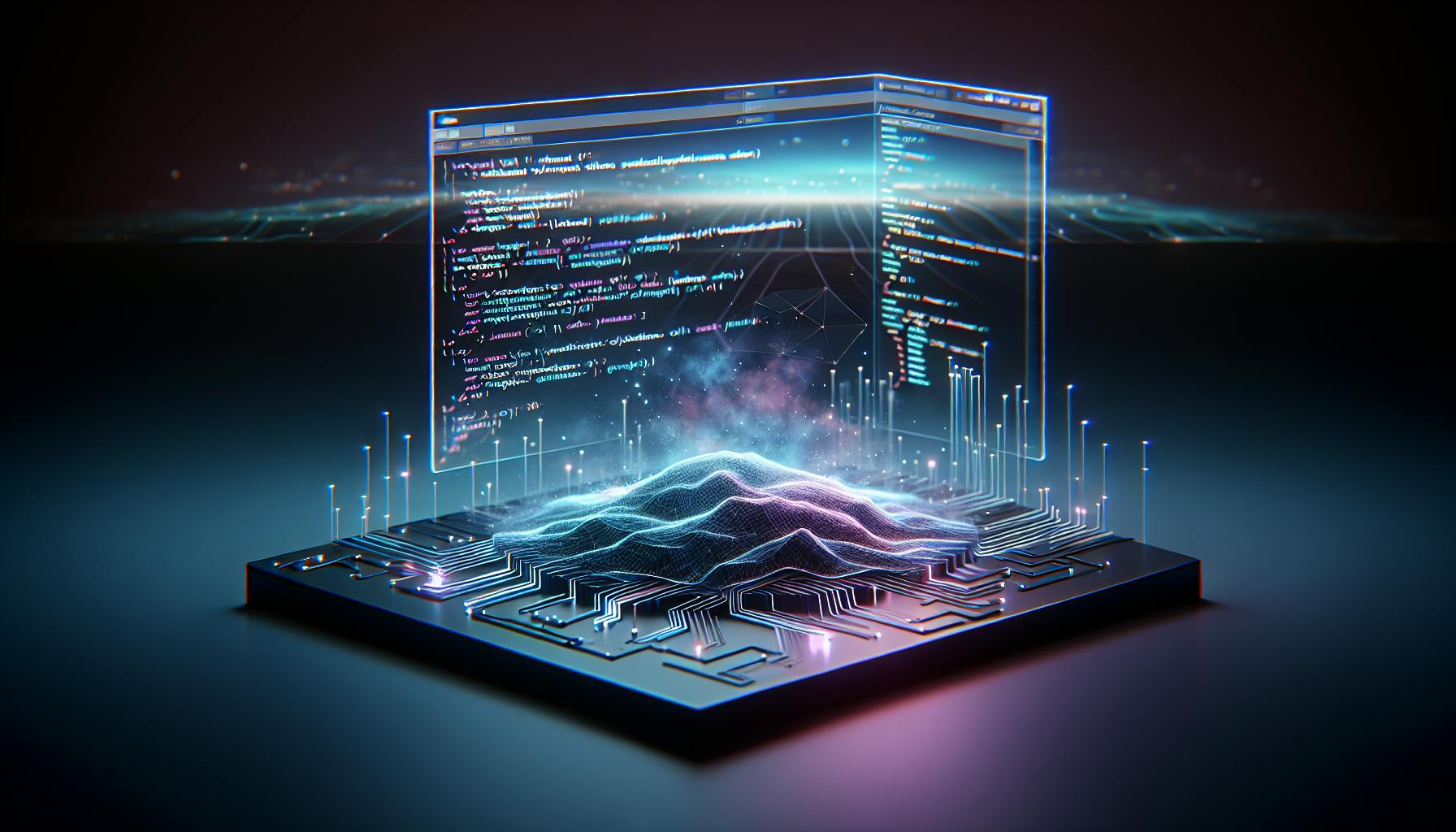
Learn how to create and manipulate arrays of objects in JavaScript. Understand variables, objects, arrays, loops, and functions. Explore finding, filtering, transforming, and sorting objects in arrays.
Creating arrays of objects in JavaScript can seem complex at first, but it's a powerful way to organize and manage data. This guide simplifies the process, breaking it down into easy-to-understand steps. Here's what you'll learn:
- Basics Required: Understanding of variables, objects, arrays, loops, and functions.
- Creating an Array of Objects: How to declare an empty array and add object values to it.
- Manipulating Arrays of Objects: Techniques for finding, filtering, transforming, and sorting objects within an array.
- Common Use Cases: Merging arrays into an array of objects and dynamically adding properties to objects.
By the end of this guide, you will be equipped with the knowledge to effectively create and manipulate arrays of objects in your JavaScript projects. Let's dive in and explore how to harness the power of arrays of objects to make your coding projects more organized and efficient.
Variables
Think of variables as containers for storing information. They can hold different kinds of data, like text (strings), numbers, and true/false values (booleans).
let name = "John"; // This holds text
let age = 30; // This holds a number
let isMember = true; // This holds a true/false value
Objects
Objects are like mini-databases for storing detailed info about something, such as a person or a product. They keep related details together.
let person = {
name: "John",
age: 30
};
In this example, person
is an object that stores name
and age
.
Arrays
Arrays are lists that can hold a bunch of data, even different types of data.
let fruits = ["Apple", "Banana", "Orange"]; // A list of text
let numbers = [1, 2, 3]; // A list of numbers
Loops
Loops are a way to do something over and over again, like going through each item in a list.
let fruits = ["Apple", "Banana", "Orange"];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
// This will show Apple, Banana, Orange
Functions
Functions are like mini-programs inside your main program. You can give them data, and they give you back a result.
function greet(name) {
return "Hello " + name;
}
let message = greet("John"); // This gives back "Hello John"
Understanding these basics will really help when we start combining them to use arrays of objects. Let's get into how to do that...
Creating an Array of Objects
Declaring an Empty Array
To start, let's make a blank list (array) where we can keep our objects. Here's how you do it:
let cars = [];
This line creates a new, empty list called cars
. It's like we have an empty box ready to fill up with information.
Adding Object Values
Now, let's put some objects into our list. We do this by using a command called push()
:
let cars = [];
cars.push({make: "Toyota"});
cars.push({make: "Ford"});
The push()
command adds new items to the end of our cars
list.
If we want to add more, we just keep using push()
like this:
let cars = [];
cars.push({make: "Toyota"});
cars.push({make: "Ford"});
cars.push({make: "Ferrari"});
Now, our cars
list has 3 objects, each one with a detail about the car's make.
We can also add more details to these objects anytime:
cars.push({
make: "Toyota",
model: "Prius",
year: 2019
});
This way, we can keep all sorts of related details together easily, like the make, model, and year of a car.
Manipulating Arrays of Objects
Finding Objects by Property
To find an object in our array based on a specific detail, like the car's make, we can use Array.find()
. This tool looks through each object and gives us back the first one that matches our search.
Here's how you can find a Toyota car in our list:
let toyota = cars.find(car => car.make === "Toyota");
This code checks each car to see if its make is "Toyota" and gives us that car if it finds a match.
Filtering Array Objects
If we want to see only certain cars, like those made in 2019 or later, we use Array.filter()
. This creates a new list with just the cars that meet our criteria.
For example:
let newCars = cars.filter(car => car.year >= 2019);
Now, newCars
will only have cars from 2019 or newer.
Transforming Objects
Sometimes, we might want to change the objects in our list a bit, like showing only the car's make and model. We can do this with Array.map()
, which lets us turn each item into something new while keeping the original data.
Like this:
let simpleCars = cars.map(car => {
return {
make: car.make,
model: car.model
};
});
Now, simpleCars
is a list with simpler car objects.
Sorting the Array
If we want to organize our cars by year, we can use Array.sort()
. This needs a special rule that tells it how to order the cars. For years, it looks like this:
cars.sort((a, b) => {
return a.year - b.year;
});
This will arrange the cars in order from oldest to newest based on their year.
sbb-itb-bfaad5b
Common Use Cases
Merge Arrays into Array of Objects
Imagine you have two lists: one with car brands and another with car models. You want to combine these into a single list where each entry is a car with its brand and model. Here's a simple way to do it using JavaScript.
For instance, you have these two lists:
let makes = ["Toyota", "Ford", "Tesla"];
And:
let models = ["Camry", "F-150", "Model S"];
You want to merge them to look like this:
let cars = [
{make: "Toyota", model: "Camry"},
{make: "Ford", model: "F-150"},
{make: "Tesla", model: "Model S"}
];
Here's a straightforward way to do it:
let cars = makes.map((make, index) => {
return {
make: make,
model: models[index]
};
});
This method takes each brand and pairs it with the matching model using their positions in the lists.
Dynamic Property Addition
Sometimes, you might want to add more details to your list of cars, like their price. Here's how you can add a new detail to each car:
let cars = [
{make: "Toyota", model: "Camry"},
{make: "Ford", model: "F-150"},
{make: "Tesla", model: "Model S"}
];
To add a price to each car, you can do this:
cars.forEach(car => {
car.price = 10000;
});
Now, each car in your list also shows its price:
[
{make: "Toyota", model: "Camry", price: 10000},
{make: "Ford", model: "F-150", price: 10000},
{make: "Tesla", model: "Model S", price: 10000}
]
This is a handy way to update details in your list whenever you need to.
Conclusion
By now, you've learned quite a bit about working with arrays of objects in JavaScript. Let's go over what we've covered:
- Creating empty arrays - You know how to start with an empty array like
let cars = []
and get it ready to fill with objects. - Adding objects to arrays - You've seen how to add objects, such as
{make: "Toyota"}
, to your array using.push()
. - Finding specific objects - You can find a particular object in your array using
.find()
based on something specific, like the make of a car. - Choosing certain objects - With
.filter()
, you can pick out objects that meet specific conditions, like cars from a certain year. - Changing objects in arrays - You've learned how to use
.map()
to change objects in your array without losing any data. - Ordering your arrays - You know how to arrange your objects in a certain order, like by year, using
.sort()
.
These skills are your toolkit for creating and managing lists of objects. Here are some ideas to try out:
- Create an array of products with details like name, price, and rating.
- Make a list of user profiles, including name, email, and age.
- Sort a list of movies by their release year.
Feel free to play around and see what kinds of data collections you can create. Working with arrays of objects can unlock many possibilities. The more you practice, the easier it gets.
I hope this guide has helped you get comfortable with using arrays of objects in your coding projects. Keep experimenting and happy coding!
Related Questions
How to create an array of objects in js?
To make an array of objects in JavaScript, just use square brackets []
and put your objects, surrounded by curly braces {}
, inside. Like this:
const cars = [
{make: "Toyota", model: "Camry"},
{make: "Ford", model: "F-150"},
{make: "Tesla", model: "Model S"}
];
It’s like making a list where each item is a detailed note about something.
How to create multiple objects in array JavaScript?
Here’s how to add many objects to an array:
- Start with an empty array
- Use the
.push()
method to add each object - Write each object with
{}
and fill in your details
Example:
let cars = [];
cars.push({make: "Toyota", model: "Camry"});
cars.push({make: "Ford", model: "F-150"});
Just repeat the .push()
step for as many objects as you want to add.
How to make an array out of an object JavaScript?
To turn an object into an array, you can use Object.entries()
like this:
let car = {make: "Toyota", model: "Camry"};
let carArray = Object.entries(car);
// [["make", "Toyota"], ["model", "Camry"]]
Object.entries()
changes your object into a list of pairs, each showing a detail and its value. You can also get just the names or just the values of the details with Object.keys()
and Object.values()
.
How do you initialize an array of objects?
To start off an array with several objects right away:
let cars = Array.from({length: 3}, () => {
return {
make: "Honda",
model: "Civic"
};
});
Here, Array.from()
makes a new array with 3 Honda Civic objects. Each time it runs, it adds another object to the array.