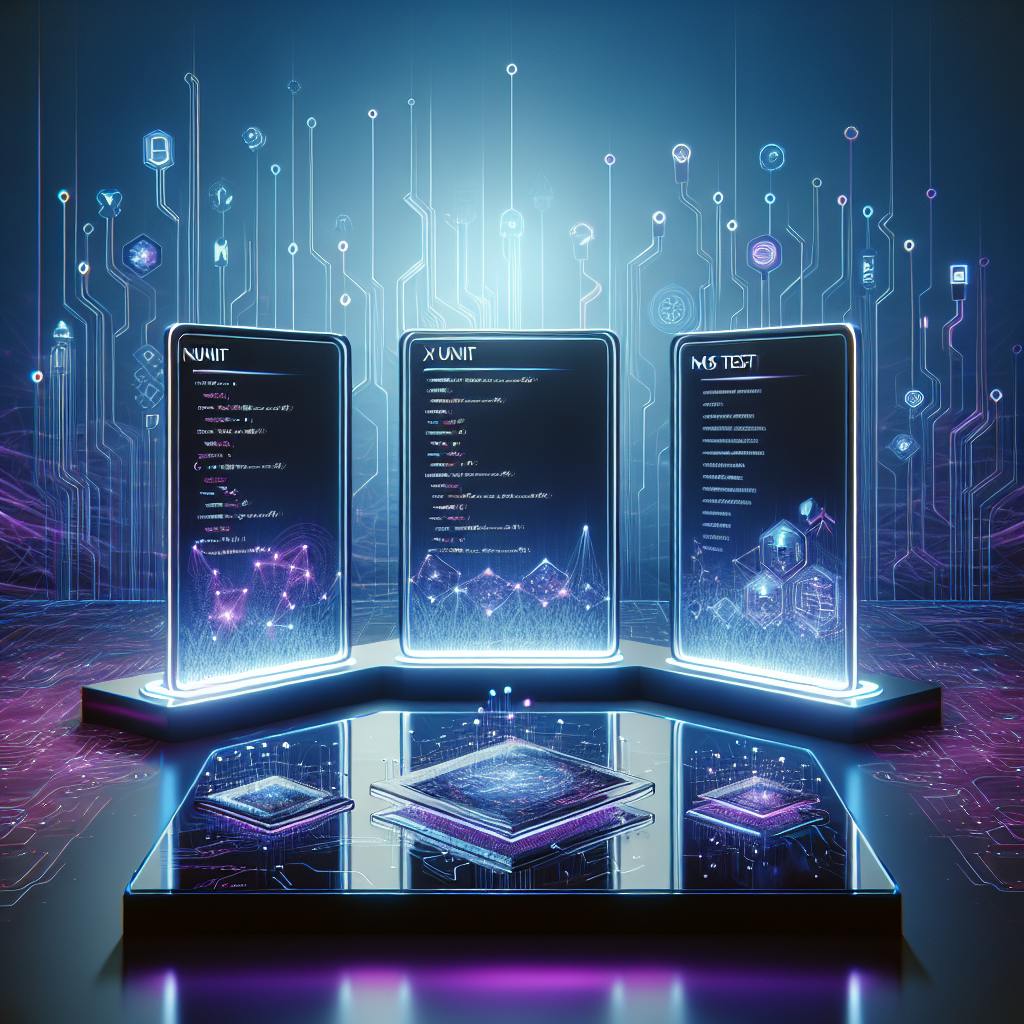
Comparison of NUnit, xUnit, and MSTest unit testing frameworks for .NET projects. Features, syntax, usage examples, and community support. Choose the best framework for your project.
Choosing the right unit testing framework for your .NET project is crucial. Here's a quick comparison of NUnit, xUnit, and MSTest:
Framework | Best For | Key Features |
---|---|---|
NUnit | Large, complex projects | Many features, strong community support |
xUnit | Modern .NET Core/.NET 5+ projects | Simple, fast, built for parallel execution |
MSTest | Teams heavily invested in Microsoft ecosystem | Seamless Visual Studio integration |
Quick comparison:
Feature | NUnit | xUnit | MSTest |
---|---|---|---|
Syntax | [TestFixture], [Test] | [Fact], [Theory] | [TestClass], [TestMethod] |
Setup/Teardown | [SetUp], [TearDown] | Constructor, IDisposable | [TestInitialize], [TestCleanup] |
Parameterized Tests | [TestCase] | [Theory], [InlineData] | [DataRow] |
Parallel Execution | [Parallelizable] | Default | Assembly-level config |
Resource Usage | Medium | Low | Medium |
Community Support | Strong | Very Strong | Good |
Choose based on your project size, team expertise, and existing toolset. Consider trying each framework on a small project to see which fits best.
Related video from YouTube
What are Unit Testing Frameworks?
Unit testing frameworks are tools that help developers test small parts of their code in .NET projects. These frameworks make it easier to write, organize, and run tests for individual pieces of code, like methods or classes.
A unit testing framework provides:
- A way to write test cases
- Tools to check if code works as expected
- Methods to set up and clean up test environments
When choosing a unit testing framework, consider these key features:
Feature | Description |
---|---|
Test organization | How the framework helps arrange and structure tests |
Assertion methods | Ways to check if code behaves correctly |
Parameterized testing | Support for running tests with different inputs |
Parallel execution | Ability to run multiple tests at the same time |
Tool integration | How well it works with other development tools |
These frameworks are important because they help catch bugs early and make sure code works properly. They're a big part of writing good software in .NET.
By using a unit testing framework, developers can:
- Find and fix problems quickly
- Make sure changes don't break existing code
- Improve the overall quality of their software
In the next sections, we'll look at three popular .NET unit testing frameworks: NUnit, xUnit, and MSTest. This will help you choose the best one for your projects.
NUnit: An Established Framework
NUnit is one of the oldest and most used unit testing frameworks for .NET. Many developers choose it because it has many features and a lot of community support.
History and Background
NUnit started as a copy of JUnit (a Java testing tool) for .NET. It has changed a lot since it began:
- It has had many updates
- NUnit 3.x was completely rewritten
- It now has many new testing features
NUnit is still widely used for testing both old and new .NET programs.
Key Features and Attributes
NUnit has many features for different testing needs:
Feature | Description |
---|---|
Assertion Library | Many ways to check if code works correctly |
Parameterized Tests | Run tests with different inputs using [TestCase] , [TestCaseSource] , and [ValueSource] |
Parallel Testing | Run multiple tests at the same time |
Test Organization | Use [TestFixture] , [SetUp] , and [TearDown] to arrange tests |
Custom Attributes | Make your own attributes to add new features |
Syntax and Usage Examples
NUnit tests are easy to write. Here's a simple example:
[TestFixture]
public class CalculatorTests
{
private Calculator _calculator;
[SetUp]
public void Setup()
{
_calculator = new Calculator();
}
[Test]
public void Add_WhenGivenTwoNumbers_ReturnsSum()
{
// Arrange
int a = 2, b = 3;
// Act
int result = _calculator.Add(a, b);
// Assert
Assert.That(result, Is.EqualTo(5));
}
[TestCase(1, 2, 3)]
[TestCase(0, 0, 0)]
[TestCase(-1, 1, 0)]
public void Add_WhenGivenMultipleInputs_ReturnsCorrectSum(int a, int b, int expected)
{
int result = _calculator.Add(a, b);
Assert.That(result, Is.EqualTo(expected));
}
}
This example shows how to use [TestFixture]
, [SetUp]
, [Test]
, and [TestCase]
attributes for simple and parameterized tests.
Strengths and Weaknesses
Pros | Cons |
---|---|
Many features | Can be hard to learn |
Good community support | Doesn't work as well with IDEs |
Can run tests at the same time | Fewer tools compared to newer frameworks |
Many ways to check results | Can be wordy for simple tests |
Flexible test setup | Can be hard to set up for complex tests |
NUnit is good for complex testing because it has many features and community support. However, it can be harder to learn than newer frameworks and might need more setup for advanced uses. Despite these challenges, many .NET developers still like using NUnit.
xUnit: A Newer Option
Origins and Philosophy
xUnit is a .NET unit testing framework made by the people who created NUnit. It aims to be simple and easy to change. Many .NET developers like it because it's easy to use and write tests with.
Key Features and Attributes
xUnit has some important features:
Feature | Description |
---|---|
[Fact] and [Theory] |
Used to mark test methods |
Constructor injection | For setting up tests |
Attribute support | To change how tests work |
Parallel testing | Runs tests at the same time for speed |
Syntax and Usage Examples
xUnit tests are easy to write. Here's a simple test:
public class MyTests
{
[Fact]
public void Add_WhenGivenTwoNumbers_ReturnsSum()
{
// Arrange
int a = 2, b = 3;
// Act
int result = a + b;
// Assert
Assert.Equal(5, result);
}
}
You can also do tests with different inputs:
public class MyTests
{
[Theory]
[InlineData(1, 2, 3)]
[InlineData(0, 0, 0)]
[InlineData(-1, 1, 0)]
public void Add_WhenGivenMultipleInputs_ReturnsCorrectSum(int a, int b, int expected)
{
int result = a + b;
Assert.Equal(expected, result);
}
}
Advantages and Limitations
Pros | Cons |
---|---|
Easy to use | Takes time to learn |
Can be changed easily | Fewer add-ons than NUnit |
Fast test running | Needs other tools for some checks |
Many .NET developers choose xUnit because it's easy to use and can be changed to fit their needs. It might take some time to learn, but many teams find it helpful for their projects.
MSTest: Microsoft's Framework
Development and Visual Studio Integration
MSTest is Microsoft's unit testing tool for .NET. It started around 2005 and was made to work well with Visual Studio. Now, MSTest V2 is open-source and works on Windows, Linux, and Mac.
MSTest V2 works better with Visual Studio than before. Developers can now use it for more than just Windows .NET projects.
Main Features and Attributes
MSTest has several useful features:
Feature | What it does |
---|---|
[TestClass] |
Marks a class with tests |
[TestMethod] |
Marks a test method |
[TestInitialize] |
Runs code before each test |
[TestCleanup] |
Runs code after each test |
[DataRow] |
Lets you test with different inputs |
These features help organize tests and set them up. MSTest V2 can also run tests at the same time, which makes testing faster.
Syntax and Usage Examples
Here's a simple MSTest example:
[TestClass]
public class MathTests
{
[TestMethod]
public void Add_WhenGivenTwoIntegers_ReturnsSum()
{
// Arrange
int a = 2, b = 3;
// Act
int result = a + b;
// Assert
Assert.AreEqual(5, result);
}
}
You can use [DataRow]
to test with different inputs:
[TestClass]
public class MathTests
{
[TestMethod]
[DataRow(2, 3, 5)]
[DataRow(0, 0, 0)]
[DataRow(-1, 1, 0)]
public void Add_WhenGivenMultipleInputs_ReturnsCorrectSum(int a, int b, int expected)
{
int result = a + b;
Assert.AreEqual(expected, result);
}
}
Pros and Cons
Pros | Cons |
---|---|
Works well with Visual Studio | Mainly for Windows developers |
Easy for Microsoft developers | Less flexible than other tools |
Good Visual Studio tools | Depends on Visual Studio |
Works on different systems | Smaller user group than NUnit or xUnit |
Can test with different inputs | Fewer advanced features than newer tools |
MSTest works great with Visual Studio and is easy for Microsoft developers. But it might not be as flexible or have as many features as newer tools like xUnit. The new MSTest V2 has fixed some problems, making it a good choice for many .NET developers.
sbb-itb-bfaad5b
Comparing the Frameworks
Let's look at how NUnit, xUnit, and MSTest are different. This will help you choose the best one for your .NET unit testing.
Syntax Differences
Framework | Test Class | Test Method | Setup | Teardown |
---|---|---|---|---|
NUnit | [TestFixture] | [Test] | [SetUp] | [TearDown] |
xUnit | No attribute needed | [Fact] or [Theory] | Constructor | IDisposable.Dispose() |
MSTest | [TestClass] | [TestMethod] | [TestInitialize] | [TestCleanup] |
NUnit and MSTest use attributes, while xUnit uses C# features.
Test Organization
NUnit and MSTest group tests with [TestFixture]
and [TestClass]
. xUnit doesn't need a special attribute for test classes.
Assertion Methods
Framework | Equality | Exception | Collection |
---|---|---|---|
NUnit | Assert.AreEqual() | Assert.Throws<T>() | CollectionAssert.AreEqual() |
xUnit | Assert.Equal() | Assert.Throws<T>() | Assert.Equal() (for collections) |
MSTest | Assert.AreEqual() | Assert.ThrowsException<T>() | CollectionAssert.AreEqual() |
xUnit's methods are shorter. NUnit and MSTest have more specific checks for different cases.
Parameterized Testing
Framework | Attribute | Example |
---|---|---|
NUnit | [TestCase] | [TestCase(2, 2, 4)] |
xUnit | [Theory] and [InlineData] | [Theory] [InlineData(2, 2, 4)] |
MSTest | [DataRow] | [DataRow(2, 2, 4)] |
All frameworks let you test with different inputs. xUnit's way is a bit more flexible.
Parallel Execution
- NUnit: Use
[Parallelizable]
to run tests at the same time - xUnit: Runs tests at the same time by default
- MSTest: Set up in the assembly to run tests at the same time
xUnit is best for running tests at the same time. NUnit and MSTest need extra setup.
Tool Integration
All three work well with common .NET tools:
- Visual Studio: Works with all three
- CI/CD: Works with tools like Jenkins, Azure DevOps, and GitLab CI
- Code Coverage: Works with tools like dotCover and OpenCover
MSTest works a bit better with Visual Studio. NUnit and xUnit have good community support and many add-ons.
Speed and Resource Use
How fast and efficient NUnit, xUnit, and MSTest are can affect how quickly you can test your code. Let's look at how these frameworks compare in speed, resource use, and handling many tests.
Execution Speed
How fast tests run matters because it affects how quickly you can check your code changes.
Framework | Speed |
---|---|
NUnit | Medium |
xUnit | Fastest |
MSTest | Medium |
xUnit runs tests the fastest because it's built to be light and quick. NUnit and MSTest are a bit slower but still work well.
Resource Use
How much computer power a framework needs is important. Using less power means you can run more tests at once.
Framework | Resource Use |
---|---|
NUnit | Medium |
xUnit | Lowest |
MSTest | Medium |
xUnit uses the least computer power. NUnit and MSTest use a bit more but are still okay for most projects.
Handling Many Tests
As you write more tests, you need a framework that can keep up. A good framework should work well even with lots of tests.
Framework | Handling Many Tests |
---|---|
NUnit | Good |
xUnit | Very Good |
MSTest | Good |
xUnit is best at handling many tests. It's made to work well with big test sets and can run many tests at the same time. NUnit and MSTest can also handle many tests, but not as well as xUnit.
In short, xUnit is the fastest and uses the least resources. It's also best for big projects with many tests. NUnit and MSTest are good choices too, but they're not as fast or light as xUnit.
Community Support and Resources
When picking a unit testing framework, it's good to look at how much help you can get from other users and what learning materials are available. These things can make using the framework easier.
Documentation Quality
Framework | Documentation Quality |
---|---|
xUnit | Very good, covers many topics |
NUnit | Good, has a dedicated book |
MSTest | Good, but not as detailed as xUnit |
xUnit has very good documentation. It helps you start with .NET Core and .NET Framework, and covers other topics too. It tells you how to use the command line, Visual Studio, and JetBrains Rider.
NUnit and MSTest also have good documentation, but not as much as xUnit. NUnit has a book called "Pragmatic Unit Testing in C# with NUnit, 2nd Ed." by Andrew Hunt and David Thomas. This book gives a lot of information about using NUnit.
Third-Party Tool Integration
All three frameworks work well with popular development tools:
Tool | xUnit | NUnit | MSTest |
---|---|---|---|
Visual Studio | โ | โ | โ |
Visual Studio Code | โ | โ | โ |
ReSharper | โ | โ | โ |
CodeRush | โ | โ | โ |
TestDriven.NET | โ | โ | โ |
This makes it easy to use these frameworks in your work.
Community Involvement
xUnit is open-source, which means many people help make it better and offer support. It's part of the .NET Foundation, so it follows good rules for working together. This makes xUnit a good choice if you like working with others and want a tool that can change to fit your needs.
How to Choose a Framework
Picking the right unit testing framework for your .NET project depends on what you need. Here's how to choose:
Look at Your Project Needs
Think about:
- How big and complex your project is
- What your team knows
- What tools you already use
When to Use Each Framework
Framework | Good For |
---|---|
xUnit | .NET Core and .NET 5 projects, teams that like new tools |
NUnit | Big, complex projects that need lots of features |
MSTest | Teams that use a lot of Microsoft tools |
Changing Frameworks
If you want to switch frameworks:
- Check if your old tests will work with the new framework
- Think about how long it will take to learn the new framework
- Plan how to move your tests over time
Tips for Choosing
1. Look at your project size
Small projects might like xUnit because it's simple. Big projects might prefer NUnit because it has more features.
2. Think about your team
If your team knows one framework well, it might be easier to keep using it.
3. Check your tools
Make sure the framework works with the tools you use, like Visual Studio.
4. Consider future needs
Think about what you might need later. Some frameworks are better for certain types of testing.
5. Try them out
If you can, try each framework with a small part of your project. This can help you see which one works best for you.
Conclusion
Picking the right unit testing framework for your .NET project depends on several things:
- How big your project is
- What your team knows
- What tools you use
Let's look at the three main frameworks:
Framework | Best for |
---|---|
NUnit | Big projects with lots of features |
xUnit | New .NET Core and .NET 5 projects |
MSTest | Teams that use many Microsoft tools |
When choosing a framework, think about:
- What your project needs
- What your team is good at
- What tools you already use
Look at each framework's:
- Features
- How to write tests
- Help from other users
By doing this, you can pick a framework that:
- Fits your project
- Helps you make good software
- Makes testing easier
FAQs
What's the difference between NUnit and MSTest?
NUnit and MSTest are both unit testing tools for .NET, but they have some key differences:
Feature | NUnit | MSTest |
---|---|---|
Type | Open-source | Made by Microsoft |
Flexibility | More options to change | Works well with Visual Studio |
Running tests at the same time | Use [Parallelizable] |
Set up in the assembly |
NUnit gives you more ways to change how tests work, while MSTest fits nicely with Microsoft tools.
Which unit testing tool is best for .NET Core?
There's no single best tool for .NET Core testing. It depends on what you need. Here's a quick look at the main options:
Framework | Good for |
---|---|
NUnit | Big projects with lots of tests |
xUnit | New .NET Core and .NET 5 projects |
MSTest | Teams using many Microsoft tools |
To pick the right one, think about:
- How big your project is
- What your team knows
- What tools you already use
The best choice will fit your project's needs and your team's skills.