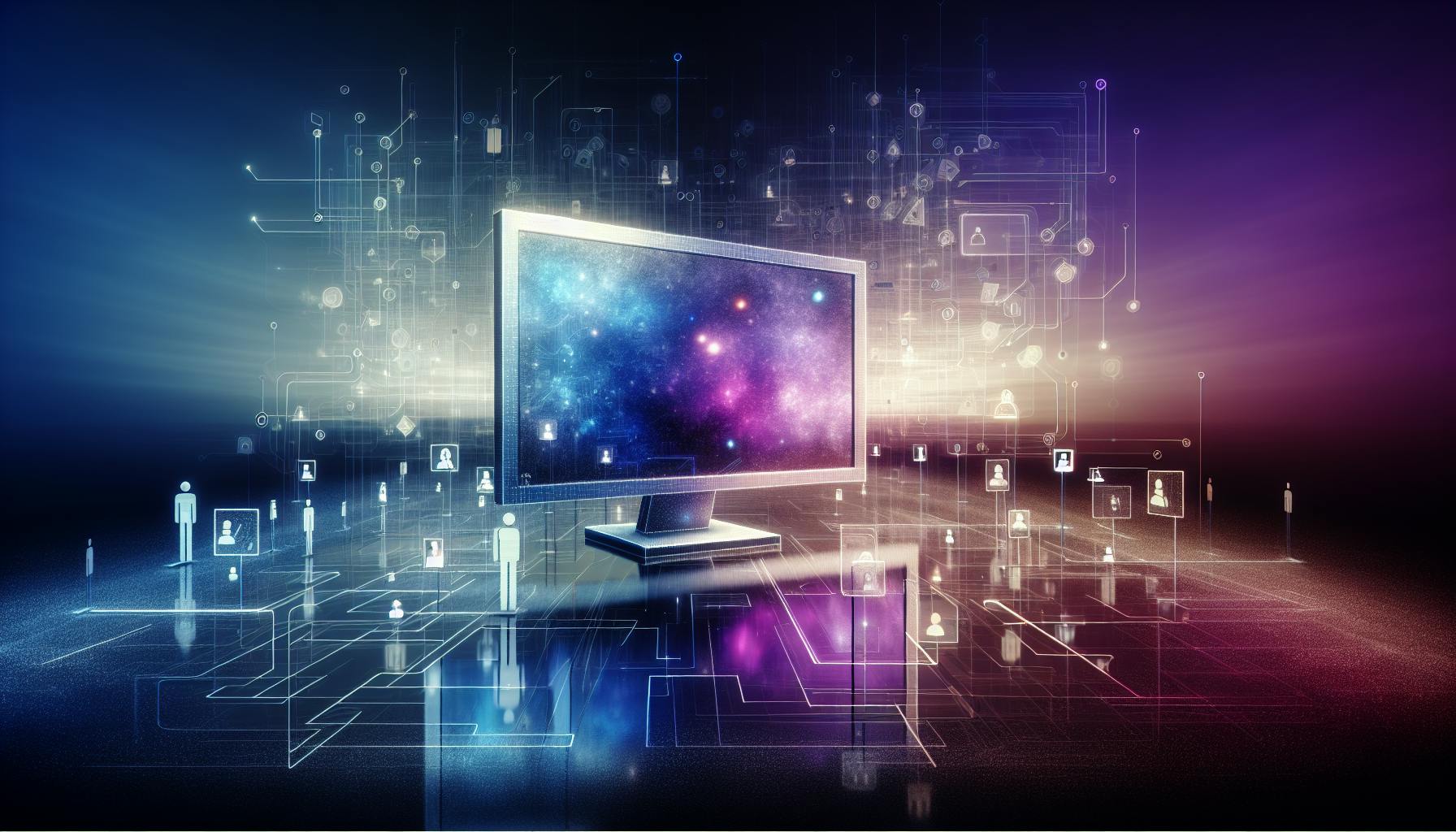
Learn the basics of WebRTC, how it works, setting up your development environment, deep diving into WebRTC APIs, building a simple video chat app, advanced features, common challenges, real-world applications, and more.
WebRTC stands for Web Real-Time Communication, a powerful tool that allows for live video chats, file sharing, and more directly from your web browser, without any additional plugins. Whether you're looking to add real-time communication features to your apps or websites, or just curious about how WebRTC works, this guide covers the essentials, including:
- What WebRTC is: A free project enabling real-time voice, video, and data sharing.
- How it works: Through APIs like MediaStream, RTCPeerConnection, and RTCDataChannel for accessing media devices, managing connections, and transferring data.
- Setting up: The basics of setting up your environment with the right tools and libraries such as
adapter.js
. - Deep dive into APIs: Understanding the core WebRTC APIs for developing your applications.
- Signaling: The process of coordinating communication between browsers.
- Building a simple video chat app: Step-by-step instructions to create your own video chat using WebRTC.
- Advanced features and best practices: Including handling network issues with STUN/TURN servers, ensuring security, and overcoming common challenges.
- Real-world applications: How WebRTC is transforming industries like healthcare, education, and business.
WebRTC makes it incredibly easy to implement real-time communication capabilities, making it a valuable skill for developers in today's interconnected world.
What is WebRTC?
WebRTC stands for Web Real-Time Communication. It's a free project that lets web browsers and mobile apps talk to each other in real-time. This means you can make voice calls, video chats, and share files directly with others without needing any extra software.
Key things about WebRTC include:
- Real-time communication - Lets you share audio, video, and files instantly.
- Embedded media capabilities - You can use your camera and microphone right from your web browser.
- Peer-to-peer connectivity - Directly connects you with others without going through a server.
- Standardized APIs - Offers a set of tools that work the same way in different web browsers.
- Built-in encryption - Keeps your chats and files safe from snooping.
- Interoperability - Works across different devices and web browsers.
- NAT/firewall traversal - Helps connect you with others, even through tricky network setups, using things like ICE, STUN, and TURN.
In simple terms, WebRTC makes it easy to chat or share files directly in your web browser without needing any extra plugins. It's widely used because it's free and works on many devices.
How WebRTC Works
The main parts of WebRTC are:
- MediaStream API - Lets you get video and audio from your camera and microphone.
- RTCPeerConnection - Manages the connection and the flow of audio/video.
- RTCDataChannel - Allows you to share files directly with others.
Here's a basic step-by-step:
- Use
getUserMedia()
to grab video or audio. - Use a signaling server to swap info needed to connect.
- Set up RTCPeerConnection on each end.
- Start streaming audio/video between each other.
This setup lets you talk and share in real-time without needing any extra software. WebRTC handles the hard parts, giving developers a simple way to add real-time communication to apps and websites.
Setting Up the Development Environment
To kick off your journey in building apps that let people talk or share files in real time, you first need to get your computer ready with the right tools and code libraries. This means picking out a good code editor, making sure your web browser is up to date, and grabbing some helpful WebRTC code.
Editor and Browser
First things first, you need a place to write your code and a web browser that can handle WebRTC. Here are some good choices:
- Code Editors: Visual Studio Code, Sublime Text, Atom
- Browsers: Google Chrome, Mozilla Firefox, Opera
It's best to use the most recent version of these browsers to make sure everything runs smoothly.
WebRTC JavaScript Libraries
The main thing you'll need is a library called adapter.js. This helps make sure your WebRTC code works the same way in any browser.
What adapter.js does for you:
- Makes different browsers work together
- Helps older browsers understand WebRTC
- Makes sure video and audio are handled the same way everywhere
To get started with adapter.js:
- Grab it from webrtc.org/adapter.
- Put the script in your HTML file.
- Use it before you start playing with WebRTC in your code.
Other libraries you might find useful include:
- Socket.io - Great for managing connections
- Simple Peer - Makes WebRTC easier to use
- Twilio Programmable Video - Offers more features and helps your app handle more users
Development Tools
Some other tools that can help you out include:
- Web Server - You'll need this to show your project and use the camera/mic. You can use something like Python's HTTP server or Node.js.
- ngrok - This tool gives you a public URL for your local server, which is super useful for testing.
- Chrome DevTools - Helps you find and fix problems in your WebRTC app.
- Wireshark - Lets you take a closer look at your app's network traffic when you're troubleshooting.
With these tools and libraries, you're all set to start creating WebRTC projects. Don't forget to look up guides and documentation online, like on MDN or WebRTC for the Curious, if you need a hand.
WebRTC APIs Deep Dive
MediaStream API
The MediaStream API lets you use the camera and microphone on your device. It's how you get video and audio to show up in a web page or app. Here's what it helps you do:
- Use
getUserMedia()
to grab video or audio from your device. - Choose which camera or mic to use.
- Put cool effects on your video or audio.
- Record videos or sounds and save them.
- Show live video or play sounds for users on a web page.
Basically, the MediaStream API is all about getting and using audio and video in real-time.
RTCPeerConnection API
The RTCPeerConnection API is super important for WebRTC. It lets browsers and apps talk directly to each other, sending audio, video, and data without needing a server in the middle.
Here's what it does:
- Send live video and audio to someone else.
- Share files or data between people.
- Work around internet roadblocks like NATs and firewalls.
- Keep your calls and chats safe with encryption.
You'll use a few key steps to make it work, like setting up the connection and dealing with network stuff. This API is what makes the magic of direct chatting and sharing possible.
RTCDataChannel API
The RTCDataChannel API is all about sending data directly between users, quickly and efficiently.
What it's good for:
- Sending files or messages in either a reliable way or as fast as possible.
- Choosing whether the order of messages matters.
- Sharing big files.
- Letting you know when you can start sending data, or if there's a problem.
You can use RTCDataChannel with RTCPeerConnection to send almost anything you want, like texts, files, or even game moves, making your app feel quick and responsive.
Signaling in WebRTC
Signaling is a crucial part of WebRTC that helps two devices (like your computer and a friend's) talk to each other to start, manage, and end a video call or file sharing. Let's break down what signaling is and why it's important.
What is Signaling and Why It's Needed
Think of signaling as the way devices exchange important info before they can actually connect for a chat or to share files. It's needed because:
- Devices need to share details like where they are on the internet and what video quality they can handle.
- It helps devices connect even if they're on different networks or behind firewalls.
- It's used to start the connection, deal with any issues, and properly end the call or sharing session.
Without signaling, your device wouldn't know how to connect with your friend's device for a WebRTC session.
Signaling Process
Here's a simple step-by-step of how signaling works:
- Your device finds your friend's device and knows where to send its messages.
- Your device asks your friend's device if it wants to connect.
- They share where they are on the internet (like sharing their address).
- They tell each other what video or audio quality they can handle.
- Your device sends a detailed plan (SDP offer) on how it wants to connect.
- Your friend's device checks your plan and sends back its own (SDP answer).
- Once they agree on everything, the video or file sharing starts.
This back-and-forth lets both devices agree on how they'll talk to each other.
Signaling Protocols
WebRTC doesn't tell you exactly how to do signaling. You can pick from several methods like:
- WebSocket
- SIP
- XMPP
- Socket.io
- Custom REST APIs
Your choice depends on what your app needs and what setup you have. Both web and non-web methods work for signaling.
Signaling Server
A signaling server is like a middleman that passes messages between your device and your friend's. It handles:
- Sharing internet addresses and ports
- Exchanging video or audio quality details
- Managing the call or sharing session
With a signaling server, your app can focus on the video or file sharing, while the server takes care of setting everything up.
In short, signaling is super important for getting WebRTC to work. Picking the right signaling method and setting up a server are key steps in making sure your real-time communication app runs smoothly.
Building a Simple Video Chat App
Building a basic video chat app with WebRTC is like putting together a simple puzzle. Let's break down the steps using the main JavaScript APIs:
Get Media from the User's Devices
The starting point is to get access to the user's camera and microphone. This is done with a bit of code that looks like this:
navigator.mediaDevices.getUserMedia({video: true, audio: true})
.then(stream => {
// Now we can use the camera & mic
})
We tell the browser what we need (video and audio) and then deal with the media stream that comes back.
Set Up an RTCPeerConnection
Now, we need to connect two people so they can see and hear each other. This is where RTCPeerConnection comes in:
const pc = new RTCPeerConnection();
pc.ontrack = event => {
// We receive the other person's video stream here
};
pc.addStream(localStream); // This adds our own video stream
This code sets up the connection for video/audio to flow between browsers.
Exchange Signaling Data
For two devices to connect, they need to share some info first. This is often done through a signaling server using something like WebSocket:
socket.on('offer', description => {
pc.setRemoteDescription(description);
// ... then create and send an answer
});
The 'offer' has details about how to connect, which both sides need to share and agree on.
Add UI Controls
Finally, we add some buttons to start and end the call. Here's how it might look in code:
startBtn.onclick = () => {
pc.createOffer()
.then(offer => pc.setLocalDescription(offer))
.then(() => sendOffer(offer));
};
hangUpBtn.onclick = () => pc.close();
These buttons let users start or hang up the call.
And that's it! With these steps, you can create a simple video chat using WebRTC. It's a great way to learn about real-time communication, peer-to-peer connections, and how to add WebRTC to your own projects.
Advanced WebRTC Features
STUN and TURN Servers
When you're using WebRTC for real-time communication, sometimes your device has trouble connecting directly to someone else's because of network barriers. That's where STUN and TURN servers come into play.
STUN servers help your device figure out its public internet address and how to get around barriers like firewalls. Think of it like asking for directions when you're not sure how to get somewhere.
- Your device asks the STUN server for help to find its public address.
- This info lets you connect with others more easily.
- It's like a helper for getting through digital roadblocks.
- Some companies provide this as a service, making it easier for you.
TURN servers step in when STUN isn't enough, acting like a middleman to pass messages back and forth.
- Helps when devices can't talk directly because of strict firewalls.
- Your calls or messages go through this server, which can slow things down a bit.
- It's like a courier service for your data.
- This service usually costs money because it uses a lot of resources.
Using both STUN and TURN makes sure you can connect with others, even when there are obstacles.
Security and Encryption
WebRTC takes your privacy and security seriously, using several smart ways to keep your calls and messages safe:
Encryption ensures that only the person you're talking to can listen in or watch. It's like having a secret code that only you and your friend know.
- Keeps your conversations private.
- Stops others from messing with your call.
Key Exchange is when your device and the one you're connecting with share a secret key at the start of a call, making sure no one else can sneak in.
Integrity Checks are like quality control, making sure the data hasn't been tampered with on its way to you.
Authentication is a way to make sure everyone is who they say they are before starting a call.
Media Traffic Protection means all the data sent is wrapped in a secure layer from start to finish, keeping eavesdroppers out.
With these measures, WebRTC helps make sure your real-time chats and calls are safe from prying eyes and unwanted interference.
sbb-itb-bfaad5b
Common Challenges and Best Practices
Challenge | Best Practice |
---|---|
Browser compatibility issues | Use adapter.js to make sure your code works the same in all browsers. Test your app on different browsers often. |
Dealing with network restrictions | Use STUN/TURN servers to get around network blocks. ICE helps with trying different ways to connect. |
Signaling complexity | Use known protocols like SIP or tools like Socket.io for easier signaling. |
Scaling to large numbers of users | Use SFU architecture, manage connections wisely, and cut down on data use. |
Call quality problems | Pick the right codec, manage your data flow, and adjust settings for better quality. |
Security vulnerabilities | Always use encryption, check your security, and keep WebRTC parts of your app separate. |
Browser Compatibility
Different browsers might handle WebRTC a bit differently. Using adapter.js helps make things more consistent. Still, it's important to check your app on various browsers to catch any issues.
Network Connectivity
Sometimes, networks or firewalls can block the direct connections that WebRTC needs. Using STUN and TURN servers helps get around these blocks, and ICE tries different ways to connect until it finds one that works.
Signaling Complexity
Setting up the initial connection can be tricky. Using existing setups like SIP or tools like Socket.io can simplify this process.
Scaling
When lots of people use your app at the same time, it's crucial to manage connections smartly. This includes using servers to help manage the load and being smart about how much data you're sending.
Call Quality
The quality of your call can depend on many things, like how you're sending the data and how much data you're trying to send. It's important to choose the right settings and adjust based on what's happening on the network.
Security
Keep your calls safe by using encryption and checking your app for security holes. It's also a good idea to keep the parts of your app that use WebRTC separate from other parts.
Real-World Applications
WebRTC is making it easier for people to communicate in real time across many different areas. Let's look at some ways WebRTC is being used today:
Healthcare
- Telemedicine: Doctors can talk to patients through video calls securely, right from a web browser or mobile app, making healthcare more accessible.
- Remote monitoring: WebRTC lets doctors keep an eye on patients from afar, like watching their health signs or checking how they're recovering through video chats.
- Medical education: WebRTC helps with teaching medical professionals remotely, making learning more flexible.
Education
- Virtual classrooms: Schools are using WebRTC for online classes that allow for live video and sharing screens.
- Remote tutoring: WebRTC supports one-on-one tutoring over the internet across different subjects.
- Web-based lectures: Teachers can stream their lectures live to students who are not physically present.
Business & Enterprise
- Unified communications: WebRTC lets teams collaborate in real time through voice, video, chat, and screen sharing, all from a web browser.
- Customer service: Support centers use WebRTC for direct video or voice chats with customers on their websites.
- Sales enablement: Salespeople can show off products through live demos directly from their browser, no extra downloads needed.
IoT & Emerging Tech
- Smart home control: WebRTC cameras enable users to see and control their home environments from anywhere.
- Drone connectivity: WebRTC can create live video links from drones.
- AR/VR apps: WebRTC supports the fast, real-time data connections needed for immersive virtual experiences.
As WebRTC gets built into more web and mobile apps, we'll see even more industries finding creative ways to use real-time communication to change how we work and interact.
Additional Resources
Here are some good places to learn more about WebRTC:
Online Courses and Tutorials
- WebRTC Course on Udemy - A detailed course that teaches you about WebRTC, including how to use it and make apps with it.
- Getting Started with WebRTC on Pluralsight - A beginner-friendly course that covers the basics of WebRTC and how to create simple applications.
- WebRTC Tutorials on Tutorialspoint - Helpful lessons that take you from the basics to more advanced WebRTC topics.
- WebRTC Samples - The official place for code examples to play with and learn from.
Books
- WebRTC Blueprints by Andrii Sergiienko - A hands-on guide with projects to help you get better at using WebRTC.
- Real-Time Communication with WebRTC by Salvatore Loreto and Simon Pietro Romano - A detailed book that covers everything about WebRTC, written by experts.
Communities
- WebRTC Discord - A lively Discord group for people who make stuff with WebRTC.
- WebRTC Reddit - A place to ask questions and talk about WebRTC stuff.
- Slack WebRTC Communities - A list of Slack groups focused on WebRTC.
Other Resources
- webrtc.org - The official WebRTC website by Google Developers.
- WebRTC Experiments - A bunch of free demos showing what you can do with WebRTC.
- w3.org/TR/webrtc - The official guidelines and rules for WebRTC.
These resources are great for anyone wanting to dive deeper into making live chats or sharing files over the web with WebRTC. The courses and tutorials are perfect for getting your hands dirty, while the books and online communities offer more detailed knowledge and a chance to talk with others.
Conclusion
WebRTC is a super cool tool that lets you chat, share files, and do a bunch more right from your web browser. It's built to let you do things in real-time, like video calls or playing games with friends, without needing any extra stuff.
In this guide, we went over the basics of WebRTC, how it works, and some important parts you need to know about. We talked about:
- Core concepts - How it lets you chat and share in real time, works even with tough network settings, and keeps your chats private.
- How it's built - The important parts like MediaStream, RTCPeerConnection, and how devices say 'hi' to each other.
- What you can do with it - Things like video calls, sending files quickly, or checking on patients from far away.
We also showed you how to make a simple video chat to see WebRTC in action. From getting the video and audio from your device, sharing this info, to making the chat window, it's pretty easy to start.
But there's a lot more you can do with WebRTC. You might want to check out:
- Better ways to connect - Using different methods to help devices talk to each other.
- Making calls better - Figuring out how to send video and audio smoothly.
- Handling lots of users - Making sure your app can support many people at once.
- Using data channels for more - Like for games or talking to smart home devices.
- Apps for phones - Making apps that work on phones too.
- Testing your app - Making sure everything works as it should.
With more and more devices supporting WebRTC, the possibilities are huge. Whether you're adding video chat to your website, making a game that works in the browser, or even creating a virtual world, WebRTC gives you the tools to do it.
So go ahead, use your creativity, dive into real-time communication, and start making cool web experiences!
Related Questions
How do I get started with WebRTC?
To begin using WebRTC, follow these steps:
- Use the MediaStream API to grab audio, video, or other data. This is how you access the camera and microphone.
- Use the RTCPeerConnection API to gather network info like IP addresses and ports. Share this info with others to set up connections, even through firewalls.
- Pick a way to talk between browsers, like WebSocket, to manage the connection. This helps with starting, stopping, and managing calls.
With these basics, you can start making apps that let people talk or share stuff in real time.
Is WebRTC TCP or UDP?
WebRTC mainly uses UDP to move data, which is faster but less reliable than TCP used by WebSocket.
This means WebRTC is quicker but might lose some data along the way. However, for most uses, this isn't a big issue.
Which software do we require for WebRTC process?
You'll need:
- JavaScript, HTML, CSS for creating web pages
- WebRTC JavaScript APIs, which are already in your browser
- A modern web browser like Chrome, Firefox, or Edge
- Web server software for hosting your site or app
- A signaling server for managing connections between browsers
No special software is required beyond these basics.
Is WebRTC an API?
Yes, WebRTC is a set of APIs that let you add real-time communication features to browsers and apps.
These APIs include:
- MediaStream API for camera and mic access
- RTCPeerConnection API for streaming between people
- RTCDataChannel API for sending data directly between users
So, WebRTC provides the tools needed to build things like video chats directly in the browser.