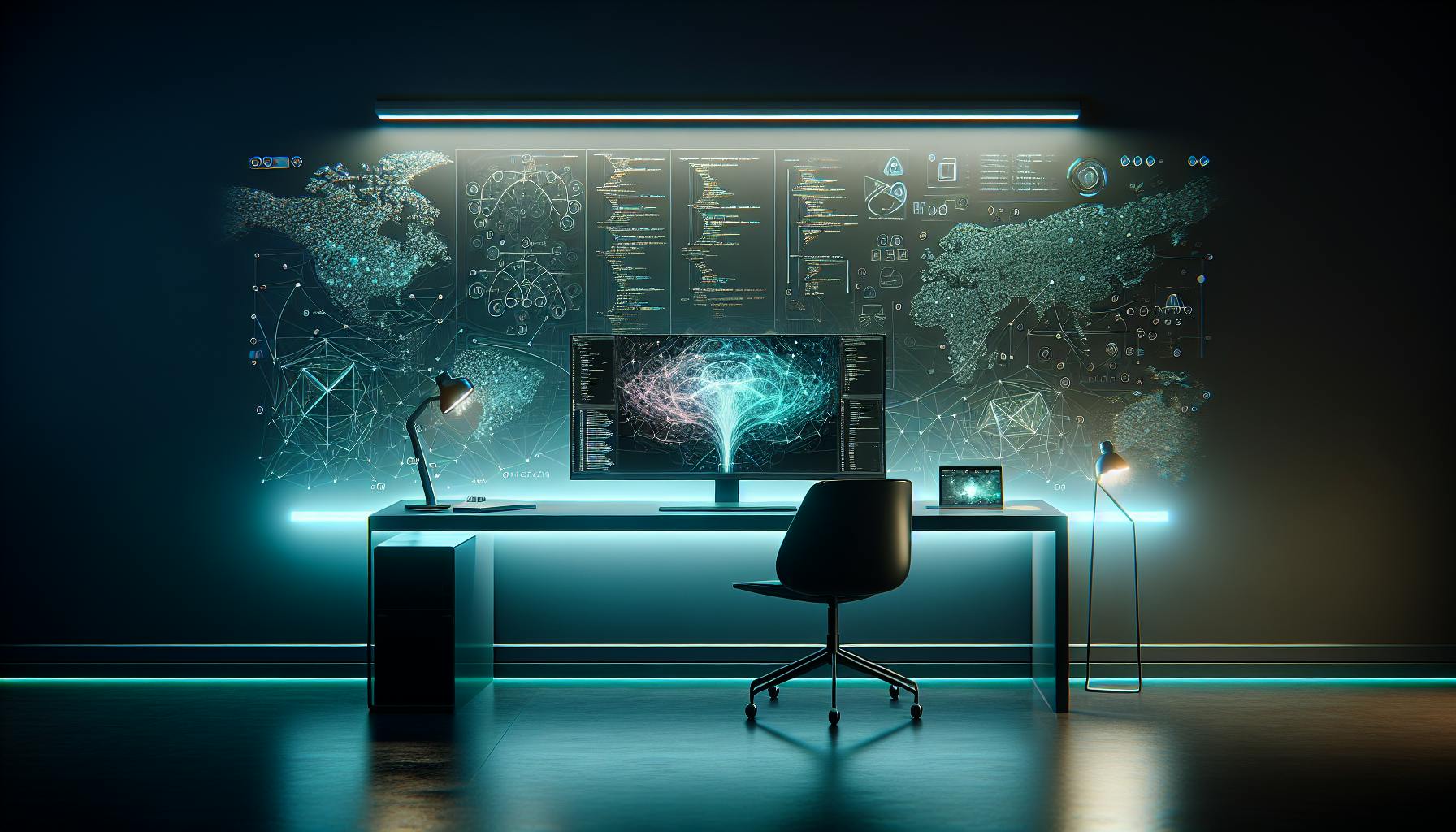
Discover the power of Scikit-learn for continuous education in machine learning. Learn about easy installation, wide applications, versatile toolset, and free commercial use.
Scikit-learn is a versatile Python library designed for machine learning. It supports various tasks such as classification, regression, and clustering, making it a go-to tool for beginners and professionals alike. Here's what you need to know:
- Easy Installation: Get started with
pip install scikit-learn
. - Wide Range of Applications: Whether you're into finance, healthcare, or any data-driven field, scikit-learn has you covered.
- Continuous Learning: The library is constantly updated, offering a plethora of resources for learning and experimentation.
- Versatile Toolset: From basic linear models to complex ensemble methods, scikit-learn equips you with the algorithms you need.
- Community and Professional Use: Highly regarded in both open-source communities and professional settings.
- Free for Commercial Use: Available under the BSD license, allowing for broad usage without cost.
Whether you're starting your first ML project or looking to keep your skills sharp, scikit-learn provides a solid foundation for continuous learning and development in the fast-evolving field of machine learning.
Continuous Education in ML
Machine learning is always changing, so you need to keep learning to stay on top. Scikit-learn is full of resources to help you do just that. You can try out new ideas, see how well they work, and learn from examples.
Scikit-learn is perfect for keeping your skills fresh. It helps you practice with real data and learn new techniques as they come out. Plus, there are lots of online courses and guides that go well with scikit-learn, making it a great set of tools for learning machine learning, no matter if you're just starting or already know a lot.
Getting Started with Scikit-Learn
Installation and Setup
First off, to get scikit-learn on your computer, you just need to type this into your computer:
pip install scikit-learn
You'll also want something called Jupyter notebooks, which lets you see your work as you go. Install it with:
pip install jupyter
It's a good idea to keep your machine learning (ML) projects in their own space, called a virtual environment. Set one up like this:
python -m venv myenv
And if you're using Linux or macOS, you can start using it by typing:
source myenv/bin/activate
Now, you're ready to start using scikit-learn by adding it to your project with:
import sklearn
You'll also need two other things: NumPy for working with numbers, and matplotlib for making graphs. Get them the same way you got scikit-learn.
Your First ML Project
Let's try something fun: predicting the price of houses with a dataset that scikit-learn gives you for free.
First, look at the data:
from sklearn.datasets import load_boston
boston = load_boston()
print(boston.data.shape)
print(boston.target.shape)
This shows us we have data on 506 houses, and for each one, we have 13 different kinds of information (like the number of rooms or how old it is), plus the price.
Next, we split our data into two groups: one to learn from and one to test our learning:
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(boston.data, boston.target)
Now, let's use a simple method called linear regression to predict house prices:
from sklearn.linear_model import LinearRegression
model = LinearRegression()
model.fit(X_train, y_train)
To see how well our model did, we check it against our test data:
print(model.score(X_test, y_test))
You can play around with the model settings to make it better, use different methods like random forests, or check your work with something called cross-validation. The scikit-learn website has lots of ideas to help you learn more.
Key Concepts for Effective ML
ML Fundamentals
Understanding a few basic ideas can really help when you're working with machine learning (ML) models. Let's break down some key concepts you'll see in scikit-learn:
Features and Labels
- Features are the bits of information you feed into the model to help it make predictions. Think of them as clues. For a house price model, these clues could be things like how many bedrooms a house has, where it's located, or how big it is.
- Labels are what you're trying to figure out. In our house price example, the label would be the actual price of the house.
Training and Testing Data
- Before a model is ready to go, it needs to learn from some data. The training data is what it practices on.
- Testing data is fresh data the model hasn't seen before, used to check how well it's learned.
Model Evaluation Metrics
It's important to know if your model is doing a good job. Here are some ways to tell:
- Accuracy: How often the model's predictions are right.
- Precision/Recall: How good the model is at identifying true positives and negatives.
- Mean Squared Error: How far off the model's predictions are from the actual values.
Supervised vs Unsupervised Learning
- Supervised: This is when models learn from data that's already been labeled. It's like having an answer key. Examples include predicting prices (regression) or sorting emails into spam and not spam (classification).
- Unsupervised: Here, models try to find patterns in data that doesn't have labels. It's like solving a puzzle without a picture. Examples include grouping similar customers together (clustering).
Here's how you might set up a simple linear regression model in scikit-learn:
from sklearn.linear_model import LinearRegression
X_train, X_test, y_train, y_test = train_test_split(data.data, data.target)
model = LinearRegression()
model.fit(X_train, y_train)
print(model.score(X_test, y_test))
Tuning and Validation
Getting your ML model just right involves a couple of steps:
Cross-Validation
- This is like doing a practice test multiple times to make sure your model really knows its stuff.
Hyperparameter Tuning
- This is about adjusting the model's settings to get the best performance. Think of it like fine-tuning a radio to get the clearest signal.
Here's an example of how you might search for the best model settings:
from sklearn.model_selection import GridSearchCV
params = {"n_neighbors": [3, 5, 7],
"weights": ["uniform", "distance"]}
grid_search = GridSearchCV(KNeighborsClassifier(), params, cv=5)
grid_search.fit(X_train, y_train)
print(grid_search.best_params_)
print(grid_search.best_score_)
The goal is to keep improving your model's ability to make accurate predictions, without it just memorizing the training data. Scikit-learn gives you all the tools you need to test and adjust your models for the best results.
Hands-On Algorithms
Linear Models
Linear models like linear and logistic regression are the starting points in machine learning with scikit-learn. We'll walk you through how to use these models on real data, focusing on:
- Model evaluation - This is about figuring out how well your model is doing. We'll use methods like cross-validation to make sure our models work well not just once, but consistently.
- Regularization - This is a way to keep your model simple to prevent it from making mistakes by overthinking. We'll adjust the strength of regularization to find the best balance.
- Pipelines - These help you keep your data processing and model training steps consistent, especially when testing your models.
Let's start with predicting house prices using the Boston housing dataset and linear regression:
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import cross_val_score
reg = LinearRegression()
scores = cross_val_score(reg, X, y, cv=5, scoring='r2')
print("Cross-validated R^2: ", scores.mean())
To make our model even better, we add regularization:
from sklearn.linear_model import Ridge
ridge = Ridge(alpha=0.1)
scores = cross_val_score(ridge, X, y, cv=5, scoring='r2')
print("Regularized R^2: ", scores.mean())
For sorting things into categories (classification), we'll use logistic regression:
from sklearn.linear_model import LogisticRegression
logreg = LogisticRegression()
logreg.fit(X_train, y_train)
print("Accuracy:", logreg.score(X_test, y_test))
Ensemble Methods
Ensemble methods like random forests and gradient boosting mix different models to predict better. Here's how to do it with scikit-learn:
Random Forests
from sklearn.ensemble import RandomForestClassifier
rf = RandomForestClassifier(n_estimators=100)
rf.fit(X_train, y_train)
print("Accuracy:", rf.score(X_test, y_test))
print("Feature importances:", rf.feature_importances_)
We can adjust settings like max_depth
to keep our model simple and effective.
Gradient Boosting Machines
from sklearn.ensemble import GradientBoostingClassifier
gb = GradientBoostingClassifier(n_estimators=100, learning_rate=0.1)
gb.fit(X_train, y_train)
print("Accuracy:", gb.score(X_test, y_test))
Adjusting the learning_rate
helps avoid overfitting. Let's also look at which features are most important:
import matplotlib.pyplot asplt
plt.barh(range(X.shape[1]), gb.feature_importances_)
plt.yticks(range(X.shape[1]), X.columns)
Usually, mixing models like this gives us better results than just using one model. Scikit-learn makes it simple to combine different models' strengths.
sbb-itb-bfaad5b
Advanced Techniques
Text Classification
Text classification helps computers understand what words mean, like figuring out if an email is spam or not. Here's how to do it step-by-step with scikit-learn, a tool for machine learning:
First, we turn words into numbers because computers are better with numbers. We use special tools (CountVectorizer
and TfidfTransformer
) to do this. It's like making a list of all the words and how often they show up:
from sklearn.feature_extraction.text import CountVectorizer, TfidfTransformer
count_vect = CountVectorizer()
tfidf_transformer = TfidfTransformer()
X_train_counts = count_vect.fit_transform(X_train)
X_train_tfidf = tfidf_transformer.fit_transform(X_train_counts)
Next, we make sure these numbers are easy for the computer to work with by normalizing them. Think of it as making sure all the numbers are in a similar range:
from sklearn.base import BaseEstimator, TransformerMixin
class Normalizer(BaseEstimator, TransformerMixin):
def fit(self, X, y=None):
return self
def transform(self, X, y=None):
return normalize(X)
We put all these steps into a pipeline, adding a machine learning model at the end. Here, we're using Logistic Regression:
from sklearn.pipeline import Pipeline
from sklearn.linear_model import LogisticRegression
pipe = Pipeline([
('count_vect', CountVectorizer()),
('tfidf', TfidfTransformer()),
('norm', Normalizer()),
('clf', LogisticRegression())
])
Finally, we use a tool called GridSearch to find the best settings for our model:
from sklearn.model_selection import GridSearchCV
params = {
'vect__ngram_range': [(1, 1), (1, 2)],
'clf__C': [0.001, 0.01, 1, 10]
}
grid = GridSearchCV(pipe, param_grid=params, cv=5)
grid.fit(X_train, y_train)
This helps us make a really good system for classifying text.
Computer Vision
Now, let's talk about how computers can learn to recognize images. Here's a step-by-step guide:
First, we get our images ready and use a tool called VGG16 to pick out important features from the images. Think of it as the computer looking at the picture and noting down important details:
from keras.applications.vgg16 import VGG16
from keras.preprocessing import image
from keras.applications.vgg16 import preprocess_input
import numpy as np
model = VGG16(weights='imagenet', include_top=False)
features = model.predict(preprocessed_images)
features = features.reshape(features.shape[0], -1)
Next, we make our dataset bigger by slightly changing the images in different ways. This helps the computer learn better:
from keras.preprocessing.image import ImageDataGenerator
datagen = ImageDataGenerator(
rotation_range=40,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
fill_mode='nearest')
augmented_images = datagen.flow(images, labels, batch_size=10)
We also make our model smarter by adding something called dropout. This helps prevent the model from making mistakes by relying too much on the training data:
from sklearn.linear_model import LogisticRegression
clf = LogisticRegression(C=1, penalty='l2',
random_state=0,
solver='lbfgs',
multi_class='multinomial',
max_iter=1000)
clf = clf.set_params(dropout=0.25)
By following these steps, we've made a smart system for recognizing images.
Continual Learning
Monitoring Data Drift
Imagine you built a program to guess house prices. Over time, what makes a house expensive might change. Maybe being close to a bus stop becomes more important, or how many bedrooms a house has matters less.
Scikit-learn helps you keep track of these changes. It has tools to measure if the new information you're getting is different from the old one:
- Kullback-Leibler (KL) divergence is a fancy way to say measuring the difference. Here's how you might do it:
from sklearn.metrics import pairwise_distances
kl_div = pairwise_distances(X_train, X_new, metric='kl')
-
You can also draw graphs to show how these changes happen over time.
-
If the changes are big enough, you might decide to update your program to keep it accurate.
Checking for these changes regularly helps you know when it's time to make your program better.
Automated Retraining
When you see that it's time to update your program because of these changes, you can set up a system to do it automatically. Scikit-learn can help you:
- Load new data from wherever you keep it.
- Preprocess this data, just like you did before.
- Retrain your program with the new data.
- Evaluate how well the updated program works.
- If it works better, keep this new version.
Here's a simple way to do it:
pipe = Pipeline([
('new_data', NewData()),
('preprocess', Transform()),
('model', ReTrainModel())
])
pipe.fit(X_test, y_test)
pipe.score(X_test, y_test)
This setup makes sure your program stays up-to-date without you having to check and update it all the time. Keeping an eye on changes and having an automatic update system means your program can keep learning and getting better on its own.
Conclusion
Scikit-learn is a super useful tool for anyone who wants to keep learning about machine learning, whether you're a developer, a data scientist, or just curious. It's easy to use, has lots of helpful guides, and works well with Python, which is a big plus.
In this guide, we went over everything from how to get started to some more advanced stuff like working with text and images. We showed you examples of how you can use scikit-learn in real projects to get better at machine learning.
If you're interested in getting better at supervised learning stuff (like sorting things into categories or predicting numbers) or unsupervised learning (like finding groups in data), scikit-learn has what you need to practice and improve.
You can learn a lot by using scikit-learn in your own projects, helping out with scikit-learn's open-source projects, or joining in on community discussions. Since it's always being updated with the latest machine learning research, it's a great tool to have for serious projects.
To wrap up, scikit-learn is all about helping you stay curious and keep getting better. We hope you'll use this powerful Python library to keep growing your skills in data science and machine learning.
Related Questions
What is the Scikit learning library mostly used for?
Scikit-learn is a toolbox that's great for machine learning jobs like sorting data (classification), predicting numbers (regression), grouping similar items (clustering), and more. It's packed with tools and algorithms, including support vector machines, random forests, and k-means, making it super handy for machine learning tasks.
What are the disadvantages of Scikit?
Some downsides of using scikit-learn include:
- It doesn't have built-in support for deep learning, which means you'll need to use it with other software like TensorFlow or Keras.
- It's not the best choice for very unique or new types of models because it's a bit rigid.
- For making graphs or exploring your data visually, you'll need to use it with other tools like Matplotlib or Seaborn.
Is scikit-learn used professionally?
Absolutely, scikit-learn is a big hit in the professional world of data science. It's a flexible tool that makes it quick to test ideas and analyze data. Data scientists often use scikit-learn along with other tools like IPython and Pandas for their data analysis projects.
Is sklearn free for commercial use?
Yes, scikit-learn is totally free to use, whether for work or personal projects. It's shared under the BSD license, which means you can use, change, and share it as much as you like, without any cost.