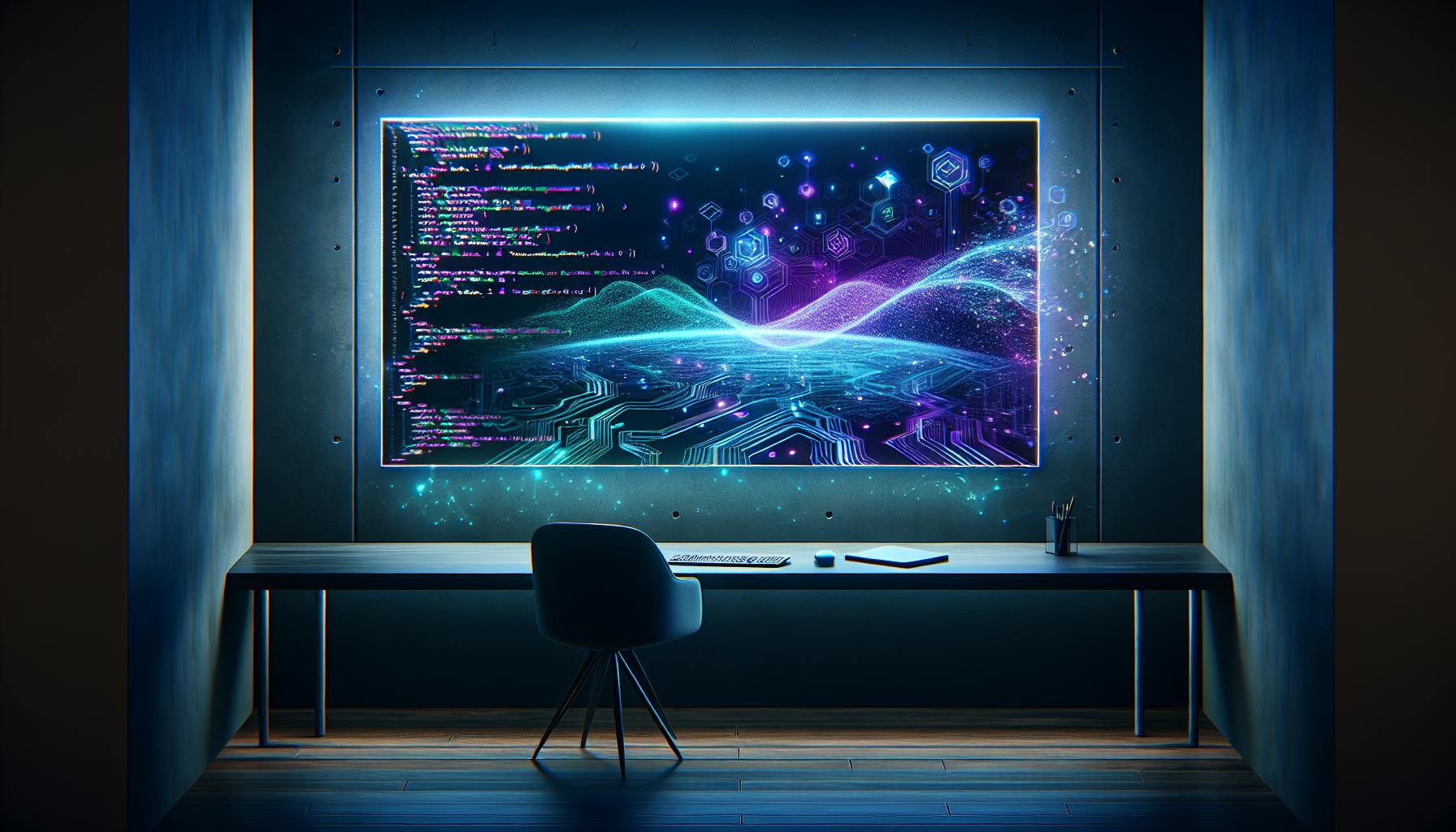
Learn about the JavaScript .map() method, its key features, how to use it, common pitfalls and solutions, real-world applications, and more. Master list transformation with .map()!
The JavaScript .map()
method is a powerful tool for developers, allowing for the transformation of list items in a clean and efficient way. Quick overview of what you need to know:
- What it does:
.map()
lets you create a new list by transforming each item in an original list according to a function you provide. - Key features:
- It applies a given function to each item in an array and returns a new array.
- The original array remains unchanged.
- It can handle numbers, strings, and objects, making it versatile.
- Useful in web development for data transformation and UI component rendering.
Understanding how to use .map()
effectively can streamline your code and make data handling tasks much simpler. Whether you're doubling numbers, capitalizing strings, or preparing data for display in a UI, mastering .map()
is a valuable skill for any JavaScript developer.
Prerequisites
Before diving into the .map()
method in JavaScript, here's what you'll need:
- A basic grasp of JavaScript, including what arrays, objects, and functions are. Think of these as the building blocks of JavaScript code.
- A place to write and test your code, like the Visual Studio Code editor.
- Node.js on your computer, which lets you run JavaScript outside a web browser. You can download it from nodejs.org.
- You can also use the Node.js command line tool. Just type
node
into your terminal to start it up. - Or, if you prefer, you can use the tools built into your web browser, like Chrome DevTools, to play with JavaScript code.
Having these things ready means you're all set to explore how to use the .map()
method in JavaScript. We'll look at how to work with lists of things—like words or numbers—by using .map()
to change each item in a list in some way. This is a key skill in web development, especially when dealing with data and making interactive web pages. So, even if you're new to coding, trying out the examples as we go will help you get the hang of it.
How to Use the JavaScript .map() Function
Step 1: Basic Numerical Transformation
Let's start with an easy example. Say you have a list of numbers and you want to double each one. Here's how you do it with .map()
:
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(n => n * 2);
// doubled is now [2, 4, 6, 8, 10]
We give .map()
a simple instruction (an arrow function) that takes each number n
and doubles it. This creates a new list with the doubled numbers.
Step 2: String Processing with .map()
.map()
isn't just for numbers. You can also use it to change text. Here's how to make the first letter of each name in a list uppercase:
const names = ['john', 'sarah', 'bob'];
const capitalized = names.map(name => {
return name[0].toUpperCase() + name.slice(1);
});
// capitalized is ['John', 'Sarah', 'Bob']
This code changes the first letter of each name to uppercase and keeps the rest the same.
Step 3: Utilizing .map() in Rendering UI Components
In web development, especially with React, .map()
helps show lists of things on the screen. Here's an example with a list of users:
const users = [
{ id: 1, name: 'John' },
{ id: 2, name: 'Sarah'},
{ id: 3, name: 'Bob' }
];
function User(props) {
return <div>{props.name}</div>;
}
function App() {
return (
<div>
{users.map(user => <User key={user.id} name={user.name} />)}
</div>
)
}
Here, we use .map()
to go through each user and make a <User />
component for them.
Step 4: Advanced Object Transformation
You can also use .map()
to change objects in a list. For example, if you want to get just the id
and age
from a list of user objects:
const users = [
{ id: 1, name: 'John', age: 25 },
{ id: 2, name: 'Sarah', age: 32 },
{ id: 3, name: 'Bob', age: 28 }
];
const userAges = users.map(user => ({
userId: user.id,
age: user.age
}));
// userAges is now:
// [{ userId: 1, age: 25},
// { userId: 2, age: 32 },
// { userId: 3, age: 28 }]
In this case, we're taking each user and making a new object that only has their id
and age
.
.map()
is a powerful tool for changing lists in JavaScript. It works for simple things like doubling numbers or more complex tasks like changing objects or showing things on a webpage.
Common Pitfalls and Solutions
When using the .map()
method in JavaScript, it's easy to run into some common issues. Let's talk about these issues and how to fix them:
Forgetting to Return Values from the Callback Function
One common mistake is forgetting to return a value from the function you use with .map()
. If you forget, you'll end up with an array full of undefined
:
// Missing return statement
const names = ['john', 'sarah', 'bob'];
const capitalized = names.map(name => {
name[0].toUpperCase() + name.slice(1);
});
// capitalized will be [undefined, undefined, undefined]
Solution: Always remember to return a value in your function:
const capitalized = names.map(name => {
return name[0].toUpperCase() + name.slice(1);
});
Attempting to Mutate the Original Array
Remember, .map()
creates a new array and doesn't change the original one. Trying to directly alter the original won't work:
const numbers = [1, 2, 3];
// This has no effect on numbers
numbers.map(n => n * 2);
Solution: To make changes, save the new array created by .map()
:
const doubled = numbers.map(n => n * 2);
Forgetting to Handle undefined Array Elements
If your array has empty spots (like undefined
), trying to do something with those spots can cause errors:
const numbers = [1, undefined, 3];
numbers.map(n => n.toFixed(2));
// Throws TypeError
Solution: Always check if there's a value before doing anything:
numbers.map(n => {
if (n === undefined) return null;
return n.toFixed(2);
});
By keeping an eye out for these common issues with .map()
, you can avoid annoying mistakes. Remember to always return something from your function, don't try to change the original array, and be careful with undefined values.
sbb-itb-bfaad5b
.map() vs. Alternatives
When you're working with lists in JavaScript, there are a few ways to go through them and make changes. Let's look at how .map()
compares to other methods like using for
loops or .forEach()
:
Approach | Use Case | Returns New Array? | Alters Original Array? |
---|---|---|---|
.map() |
Changing each item to make a new list | Yes | No |
for loop |
Going through a list when the order matters | No, you need to make a new list yourself | Yes, if you change things |
.forEach() |
Doing something like showing each item | No | Yes, if you change things |
.map()
is special because it gives you a new list after changing each item, but it doesn't mess up the original list.
Here's a quick breakdown:
- Use
.map()
when you want to change each item in a list and get a new list out of it. - Use
for
loops when you need to control exactly how you go through a list. But remember, you have to handle everything yourself. - Use
.forEach()
when you just want to do something with each item, like showing them, but you're not making a new list.
Choosing the right method depends on what you need to do. .map()
is great for when you need to change items in a list and get a new list without changing the original one.
Real-World Applications
The JavaScript .map()
method is super useful for handling data in real-world projects, especially when you're building websites or web apps. Let's look at some common ways developers use this method:
Data Transformation in React Apps
When working with React, a popular tool for making websites, you often need to change data you get from the internet or databases to show it on your site. Here's how .map()
comes in handy:
// Fetch array of users from an API
const users = [
{id: 1, name: "John"},
{id: 2, name: "Sarah"}
];
// Use .map() to get just the names
const names = users.map(user => user.name);
// Results in ["John", "Sarah"]
This code takes user data and pulls out just the names, leaving the original users
array unchanged.
Generating Lists of Components
In React, you often show lists of things on the screen. .map()
is perfect for this:
function User(props) {
return <li>{props.name}</li>;
}
function App() {
const users = [/* array of user objects */];
return (
<ul>
{users.map(user => (
<User key={user.id} name={user.name} />
))}
</ul>
);
}
This code goes through each user and makes a list item for them.
Processing Query Parameters
Sometimes, you get data from a webpage's address, like numbers or names. .map()
can help make sense of this data:
// If the webpage address is ?ids=1,2,3
const ids = url.query.ids.split(",").map(Number);
// Turns the string '1,2,3' into numbers [1, 2, 3]
It splits the string into pieces and then turns each piece into a number.
Data Filtering
You can also use .map()
to pick out certain items from a list based on a condition:
const products = [
{name: "T-Shirt", stock: 0},
{name: "Jeans", stock: 10}
];
const inStock = products.map(product => {
if (product.stock > 0) return product;
});
// Results in an array with just the jeans
This creates a new list that only includes products that are in stock.
In short, .map()
is really handy for changing data and making lists of things for websites. It's a key tool for web developers.
Conclusion
The JavaScript .map()
method is super useful for web developers who work with lists of data. It helps you change lists in a way that doesn't mess up the original list. Here's a quick summary of what we've learned:
.map() goes through lists and changes the data
Instead of using old-school loops, .map()
makes it simple. You just tell it how you want to change each item, and it does the rest. This makes your code easier to understand because you're just focusing on changing the data.
It makes a new list and keeps the old one the same
One of the best things about .map()
is that it doesn't change the original list. This means you can change data without worrying about messing anything up. You end up with a new list, and the old one stays as it is.
Useful for all sorts of things
Whether you're doing simple math, changing words, or making parts of a website with React, .map()
is really handy. It's great for getting data ready to show on a website.
A cleaner way to change lists than loops or .forEach()
.map()
is a clear way to say, 'I want to make a new list from this old one by changing each item.' It's more straightforward than using loops or .forEach()
, which can be a bit more complicated.
In short, learning how to use .map()
will make your life easier when dealing with lists of data in JavaScript. It's like a handy tool that keeps things clean and avoids messing up your data.
More Information
If you're looking to learn more about how to use the .map()
method in JavaScript, here are some helpful places to check out:
- MDN Web Docs on Array.prototype.map(): This is a super detailed guide that explains everything about
.map()
. It's like a handbook for how this method works. Check it out here. - JavaScript Array Map Method: W3Schools has a straightforward tutorial with examples that show you how to use
.map()
. It's a great place to start if you're new to this. Learn more here. - Eloquent JavaScript on Array Methods: This chapter from a book dives into
.map()
and other ways to work with lists in JavaScript. It's a bit more in-depth and perfect if you love reading. Read the chapter here. - Array.prototype.map() on DevDocs: If you need a quick reference or a reminder of how
.map()
works, this is the place. It's like a cheat sheet for JavaScript. Check it out here.
These resources will give you a deeper understanding of the .map()
method, show you how to use it in different situations, and offer more examples. They're great for both beginners and those who want to brush up on their JavaScript skills.