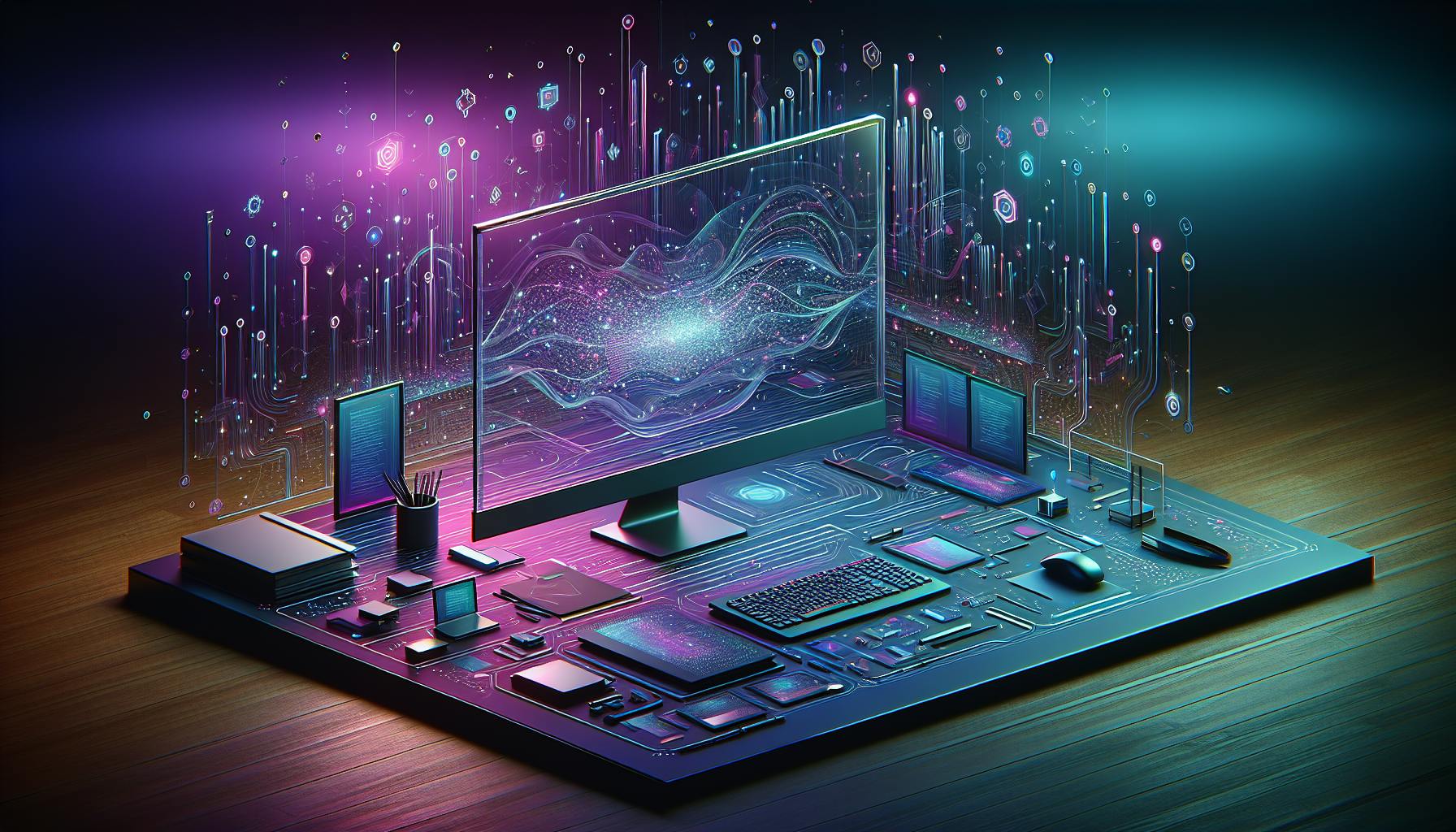
Learn the basics of Apollo GQL, from GraphQL fundamentals to advanced features like subscriptions and Apollo Federation. Discover how to set up Apollo Client and Server for efficient data management in your apps.
If you're curious about Apollo GQL and how it can make managing data in your apps easier, you've come to the right place. At its core, Apollo GQL leverages GraphQL to help you ask for exactly what you need - no more, no less - making your apps faster and more efficient. Here's a quick rundown of what you'll learn:
- GraphQL Basics: Understand why GraphQL is a game-changer for fetching data compared to traditional REST APIs.
- Apollo Client and Server: Discover how these tools simplify data management, from fetching and updating data to caching and real-time updates.
- Setting Up Apollo: Step-by-step guidance on getting Apollo Client and Server up and running in your project.
- Advanced Features: Explore subscriptions for real-time updates, Apollo Federation for building a unified API from multiple services, and Apollo Studio for monitoring your GraphQL usage.
- Best Practices: Tips on performance optimization, caching strategies, and error handling to make your Apollo applications robust and efficient.
This guide is designed to provide a comprehensive overview of Apollo GQL, from the basics for beginners to more advanced features for experienced developers. Whether you're looking to integrate Apollo into your next project or aiming to achieve the Apollo Graph Developer - Associate Certification, you'll find valuable insights and practical tips to help you along the way.
What is GraphQL?
GraphQL is a special way to ask for data from a server, kind of like making a specific order at a restaurant instead of getting a pre-set meal. It was made by Facebook to make it easier to get exactly the data you need from the internet without extra stuff you don't want.
Some important points about GraphQL:
- It's different from the old way of getting data, which is called REST APIs
- You can ask for just the parts of the data you need, which means no more getting too much or too little
- It uses a special plan or schema to understand the data structure
- It's great for apps that need data in a smart and efficient way
The best parts about using GraphQL instead of REST include:
- Getting just the right amount of data
- Being able to get complex, connected data with one ask
- Moving away from fixed ways to get data
- Automatically knowing what data you can ask for because of schemas
- Using one way to ask for all your data needs
Overall, GraphQL makes getting data for apps simpler and more direct.
How GraphQL Works
GraphQL uses a server that shows a plan or schema of all the data you can get and how to get it. Here's what's important to know:
- The schema is like an agreement between the client (like your app) and the server
- You can ask for specific pieces of data
- The GraphQL server checks your ask to make sure it's okay and then gets you your data
Here's how it usually goes:
- Your app sends a list of data it wants to the GraphQL server
- The server checks this list against its schema
- The server gets the data from its databases or services
- The server sends back the data in a format your app can use
So, GraphQL is like a middleman that lets your app ask for exactly what it needs, making things more efficient.
Benefits of GraphQL
Here are some main benefits of using GraphQL:
Get Just What You Need:
- No more getting too much or too little data
- You decide exactly what data you want
- One ask can get you lots of connected data
Keep Things Running Smoothly:
- Since there's a plan, your app won't break when things change
- Only wrong asks cause issues
Easier for Developers:
- It's simpler to get data from one place
- Everything's clear and documented
- Tools like GraphiQL make it even easier
Works Well for the Backend:
- You can mix different data sources
- You can add special logic into how data is given out
In short, GraphQL fixes a lot of headaches when it comes to getting data for apps. It gives you control and flexibility, making everything faster and easier for developers.
Introduction to Apollo GraphQL
Apollo GraphQL is a set of tools that helps developers make and manage applications more effectively using GraphQL. It's like a toolkit for dealing with data in your apps. Apollo GraphQL has three main parts:
Apollo GraphQL Components
Apollo Client is a tool for handling data in your app. It helps you get data from a server, keep it in your app for quick access, and make sure your app's display updates when the data changes.
Apollo Server is a tool for setting up a GraphQL server with Node.js. It's designed to make it easy for you to create and run your own GraphQL APIs. It takes care of the heavy lifting like creating schemas, setting up resolvers, and keeping an eye on performance.
Apollo Federation lets you combine multiple GraphQL services into one big service. This is useful if you're moving from a single large application to smaller services or if you want to bring together different APIs but still work with them as a single unit.
These tools work together to help you manage data more efficiently in your apps. They make it easier to get data, update it, and keep everything in sync. Plus, with extra tools like Apollo Studio, you can check on and improve the performance of your GraphQL services.
In using Apollo GraphQL, you'll often find yourself building a schema, connecting to data sources, writing query and mutation resolvers, and maybe even connecting your services to Apollo Studio. If you're just getting started, you might want to set up Apollo Client or Apollo Server, and there's plenty of help available from the Apollo community, as well as tools like Node.js, npm, git, and VS Code to support your work.
Setting Up Your Apollo Environment
Prerequisites
Before diving into Apollo GraphQL, let's make sure you have everything you need:
- Node.js and npm - Apollo needs Node.js to work. Think of Node.js like the engine for running JavaScript not just on websites but on your computer, too. npm is a tool that comes with Node.js to help you install other tools and libraries like Apollo. You can download both from nodejs.org.
- JavaScript knowledge - Since Apollo is based on JavaScript, knowing how to code in JavaScript is really helpful. This way, you can easily work with both Apollo Client and Server.
- Understanding GraphQL - To really get the hang of Apollo, you should know the basics of GraphQL, like what schemas and queries are. If you're new to GraphQL, there are plenty of free resources to help you learn.
- Editor - You'll need a code editor, basically a program where you can write and edit your code. VS Code is a good choice and it works well for JavaScript and Node.js projects.
Installing Apollo Client
To start using Apollo Client, you can add it to your project.
Here's how to add it if you're starting from scratch:
npm install @apollo/client
If you're working on a React app, you'll want to install it like this:
npm install @apollo/client react-apollo graphql
Or, if you prefer Yarn:
yarn add @apollo/client react-apollo graphql
After installing, you can bring Apollo Client into your app's code and get it ready to use.
Installing Apollo Server
For Apollo Server, you can set it up in your project like this:
npm install apollo-server graphql
Or with Yarn:
yarn add apollo-server graphql
Once it's installed, you can start setting up Apollo Server by creating typeDefs and resolvers, which are basically the rules and processes for how your server will handle data requests.
This is just the beginning of setting up your environment for Apollo Client and Server. Next, you can move on to connecting to data sources, building your schema, and writing query and mutation resolvers. It's all about preparing to manage and use data effectively in your apps with Apollo and GraphQL!
Apollo Client Deep Dive
Fetching Data
Apollo Client makes it easy to get data from a GraphQL API. Here's what you need to know about getting data:
- You write queries with the
gql
tag from@apollo/client
. This looks a lot like the queries you send to a GraphQL server. - The
useQuery
React hook runs a query and takes care of things like loading state, errors, and more.
const {data, loading, error} = useQuery(QUERY);
- While your query is running,
loading
will betrue
. When the data comes back,data
will have your info andloading
will change tofalse
. - If something goes wrong, the
error
part will tell you what happened.
In short, Apollo Client handles sending your queries, tracking if they're still loading or if there was an error, and then gives you the data.
Managing Local State
Apollo Client has tools for keeping track of data right in your app:
- Reactive variables let you keep data that updates automatically. If the data changes, your app will know and update too.
const [todos, setTodos] = useReactiveVar(todosVar);
- You can also use InMemoryCache to directly read and write data without sending a query.
client.cache.readQuery({ query });
client.cache.writeQuery({ query, data });
Apollo Client gives you ways to manage your app's data and update your UI without always needing to talk to your GraphQL API.
Caching
One of the best things about Apollo Client is how it handles caching:
- It uses normalization to save query results by id, which helps merge data efficiently.
- Cache directives like
@client
help you decide what data stays local and what needs fetching. - Policies set rules for how to read and write to the cache, like what to do when data is missing.
With Apollo Client, you get fast access to data stored in memory, which makes your app quicker. Plus, it keeps the data in sync with the server automatically, so you don't have to worry about it.
Building GraphQL APIs with Apollo Server
Setting Up Apollo Server
To start with Apollo Server, you'll need to install it. Use npm like this:
npm install apollo-server graphql
After that, you can set up your server with this bit of code:
const { ApolloServer } = require('apollo-server');
const server = new ApolloServer({
typeDefs,
resolvers,
});
server.listen().then(({ url }) => {
console.log(`๐ Server ready at ${url}`)
});
This code sets up a new server that knows your GraphQL layout and how to find data.
To make your server smart about where to get its data, like from a database or another service, you add a data sources part:
const server = new ApolloServer({
typeDefs,
resolvers,
dataSources: () => {
return {
booksAPI: new BooksAPI(),
};
}
});
Now your server can use these sources to grab data.
Defining Schemas
In Apollo Server, you use a special language to describe your data layout:
type Author {
id: Int!
name: String!
books: [Book!]!
}
type Book {
id: Int!
title: String!
author: Author!
}
Here's what you need to know about this setup:
type
tells you what kind of data you're working with- Each piece of data has a name and a type, like
id: Int!
- The
!
means that piece of data must be there [Book!]!
means a list of Book types
This setup tells Apollo Server what your data looks like.
Writing Resolvers
Resolvers are like helpers that find the data for your schema. Here's how you might write them:
const resolvers = {
Query: {
books: (parent, args, context) => {
return context.dataSources.booksAPI.getBooks();
}
},
Book: {
author: (parent, args, context) => {
return context.dataSources.booksAPI.getAuthorById(parent.authorId);
}
}
}
- Parent has info from the last helper
- Args holds the details for the query
- Context lets you access your data sources
Resolvers can also handle mutations, which are ways to change your data.
sbb-itb-bfaad5b
Advanced Apollo GraphQL Features
Real-time Updates with Subscriptions
Subscriptions are a way to get updates from the server to your app as they happen, without having to ask over and over again. Here's the simple idea behind it:
- You tell the server what info you're interested in by sending a subscription query. Then, you wait.
- Whenever that info changes or gets added on the server, it sends the update right to your app.
- Your app gets this new info and can change what's on the screen to match, all without needing to ask again.
For example, you might use a subscription to get new messages in a chat app as soon as they're sent.
Subscriptions are great for things that need to stay up-to-date all the time, like chats, live updates for products, or data on a dashboard. They make your app feel faster and more alive because it always shows the latest info.
Apollo Federation
Apollo Federation is a way to make several smaller GraphQL services work together as if they were one big service. It's like building a team where each member does a specific job, but together, they seem like one person doing everything.
Here's why it's cool:
- Teams can work independently - Different groups can take care of their parts of the app.
- You can move slowly - There's no rush to change everything at once. Start with one part and go from there.
- Things stay organized - You keep the rules for each part of your app with the code that it's related to.
It uses a main gateway to connect data from all these smaller services into one big, easy-to-use API. This helps big companies use GraphQL in a way that's easier to manage.
Apollo Studio
Apollo Studio is a tool that helps you keep an eye on how your GraphQL operations are doing. It offers:
- A place to keep all your schema definitions - Think of it as a library for your GraphQL blueprints.
- A way to see how fast your queries are working - It tells you how well your queries are doing so you can make them better.
- A log of errors - If something goes wrong, it'll tell you, so you can fix it.
- A check for changes - Before you make your app live with new changes, it helps you make sure nothing will break.
Connecting your Apollo Server to Apollo Studio gives you a clear view of how your GraphQL is being used. This can help you deliver data more efficiently and keep your app running smoothly.
Best Practices
Performance
To make Apollo GraphQL work faster and better, follow these tips:
- Batch queries - Group several queries into one to cut down on network use. Apollo Client does this for you most of the time.
- Persisted queries - Keep your query text on the server so you don't have to send the whole query every time. This makes requests smaller.
- CDN caching - Use a CDN to store full query results. This lets you get fast responses straight from the cache.
- Field-level caching - Set up your cache to save specific fields rather than entire queries for more detailed control.
- Pagination - Break your data into smaller parts that can be loaded bit by bit to avoid loading everything at once.
- Async resolvers - If some data takes longer to get, make those resolvers async so they don't hold up other data.
Caching
For better caching with Apollo Client, try these:
- Normalization - By saving data with an id field, Apollo can combine data from different queries easily.
- Field policies - Create specific rules for how to read and write cache data for each type or field.
- Eviction policies - Make rules for when to remove data from the cache that's not being used.
- Split queries - Keep data that can be reused in separate queries to improve caching.
- Optimistic UI updates - Change the UI right away when you make updates and go back if there's a problem.
Error Handling
To deal with errors well in Apollo GraphQL:
-
Require error handling - Make sure error handling is a must by using
require('graphql-tag/require-error-handling')
. - Handle errors globally - Set up an error policy to manage errors in one place.
- Log errors - Use services like Sentry to keep track of errors so you can find and fix common problems.
- Mask errors - Don't show detailed server errors to clients; give them a general message instead.
- Retry on failure - Set up failed requests to try again automatically.
- Error codes - Use standard error codes so you can handle specific errors in certain ways.
Building an Apollo Client and Server Example App
Server Setup
First, let's get your Apollo Server ready. You'll need to install some things:
npm install apollo-server graphql
Next, set up your server by creating a new ApolloServer instance. You'll need to tell it about your typeDefs
(which is like the blueprint of your data) and resolvers
(which are the instructions on how to get the data):
const server = new ApolloServer({
typeDefs,
resolvers
});
server.listen();
If your server needs to get data from somewhere, like a database or another website, you can connect it using the dataSources
property:
const server = new ApolloServer({
typeDefs,
resolvers,
dataSources: () => {
return {
booksAPI: new BooksAPI()
};
}
});
This way, your server knows where to look for data.
Client Setup
To start using Apollo Client in a React app, you need to add some packages:
npm install @apollo/client graphql
Then, you need to wrap your app with an ApolloProvider
to make the Apollo Client available everywhere in your app:
import { ApolloClient, InMemoryCache, ApolloProvider } from '@apollo/client';
const client = new ApolloClient({
cache: new InMemoryCache()
});
ReactDOM.render(
<ApolloProvider client={client}>
<App />
</ApolloProvider>,
document.getElementById('root')
);
Fetching Data
You can grab data in your components using the useQuery
hook. Here's how you can get a list of books:
import { useQuery, gql } from '@apollo/client';
const GET_BOOKS = gql`
query GetBooks {
books {
title
author
}
}
`;
function BookList() {
const { loading, error, data } = useQuery(GET_BOOKS);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error!</p>;
return data.books.map(book => (
<div key={book.title}>
<p>{book.title}</p>
<p>{book.author}</p>
</div>
));
}
This code asks for books and shows them on the page.
Adding Mutations
If you want to add or change data, you use the useMutation
hook. Here's an example of adding a book:
const ADD_BOOK = gql`
mutation AddBook($title: String!, $author: String!) {
addBook(title: $title, author: $author) {
id
}
}
`;
function AddBook() {
const [addBook, { data, loading, error }] = useMutation(ADD_BOOK);
const handleSubmit = async (e) => {
e.preventDefault();
await addBook({
variables: {
title: formData.title,
author: formData.author
}
});
};
// render component
}
This lets you call the mutation to add a book and handle the response right in your component.
Conclusion
Apollo GraphQL is like a toolbox for making apps with GraphQL. It helps with storing data, managing what you see on your screen, and talking to the internet, so you can focus on making your app special.
There's a big group of people using Apollo who are ready to help beginners and those looking to get better. You can find everything you need to know in Apollo's guides, from the basics to tips for using it in big projects. There are also places like GitHub where you can ask questions and get answers.
Apollo makes it easier to work with data and keep your app looking fresh. With GraphQL, you can ask for exactly what you need, making Apollo a top pick for apps that are ready to go live.
Whether you're just starting with Apollo Client or Apollo Server, or aiming for something like the Apollo Graph Developer - Associate Certification, Apollo has what you need to make impactful apps. We suggest using Apollo Client and Server for your next project.