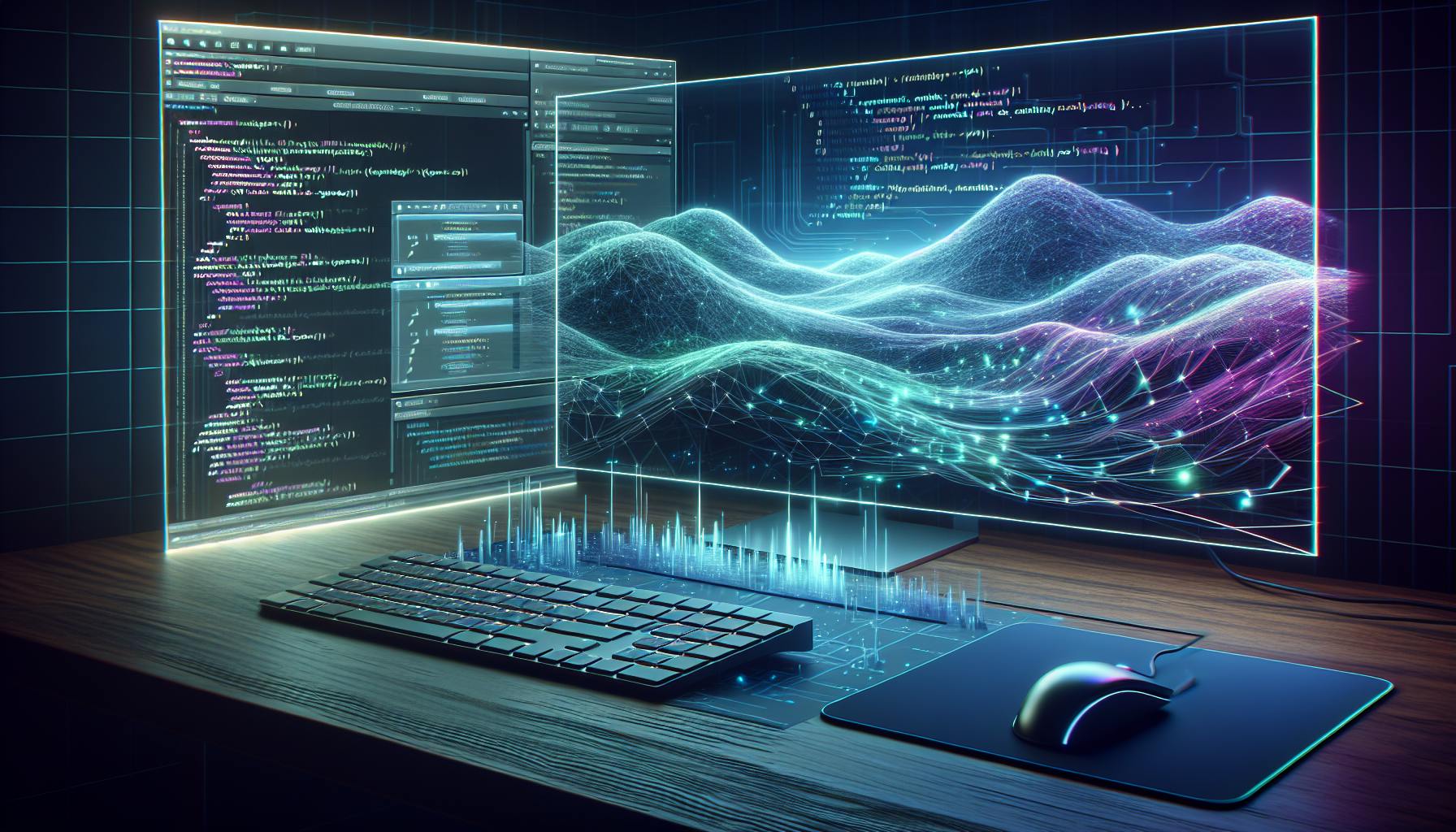
Learn how to effectively comment JavaScript code to improve understanding, maintenance, and collaboration. Explore single-line, multi-line, and JSDoc comments, along with best practices and advanced techniques.
Commenting your JavaScript code is crucial for understanding, maintaining, and collaborating on code projects. Here's a quick guide to do it right:
- Understand why comments matter: They make code easier to understand, facilitate updates and fixes, improve teamwork, and aid in debugging.
- Know the types of comments:
- Single-line comments (
//
) for brief notes. - Multi-line comments (
/* */
) for longer explanations. - JSDoc comments (
/** */
) for detailed documentation. - Choose the right type of comment based on the length and purpose of your note.
- Best practices include:
- Explaining the 'why' more than the 'how'.
- Keeping comments up-to-date with code changes.
- Balancing comment depth, highlighting complexity without overwhelming.
- Advanced techniques like JSDoc can automate documentation, and strategic commenting can assist in debugging.
Remember to explain intentions, keep comments relevant, use consistent conventions, focus on complex parts, and document workarounds and special cases. Efficiently commenting on your JavaScript not only makes your code more comprehensible but also facilitates a smoother development process for both you and your collaborators.
Single-Line Comments
Single-line comments in JavaScript are pretty straightforward. They start with two slashes (//
). Anything you write after these slashes on the same line won't run as code. It's just for you or others to read.
For example:
// This is a single-line comment
let x = 10; // Initialize x to 10
These comments are perfect for quick notes or to stop a line of code from running without deleting it. They're handy for checking your code piece by piece.
Multi-Line Comments
For longer notes that take up more than one line, you use multi-line or block comments. These are surrounded by /* */
. Everything between these markers is a comment, no matter how many lines it covers.
For example:
/* This is a
multi-line comment
block in JavaScript */
Multi-line comments are great for detailed explanations or for turning off big chunks of code at once. They make it easy to comment out many lines without needing a bunch of single-line comments.
JSDoc Comments
JSDoc comments are a bit special. They're used to explain your code in a way that can be turned into a documentation guide. These comments start with /** */
and can include details like what a function does, its parameters, and what it returns.
/**
* Represents a person.
* @constructor
* @param {string} name - The name of the person.
*/
function Person(name) {
this.name = name;
}
JSDoc comments are really useful for making clear, detailed documentation directly from your code. They help others understand the purpose of functions and how to use them.
When to Use Each Type of Comment
Use single-line comments for short notes in your code. They're good for:
- Explaining what a simple piece of code does, like setting up a variable
- Turning off code lines for a bit to test things out
- Dropping quick reminders to yourself
Multi-line or block comments are better for longer talks about your code. Use them to:
- Give the big picture of what a chunk of code is meant to do
- Dive deep into explaining tricky code parts
- Easily stop a bunch of code from running all at once
- Add notes about who wrote the code, copyright stuff, or tasks to do later
JSDoc comments are for when you're getting into the nitty-gritty of functions, classes, and more. Use them to:
- Describe what a function is for and how to use it
- Talk about what goes into a function and what comes out
- Explain what's needed for a class and what it does
- Help make automatic guides from your code
Choosing the right type of comment depends on how much you need to say and why. Single-line comments are great for quick thoughts. Multi-line comments give you space for bigger explanations. And JSDoc comments are all about documenting the details of your code.
Using comments the right way helps everyone understand your code better. It makes it easier for people to work with your code later, including you. Even though clear code often speaks for itself, the right comments can make everything much clearer and easier to work with.
Best Practices for Commenting Code
Explain Intent Over Mechanics
When you add comments to your code, try to talk about the 'why' more than the 'how'. It's better to help others understand why you chose to do something in a certain way. For instance:
// Sort users by last name
// To make it easier to find people in the contacts list
users.sort((a, b) => a.lastName > b.lastName);
This comment tells us why the sorting is important, not just that it's happening.
Keep Comments Up-to-Date
Comments that don't match the code anymore can be really confusing. Make sure to change your comments if you change your code. Get rid of comments that aren't needed anymore to keep things clean.
Balance Comment Depth
You don't want too much or too little in your comments. Just enough detail to help someone understand without overwhelming them. Focus on parts of the code that are tricky or not obvious, and always aim to explain why you did something.
Highlight Complexity
If part of your code is really complicated or handles special cases, make sure to explain it well. This helps anyone reading your code understand those tough parts better. For example:
// Use a default value if we get null
// This fixes crashes because sometimes the API sends back null
if (!value) {
value = DEFAULT_VALUE;
}
By explaining the tricky bits and the reasons behind your decisions, your comments make the code easier for others to work with.
Writing Effective Comments
When you comment your JavaScript code, you're helping make it easier for everyone to understand what's going on. Here are some simple ways to do that well:
Explain the Why, Not Just the What
Your comments should help people understand why you wrote the code the way you did, not just what it does.
// Sort users by last name
// This makes it easier to find people in the contacts list
users.sort((a, b) => a.lastName > b.lastName);
Talking about why you made certain choices helps make your code clearer.
Keep Comments Relevant and Up-To-Date
Make sure your comments still match what your code does. If you change your code, check the comments too and update them if needed.
Use Consistent Commenting Conventions
It helps to comment your code in a similar way each time. This makes it easier for people to quickly understand your notes.
You can use something like JSDoc for a neat, uniform look:
/**
* Represent a person.
* @constructor
* @param {string} name - The name of the person.
*/
Or mark things you need to do later like this:
// TODO: Fix this part later
Comment Complex Parts, Not Obvious Parts
Focus on explaining the tricky parts of your code. If something is simple and clear, you probably don't need to comment it.
// This is a tricky way to check phone numbers
const phoneRegex = /^\d{3}-\d{3}-\d{4}$/;
// Here's an easy function that shows a number
const printNumber = (num = '') => {
console.log(num);
}
Explain Workarounds and Special Cases
If you had to use a clever trick to solve a problem, tell people about it in your comments. This helps everyone understand your code better.
// Sometimes the server messes up and sends back nothing
// We use a backup plan to avoid problems
if (!response) {
response = DEFAULT_RESPONSE;
}
By keeping your comments clear and focused on what matters, you make it easier for others to work with your code. Good comments help everyone understand not just how the code works, but why it's written that way.
sbb-itb-bfaad5b
Advanced Commenting Techniques
JSDoc for Documentation
JSDoc is a powerful tool that helps you create documentation from your JavaScript code comments. It's like leaving detailed notes on your code that can be turned into a guidebook. JSDoc asks you to describe things like what a function does, what each part of the code is for, and what kind of information it gives back.
Why JSDoc is great:
- Consistency - It keeps your comments looking the same, which makes everything easier to understand.
- Clarity - It encourages you to explain important details, making your code easier to follow.
- Auto-Generated Docs - With JSDoc, you can automatically turn your comments into a neat documentation website.
Here's a simple JSDoc example:
/**
* Calculates the area of a circle.
* @param {number} radius - The radius of the circle.
* @returns {number} The area of the circle.
* @throws {TypeError} If the radius isn't a number.
*/
function calculateCircleArea(radius) {
// Here's where the magic happens
}
This example shows how to use JSDoc to explain:
- @param - What you need to give the function.
- @returns - What the function gives back.
- @throws - What could go wrong.
Using JSDoc helps everyone understand your code better and makes creating documentation a breeze.
Commenting for Debugging
Comments are super handy when you're trying to fix bugs in your code. You can use them to turn off parts of your code to see where problems might be hiding.
Here's how comments can help with debugging:
- Turn Off Big Parts - Use a big comment to quickly stop a lot of code from running.
- Find the Problem - Turn off certain parts one by one to figure out where the issue is.
- Keep Things Safe - Comment out new stuff to go back to a version that you know works.
Check out this example:
/*
function loadData() {
// This part is fine...
processData() // We're not running this for now
renderPage()
}
function processData() {
// Oops, found a bug here!
}
*/
By not running processData
, we can see that's where our bug is, without having to erase any code.
Using comments this way can save you a lot of time when you're trying to figure out what's wrong with your code.
Conclusion
Commenting your JavaScript code the right way is super important if you want to keep your code easy to work with, especially when you're part of a team. Here's what you should remember:
- Talk about why, not just how - When you comment, focus more on explaining why you did something instead of just what it does. This gives important background info.
- Make sure comments match the code - Comments should always match what the code is doing. If you change your code, update your comments too to avoid any mix-ups.
- Point out the tricky parts - Use comments to explain parts of your code that might not be obvious or that deal with special situations. This makes it easier for others to get what's going on.
- Be consistent - Try to use the same style of comments throughout your code. This keeps things clear. Tools like JSDoc can help with this.
- Find the right balance - Your comments should have enough detail to be useful but not so much that they're overwhelming. Stick to the important stuff.
- Help with fixing bugs - You can use comments to temporarily stop parts of your code from running. This can help you figure out where a problem is faster.
Following these tips for commenting your JavaScript will help keep your code easy to understand and work with. Good comments that explain why you made certain choices are super helpful for anyone who needs to use or change your code later. They make managing and working on projects much smoother for everyone involved.
Related Questions
What is the best way to comment in JavaScript?
In JavaScript, you can add short notes with //
for simple, one-line comments. This is good for quick explanations or notes right next to your code.
Example:
// This sets up the variable
let x = 10;
For longer notes that need more space, use /* */
to start and end your comment section.
How do you comment code efficiently?
Here are some tips for good commenting:
- Make sure your comments add value and don't just repeat what the code says
- Explain what functions or parts of the code do
- Keep your language simple and clear
- Use correct spelling and punctuation
- Stick to the same style of commenting throughout your code
How to comment out large sections of code in JavaScript?
To disable big parts of your code temporarily, use /* */
around the section you want to ignore:
/*
function processData() {
// code here
}
function renderPage() {
// code here
}
*/
This method is handy for testing without removing any code.
How to code efficiently in JavaScript?
Here are some strategies:
- Use
const
andlet
for variables - Make loops as efficient as possible
- Limit how often you change the webpage directly since it can slow things down
- Avoid things like alerts that stop your code from running
- Be smart about event listeners to avoid unnecessary ones
- Break your code into smaller, reusable pieces
- Use Promises and async/await for tasks that don't happen instantly
Keeping these ideas in mind helps make your JavaScript code better in terms of performance, easy to understand, and reusable.