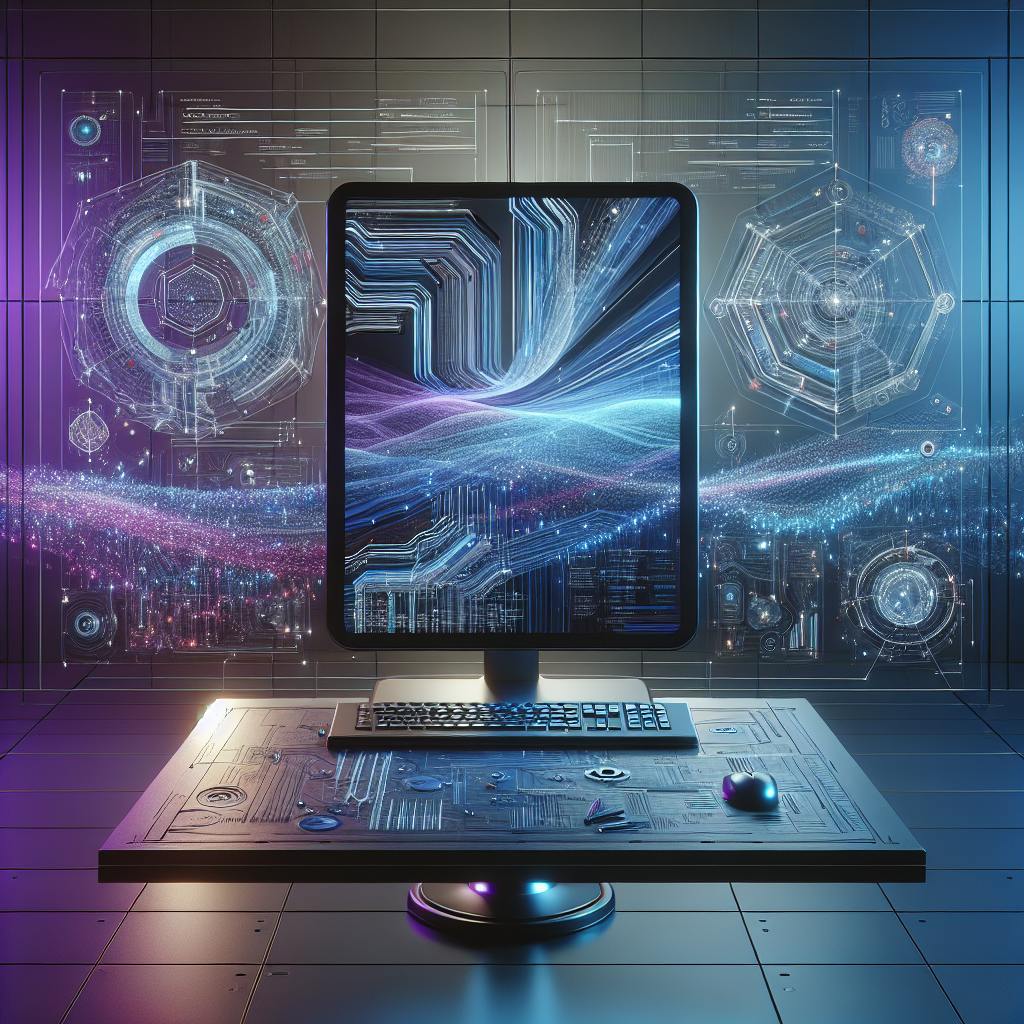
Learn how to master algorithm complexity by optimizing time and space. Explore key points, common classes, optimization strategies, and advanced topics in this comprehensive guide.
Algorithms are at the heart of software systems, and their efficiency is crucial for performance and scalability. This guide covers the essentials of analyzing and optimizing algorithm complexity in terms of time and space.
Key Points:
- Time Complexity measures how an algorithm's running time increases with input size
- Common classes: O(1), O(log n), O(n), O(n log n), O(n^2)
- Analyze by counting operations and expressing as a function of input size
- Space Complexity measures an algorithm's memory usage
- Common classes: O(1), O(n), O(n^2)
- Analyze by counting variables, data structures, and function calls
- Optimization Strategies:
- Choose efficient data structures and algorithms
- Reduce unnecessary operations
- Apply techniques like divide and conquer, dynamic programming, and greedy algorithms
- Balancing Time and Space:
- Consider trade-offs between time, space, readability, and simplicity
- Choose algorithms based on input size, memory constraints, and performance needs
Quick Comparison of Common Time Complexities:
Algorithm | Time Complexity |
---|---|
Linear Search | O(n) |
Binary Search | O(log n) |
Bubble Sort | O(n^2) |
Merge Sort | O(n log n) |
Fibonacci (Recursive) | O(2^n) |
The guide also covers advanced topics like amortized analysis, randomized algorithms, parallel and distributed algorithms, and approximation algorithms.
Related video from YouTube
Understanding Time Complexity
Time Complexity Explained
Time complexity measures how long an algorithm takes to run based on the input size. It shows how the running time increases as the input grows. Knowing time complexity helps in assessing an algorithm's performance, especially with large datasets.
Common Time Complexity Classes
- O(1) - Constant Time: The running time is the same, no matter the input size. Examples: Accessing an array element, stack operations.
- O(log n) - Logarithmic Time: The running time grows logarithmically with the input size. Examples: Binary search, finding the longest word in a sorted list.
- O(n) - Linear Time: The running time grows linearly with the input size. Examples: Iterating through an array, linear search.
- O(n log n) - Linearithmic Time: The running time grows as a product of linear and logarithmic factors. Examples: Merge Sort, Heap Sort.
- O(n^2) - Quadratic Time: The running time grows quadratically with the input size. Examples: Nested loops, Bubble Sort.
Algorithm Examples with Time Complexities
Algorithm | Time Complexity |
---|---|
Linear Search | O(n) |
Binary Search | O(log n) |
Bubble Sort | O(n^2) |
Merge Sort | O(n log n) |
Fibonacci (Recursive) | O(2^n) |
Analyzing Time Complexity
- Identify the input size 'n'.
- Count the number of operations for each step.
- Express the total operations as a function of 'n' using Big O notation.
- Consider the worst-case scenario.
Analyzing Time Complexity Step-by-Step
Step-by-Step Process
To analyze the time complexity of an algorithm, follow these steps:
- Identify the input size (n): Determine the primary input that affects the algorithm's performance, typically represented by a variable like 'n'.
- Break down the algorithm: Divide the algorithm into its basic operations (e.g., arithmetic, comparisons, assignments, loops, recursive calls).
- Count operations: Calculate how many times each operation is performed in relation to the input size 'n'.
- Express as a function of 'n': Represent the total number of operations as a function of 'n' using Big O notation (e.g., O(n), O(n^2), O(log n)).
- Consider the worst case: Analyze the time complexity for the worst-case scenario, where the algorithm performs the maximum number of operations.
Identifying Input Size and Operations
The input size is the primary factor that influences an algorithm's performance. It could be the length of an array, the number of nodes in a tree, or the size of a matrix. Clearly identifying the input size is crucial for accurate time complexity analysis.
After determining the input size, identify the key operations within the algorithm that contribute to its time complexity. These may include loops, recursive calls, comparisons, arithmetic operations, and data structure operations.
Using Big O Notation
Big O notation is a standardized way to express the time complexity of an algorithm. It represents the upper bound of an algorithm's growth rate as the input size increases. Common Big O notations include:
- O(1) - Constant time
- O(log n) - Logarithmic time
- O(n) - Linear time
- O(n log n) - Linearithmic time
- O(n^2) - Quadratic time
By expressing the total number of operations as a function of 'n' using Big O notation, you can easily compare the time complexities of different algorithms.
Best, Average, and Worst Cases
When analyzing time complexity, it's essential to consider the best, average, and worst-case scenarios. The worst-case scenario represents the maximum number of operations an algorithm can perform, providing a conservative estimate of its performance.
While the worst-case analysis is often the primary focus, understanding the best and average cases can also be valuable. The best case represents the minimum number of operations, while the average case considers all possible inputs and calculates the average performance.
Evaluating algorithms across different scenarios helps identify potential performance bottlenecks and guides optimization efforts.
Optimizing Time Complexity
Optimizing time complexity is key to developing efficient algorithms. This section covers strategies and techniques to reduce the time complexity of algorithms, making them faster and more scalable.
Optimization Strategies
Consider these strategies to improve an algorithm's performance:
- Choosing the right data structure: Using the appropriate data structure can reduce time complexity. For example, a hash table can lower time complexity from O(nยฒ) to O(n) in some cases.
- Utilizing efficient algorithms: Implementing efficient algorithms like divide-and-conquer or dynamic programming can significantly reduce time complexity.
- Reducing unnecessary operations: Eliminating redundant operations can improve an algorithm's time complexity.
Optimization Techniques
Here are some techniques to reduce time complexity:
- Divide and conquer: Break down a problem into smaller subproblems and solve them recursively.
- Dynamic programming: Store solutions to subproblems and reuse them to avoid redundant calculations.
- Greedy algorithms: Make the optimal choice at each step, aiming for a global optimum.
Time Complexity Trade-offs
When optimizing time complexity, consider these trade-offs:
Trade-off | Description |
---|---|
Time vs. Space | Reducing time complexity may increase space complexity, and vice versa. |
Time vs. Readability | Optimizing time complexity may make the code less readable. |
Time vs. Simplicity | Simplifying an algorithm may increase its time complexity. |
Understanding Space Complexity
Space complexity is a key part of algorithm analysis. It affects how well an algorithm performs and scales. Let's explore what space complexity is, why it matters, and how to analyze it.
Space Complexity Explained
Space complexity is the amount of memory an algorithm needs to solve a problem. This includes memory for variables, data structures, function calls, and temporary storage. Knowing space complexity helps in managing memory use, especially in limited environments.
Common Space Complexity Classes
Here are some common space complexity classes:
Class | Description |
---|---|
O(1) | Constant space; memory use doesn't change with input size. |
O(n) | Linear space; memory use grows linearly with input size. |
O(n^2) | Quadratic space; memory use grows quadratically with input size. |
Algorithm Examples with Space Complexities
Here are examples of algorithms with different space complexities:
- Binary Search: O(1) space complexity, as it only needs fixed memory for the search key and array indices.
- Merge Sort: O(n) space complexity, as it needs extra memory for temporary arrays during merging.
- Fibonacci Sequence: O(n) space complexity, as it needs memory to store previous Fibonacci numbers.
Analyzing Space Complexity
To analyze space complexity, follow these steps:
- Identify the input size: Determine the size of the input data affecting memory use.
- Count the memory usage: Count variables, data structures, and function calls needing memory.
- Determine the space complexity class: Based on memory usage, classify it as O(1), O(n), or O(n^2).
sbb-itb-bfaad5b
Analyzing Space Complexity Step-by-Step
Step-by-Step Process
Analyzing space complexity involves a systematic approach to identify the memory usage of an algorithm. Here's a step-by-step process to help you analyze space complexity:
- Identify the input size: Determine the size of the input data that affects memory usage.
- Count the memory usage: Count variables, data structures, and function calls that require memory.
- Determine the space complexity class: Based on memory usage, classify it as O(1), O(n), or O(n^2).
Identifying Memory Usage
To identify memory usage, consider the following:
- Input size: The size of the input data affects memory usage.
- Variables and data structures: Count the memory required for variables, arrays, linked lists, trees, and other data structures.
- Function calls: Consider the memory required for function calls, including recursive calls and stack memory.
- Auxiliary data structures: Identify any additional data structures used during the algorithm's execution, such as temporary arrays or hash tables.
Using Big O Notation
Represent space complexity using Big O notation, which provides an upper bound on the memory usage. For example:
- O(1) represents constant space complexity, where memory usage does not change with input size.
- O(n) represents linear space complexity, where memory usage grows linearly with input size.
- O(n^2) represents quadratic space complexity, where memory usage grows quadratically with input size.
Best, Average, and Worst Cases
Evaluate space complexity across different scenarios:
- Best case: The algorithm's memory usage when the input is optimized for minimal memory usage.
- Average case: The algorithm's memory usage for typical input data.
- Worst case: The algorithm's memory usage when the input is optimized for maximum memory usage.
Optimizing Space Complexity
Optimizing space complexity means reducing the memory an algorithm uses without hurting its performance. This is important in environments with limited memory, like embedded systems or mobile devices.
Optimization Strategies
Here are some strategies to reduce space complexity:
- Choose the right data structures: Use data structures that need less memory. For example, a hash table can use less memory than a binary search tree.
- Use efficient algorithms: Replace algorithms with high space complexity with those that use less memory. For instance, a recursive algorithm might use less memory than an iterative one.
- Reduce auxiliary space: Minimize the use of extra data structures like temporary arrays or variables.
Optimization Techniques
Several techniques can help optimize space complexity:
- Dynamic programming: Break down problems into smaller parts and store their solutions to avoid redundant calculations.
- Memoization: Save the results of expensive function calls and reuse them when needed.
- Space-time trade-offs: Sometimes, using a slower algorithm with lower space complexity can be more efficient than a faster one with higher space complexity.
Space Complexity Trade-offs
Optimizing space complexity often involves trade-offs with other aspects of algorithm design:
Trade-off | Description |
---|---|
Time vs. Space | Reducing space complexity may increase time complexity, and vice versa. |
Code Readability | Optimizing space complexity may make the code harder to read. |
Scalability | Optimizing space complexity can improve scalability but may limit the algorithm's ability to handle large inputs. |
Balancing Time and Space Complexity
Balancing time and space complexity is key in algorithm design. Optimizing one can negatively impact the other. This section explains why balancing both is important, strategies to achieve it, and examples of trade-offs.
Considering Both Complexities
Focusing only on time or space complexity can lead to poor solutions. An algorithm with low time complexity might use too much memory, making it unsuitable for limited-resource environments. Conversely, an algorithm with low space complexity might be slow, leading to poor performance. Considering both helps create efficient algorithms.
Balancing Strategies
To balance time and space complexity, use these strategies:
- Space-time trade-offs: Sometimes, a slower algorithm with lower space complexity is better than a faster one with higher space complexity.
- Dynamic programming: Break problems into smaller parts and store their solutions to avoid redundant calculations.
- Memoization: Save results of expensive function calls and reuse them when needed.
Time-Space Trade-off Examples
Here are some examples of time-space trade-offs:
Algorithm | Time Complexity | Space Complexity |
---|---|---|
Merge Sort | O(n log n) | O(n) |
Quick Sort | O(n log n) | O(log n) |
Bubble Sort | O(n^2) | O(1) |
Merge sort has higher space complexity than quick sort but similar time complexity. Bubble sort has lower space complexity but higher time complexity.
Choosing an Algorithm
When choosing an algorithm, consider:
- Input size
- Memory constraints
- Performance needs
For limited memory environments, a space-efficient algorithm might be better, even if it's slower. If speed is critical, a time-efficient algorithm might be chosen, even if it uses more memory. Balancing these factors helps create efficient algorithms.
Advanced Topics
Amortized Analysis
Amortized analysis looks at the average time per operation over a sequence of operations. Instead of analyzing each operation individually, it provides a bound on the total time for a series of operations. This is useful when some operations are costly, but most are cheap.
In amortized analysis, we use a potential function to relate the amortized time to the actual time, giving an upper bound on the total time.
Randomized Algorithms
Randomized algorithms use random numbers to make decisions during execution. They are useful when no efficient deterministic algorithm exists. There are two types:
- Las Vegas algorithms: Always give the correct result but may take varying amounts of time.
- Monte Carlo algorithms: Always run in the same time but may give incorrect results.
These algorithms are often used in cryptography, data structures, and optimization.
Parallel and Distributed Algorithms
Parallel and distributed algorithms use multiple processors or nodes to solve problems faster.
- Parallel algorithms: Used in scientific simulations, data analysis, and machine learning.
- Distributed algorithms: Used in cloud computing, network protocols, and distributed databases.
When designing these algorithms, consider communication overhead and synchronization between processors or nodes.
Approximation Algorithms
Approximation algorithms find near-optimal solutions when exact solutions are impractical due to high computational cost. They are often used for NP-complete problems.
- Approximation schemes: Provide a guarantee on the solution quality.
- Heuristic algorithms: Do not guarantee solution quality but often perform well in practice.
These algorithms are used in optimization, machine learning, and data analysis.
Conclusion
Key Takeaways
In this guide, we've covered the essentials of algorithm complexity, including time and space complexity, optimization strategies, and advanced topics. Here are the main points:
- Time and Space Complexity: Key for writing efficient algorithms.
- Optimization: Analyze complexity, find bottlenecks, and apply techniques.
- Balancing: Essential for optimal performance.
- Advanced Topics: Amortized analysis, randomized algorithms, parallel and distributed algorithms, and approximation algorithms.
Continuous Learning
Mastering algorithm complexity is an ongoing process. To keep improving:
- Practice on platforms like LeetCode, HackerRank, and CodeChef.
- Study and learn from others' solutions.
- Stay updated with the latest in algorithms and data structures.
- Apply your knowledge to real-world problems.
Further Resources
To deepen your understanding, check out these resources:
- "Introduction to Algorithms" by Cormen et al.
- "Algorithms" by Sedgewick and Wayne
- "The Algorithm Design Manual" by Skiena
- Online courses on Coursera, edX, and Udemy
- Research papers and articles on algorithm complexity and optimization
FAQs
What is the optimization algorithm?
Optimization algorithms use mathematical models and methods to find the best solution. They start with initial solutions and improve them step-by-step by evaluating their effectiveness based on a specific goal and constraints.