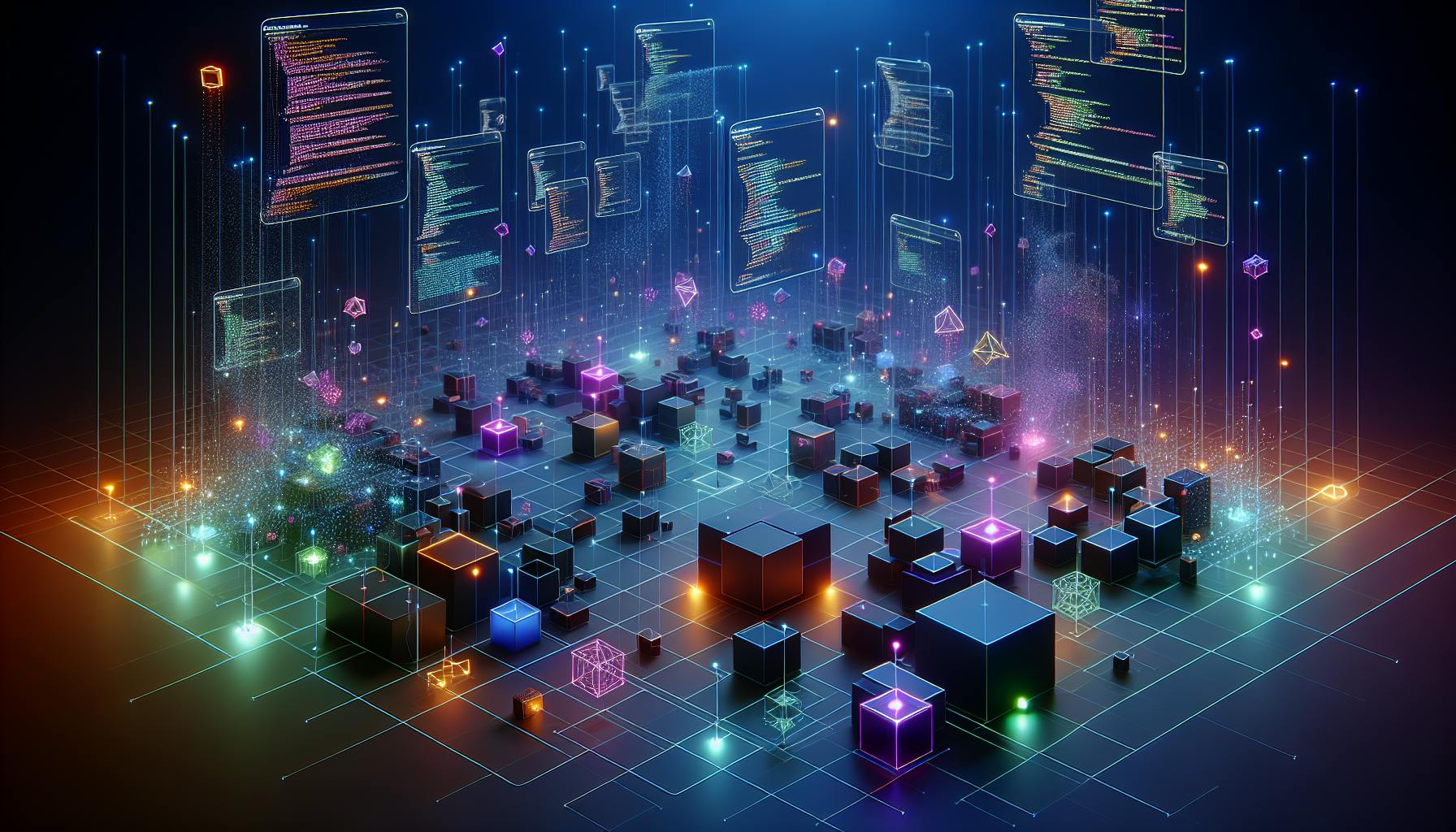
Learn about modular programming, its core principles, advantages, challenges, and how it benefits software development. Explore the anatomy of a module and the future trends in modular programming.
Modular programming is a technique that simplifies complex software development by breaking it down into smaller, manageable pieces called modules. Each module performs a specific task and can be used independently or combined with other modules to build larger systems. This approach offers several benefits such as easier code maintenance, update simplicity, and the reusability of code across projects. Here's a quick overview:
- Modular programming organizes code into distinct sections with specific functions.
- Key principles include high cohesion within modules and loose coupling between them.
- Advantages include easier updates, reusability of code, and efficient teamwork.
- Challenges might involve increased code volume and complexity for smaller projects.
In today's software development landscape, modular programming is increasingly favored for its efficiency and flexibility, paving the way for more scalable and maintainable codebases.
What is Modular Programming?
Modular programming is a way of creating software by breaking it down into smaller, independent pieces called modules. Each module is like a mini-program that handles a specific part of the bigger program.
Here are some key points about modular programming:
- Abstraction - Modules focus on doing a specific job and hide how they do it from the rest of the program. This means you can use a module without knowing the details of how it works.
- Encapsulation - Modules bundle together everything they need to do their job, like data and procedures. This keeps things tidy and under control.
- Information hiding - Modules only show the necessary parts to the outside world, keeping their inner workings private. This helps prevent other parts of the program from relying too much on how a module does its job, which can change.
Modular programming started in the late 1960s to help manage growing and more complex software systems. It's a smart way to organize programs, making them easier to build, test, fix, and reuse. Nowadays, it's a fundamental part of designing and building software.
Core Principles of Modular Programming
The main ideas behind modular programming include:
- High cohesion - Everything inside a module is closely related and works towards the same goal.
- Loose coupling - Modules connect with each other only through their outer layers, so they don't need to know much about each other to work together.
- Abstraction - Modules hide their inner workings, showing only what's needed for them to be used.
- Self-containment - Modules have all they need to function on their own, reducing dependence on outside parts.
Following these principles makes modules easier to handle, test, fix, add to, and reuse. Changes in one module won't mess up others, and it's easier to keep track of where problems might be.
Modular Programming vs Traditional Programming
Modular programming and traditional programming take different approaches to building software. Here's how they compare:
Modular Programming | Traditional Programming |
---|---|
Focus on keeping things related and wrapped up nicely | Often mixes different things together |
Keeps details hidden and shows only what's needed | Less careful about hiding details |
Modules are loosely connected | Parts are tightly intertwined |
Easier to manage dependencies | Dependencies can be a mess |
Allows for working on different parts at the same time | Usually, you work on one thing after another |
Makes it easier to use parts again | Reusing parts can be tricky |
In short, modular programming organizes related functions and data into separate chunks that can be handled more easily. This makes it simpler to build, understand, fix, and improve software, especially when it gets big and complex.
The Anatomy of a Module
Components of a Module
A module is like a little box that contains three main things:
-
Interface - This is the part of the module that talks to the outside world. It's like a list of commands that other parts of the program can use.
-
Implementation - This is the secret sauce inside the module. It's all the code and steps that make the module work but aren't shown to everyone.
-
Data structures - These are like the module's personal notebooks. They hold all the information the module needs to do its tasks. Keeping this info inside the module helps keep things neat and tidy.
These parts work together to let a module do its job well without showing everyone how it's done. The interface is like a door, letting other parts of the program use what they need without letting them see everything inside.
Types of Modules
Modules can be different kinds:
- Program control modules - These are the bosses. They manage how the program starts, ends, deals with mistakes, interacts with users, and makes sure other modules are working together nicely.
- Specific task modules - These are the specialists. They focus on doing one thing really well, like working with numbers, managing text, or talking to devices.
For example, in C programming, you might see:
stdio.h
- Helps with showing and getting informationstring.h
- Makes working with text easiermath.h
- Deals with math problems
In JavaScript, something like TinyMCE breaks tasks into smaller parts, each focusing on things like the look of the program, editing content, or uploading files.
Modules and APIs
Modules can also work together using something called APIs. An API is like a menu that tells you what you can ask the module to do, but doesn't show you how it's done.
This is great because it means the module can promise to do certain things without having to worry about changes inside the module messing things up for everyone else. It's like saying, "You can count on me to do this, no matter what I'm doing behind the scenes."
APIs make it easy to understand what a module can do just by looking at the 'menu'. This helps when you're building or fixing software, making sure everything works well together without any surprises.
Implementing Modular Programming
Choosing the Right Module
When you're breaking down a program into smaller parts, it's key to make sure each part, or module, has just one job. This is called the Single Responsibility Principle. Here’s how to do it right:
- Keep everything in a module related to its main job. This makes it easier to get what the module is about and to change things if needed.
- Make sure modules don’t depend too much on each other. They should talk through well-defined ways, not get tangled up.
- Put all the bits and pieces a module needs to do its job inside it. This keeps things organized.
Keeping modules small, focused, and independent makes the software easier to handle and change later on.
Modular Programming in Different Languages
Different programming languages have their own tools for making software modular:
C
- Lets you use header files (like .h files) to share functions and data structures between files. For example, using
#include <stdio.h>
. - The
static
andextern
keywords help manage who can see and use certain functions or variables.
Java
- Uses
public
andprivate
keywords to control who can access what. - Packages group related stuff together, making it easier to find and use.
- Interfaces let you define a set of actions that classes can perform.
OCaml
- Lets you clearly say what a module will share with the outside world using
sig .. end
. - Functors let you customize modules based on other modules.
- You can nest modules within each other, organizing your program neatly.
These tools help manage what parts of your program can do and use, keeping things tidy and under control.
Modular Design Patterns
Here are some design patterns that help with modular programming:
-
Facade - Makes a simple front for more complex interactions between modules.
-
Adapter - Lets modules that normally wouldn't work together connect through a middleman.
-
Bridge - Keeps the big ideas separate from the nitty-gritty details, so you can change them independently.
Using these patterns can help keep the connections between modules clean and easy to manage.
Advantages and Challenges
Advantages of Modular Programming
Modular programming has a lot of good points:
- Easier to keep up - Since each part does its own thing, updating, fixing, or adding new stuff doesn't mess with the rest. Different developers can work on their own parts at the same time.
- Use parts again - You can take a module you've made and use it in another project. This saves time because you don't have to start from scratch every time.
- Simpler to handle - Splitting a big program into smaller parts makes it less overwhelming. It's easier to figure out what's going on, which helps when you're building or fixing things.
- Work together better - Teams can work on different parts at the same time. This means you can finish big projects faster.
- Neater projects - Putting related stuff together in modules makes your project more organized. This helps when you're dealing with a lot of code.
Modular Programming | Non-Modular Programming |
---|---|
Clear, focused modules | Mixed-up components |
Loose ties between modules | Tight ties between parts |
Modules you can use again | Hard to reuse code |
Easier to update | Harder to keep up |
Lets teams work together | Slower, one-at-a-time work |
Challenges and Disadvantages
But, modular programming isn't perfect. Here are some downsides:
- Might make more code - Splitting things into modules can end up making more code overall, which might slow things down. You'll need to work on keeping the code lean.
- Could get too complicated - For small projects, having lots of modules might make things too complex. Simple updates could become harder.
- Security stuff to think about - If you're not careful about who can access what, having open module interfaces could be risky. You'll need to make sure everything's secure.
Sometimes, sticking everything in one big chunk (monolithic code) might be better, especially for small, simple projects. It can be easier to manage, smaller, and more secure. But for big projects with lots of moving parts and a team behind them, going modular usually makes more sense.
Modular Code | Monolithic Code |
---|---|
Usually more code | Less code |
Extra layers of stuff | Straightforward |
Needs work to keep safe | Easier to lock down |
Too much for simple stuff | Just right for simple things |
sbb-itb-bfaad5b
The Future of Modular Programming
Current Trends and Predictions
Today, more and more developers are using modules because it makes it easier to manage big projects. They can pick and choose ready-made parts to solve specific problems. Here's what we think will happen next:
- More and more tools and pieces of software will be broken down into smaller parts that do one thing really well. This makes it easier for developers to find what they need and use it in their projects.
- The tools we use to keep track of and update these modules will get better, making life easier for developers.
- Big systems might be built like a collection of smaller services that can grow or change independently.
- There will be a trend towards putting together applications quickly using pre-made modules, kind of like snapping together Lego blocks.
Modules are super important for keeping things manageable as we build bigger and more complex software.
Modular Programming in Industry
Big names are getting on board with modular programming. Here are a few examples:
- TinyMCE breaks down the editing process into over 30 specific modules for different tasks. This makes it quick to set up different versions of their editor.
- Amazon designed Polly, their talking text service, with modules so you can change how it speaks easily.
- Spotify uses a similar idea but for their teams, allowing them to work on small parts of the app independently. This helps them make changes faster.
Using modules helps these companies share features across different products and grow without getting bogged down.
The Value for Developers
For developers, knowing how to work with modules can really help your career:
- It means you can use parts that others have made instead of starting from scratch, saving time.
- Understanding how to design with modules makes you better at building systems that are strong and can grow.
- Companies are looking for developers who can work well with modular systems.
Learning about modular programming also teaches you important skills like keeping parts of a program independent, organized, and private. Getting good at this makes you a better developer overall.
Conclusion
Modular programming is a smart way to handle complex software projects. It's like breaking a big project into smaller, easier-to-manage pieces. Each piece, or module, focuses on doing one thing well and works independently. This approach helps teams work on different parts at the same time, makes updates easier, and lets you reuse parts in other projects.
Here's why modular programming is great:
- Easier updates - When you need to change something, you usually only have to mess with one module. This means less chance of accidentally messing up something else.
- Reuse stuff - You can take a module you've already made and use it again in a different project, saving time and effort.
- Teamwork - Since modules work independently, different people or teams can work on their own modules without getting in each other's way.
- Adding new features - When you want to add something new, you can often just plug in a new module.
Looking ahead, modular programming is expected to play a big role in making software. As software gets more complicated, having a library of modules you can pick and choose from will help developers build things faster.
We'll probably see new tools that make working with modules even easier, like:
- Tools that show how modules connect - This will help us understand how changing one module might affect others.
- Ways to swap modules in and out without starting over - This means you can update or replace parts of your software without redoing everything.
- Places to find and share modules - Imagine a library where you can find modules for different tasks and share your own.
The future of building software is likely to focus on combining these modules in smart ways, rather than starting from scratch. This modular approach is key to managing complex software projects.
Related Questions
What is a module in programming?
In simple terms, a module in programming is like a small, independent box that does a specific job. It's a chunk of code that you can use over and over in different projects. Modules keep things neat by hiding how they work inside and just showing what they can do to the outside world. This makes them easy to use again, keeps your project organized, and lets you update parts without messing up everything else.
What is the difference between OOP and modular programming?
OOP (object-oriented programming) and modular programming are two ways of organizing your code. OOP groups data and functions into objects, uses something called inheritance (where objects can inherit features from other objects), and keeps data tightly secured. Modular programming, on the other hand, puts code into modules without using inheritance, and focuses on making these modules reusable in different projects. While both methods aim to keep things organized and reusable, OOP does this with a focus on objects, and modular programming does it with modules.
What is modular vs structured programming?
Both modular and structured programming help break down big programs into smaller parts. The main difference is how they do it. Structured programming uses simple steps and loops to organize code, without much emphasis on hiding or reusing parts of the code. Modular programming goes a step further by wrapping up code and data into modules that can be used again in other projects. This means modular programming is great for making things that are easy to use again and keep organized.
Is Python a modular language?
Yes, Python is definitely a modular language. It has features like import statements to bring in libraries, packages to help organize code, and classes to bundle data and functions together. Python's design encourages using separate modules that work together but don't step on each other's toes, making it easier to manage big projects and reuse code.