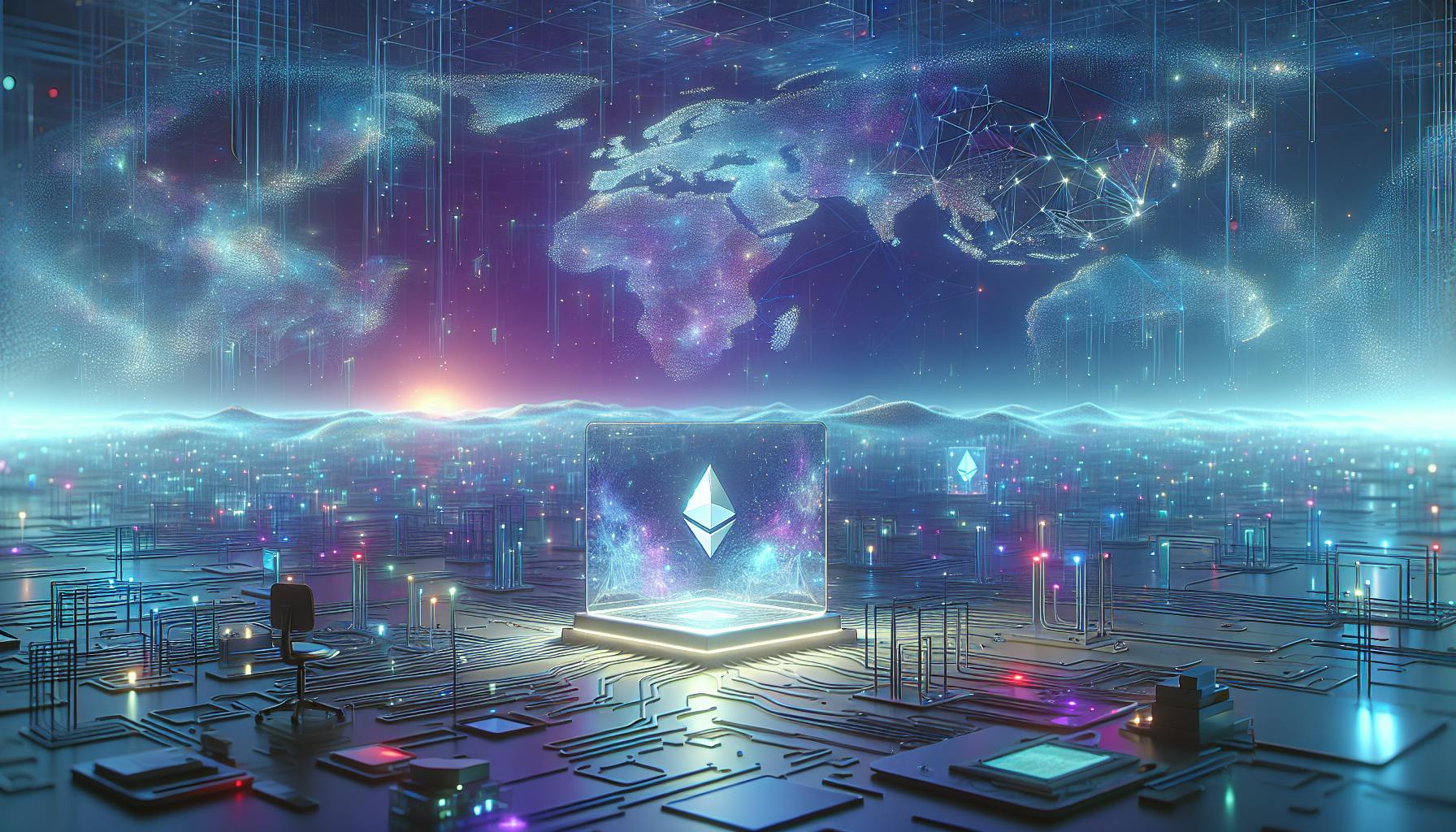
Learn the basics of Ethereum development, including smart contracts, gas transactions, security measures, and more. Get started with Ethereum tools and frameworks for building decentralized applications (DApps) on the blockchain.
Ethereum is a global, decentralized platform for money and new kinds of applications. It's like a vast, worldwide computer that anyone can use, powered by its currency, Ether (ETH). Here's what you need to know to start developing on Ethereum:
- Ethereum Network Basics: A decentralized system running on thousands of computers worldwide, not controlled by any single entity.
- Smart Contracts: Automatic agreements that run as programmed, without downtime, censorship, or fraud.
- Development Tools: Utilize Ethereum clients, frameworks like Hardhat, and libraries such as Web3.js for app development.
- Gas and Transactions: Understand the concept of gas as a transaction fee and how it powers the Ethereum Virtual Machine (EVM).
- Ethereum vs. Bitcoin: While both are cryptocurrencies, Ethereum's additional capabilities support complex contracts and applications.
- Security Measures: Learn about common vulnerabilities and practices to secure your smart contracts.
This introduction covers the essentials for developers interested in Ethereum, from its structure and how it operates to the tools and best practices for building on it.
Overview of the Ethereum Network
Think of the Ethereum network as a big, global system that runs without a boss. It works on lots of computers all over the world. Here’s what makes it special:
- Accounts - These are like your ID for using Ethereum. They keep track of your digital money (ether) and the smart contracts you’re part of.
- Transactions - When you want to send ether to someone or use a smart contract, you make a transaction. This is how you tell the Ethereum network what you want to do.
- Blocks - Transactions are grouped into blocks. These blocks are checked and added to a chain, which keeps a record of everything that’s happened.
- Smart Contracts - These are programs that run on Ethereum. They work automatically based on the rules they were given. This is how you build apps that can be trusted to work as promised.
- Gas - This is a bit like a fee for doing stuff on Ethereum. It’s paid in ether and goes to the people who help keep the network running.
- Nodes - These are the computers that make up the Ethereum network. They store all the transaction records and check new transactions.
This setup helps make things more secure and open.
Setting up a Development Environment
If you want to create Ethereum apps, you’ll need some tools. Here’s a quick list:
-
Ethereum Clients - These are programs that let you test your apps by running a mini version of Ethereum. Geth and OpenEthereum are two you might use.
-
Development Frameworks - These are like toolkits that make it easier to build your app. Hardhat, Truffle, and Embark are some examples.
-
IDEs - These are programs that help you write and manage your code. Remix and Visual Studio Code are good ones for Ethereum coding.
-
Libraries - These are collections of code that help your app talk to Ethereum. Web3.js (for JavaScript) and Ethers.js (for TypeScript) are popular.
-
Wallets - These let you manage your Ethereum accounts and sign transactions safely. MetaMask is a common choice.
Start with picking an Ethereum client and a development framework. Then, get the libraries you need, connect a wallet, and you’re ready to start coding!
Understanding Ethereum
Core concepts behind the Ethereum blockchain protocol.
Ethereum Virtual Machine Explained
Think of the Ethereum Virtual Machine (EVM) as the heart of Ethereum. It's like a big, worldwide computer that runs the code for smart contracts. Here's what you need to know about it:
- It acts like a giant computer made up of many accounts that can talk to each other using a special kind of computer language.
- There are two types of accounts: ones that people control with passwords (private keys) and ones controlled by code (contract accounts).
- Smart contract code is turned into a special code that the EVM can understand. All the computers in the Ethereum network use the EVM to make sure everything runs right.
- To do anything on the EVM, like running contracts or storing information, you need to pay gas. This is a way to make sure those helping the network run get paid.
So, the EVM lets developers build apps that are secure and work across the whole Ethereum network.
How Ethereum Differs from Bitcoin
Ethereum and Bitcoin might seem similar, but they're used for different things. Here are the main differences:
- Ethereum lets developers write complex contracts using a language called Solidity, which is more advanced than Bitcoin's scripting. This means you can do more complex things on Ethereum.
- Ethereum uses a system that's easier on the environment than Bitcoin's, and it's better for running big apps.
- Ethereum's system works faster, making it smoother to use apps on it.
- Ethereum has a special way (ERC-20) to create and trade digital money, making it easy to use different kinds of digital assets.
In short, while Bitcoin is like digital cash, Ethereum is more like a platform for building and running apps.
Understanding Gas and Transactions
Gas on Ethereum is like a fee for doing things on the network, such as sending money or using smart contracts. Here's the scoop on gas:
- You pay gas fees in Ether. The cost depends on how much computing work your action requires.
- Gas stops people from spamming the network and pays for the computing energy used.
- The gas price is what you're willing to pay per unit. Paying more can make your transaction happen faster.
- Bigger actions, like running smart contracts, need more gas because they're more complex.
So, gas makes sure the network runs smoothly by charging for the resources used.
Smart Contracts
Introduction to Smart Contracts
Smart contracts are like automatic deals that live on the Ethereum blockchain. They start working when certain conditions are met. They let you set up rules for things like who owns a digital item or how to manage a loan, and then make sure those rules are followed without anyone having to oversee it.
Some things you can do with smart contracts include:
-
Digital assets - They can represent things like digital art (NFTs) or tokens and manage who owns them and how they're moved around.
-
Financial stuff - Apps for decentralized finance use them for things like loans, insurance, and trading without a middleman.
-
Identity & reputation - They help manage who you are online and prove your credentials in a secure way.
-
Supply chain - They keep track of items from start to finish, making things more transparent and automated.
Smart contracts are a big deal because they offer trust, automation, and new possibilities for apps on Ethereum.
Solidity Basics
Solidity is the main language for creating smart contracts on Ethereum. It's a bit like JavaScript and lets you write rules for contracts. It has features like:
- Types of data like addresses, numbers, and text
- Gas model - you need gas (a kind of fee) to make your contract work
- Functions that can change data or do tasks
- Contracts are turned into a special code and put on the Ethereum network
Here's a simple example of a Solidity contract:
pragma solidity ^0.8.0;
contract MyContract {
uint myVariable = 10;
function myFunction() external {
// do something
}
event LogEvent(address sender);
}
Solidity lets you do more advanced stuff too, like creating complex contracts with libraries and other features.
Deploying Your First Smart Contract
Here's how to put your first smart contract out there:
-
Get ready - Download Node.js, npm, and tools like Hardhat.
-
Write your contract - Use Solidity to write your contract's code.
-
Make it into code the network can understand - Use a tool to turn your Solidity code into EVM bytecode.
-
Test it - Check your contract works as expected.
-
Put it on the network - Use a tool to add your contract to Ethereum.
-
Talk to your contract - Make a user interface to interact with your contract's functions.
For example, to store a number, you'd follow these steps with Hardhat and other tools like Ethers.js and Metamask.
Common Smart Contract Use Cases
Smart contracts are used for lots of cool things:
NFTs
- Make sure digital items are rare and owned properly
- Used for trading art, collectibles, and more
Decentralized Finance
- Create markets for loans and trading without central control
- Use special coins that keep their value stable
Supply Chains
- Keep an eye on goods from start to finish
- Make sure luxury items are real
- Make manufacturing more open
- Use smart devices and data feeds to automate processes
Smart contracts are shaping the future of how we use digital services!
Ethereum Development Tools and Frameworks
Overview of Popular Development Tools
When making apps for Ethereum, developers have a bunch of helpful tools and setups to choose from. Let's talk about some of the big ones:
Hardhat
Hardhat is a tool that makes it easier to build, put out, test, and fix Ethereum software. Here's what it does:
- Helps you see errors and debug with built-in tools
- Lets you work on different networks easily
- Has a system for testing your work automatically
- You can add more features with plugins
Hardhat is great because it takes care of the boring stuff and makes the whole process smoother.
Truffle
Truffle is like a multi-tool for Ethereum developers. It helps you to:
- Prepare and move smart contracts with simple commands
- Test contracts automatically with Mocha and Chai
- Set up custom networks for different environments
- Use the Truffle console to work directly with contracts
- Create user interfaces with extra tools
Truffle also has Drizzle for making app interfaces that update in real-time and Ganache for a private blockchain to test things quickly.
Remix
Remix is an online tool for writing Solidity smart contracts. It's cool because:
- You can start right away in your web browser
- It checks your code, tests, and helps you fix bugs
- You can add more features with plugins
- It lets you deploy and run contracts straight from the interface
Remix is super user-friendly, making it a great starting point for new developers.
These tools help you do everything from writing code to testing and putting your app out there. They make it easier to deal with complex tasks and help you build all sorts of applications on Ethereum.
Building Decentralized Applications (DApps)
Architecture of a DApp
Decentralized applications, or dApps for short, are apps that don't rely on a single computer or server. Instead, they run on a network of many computers, like Ethereum. Let's break down what goes into making a dApp:
Frontend
The frontend is what you see and interact with on your screen. It could be a website or an app on your phone, made with tools like React or Vue.js. This part talks to the backend (where the smart contracts are) using a special kind of link called an API.
Backend
The backend is where the smart contracts live. Think of these as the rules or the brain of the app, which live on the blockchain. They're written in languages like Solidity (for Ethereum).
Blockchain
This is the network of computers where the backend code and data are stored. Ethereum is a popular choice for this. It keeps the app running even if parts of it (like the frontend) stop working.
This setup means the app doesn't depend on one place or company to keep running. It's spread out across many computers.
Connecting Smart Contracts with Frontend
To make your web app and smart contract talk to each other, follow these steps:
-
Add Web3.js or Ethers.js to your web app. These are tools that help your app communicate with the blockchain.
-
When you set up your smart contract, you'll get a contract address and ABI (a way for your app to understand the contract).
-
Use Web3.js or Ethers.js in your app to connect to your contract using its address and ABI.
-
Now you can start using your contract's functions right from your app, like reading data or sending transactions.
-
Make sure your app updates when something changes in the contract, like showing a new token balance.
Here's a simple example of how it might look in code:
// Frontend code
import { ethers } from "ethers";
// Contract ABI
const abi = [...];
// Contract Address
const address = "0x...";
// Make contract instance
const contract = new ethers.Contract(address, abi);
// Call contract function
const data = await contract.myFunction();
This way, your web app can easily work with the smart contract in the backend!
sbb-itb-bfaad5b
Advanced Concepts
Introduction to Oracles
Oracles are helpers that give smart contracts information from the outside world. Since blockchains can't directly grab data like weather updates or stock prices, oracles are used to get and send this information to the blockchain.
Here's how they work in simple steps:
-
An oracle watches certain data sources outside the blockchain, like temperature readings or financial market prices.
-
When a smart contract needs this external data, it asks the oracle.
-
The oracle gets this data and sends it back to the smart contract in a way it can understand.
-
The smart contract then uses this info to do something, like make a payment if it rains a lot.
Oracles are super important for things like insurance claims, tracking items in a supply chain, and financial services. They connect blockchains with the real world.
Some well-known oracle services on Ethereum are Chainlink and DIA. They offer secure ways for smart contracts to get data they need.
Understanding Token Standards
Ethereum uses some common rules for creating digital items, called token standards:
ERC-20
This is for making tokens that are all the same, like digital coins. Each token is just like the others, which makes them perfect for things like digital money or points in a system.
ERC-721
This one's for unique digital items, like a one-of-a-kind digital artwork or collectible. Each item is different and special in its own way.
ERC-1155
This combines the best of both ERC-20 and ERC-721, allowing for items that share some qualities but are also unique in some ways. It's a bit more complex but offers more flexibility.
These rules help make sure that digital items can work well together across different apps and services.
Layer 2 Solutions and Scaling Ethereum
Ethereum is working on ways to handle more transactions faster and with lower fees. Here are a few methods:
Rollups
Rollups take a bunch of transactions, squish them into one small package, and only send this summary to the main Ethereum blockchain. This means less work for the main network.
Some popular rollup solutions are Optimistic Rollups and Zero-Knowledge Rollups (zkRollups).
Sidechains
Sidechains are like helper chains that run next to the main Ethereum blockchain. They take on some of the work, making things faster. They have their own rules and focus on specific kinds of transactions.
Projects like Polygon and xDai are examples of sidechains.
Sharding
Sharding breaks the Ethereum network into 64 smaller chains, which lets it process many more transactions at once. It's a big project that's still being worked on and could really speed things up.
Security in Ethereum Development
Common Vulnerabilities and How to Avoid Them
When you're building apps on Ethereum, keeping them safe is super important. Here are some issues that can pop up and how to dodge them:
Reentrancy
This is like when a contract tricks itself into sending more money than it should. You can stop this by:
- Making sure everything else is done before sending money out
- Double-checking the steps before moving money
Integer Overflows
This happens when numbers get too big and start acting weird. You can avoid this by:
- Using tools like SafeMath to handle big numbers safely
- Making sure numbers don't get too big
Access Control Issues
This is when the wrong person can do things they shouldn't. Keep things safe by:
- Only letting the right people do certain things
- Using checks to make sure someone has permission
Bad Randomness
This is about making sure things are truly random, so they can't be guessed. Make it better by:
- Using reliable sources for randomness like Chainlink
- Mixing up different bits of data in unexpected ways
Sticking to smart contract design tips, like the ones from OpenZeppelin, helps keep things tight. Also, getting your code checked and using math proofs can make sure it's solid.
Smart Contract Auditing and Formal Verification
To make sure your smart contracts are bulletproof, developers do a few things:
Auditing - Getting a pro to look over your code for sneaky bugs.
Formal Verification - Using math to prove your code does what it's supposed to.
Testing - Running tests to see if things work as expected.
For checking your work, you can use services like Quantstamp for audits.
For the math part, tools like the K framework can help prove your code is on point.
And for testing, using setups like Hardhat or Truffle makes sure your contracts behave.
Mixing all these methods gives you the best shot at keeping your smart contracts safe before letting them loose in the real world. Catching issues early can save a lot of headaches!
Hands-on Tutorials and Resources
Here are some top picks for getting your hands dirty with Ethereum development and creating real apps:
Interactive Coding Tutorials
- Buildspace - Simple guides on creating NFTs, DeFi apps, DAOs, and more.
- CryptoZombies - A fun way to learn by coding your own zombie game on the blockchain.
- Ethernaut - A game to test and improve your smart contract security skills.
Documentation Hubs
- Ethereum.org Developer Portal - The official place for all the basics.
- Chainstack Docs - How-tos on deploying apps, setting up your infrastructure, and more.
- DappUniversity - Guides on everything from wallets to trading platforms.
Developer Communities
- Ethereum Stack Exchange - A place to ask and answer Ethereum coding questions.
- Web3 University - A community offering courses for Web3 creators.
- r/ethdev - A Reddit community for Ethereum developers.
Code Libraries & Examples
- OpenZeppelin Contracts - A set of secure, reusable smart contracts.
- scaffold-eth - A starting point for Ethereum development with example projects.
- Solmate - A lightweight library for Solidity coding shortcuts.
These resources provide direct ways to boost your Web3 development skills through hands-on coding, learning materials, communities, and code samples focused on Ethereum.
Conclusion
Ethereum is like a big playground for creating new types of online apps that don't have to rely on a single company or server. This guide has walked you through the basics of how Ethereum works, what smart contracts are, and the tools you can use to build on this platform.
Here's a quick recap of what we covered:
- We started with the basics, like how Ethereum keeps track of who owns what and how it makes sure everything runs smoothly with something called gas.
- We talked about smart contracts, which are like automated rules that do stuff on their own once certain conditions are met.
- We went over the tools and programming languages that help you build and connect apps to Ethereum.
- We discussed some advanced stuff like oracles, which let smart contracts use data from the outside world, and ways to make Ethereum faster and able to handle more transactions.
- We also highlighted the importance of keeping your code safe and shared some resources to help you learn more and practice your skills.
Even though Ethereum can be a bit complex and faces some challenges with handling lots of transactions, there's a lot of work being done to improve it. For developers, this is a great time to dive in, learn how it all works, and start making your own Web3 apps.
The Ethereum community is full of people ready to help beginners, and there's no shortage of learning materials. By getting involved and trying things out, you'll get better and contribute to the growth of this exciting technology.
We suggest you start with the tutorials we mentioned, join online groups to ask questions, and play around with the tools and code. The sky's the limit when it comes to what you can build on Ethereum. So go ahead, get your hands dirty, and see what amazing things you can create!
Related Questions
How do developers use Ethereum?
Ethereum lets developers create and run apps and contracts that don't rely on any single computer or company. They use tools like Solidity, Hardhat, and web3.js to write and interact with Ethereum. Here are some things developers do with Ethereum:
- Make finance apps for things like loans and trading.
- Create unique digital items called NFTs.
- Start and manage groups that run on code, known as DAOs.
- Track items in a supply chain.
- Develop games and digital collectibles.
Ethereum's setup helps developers build apps that are open and don't need a middleman.
How to learn Ethereum development?
To start with Ethereum development, try these steps:
- Get to know Solidity, the main language for Ethereum contracts.
- Practice writing and testing contracts on Remix, an online platform.
- Go through tutorials and courses online.
- Join online groups to get help and advice.
- Try out frameworks like Hardhat or Truffle.
- Work on small projects to use what you've learned.
- Help out with Ethereum projects on GitHub.
- Keep up with new developments online.
Practicing and working on actual projects is the best way to learn.
What are the basic concepts of Ethereum?
Here are some key ideas behind Ethereum:
- Smart contracts - Code that runs by itself when certain conditions are met.
- Ethereum Virtual Machine (EVM) - The system that runs the code.
- Gas - A way to measure and pay for the work done by the network.
- Ether - The currency used on Ethereum.
- Nodes - Computers that keep the network running.
- Accounts - Places that can hold Ether and interact with contracts.
- Transactions - Actions that change something on Ethereum.
- Consensus mechanism - How the network agrees on changes, moving from a system called proof-of-work to proof-of-stake.
Understanding these ideas is important for using and building on Ethereum.
What programming language is used in Ethereum?
Solidity is the main language for writing Ethereum contracts. It's designed for creating smart contracts and is similar to languages like JavaScript. Here's what you should know about Solidity:
- It's structured and allows for creating complex contracts.
- It's similar to JavaScript, making it somewhat familiar to many developers.
- It includes features for safe programming, like managing big numbers.
- It supports secure coding practices.
Other languages are also being developed for Ethereum, but Solidity is the most used one.