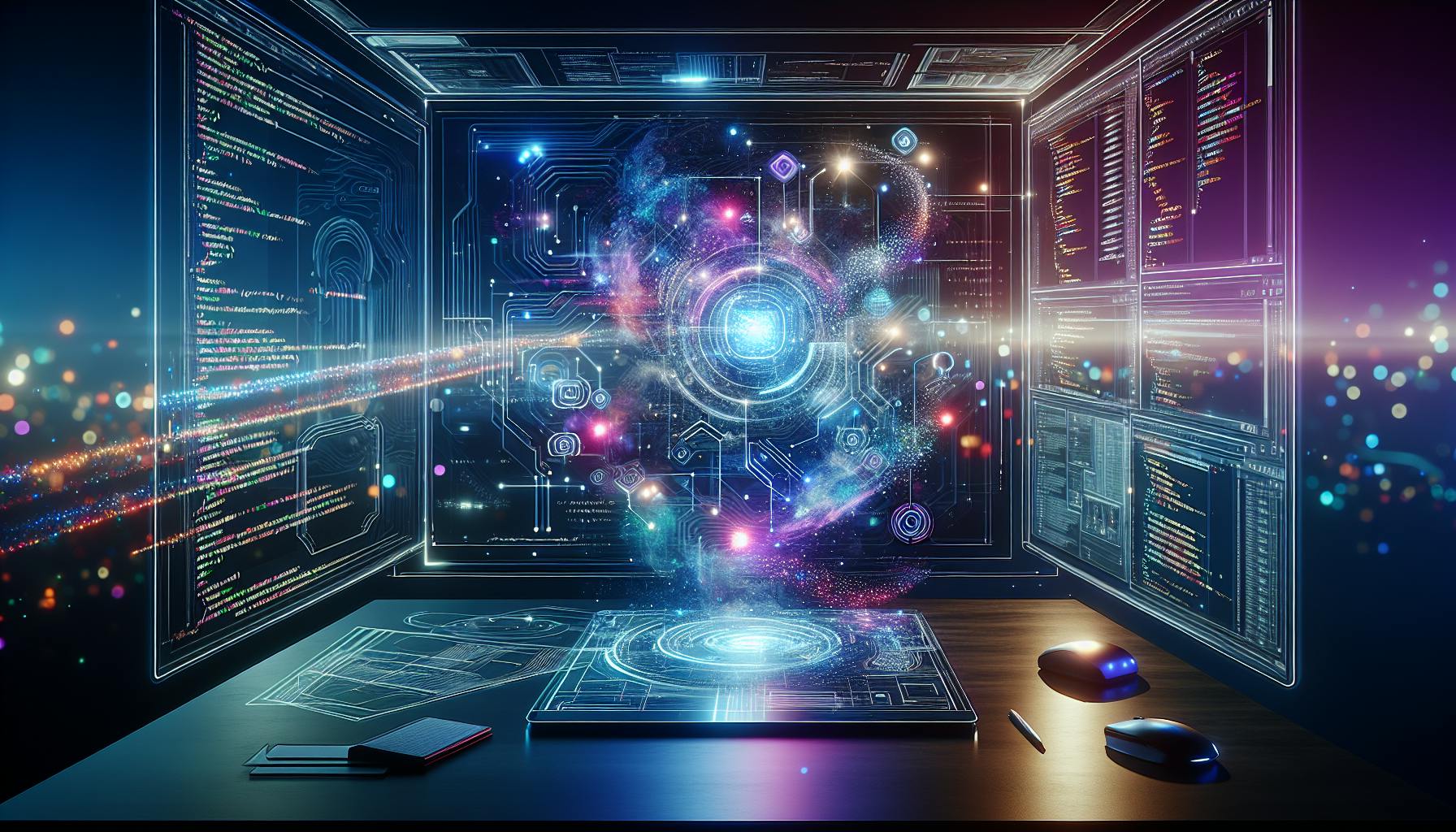
Learn the basics of Blazor, a powerful web development tool for building modern web applications using C# and .NET. Explore key benefits, architecture, and get started with your first app.
Blazor is a cutting-edge web development tool that lets you build web apps using C# and .NET instead of JavaScript. This guide introduces the basics of Blazor, including its key benefits, architecture, and how to get started with your first app. Whether you prefer to work in the browser with Blazor WebAssembly or on the server with Blazor Server, this overview covers what you need to know to begin your Blazor journey. Here's a quick look at what we'll cover:
- What Blazor Is: A framework for building interactive web applications using C#.
- Blazor's Key Benefits: Write in C#, use .NET libraries, and build fast, secure web apps.
- Architecture and Hosting Models: Understanding the differences between Blazor Server and WebAssembly.
- Getting Started: Setting up your environment and building your first app.
- Key Concepts: Components, routing, and data binding for dynamic content.
- Advanced Topics: Authentication, deployment, and performance optimization.
- Learning Resources: How and where to learn more about Blazor.
Whether you're new to web development or an experienced developer looking to leverage your .NET skills, Blazor offers a powerful platform for building modern web applications.
Key Benefits of Blazor
Here's why Blazor is a good choice for web development:
- You can write web apps in C#, not JavaScript
- You can use what you know about .NET
- You can have the same logic work on both the server and the client
- You can build web apps that feel fast and smooth, like native apps
- You get to use .NET's strong points like security and stability
- Debugging is easier with tools like Visual Studio
- What you learn in Blazor can be used in other areas too
Blazor is becoming popular for making modern web apps because it uses tools and languages that many developers are already comfortable with.
Blazor Architecture and Hosting Models
Blazor Server
Blazor Server apps work on the server inside an ASP.NET Core application. They use something called SignalR to talk to the client's browser. Imagine you click a button on a webpage; this click is sent over to the server via SignalR. The server figures out what's changed on the page because of that click and only sends back those changes. This way, the page updates without having to reload everything, making it feel quicker.
Blazor WebAssembly
Blazor WebAssembly apps run right in the browser using WebAssembly. When you first visit the app, it downloads everything it needs, including the .NET runtime, right into the browser. After that, the app can run and update itself without needing to talk back to the server. It can also talk directly to Web APIs from the browser.
Comparison Table: Blazor Server vs. Blazor WebAssembly
Blazor Server | Blazor WebAssembly | |
---|---|---|
App Location | Server | Client Browser |
Connection | SignalR | WebAssembly |
Initial Load Time | Fast | Slow |
Runtime Location | Server | Client |
State Management | Server | Client |
Scalability | Excellent | Limited |
Offline Access | No | Yes |
Getting Started
Prerequisites
To dive into Blazor, here's what you need:
- The latest .NET 6 SDK. Grab it from the .NET official site.
- A code editor. Visual Studio Code or Visual Studio works great.
- A basic understanding of C# and how websites work.
Don't forget to check if .NET SDK is correctly installed by typing this in your command line:
dotnet --version
Setting up Development Environment
Here's how to get your computer ready for Blazor development:
- Download and install the .NET 6 SDK.
- Pick Visual Studio Code or Visual Studio from their websites and install it.
- If your editor asks, add the C# extension.
- Make a new folder for your Blazor project.
- Open your command line tool in this folder.
- Type
dotnet new blazorserver -o MyBlazorApp
to create a new project. - Go into your new app's folder with
cd MyBlazorApp
and start it by typingdotnet run
. - Check out your new Blazor app running at http://localhost:5000!
Creating Your First App
Let's make a simple Blazor app that shows a "Hello World!" message:
- Set up your development environment by following the steps above.
- Find and open
Pages/Index.razor
in your new Blazor project. - Change the code there to:
@page "/"
<h1>Hello World!</h1>
- Start your app again. You should see "Hello World!" on the main page.
- Try changing the message to "Hello [Your Name]!". You'll see the change right away, no need to refresh the page.
That's all! You've just made your first Blazor app. To add more pages or learn more tricks, take a look at Microsoft's Blazor documentation.
Key Blazor Concepts
Understanding Blazor Components
Blazor components are like the building blocks for your website. They're parts of the webpage, like buttons or forms, that you can use over and over. They're written in a mix of C# (that's a programming language) and HTML (which is what web pages are made of).
Here's what you should know about these components:
- They end with
.razor
and have both C# code and HTML. - The C# part can do things like react to clicks or keep track of information.
- The HTML part shows the component on the webpage.
- You can use components inside other components, which is super handy.
- Components go through different stages, like starting up, and can react to changes.
- They can also take in data and change how they look or act based on that data.
For instance, check out this simple component that counts clicks:
@page "/counter"
<h1>Counter</h1>
<p>Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
}
This piece of code makes a counter that keeps track of how many times you click a button. You can add it to different pages easily.
Routing in Blazor
Routing is how your app knows what to show when someone visits a certain page. It connects web addresses to your components.
Important things about routing:
- Use
@page
to make a component show up at a specific address. - You can use
NavigationManager
to move around in your app without reloading the page. - You can pass data to components through the address.
- Routing works for both parts of your app that run in the browser and those that run on a server.
- You can set up routes in flexible ways to match different addresses.
- There are ways to make sure only certain people can visit some pages.
For example, to make the counter show up when someone goes to /counter
, you just add @page "/counter"
at the top of its code.
Data Binding
Data binding is like a bridge between your C# code and what people see on the webpage. It lets your code update the webpage or react when people do things like click a button.
Here are some ways you can use data binding:
@someValue
to show information@onclick
to make something happen when a button is clicked@bind
to keep form inputs connected to your data@someValueChanged
to get notified when something changes
So, if you want to show how many times a button has been clicked, you can use data binding to connect the button click to the counter.
<p>Current count: @currentCount</p>
<button @onclick="IncrementCount">Click me</button>
Data binding makes your components interactive and keeps your webpage up to date.
sbb-itb-bfaad5b
Building Complex Apps
Authentication and Authorization
Making sure only the right people can access your Blazor app is super important. Here are some steps to do that:
- Use ASP.NET Core Identity to handle user sign-ups, logins, and roles. It works well with Blazor.
- Keep user information safe by using techniques like hashing and salting for passwords.
- Use JWTs (JSON Web Tokens) or cookies to make sure each user request is legit.
- Use cascading parameters to share user info across your app.
- Check if users have the right roles and permissions before letting them see certain pages or use features.
- Keep your user management code separate from the rest of your app to make it easier to handle.
- You might want to use Blazored LocalStorage to keep user data on their device.
Here's a simple example of making a page that only logged-in users can see:
@attribute [Authorize]
<h3>This is only for logged-in users!</h3>
@code {
[CascadingParameter]
private Task<AuthenticationState> authenticationState { get; set; }
private async Task CheckUser()
{
var user = (await authenticationState).User;
if(!user.Identity.IsAuthenticated)
{
// Redirect unauthenticated users
}
}
}
Deployment Options
When you're ready to share your Blazor app with the world, here are your choices:
- IIS - Good for hosting Blazor Server apps with lots of features.
- Azure App Service - Easy to use for putting your app on the cloud with scaling.
- Static Hosting - Simple and scalable for Blazor WebAssembly apps that run in the browser only.
- Containers - Use Docker to package your app and run it anywhere, like with Kubernetes.
Think about these things when picking where to put your app:
- How big you expect to grow
- If you need server support or just browser
- How easy it is to keep up and manage
- Your budget
- Where your users are
- Keeping things secure and meeting rules
Make sure to:
- Turn on production mode
- Shrink your app's files
- Cut out any code you don't need
- Keep important data ready to go
Performance and Optimization
To make your Blazor app run smoothly and quickly, try these tips:
- Use Lazy Loading - Only load parts of your app when they're needed to keep the initial download small.
- Enable Prerendering - Show the first page quickly while the rest of the app loads.
- Compress Output - Make files smaller so they're faster to download.
- Limit Refreshes - Don't update the screen too much to keep things speedy.
- Cache Data - Save data on the device to avoid asking for it again.
- Go Headless - Run parts of your app without showing anything to save time.
- Analyze Issues - Use tools to find and fix slow spots.
- Adjust Virtualization - Make lists show up better without slowing things down.
With a little work, Blazor apps can be as fast as apps on your phone or computer.
Conclusion
Blazor is like a new tool for making websites that lets people who already know how to use .NET and C# jump right in. Whether you want your app to run in a web browser or on a server, Blazor has you covered.
Here's what we learned:
- Blazor is great for .NET developers because it lets you use C# instead of JavaScript. This means you can stick with what you know.
- Whether to use Blazor Server or Blazor WebAssembly depends on what you need your app to do, like if you want it to work offline or handle many users.
- Blazor has things like components, routing, and data binding that might sound familiar if you've made websites before, but here they work with .NET.
- You can build big, safe apps with Blazor and put them online in different ways, including on the cloud or in containers.
- You can make your Blazor app faster by only loading what you need when you need it, making files smaller, and storing some data so it's quicker to get.
To sum up, Blazor makes it easier for .NET developers to make web apps by using skills they already have. As more people start using Blazor, it'll get even better.
If you want to learn more, check out the Blazor documentation on Microsoft's website. There's also a big community of .NET users online where you can find help and tips.
Related Questions
How do I start learning Blazor?
To get started with Blazor, here's what you can do:
- Check out the Blazor documentation on the Microsoft website. It's a good place to begin.
- Try some tutorials on Microsoft Learn about publishing a Blazor app and making a REST API.
- Look for Blazor video tutorials on YouTube to see how things are done.
- Start with a small project to practice what you learn.
Learning by doing is a great way to understand Blazor better.
Is Blazor easy to learn?
If you're already comfortable with C# and .NET, learning Blazor will be smoother. The concepts and syntax are similar to what you already know.
But if you're new to C#, .NET, or making websites, Blazor might seem a bit tricky at first. Understanding how websites work can take some time, but having some programming background helps a lot.
What should I learn before Blazor?
Before diving into Blazor, you should be familiar with:
- Basic C# programming
- How websites are built with ASP.NET Core
- Basic website design with HTML and CSS
- How websites and servers talk to each other
Knowing these will help you pick up Blazor more easily.
What are the prerequisites to learn Blazor?
To start learning Blazor, you should know:
- How to write simple HTML
- The basics of C# programming
- How web browsers show websites
- Basic programming skills
With these under your belt, learning Blazor will be much more straightforward.