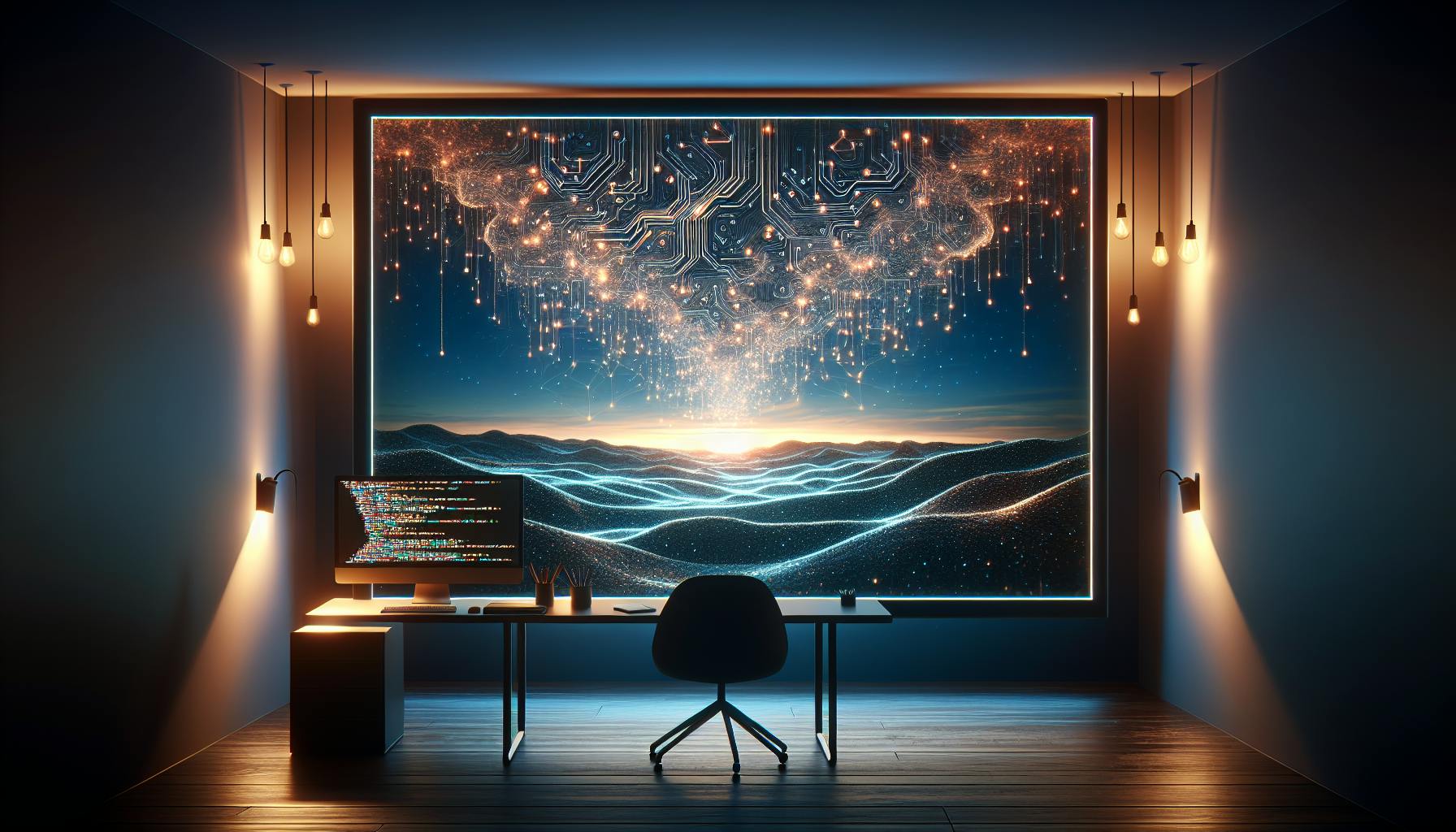
Learn how to efficiently filter arrays in JavaScript using the filter() method. Explore real-world examples and combine filter() with other array methods for advanced data handling.
Looking to sift through your JavaScript arrays and pull out only what you need? The filter()
function is your go-to tool. Here's a quick primer:
- What is
filter()
? A method to create a new array filled with elements that pass a test you define. - How does it work? Write a function that returns
true
for items you want to keep, andfalse
for those you don't. - Real-world applications: From improving search functionality on websites to sorting through database results,
filter()
is incredibly versatile. - Combine with other methods: Enhance its power by pairing
filter()
withmap()
andreduce()
for more complex data handling.
By the end of this guide, you'll understand how to use filter()
to efficiently work with arrays in JavaScript, picking out just the items you need for any given task.
Filter Method Syntax
Here's how you write filter()
:
let newArray = arr.filter(callback(element, index, array){
// say true or false
});
The function you write for filter()
can take three pieces of info:
element
- The item from the array you're looking at right nowindex
- Where this item is in the arrayarray
- The whole array you're filtering
Most of the time, you'll just need the element
to check if it fits what you're looking for.
How Filtering Works
filter()
goes through each item in the array, one by one, and shows it to your function.
If your function says true
, that item gets to be in the new array. If it says false
, it doesn't.
In the end, filter()
hands you a new array filled with just the items your function said true
for. This way, you can focus on just the items you need for whatever you're doing.
Using Filter() with Array Methods
Filtering arrays is a handy trick, but when you mix filter()
with other array tricks, you can do even more cool stuff.
Filtering and Mapping
Let's say you have a bunch of numbers, and you only want the even ones. First, you use filter()
to pick out the evens. Then, you use map()
to do something with them, like doubling each number.
Example:
let numbers = [1, 2, 3, 4, 5, 6];
let doubledEvens = numbers
.filter(n => n % 2 === 0)
.map(n => n * 2);
// doubledEvens = [4, 8, 12]
This way, you can first pick out the numbers you want, and then change them in a second step.
Filtering and Reducing
Or, you can filter an array to keep certain numbers, like those bigger than 10, and then use reduce()
to add them all up.
Example:
let numbers = [1, 12, 3, 14, 5, 16];
let sumOfNumbersAbove10 = numbers
.filter(n => n > 10)
.reduce((a, b) => a + b, 0);
// sumOfNumbersAbove10 = 42
This method helps you first find the numbers you're interested in, and then combine them in a useful way.
The main point is that filter()
works well with other tricks. You can link a few of them together to process your data in neat and tidy steps.
sbb-itb-bfaad5b
Real-World Examples of Array Filtering
Search Filtering
Filtering arrays comes in really handy when you want to help users find what they're looking for on websites, like on a shopping site. Imagine you're looking at a bunch of products, and you start typing in a search bar to find something specific. We can use a neat trick with arrays to only show products that match what you're typing.
// Array of product objects
let products = [
{
name: "Blue sneakers",
brand: "Adidas",
price: 99.99
},
{
name: "Red headphones",
brand: "Beats",
price: 149.99
},
//...more products
];
// Get array of products matching search term
function filterProducts(searchTerm) {
return products.filter(product => {
return product.name.toLowerCase().includes(searchTerm.toLowerCase());
});
}
let searchResults = filterProducts("red");
// This will show only the red headphones
This way, the website can quickly show you just what you're looking for without having to wait for the page to reload.
Filtering Database Results
filter()
is also super useful when we have a bunch of data, like from a database, and we want to show only certain bits of it. For example:
// Fetch list of users from database
let users = db.getAllUsers();
// Get users over age 21
let ofAge = users.filter(user => user.age >= 21);
Here, we're using filter()
to pick out only the users who are old enough, based on what we need to show in the app. It's like using a sieve to keep only the bits we're interested in.
Conclusion
The filter()
method is a handy way to pick out certain items from a list in JavaScript. Here's what you should keep in mind:
filter()
uses a special function that checks each item to see if it should be kept or not.- If the function says 'yes' (or
true
), that item goes into a new list. - You can mix
filter()
with other tools likemap()
andreduce()
to work on lists step by step. - It's really useful for things like making search features on websites or sorting through a lot of information quickly.
Using filter()
helps you narrow down lists to just what you need. It's great for when you're looking for something specific or dealing with lots of data.
By linking filter()
with other methods, you can neatly organize how you handle list data.
So, when you need to sort out items in a list according to certain rules, remember that filter()
is a straightforward way to do just that in JavaScript.