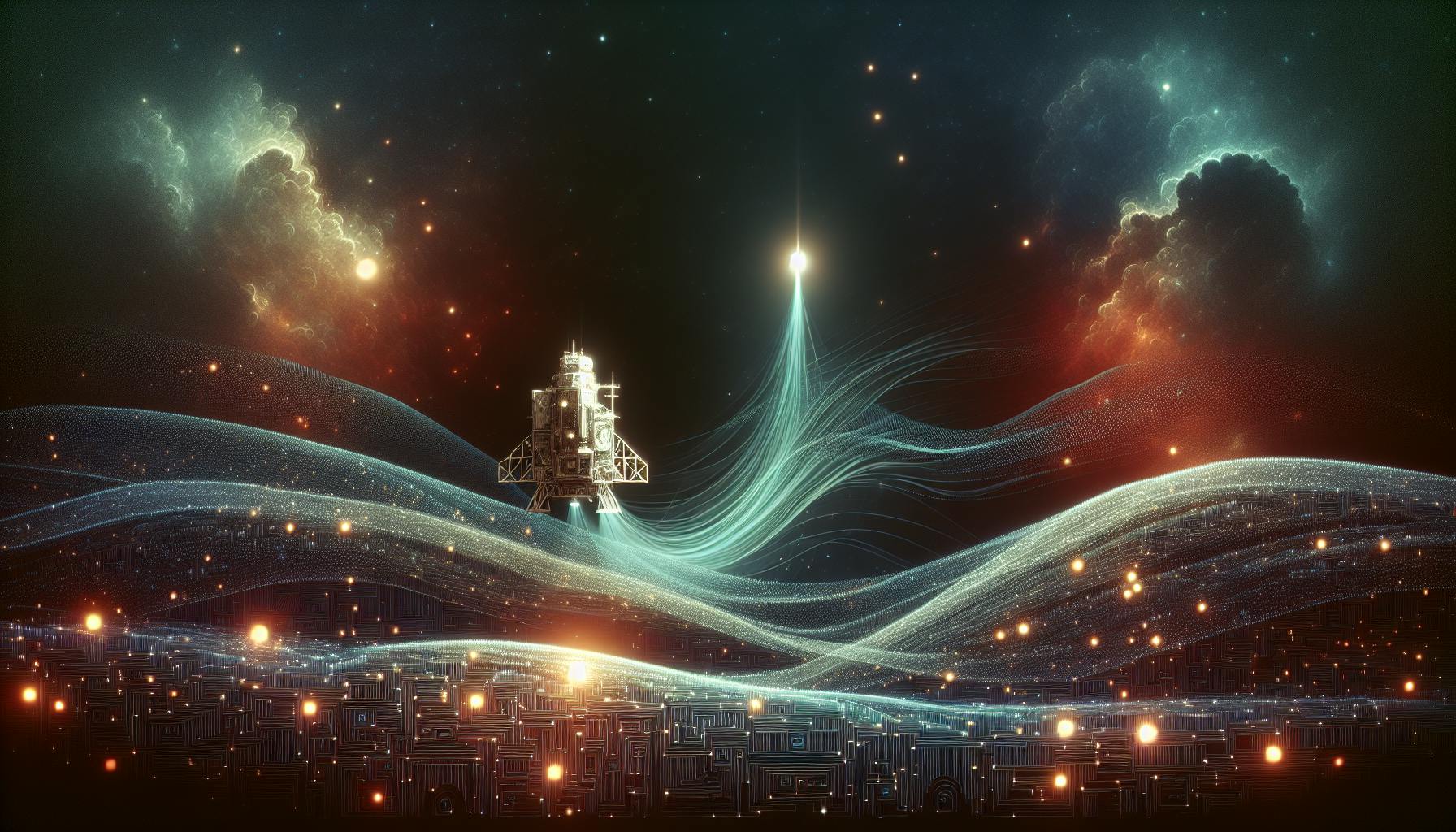
Learn the basics of GraphQL and Apollo Client for web and mobile apps. Explore queries, mutations, subscriptions, schemas, error handling, and performance optimization.
Starting your journey with GraphQL and Apollo Client? Here's a straightforward guide to kick off your project. We'll explore:
- What GraphQL is and its advantages over traditional REST APIs.
- Setting Up Apollo Server: The basics of getting a GraphQL server up and running.
- Key Concepts: Understanding queries, mutations, and subscriptions in GraphQL.
- Apollo Client with React: How to integrate Apollo Client into your React application for fetching and managing data.
- Executing Queries: Learn how to use Apollo Client to make queries to a GraphQL API.
- Handling Local Data and Caching: Tips for managing local data and optimizing application performance with caching.
- Advanced Queries and Mutations: Dive into more complex GraphQL queries with variables, pagination, and nested objects.
- Real-time Updates with Subscriptions: How to set up and use subscriptions for live data updates.
- Error Handling and Debugging: Strategies for efficiently spotting and fixing issues.
- Optimizing Performance: Techniques to make your app faster and more responsive.
- Next Steps: Advanced Apollo Client features, performance optimization, authentication, and real-world practice.
We also compare Apollo Client with other GraphQL clients like Relay and urql, highlighting their features, community, and learning curve to help you choose the right tool for your project.
This guide aims to equip you with the knowledge to confidently start building applications using Apollo Client and GraphQL, enhancing your data fetching and state management processes in web and mobile apps.
Queries
Think of queries in GraphQL like making a specific shopping list. You tell the server exactly what info you want, and it gives you just that. This is great because:
- You only get the info you need, saving time and data
- You don't have to ask multiple times for related info
- The answers you get back are predictable
For instance, if you ask for a user's name and email like this:
{
user(id: 5) {
name
email
}
}
You'll get exactly that:
{
"user": {
"name": "John Doe",
"email": "john@example.com"
}
}
Mutations
While queries are for getting data, mutations are for changing it. Think of them like updating your profile or posting a picture online. You can:
- Add new info
- Change existing info
- Remove info
Here's a quick example:
mutation {
updateUser(id: 5, email: "john@newexample.com") {
name
email
}
}
This lets you update info while deciding what you want to know after the change, just like with queries.
Subscriptions
Subscriptions are about getting live updates. When something changes on the server, you get notified right away. It's perfect for things like getting a message or seeing a new post.
Schemas
Schemas are like blueprints for your data. They show:
- What kind of data is available
- How data is connected
- What you can ask for with queries, mutations, and subscriptions
They're great because they make everything clear and organized.
Advantages over REST
Here's why GraphQL can be better than REST:
- One place to ask for everything
- Gets data more efficiently
- Has a clear set of rules for data
- Explains itself, so you know what you can ask for
- A common standard that makes it easier to use
For a lot of modern websites and apps, GraphQL makes getting and updating data simpler and faster, which makes everyone's life a bit easier.
Getting Started with Apollo Client
Setting Up Your Environment
To start using Apollo Client, you need to prepare your project. If you're working on a new project, you can quickly set up a React app like this:
npx create-react-app my-app
cd my-app
This command creates a new React project with everything you need.
Or, if you prefer, you can start a project on your own or use an online tool like CodeSandbox to jump right in.
Make sure you have these ready:
- Node.js and npm on your computer
- A
package.json
file for managing your project's parts - React (or another framework like Vue or Angular) for making the user interface
After setting up your project, you're ready to add Apollo Client.
Installing Dependencies
Apollo Client comes in pieces you add to your project with npm, a tool for adding and managing those pieces. You mainly need two things:
@apollo/client
- This is the main part of Apollo Client.graphql
- This helps read and understand GraphQL queries.
You can add them with this command:
npm install @apollo/client graphql
And that's it! You've now got Apollo Client in your project, ready to talk to a GraphQL API and manage your data fetching and updates.
Initializing Apollo Client
To start using Apollo Client, we need to bring in some bits and pieces from the @apollo/client
package:
import {
ApolloClient,
InMemoryCache,
gql
} from '@apollo/client';
This code grabs:
ApolloClient
- This is the main tool we'll use from Apollo.InMemoryCache
- A way to store data so we can use it again without asking for it from the server every time.gql
- A helper that lets us write our data requests in GraphQL language.
Next, we set up ApolloClient
by telling it where to send these requests and how to store data:
const client = new ApolloClient({
uri: 'https://api.example.com/graphql',
cache: new InMemoryCache()
});
Here, we:
- Point it to the
uri
where our GraphQL server lives. - Use
InMemoryCache
to keep our data handy. - Give these details to
ApolloClient
so it knows what to do.
Now, client
is all set to ask for and change data on our behalf.
For instance, to ask for a user's info, we can do something like this:
client.query({
query: gql`
query GetUser {
user(id: 5) {
name
email
}
}
`
}).then(result => console.log(result));
We write our request in GraphQL (thanks to gql
), and when we get the answer, we just show it.
ApolloClient
helps us by:
- Keeping data in a cache so our app runs faster.
- Handling when data is loading or if there's an error.
- Making sure our app's display is always up to date.
- Working well with React and other modern tools for building apps.
Apollo Client makes it easier to work with data in our web and mobile apps. It helps us get and manage data without a lot of hassle, and fits right in with the tools we're already using.
Now that we've got Apollo Client ready, let's see how we can connect it to our React app and start showing data!
Connecting Apollo Client to React
To make Apollo Client work with your React app, you use something called ApolloProvider
. Think of ApolloProvider
as a big hug around your React app. It shares Apollo Client with every part of your app, kind of like how a parent shares snacks with kids in every corner of the house.
Here's a simple way to do it in your index.js
file:
import React from 'react';
import ReactDOM from 'react-dom';
import { ApolloClient, InMemoryCache, ApolloProvider } from '@apollo/client';
const client = new ApolloClient({
uri: 'https://api.example.com/graphql',
cache: new InMemoryCache()
});
ReactDOM.render(
<ApolloProvider client={client}>
<App />
</ApolloProvider>,
document.getElementById('root')
);
Now, any part of your app can talk to Apollo Client without any hassle. For instance, to ask for some data:
import { useQuery } from '@apollo/client';
function MyComponent() {
const { loading, error, data } = useQuery(QUERY);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error :(</p>;
return <div>{data.user.name}</div>;
}
What ApolloProvider
does for us is pretty cool:
- It makes sure every part of our app can easily use Apollo Client.
- It keeps an eye on our data requests and the cache.
- It deals with the waiting times and mistakes for us.
- It updates our app when new data comes in.
By wrapping our app with ApolloProvider
, we make sure we can get and show data anywhere in our app without jumping through hoops. It's like giving your app a superpower to talk to the data server with minimal fuss.
In short, ApolloProvider
is the key to making everything work smoothly in React with Apollo Client. With just a little setup, your whole app can easily grab data from GraphQL, making things a lot simpler.
Executing Your First Query
To get data in a React component using Apollo Client, we use something called the useQuery
hook. Let's go through how to do it step by step:
- First, we need to tell Apollo Client what data we want. We do this by writing a query in a special way using GraphQL. We use a helper called
gql
to make our query look right:
const GET_USER = gql`
query GetUser {
user(id: 5) {
name
email
}
}
`;
- Then, let's make a React component that uses this query:
function DisplayUser() {
const { loading, error, data } = useQuery(GET_USER);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error! {error.message}</p>;
return (
<div>{data.user.name}</div>
);
}
Here's the deal:
- We use
useQuery
and give it ourGET_USER
query. useQuery
gives us backloading
,error
, anddata
which we use to decide what to show.- If we're waiting for data, we show a loading message. If something goes wrong, we show an error message.
- Once we have the data, we show the user's details.
- Now, let's put
DisplayUser
in our app so it can do its thing:
function App() {
return (
<div>
<h2>My App</h2>
<DisplayUser />
</div>
);
}
And that's it! Now DisplayUser
will run the query and take care of showing the data in our app.
Here are the main points to remember:
- We write queries using
gql
- We use
useQuery
with our query - We prepare our UI to show loading messages, errors, and finally, the data
- We use the data to show something useful
This is how you fetch and display data with Apollo Client in React. You can now start creating UIs based on different queries using this method!
Advanced Queries and Mutations
GraphQL lets you do more than just simple requests for data. You can make your data requests flexible and reusable with things like variables, special conditions, and reusable parts.
Query Variables
You can make your queries flexible by using variables. Here's an example:
query GetUser($id: ID!) {
user(id: $id) {
name
}
}
You can then provide the value for $id
separately, like this:
{
id: 5
}
This approach helps you avoid repeating yourself and use the same query in different situations.
Pagination
For lists of data, you can control which part of the list you see with skip
and first
:
query GetUsers($first: Int, $skip: Int) {
users(first: $first, skip: $skip) {
name
}
}
Changing $first
and $skip
lets you see different pages of data.
Nested Objects
You can ask for data inside other data like this:
{
user {
name
address {
line1
city
}
}
}
This way, you get all the related data you need in one go.
List Fields
For lists, you can directly ask for specific details about each item:
{
user {
friends {
name
}
}
}
Performing Mutations
Mutations let you change data on the server:
mutation UpdateUser($id: ID!, $email: String) {
updateUser(id: $id, email: $email) {
id
email
}
}
You just need to provide the values for $id
and $email
when you run the mutation.
Directives
Directives like @include(if: Boolean)
can change how your query works based on conditions:
query GetUser($fetchFriends: Boolean!) {
user {
name
friends @include(if: $fetchFriends) {
name
}
}
}
In this case, friends
will only be included if $fetchFriends
is true
.
Fragments
Fragments let you reuse parts of your queries:
fragment UserDetails on User {
id
name
email
}
query GetUser($id: ID!) {
user(id: $id) {
...UserDetails
}
}
This way, you can use UserDetails
in many queries without rewriting it.
By using these advanced features of GraphQL, you can make your data requests more powerful and easier to manage.
sbb-itb-bfaad5b
Real-time Updates with Subscriptions
Subscriptions in GraphQL are like signing up to get the latest news about something. They let you know right away when something changes or gets updated on the server. This is super handy for chat apps, live updates, or when you want to be notified about something immediately.
Here's a simple way to use subscriptions with Apollo Client:
1. Set up subscriptions on the server
First off, you need a server that can handle subscriptions, such as Apollo Server. On this server, you'll set up special functions called subscription resolvers. These functions are in charge of sending out updates.
For example:
const NEW_MESSAGE = 'NEW_MESSAGE';
const resolvers = {
Subscription: {
newMessage: {
subscribe: () => pubsub.asyncIterator(NEW_MESSAGE)
}
}
}
This code means that any new message will be sent out to anyone who wants to know about it.
2. Subscribe in your Apollo Client
On the client side, you can use something called useSubscription
to listen for these updates:
const { data } = useSubscription(NEW_MESSAGE);
if(data) {
showNewMessage(data.newMessage)
}
Whenever a new message comes in, you'll know about it right away and can show it in your app.
This approach works for lots of different real-time features like:
- Chat or messaging
- Notifications
- Showing who's online
- Live forms
3. Publish events
Back on the server, whenever you want to send out an update, you do something like this:
pubsub.publish(NEW_MESSAGE, {newMessage: 'Hello!'});
This sends the message out to everyone who's listening.
Using subscriptions, your apps can instantly update based on what's happening on the server. It's like having a direct line to the server that keeps you in the loop in real-time.
Error Handling and Debugging
When you're building apps with Apollo Client, it's important to know how to deal with mistakes and figure out problems. Here are some tips to help you out:
Enable Error Logging
To keep an eye on errors, you can set up Apollo Client to show them in your browser's console:
const client = new ApolloClient({
uri: 'https://api.example.com/graphql',
cache: new InMemoryCache(),
onError: ({ graphQLErrors, networkError }) => {
if (graphQLErrors)
graphQLErrors.forEach(({ message, locations, path }) =>
console.log(
`[GraphQL error]: Message: ${message}, Location: ${locations}, Path: ${path}`,
),
);
if (networkError) console.log(`[Network error]: ${networkError}`);
}
});
This step makes it easier to spot and fix errors.
Handle Loading and Error States
When using useQuery
, you can deal with waiting times and errors like this:
function MyComponent() {
const { loading, error, data } = useQuery(GET_USERS);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error :( Please try again</p>;
return <UserList data={data} />;
}
This way, you let people know if the app is still loading data or if something went wrong.
Inspect Cache Content
To check what's in your data cache, use:
client.cache.inspect();
This can help you figure out issues with old or incorrect data being stored.
Enable Debugging Mode
Turn on debug mode for more detailed info:
const client = new ApolloClient({
cache: new InMemoryCache(),
uri: 'https://api.example.com/graphql',
debug: true,
});
This will show you extra details in the console to help with troubleshooting.
By following these tips, you can better manage errors and debug your Apollo Client apps. Turning on error logging and debugging, showing messages when loading or errors happen, checking the cache, and using debug mode are all good practices for building strong apps.
Optimizing Performance
Making your Apollo Client app run faster is key to keeping users happy. Hereโs how you can make things snappier:
Enable Query Caching
Apollo Client automatically saves data from queries. But you can tweak how it does this for even better results:
const client = new ApolloClient({
cache: new InMemoryCache({
typePolicies: {
Query: {
fields: {
users: {
// Cache for 10 seconds
maxAge: 10,
},
},
},
},
}),
});
This means the users
query won't ask the server for new data for 10 seconds.
Use Field Policies
You can tell Apollo how to handle caching for specific bits of data:
new InMemoryCache({
typePolicies: {
User: {
fields: {
// Don't cache this field
friends: {
read() {
return undefined;
}
}
}
}
}
})
Here, Apollo wonโt save the friends
data, so it always gets the latest info.
Batch GraphQL Requests
Apollo can send several queries or mutations together in one go. This means less waiting for data to come back.
Deduplicate Requests
Turn on queryDeduplication
to stop Apollo from sending the same request more than once:
const client = new ApolloClient({
cache: new InMemoryCache(),
queryDeduplication: true
});
This helps avoid asking for the same data twice.
By using these tricks, you can make your app faster and more efficient. This leads to a smoother experience for your users!
Next Steps
Great job on getting the basics of Apollo Client and GraphQL for your apps! Now that you know how to ask for data, change it, store it efficiently, and hook up Apollo with React, here's what you can do next:
Learn Advanced Features
Explore more about what Apollo offers:
- Use Apollo Client hooks like
useReactiveVar
for handling local data - Use
fetchMore
to manage loading more data when needed - Update your cache after making changes with
update
- Make use of
onSubscriptionData
for updates from subscriptions - Look into server-side rendering with
getDataFromTree
You can find more info in the docs.
Optimize Performance
Speed up your app by:
- Setting custom rules for storing data
- Loading queries in advance
- Sending multiple requests together
- Keeping an eye on how fast your app runs
For tips on making your app work faster, check out the performance guide.
Add Authentication
Add login features and control who can see what with:
- JSON Web Tokens
- Using different
fetchPolicy
options in Apollo Client - Using
@client
and other directives
For more on adding login features, see the authentication tutorial.
Practice with Example Apps
Get real experience by building example apps with React and Apollo:
- Space Explorer - A fun way to learn Apollo features
- Shopify - Examples for creating a store and checkout
- Launchpad - Starting points for different types of apps
Try making your own projects using GraphQL and Apollo to get better!
We hope these tips help you keep getting better at using Apollo Client and making cool apps. Reach out if you have more questions!
Comparison of GraphQL Clients
When you're working with GraphQL in your apps, you'll need some tools to help you out. Let's look at a few popular ones and see how they stack up against each other:
Feature | Apollo Client | Relay | urql |
---|---|---|---|
Caching | Has its own way to save data for later | Keeps data around and cleans up what's not needed | Lets you add your own, doesn't come with it |
Bindings | Works with lots of different ways to build apps | Just for React apps | Good for several app-building tools |
Community | Lots of people use it | Not as many, but they really like React | Not too big, but it's getting there |
Learning Curve | Not too hard to start with | Takes a bit to get used to | Pretty easy to pick up |
Apollo Client is packed with features, has really good guides, and you can use it in many ways. It's pretty easy to start with and has a way to remember data so you don't have to ask for it again and again. A lot of people use it, so it's easy to find help.
Relay is great if you're making React apps and need to handle a lot of data. It's a bit tricky at first because it uses its own special way of doing GraphQL. It's got a smaller group of users, but they're all about React.
urql is all about being simple and not making things too complicated. It doesn't remember data on its own, but you can set it up your way. It's still growing, but it's a good choice if you want something straightforward.
Picking the right tool depends on what you're working on, what kind of data you need to handle, and what you're comfortable with. Apollo Client is a solid choice with lots of support, Relay is powerful for React, and urql is good for simpler projects. Think about what you need for your project to make the best choice.
Conclusion
Using GraphQL and Apollo Client can really make dealing with data in your apps a lot easier. Let's go over the main points we talked about:
- Understanding GraphQL - Think of GraphQL as a smarter way to ask for data compared to the old methods. It lets you ask for exactly what you need. We looked into queries (asking for data), mutations (changing data), subscriptions (getting live updates), and schemas (blueprints of your data).
- Setting up Apollo Client - You start by adding some tools to your project using npm, like
@apollo/client
andgraphql
. Then, you get Apollo Client ready and connect it with your React app using something calledApolloProvider
. - Writing queries and mutations - You can ask for data or change it using special commands called queries and mutations. We use something named
gql
to write these and hooks likeuseQuery
anduseMutation
to make them work in React. - Advanced features - We also talked about making your data requests even better with things like variables, directives, and fragments.
- Optimizing performance - There are tricks to make your app run faster, like caching (saving data to use later) and batching requests (asking for multiple things at once).
- Error handling - It's important to keep an eye on errors and deal with them properly to make sure your app works smoothly.
- Additional learning - Lastly, we suggested practicing with example apps, learning how to add login features, and checking out how to use Apollo with React Native.
We've covered a lot when it comes to using GraphQL and Apollo for managing data in your apps. If you have any more questions, feel free to ask!