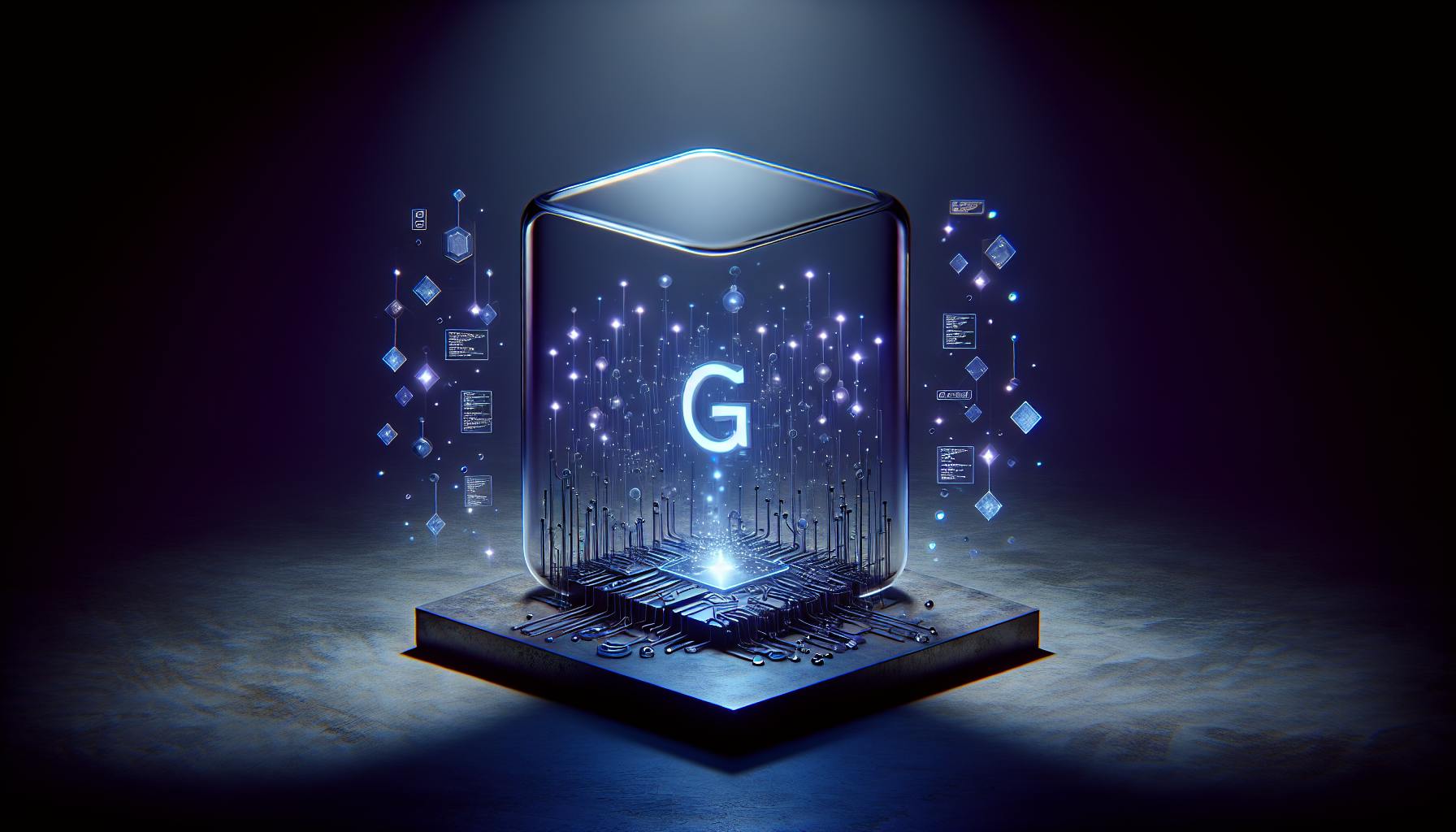
Learn how to simplify GraphQL queries by leveraging fragments, introspection, and JSON scalars. Discover strategies for efficient data retrieval while maintaining performance and security.
Getting all the fields in a GraphQL query can seem like a chore, especially when you just want to fetch everything without specifying each detail. Unlike traditional databases that allow a 'select *', GraphQL requires you to be explicit about what data you're asking for to ensure efficiency and security. However, there are strategies to simplify this process:
- Use Fragments to group commonly used fields.
- Leverage Introspection to dynamically discover fields available in the schema.
- Consider JSON Scalars for a more flexible, albeit less precise, way to fetch data.
This guide will delve into these strategies, providing insights on how to effectively use GraphQL to get all the fields you need while maintaining optimal performance and security. We'll also touch on best practices to ensure your queries remain efficient and your application stays responsive.
Queries and Mutations
- Queries let you ask for data.
- Mutations let you change data.
- You have to name every piece of data you want in your request.
For instance:
query {
user {
name
}
}
In this example, we're asking for a user
and only want to know their name
. If we were curious about their email
or address
, we'd have to say so directly.
GraphQL doesn't have a way to say 'give me everything about this user.' If new info is added to the user
, our request won't include it unless we add those new details ourselves.
This detailed approach means you always know what data you're getting.
Type System
GraphQL uses a detailed system to describe what kind of data an API can give you. Here are some types you might see:
- Scalar - Basic types like
String
,Int
,Boolean
, etc. - Object - Types with a structured set of fields, like a
user
. - Interfaces - Types that list common fields they share.
- Unions - Types that could be one of several objects.
- Enums - Special types with a set list of values.
Each type of data, like a user
's name
, is defined in a big list called a schema. This list tells us what kind of data each field holds and whether it's optional or required.
So, when we ask for a user { name }
, we're saying we want the name
from the User
object, which will give us a text string as defined in the schema.
Having this detailed system helps everyone understand what data is available and how to ask for it. Now, let's dive into how we can efficiently ask for all the data we want.
The Challenge of Querying All Fields
When you use GraphQL, you have to be clear about which details you want from the data. This is a good thing for a few reasons:
Performance and efficiency
- The server only grabs the data you asked for, which means it works faster.
- You don't waste internet data on stuff you don't need.
- You won't get extra info you're not looking for.
Reliability and stability
- If fields in the data change, it won't mess up your requests.
- You have to choose to ask for big chunks of data, so you won't accidentally slow things down.
Understandability and maintainability
- It's easy to see what data you're getting just by looking at your request.
- You don't have to worry as much about changes in the data messing up your app.
But, having to list every detail you want can be a pain:
Tedious to specify all fields
It's boring to write out every single field, especially if you're just trying to learn about the data.
Fragile to schema changes
Your requests might break if the data changes, which can be annoying.
Hard to access comprehensive data
Sometimes, you really do need to see everything about something, but that's tough if you don't know what to ask for.
Here are some ways to make it easier:
Fragments
Fragments let you group fields you use a lot into one piece you can use over and over. Like this:
fragment UserFields on User {
id
name
email
}
This helps keep things tidy and makes it less of a headache when the data changes. But you still have to list out all the fields.
Introspection
With introspection, you can ask GraphQL about itself to find out what fields are available. This way, you can build your requests on the fly. But be careful, because if everyone can do this, it might lead to security issues. Also, introspection doesn't actually get you the data.
Custom Scalars
Custom scalars are a way to say, 'Just give me whatever is here,' without worrying about the details. It's handy but skips over some of the benefits of GraphQL, like making sure the data is the right type.
Even though GraphQL makes you be specific, there are still ways to streamline getting all the data you need.
Strategies for Simplifying Queries
Introspection
Introspection is like asking GraphQL to tell you about itself. You can find out what kind of information you can ask for without having to guess or check the documentation all the time.
For instance, you might use a special query to get a list of all the things you can ask about, like this:
{
__schema {
types {
name
fields {
name
}
}
}
}
This gives you a big list of all the types and fields you can ask for. It's handy for building your queries on the fly, but you have to be careful. If everyone can peek at your schema, they might find ways to ask tricky questions. To keep things safe, you might want to:
- Only let trusted people use introspection
- Limit how often these queries can be run
- Hide sensitive info from being easily found
Fragments
Fragments are like shortcuts. You define a set of fields you use a lot and give it a name. Then, instead of writing out those fields every time, you just use the shortcut.
Here's an example:
fragment UserFields on User {
id
name
email
}
query {
user(id: "1") {
...UserFields
}
}
Now, whenever you need those fields, just use ...UserFields
. It saves time and keeps your queries tidy.
JSON Scalars
Some GraphQL setups let you use something called JSON scalars. This is a fancy way of saying, 'Just give me everything about this thing, and I'll figure it out.' It's like asking for a whole page of a book instead of a paragraph.
Here's how it might look:
type User {
info: JSON
}
{
user {
info
}
}
This gives you everything about the user in one go. It's super convenient but skips over the part where GraphQL helps you ask for just what you need.
Code Examples
Here's a simple way to use introspection to get all fields:
// Fetch schema introspection
const schemaData = await introspectSchema(schema);
// Find the 'Query' type
const queryType = schemaData.__schema.types.find(
type => type.name === 'Query'
);
// Make a query using all fields
let query = '{';
for (let field of queryType.fields) {
query += ` ${field.name}`;
}
query += '}';
// Run the query
const result = await graphql(schema, query);
And here's how you can use fragments to avoid repeating yourself:
const userFragment = `
fragment UserFields on User {
id
name
email
}
`;
const query = `
{
user(id: "1") {
...UserFields
}
}
${userFragment}
`;
These tips should help make asking for data in GraphQL a bit easier. If you have questions, feel free to ask!
Best Practices
When you're trying to make your GraphQL queries simpler by getting all the fields at once, remember these tips to keep things running smoothly:
Carefully Manage Access
Not everyone should see everything in your data or know how your system is built. To keep things safe, you might want to:
- Set up rules on who can ask for what data
- Slow down how often people can ask for everything, so your system doesn't get overwhelmed
- Make sure only the stuff that's okay to share is available to everyone
Balance Performance Needs
Asking for all the data at once can make your system work really hard. Think about:
- Only getting everything when you really need to, to keep things fast
- Checking how this affects your system before making it live for everyone
- Watching out for any problems early on
Maintain Strong Typing
Using GraphQL's flexible setup can sometimes make it hard to keep track of what type of data you're dealing with:
- Try not to use big, catch-all fields too much
- Make sure the data you get fits what you were expecting
- Be clear about what your custom data types are
Keep Code Maintainable
Queries that ask for all fields might stop working right if the data changes:
- Group fields together to make changes easier
- Use tools like Apollo codegen to update your code automatically
- Keep tweaking and testing your code, especially after updates
Document Responsible Usage
Help the people using your API to do it the right way:
- Tell them how big their queries should be
- Suggest asking for data piece by piece, as needed
- Explain the rules about asking for lots of data at once
By thinking things through and testing often, you can make the most of GraphQL's ability to be flexible while keeping your app fast and safe. Always weigh the pros and cons and keep an eye out for any changes.
sbb-itb-bfaad5b
Conclusion
Making GraphQL queries simpler can help developers a lot, but it's important to keep everything secure and running smoothly. Here's a quick summary of the main points:
Use what's already there
- Fragments help you not to write the same list of fields over and over.
- Introspection lets you peek at what data you can ask for without guessing.
Be smart with custom stuff
- JSON scalars let you grab all data in one go but be careful because it's not as precise.
- Making queries that ask for everything based on the schema might slow things down.
Stick to the best practices
- Control who can see what to keep a balance between being open and safe.
- Think about how allowing everyone to ask for everything affects speed.
- Try to keep data types clear to avoid confusion.
- Update your documentation as things change so everyone knows how to use your API right.
The idea is to find a good middle ground. Get the data you need without making things slow or risky.
Test different ways to make queries simpler and see what fits your project. It's all about balancing the benefits and drawbacks, and maybe mixing a few methods together.
With a bit of planning, you can make the most out of GraphQL's flexibility while dodging the usual issues. Aim for practical solutions, not perfect ones, and be ready to adjust. GraphQL makes it easier to ask for data in a smart way when you use it right.
Additional Resources
Here are some handy tools and places to learn more about getting all the details you need in GraphQL without too much hassle:
Documentation
- GraphQL Official Docs - A great starting point to understand how GraphQL works.
- Apollo Docs - Helpful info on setting up and using GraphQL with Apollo Server.
Tutorials
- How to GraphQL - Step-by-step lessons for beginners to get the hang of GraphQL.
- GraphQL Guide - Tips and videos to help you get better at using GraphQL.
Tools
- GraphiQL - A web tool for trying out GraphQL queries.
- GraphQL Playground - An advanced tool for working with GraphQL.
- Apollo Studio - A comprehensive platform for developing with GraphQL.
Community
- GraphQL Forum - A place to chat about GraphQL and ask for advice.
- GraphQL Stack Overflow - Where you can ask and answer questions about GraphQL.
- GraphQL Reddit - A community for sharing news and talking about GraphQL.
With these resources, learning how to manage your GraphQL queries should be easier. If you've got more questions, just ask!
Related Questions
How do I fetch all data from GraphQL?
To get all the data from GraphQL, you use something called queries. If you want to grab everything, you can use a tool within GraphQL called introspection
to create a query that asks for all the fields. But, doing this can make things slow, so it's usually better to just ask for the bits you really need.
What is the shortcut for all fields in GraphQL?
Some tools that let you work with GraphQL, like GraphiQL, have a quick shortcut (Ctrl/Cmd + Shift + R) that fills in a query with all the fields for a type for you. This is a quick way to write queries but remember, asking for everything all the time can lead to too much unnecessary data.
What is the equivalent of select * in GraphQL?
GraphQL doesn't have a simple 'give me everything' command like SQL's SELECT *
. You need to tell it exactly what information you want by listing each field. This makes you think about what you really need. There are some ways around this, like using introspection or custom scalars, but they skip over the careful planning part of GraphQL.
What is query in Gql?
A query in GraphQL is how you ask for specific data from a server. It lets you say exactly which pieces of information you want. This is different from traditional web requests, where you get a fixed set of data. With GraphQL, you have the power to shape your response to fit exactly what you need.