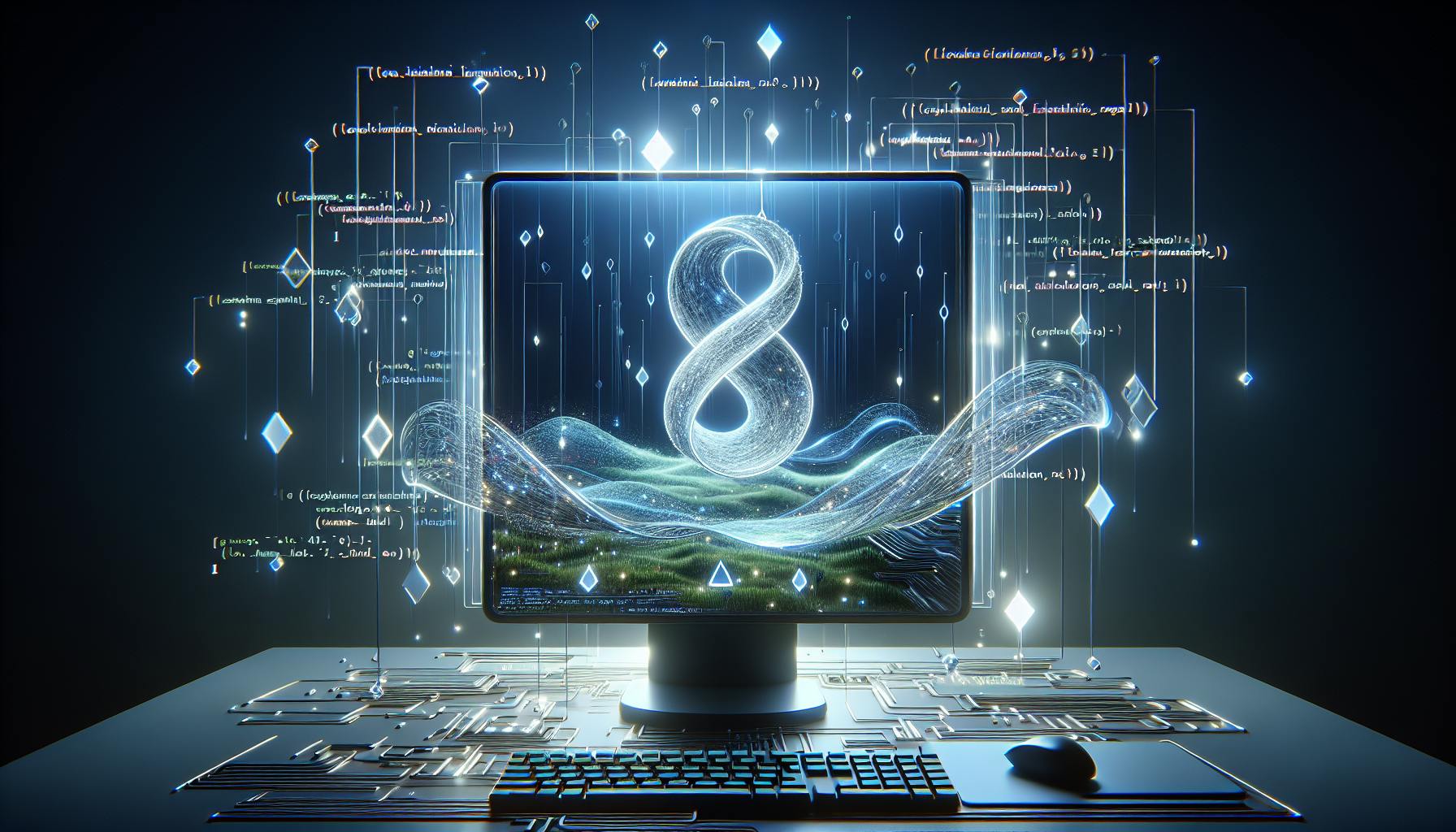
Learn how to efficiently use the for...of loop in JavaScript with index access. Discover different methods like Array.prototype.entries(), Array.prototype.keys(), and manual index handling. Improve your coding practices!
When working with JavaScript, looping through arrays or collections and knowing the index of each item can significantly boost your code's efficiency and readability. This guide dives into the for...of
loop—a powerful feature introduced in ES6—for iterating over iterable objects like arrays, strings, and more. Here's a quick overview:
- Using
Array.prototype.entries()
: Access both index and value of list items. - Using
Array.prototype.keys()
: Iterate over indexes, then access values separately. - Manual Index Handling: Keep track of the index with a simple counter.
Choosing the right method depends on your specific needs, such as whether you need direct access to items based on their index or if maintaining the order of operations is crucial. Here's what you'll learn:
- The importance of knowing item indexes in a loop.
- How to access the index in a
for...of
loop using different techniques. - A comparison of methods—
entries()
,keys()
, and manual indexing—highlighting their access to index and value, readability, and performance. - Practical use cases and recommendations for each method.
This guide aims to equip you with the knowledge to use indexes effectively in your JavaScript loops, enhancing your coding practices. Whether you're sorting, grouping, or directly accessing items, understanding how to leverage indexes in for...of
loops will make your code cleaner and more efficient.
Why Indexes Matter
Knowing where each item sits in the list can be super useful for things like:
- Looking up related info that's stored in a different place by using the position number
- Doing something special based on where the item is in the list
- Showing position numbers next to items
- Making changes to lists more efficiently
So, being able to get the position number while using for...of
can make your code more flexible and easier to read.
Accessing the Index
When you're looping through lists in JavaScript with for...of
, you don't automatically see what position, or index, each item is in. But sometimes, you really need to know that. Let's look at some easy ways to find out the index while still using for...of
.
Using Array.prototype.entries()
The Array.prototype.entries()
method gives you a way to loop through both the item and its index. Here's how you can use it:
const array = ['a', 'b', 'c'];
for (const [index, value] of array.entries()) {
console.log(index, value);
}
// Prints:
// 0 'a'
// 1 'b'
// 2 'c'
This breaks down each item into an index and a value, so you get both pieces of information every time through the loop.
Using Array.prototype.keys()
If you just want the indexes, you can use Array.prototype.keys()
:
const array = ['a', 'b', 'c'];
for (const index of array.keys()) {
const value = array[index];
console.log(index, value);
}
// Prints:
// 0 'a'
// 1 'b'
// 2 'c'
This method loops over the indexes, and then you can grab the values yourself.
Manual Index Handling
Or, you can just keep track of the index yourself with a simple counter:
const array = ['a', 'b', 'c'];
let i = 0;
for (const value of array) {
console.log(i, value);
i++;
}
// Prints:
// 0 'a'
// 1 'b'
// 2 'c'
This is a straightforward way, but it's a bit cleaner to use entries()
or keys()
if you can.
In short, entries()
and keys()
are great for looping with indexes in for...of
. And if they don't fit your needs, counting the index yourself is always an option. With these methods, you can make for...of
loops work even better for you.
Comparison of Techniques
When you're using for...of
in JavaScript to loop through things like arrays or strings, you might want to know both what you're looking at and where it is in the list. We've talked about a few ways to do this, like entries()
, keys()
, and just keeping track yourself. Let's see how they stack up against each other.
Index and Value Access
Here's a quick look at what you get from each method:
Method | Index | Value |
---|---|---|
entries() | Yes | Yes |
keys() | Yes | No |
Manual Counter | Yes | No |
entries()
is the only one that gives you both the spot in the list (index) and what's in that spot (value) right away. The other two make you find the value on your own if you need it.
Readability
Array.prototype.entries()
is neat because it keeps things simple. You don't have to add extra steps to keep track of the index yourself.
But, if you do keep track of the index on your own, it's super clear what you're doing when someone else reads your code. So, it's a bit of a trade-off between keeping things simple and making them clear.
Performance
If we're talking speed, doing the counting yourself is usually the quickest.
Using entries()
or keys()
means JavaScript has to do a bit more work behind the scenes, which can slow things down a tiny bit. But for most things you're doing, this won't really matter. The slowdown is only noticeable if you're working with really big lists or doing a lot of loops.
So, while keeping track of the index yourself might be a bit faster or clearer, entries()
does the heavy lifting for you and makes your code look cleaner. It's usually the best choice unless you have a good reason to count the index yourself.
sbb-itb-bfaad5b
Use Cases and Recommendations
When you need to work with the positions of items in a list while looping through it in JavaScript, different methods are better for different situations. Here are some tips on which method to use when.
Random Access
If you need to pick out and use specific items from a list based on their position, using Array.prototype.entries()
or just keeping track of the position yourself can be really helpful.
entries()
lets you get both the position and the item together:
for (const [index, value] of array.entries()) {
// You can get to the item directly with:
array[index];
}
- Keeping track of the position yourself also works well:
let i = 0;
for (const value of array) {
// Get to the item using the position counter:
array[i];
i++;
}
Both ways let you directly access items in the list when you need them.
Order-Dependent Operations
When the order of items matters, Array.prototype.entries()
and Array.prototype.keys()
are great choices.
These methods let you go through the list in order, which is perfect for:
- Sorting items based on their position
- Grouping items in a certain sequence
- Keeping track of how items are reordered
For instance:
const originalOrder = ['c', 'a', 'b'];
// Sort by position:
const sorted = originalOrder.sort(
(a, b) => array.indexOf(a) - array.indexOf(b)
);
// Map original position to new position
const sortOrder = originalOrder.map(x => sorted.indexOf(x));
console.log(sortOrder); // [1, 0, 2]
The main thing is that entries()
and keys()
let you look at the list in order, which is super useful for doing things based on where items are in the list.
In short, use entries()
and keeping track of the position yourself for when you need to directly access items, and use entries()
and keys()
when you're focused on the order of items. Pick the method that fits what you're trying to do best.
Conclusion
When you loop through things in JavaScript, knowing the position or 'index' of each item can be super useful. Here's what you should remember:
- The
Array.prototype.entries()
method helps you by giving both the index and the value of items as you loop through them. This keeps it simple. - Using
Array.prototype.keys()
gets you just the indexes. This means you'll need to grab the values yourself. - Keeping track of indexes on your own is pretty straightforward and makes things very clear.
- If you're really worried about making your code run fast, doing the index tracking yourself can be quicker than using
entries()
orkeys()
. - For times when you need to pick items based on where they are, use
entries()
or do the index tracking yourself. And for when the order matters,entries()
andkeys()
are your go-to. - Most of the time,
entries()
is your best bet for keeping your code easy to read and write, unless you have a specific reason to track the index yourself.
Knowing the index can help with sorting, grouping, and directly getting to certain items in your array. Mixing this with for...of
loops lets you write code that's cleaner and works better.
I hope this guide makes using indexes in your JavaScript loops a bit clearer. With a bit of practice, these tips will help you code more effectively. Happy coding!
Related Questions
How to get an index in a for of loop in JavaScript?
To find out the position of each item in a list while using a for...of
loop, you can use the entries()
method. This method gives you back each item as a small array where the first thing is the position (index) and the second thing is the item itself:
const array = [1, 2, 3];
for (const [index, value] of array.entries()) {
console.log(index, value);
}
// 0 1
// 1 2
// 2 3
This is a handy way to keep track of where you are in the list as you go through it.
How to get the index of a list in JavaScript?
To find the position of a specific item in a list, you can use the findIndex()
method. This method looks through your list and gives back the position of the first item that matches a certain condition.
For instance, if you want to find where 'banana' is in a list of fruits:
const fruits = ['apple', 'banana', 'orange'];
const bananaIndex = fruits.findIndex(fruit => fruit === 'banana');
// 1
How do you find the index of a for loop?
The simplest way to keep track of the position in a regular for
loop is to use a counter variable:
const list = [1, 2, 3];
for (let i = 0; i < list.length; i++) {
// i is the index
console.log(i);
}
Here, the i
variable starts at 0 and goes up by one each time the loop runs, acting as both the counter and the position tracker.
How to use forEach with index in JavaScript?
When you use the forEach()
method, it gives you the position of each item as the second thing in its callback function:
['a', 'b', 'c'].forEach((value, index) => {
console.log(index, value);
});
// 0 'a'
// 1 'b'
// 2 'c'
So, by adding a second parameter to your function, you can easily keep track of each item's position as you go through the list.