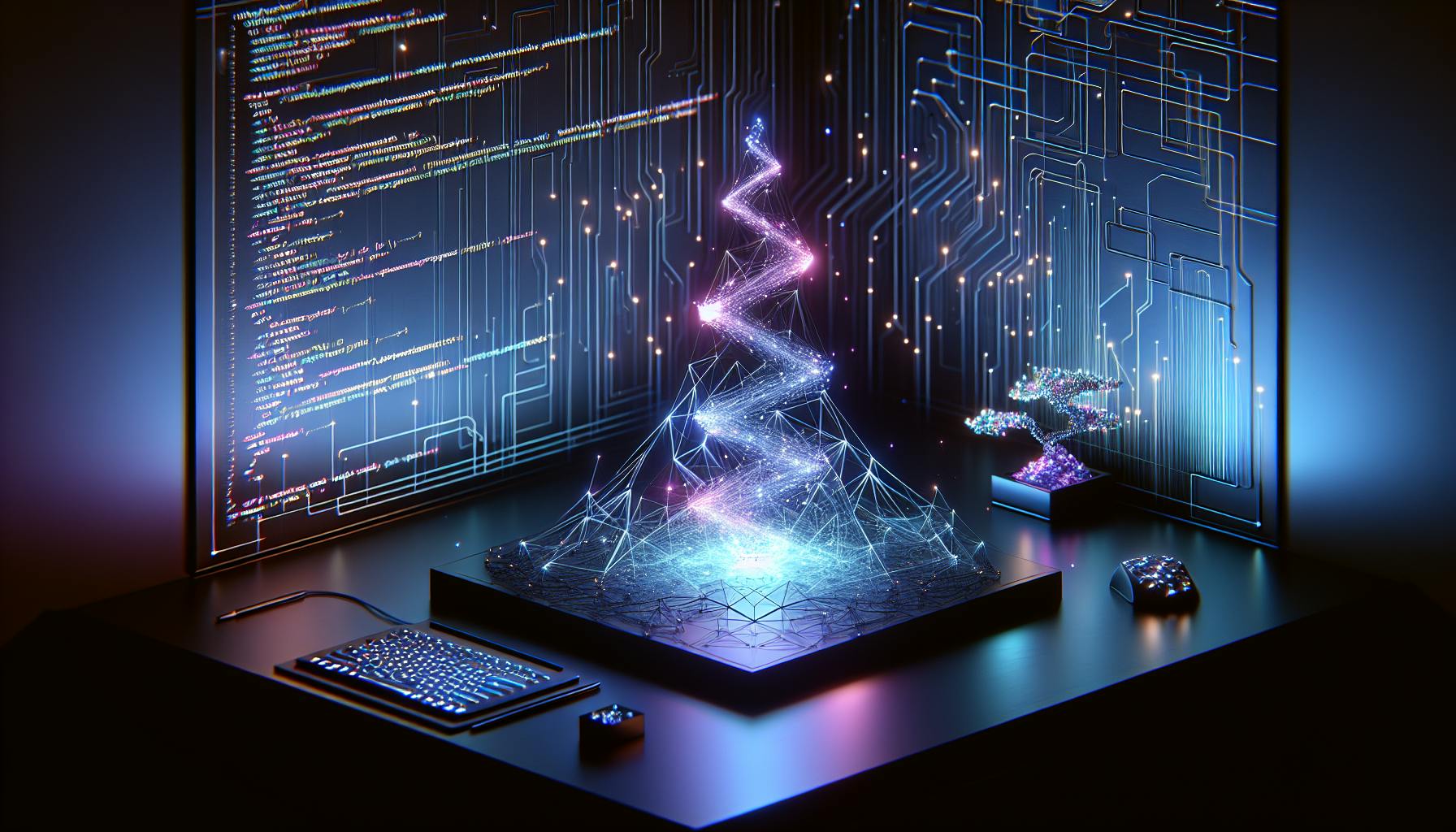
Learn how to use the join() method in JavaScript to convert arrays into strings with customizable separators and handle null values. Discover practical examples and common FAQs answered.
The join()
method in JavaScript is an essential tool for converting arrays into strings. This method allows you to specify a separator for the items in the array, defaulting to a comma if none is provided. It's a versatile function, useful for a range of tasks like creating readable strings from data arrays, constructing URLs, or formatting output. Here's a quick overview:
- Converts arrays to strings: Easily transforms list items into a single, cohesive string.
- Customizable separator: Choose any character(s) as your separator, including spaces, commas, or special symbols.
- Preserves the original array: Returns a new string without altering the original array.
- Handles nested arrays and null values: Joins nested arrays into the string and skips over
null
orundefined
values. - Broad browser compatibility: Works across nearly all web browsers, ensuring wide usability.
By understanding how to effectively use the join()
method, you can streamline your JavaScript coding tasks, making data manipulation and presentation more straightforward.
Syntax and Parameters
Here's how you write it:
array.join(separator)
What this means:
array
is just your list of items you want to stick together.separator
is what you want to put between each item. It's optional. If you don't use it, it'll just use a comma.
You can use different things as separators, like:
- A comma (
,
) - A space ( )
- A plus sign (
+
) - A pipe (
|
) - Or even nothing at all (
""
), if you want to squish everything together without any space.
Here are some quick examples:
let arr = ["Hello", "world"];
arr.join(); // "Hello,world" (uses a comma by default)
arr.join(""); // "Helloworld" (no space in between)
arr.join(" "); // "Hello world" (uses a space)
arr.join("+"); // "Hello+world" (uses a plus sign)
So, the join() method is a straightforward way to turn a list of items into a single string, and you get to choose how to separate those items. It's a handy tool for making arrays easier to read or use.
How Does the Join Method Work?
The join()
method in JavaScript is like a glue for sticking all the pieces of an array together into one string.
It takes each thing in the array, puts a separator you choose between each one, and sticks them into one line. This makes it super easy to turn a list of things into a single line of text.
Some key points about how join()
works:
Default Behavior
If you don't tell it what to use as a separator, join()
will automatically use a comma (,
).
For example:
let arr = ["Hello", "world"];
arr.join(); // Returns "Hello,world"
Here, it just puts a comma between "Hello" and "world".
You can choose to have nothing between them like this:
arr.join(""); // Returns "Helloworld"
Nested Arrays
If your array has other arrays inside it (we call these nested arrays), join()
will also combine those inner lists into the string.
For example:
let nested = [["Hello"], ["world"]];
nested.join(); // Returns "Hello,world"
This means it looks inside each nested array and joins those elements too.
So, join()
goes through your list, puts your chosen separator between items, and makes one big string out of it. It's a straightforward tool in JavaScript for making lists easier to work with.
Common Questions
Using a Custom Separator
You can pick your own separator when you use the join()
method. This means you can decide what goes between each item in your list when you turn it into a string.
Here's a simple example:
let fruits = ["apple", "banana", "orange"];
let fruitsString = fruits.join(" + ");
console.log(fruitsString); // "apple + banana + orange"
By choosing " + " as the separator, it gets placed between each fruit in the final string.
You can really use any text you want as a separator. Whether it's commas, spaces, plus signs, or something else, it's up to you.
Handling Null Values
If your list has null
or undefined
spots, the join()
method just ignores them and doesn't add anything extra in their place.
For example:
let arr = ["Hello", null, "world"];
arr.join(""); // "Helloworld"
This means if there are empty spots in your list, they won't mess up your final string. They're just skipped over.
Browser Compatibility
The join()
method works in pretty much every web browser, including all the new ones and even old ones like Internet Explorer 6. It works on computers and phones.
This means you can use join()
in your website or app, and almost everyone who visits will be able to use it without any problems. It follows the rules set by the ECMAScript Language Specification, which is a big deal in the world of web development.
sbb-itb-bfaad5b
Practical Examples
Sentence Creation
Let's look at a simple way to use the join() method. Imagine you have a bunch of words in an array, and you want to turn them into a full sentence:
let words = ["This", "is", "a", "simple", "sentence"];
let sentence = words.join(" ");
console.log(sentence); // "This is a simple sentence"
By choosing a space (' ') as the separator, we make sure there's a space between each word, just like in a normal sentence.
You can even create whole paragraphs like this:
let paragraphWords = [
["This", "is"],
["a", "simple"],
["paragraph", "created", "with", "join()"]
];
let paragraph = paragraphWords.map(sentence => sentence.join(" ")).join(". ");
console.log(paragraph);
// "This is. a simple. paragraph created with join()."
Here, we first join words in each smaller array to make sentences. Then, we join those sentences with a period and a space ('. ') to form a paragraph.
URL Building
Join() is also super useful for putting together URL paths. Here's how you can do it:
let urlSections = ["help", "faq", "javascript", "join"];
let url = urlSections.join("/");
// "help/faq/javascript/join"
This turns our array into a neat URL path, separating each part with a slash ('/').
And for a more complete URL example:
let urlParts = [
"https://www.example.com",
"topics",
"javascript",
"join-method"
];
let fullUrl = urlParts.join("/");
// https://www.example.com/topics/javascript/join-method
This way, join() helps us build the whole URL by adding slashes between the parts. It's a handy tool for making URLs out of JavaScript arrays.
Conclusion
The join()
method is super useful for anyone coding in JavaScript. It helps you change a list of things into a single line of text. Here's what to remember:
join()
makes a string from an array by putting a separator, like a comma, between each part. You can pick any separator you want, though.- It's great for a bunch of tasks. You can make sentences, create links for websites, put together data for logs, and lots more. It's a really handy tool for working with lists.
join()
works on all the browsers people use today, and even on really old ones. It's based on the ECMAScript Language Specification, which is the official rulebook for JavaScript.- If you have lists within lists,
join()
smoothes them out into one string. And if there are any empty spots in your list, likenull
values, it just skips them. - You get to choose what goes between the items in your list. Use spaces for sentences, slashes for web addresses, or whatever fits your need.
Getting good at using join()
will really help you out, whether you're showing text to users, sending data somewhere, making web addresses, or just organizing info. It's a tool you'll find yourself using a lot once you know how it works.
Related Questions
How does join () work in JavaScript?
The join()
method in JavaScript combines all the pieces of an array into one string. It puts something called a separator between each piece. By default, this separator is a comma, but you can choose whatever you like, such as a space or a dash.
For example:
let arr = ["Hello", "world"];
arr.join(); // "Hello,world" (uses a comma by default)
arr.join(""); // "Helloworld" (no separator)
arr.join(" "); // "Hello world" (uses a space as a separator)
In short:
- It turns array elements into a string
- Uses a comma between elements by default
- You can choose any separator you like
The original array stays the same. join()
just gives you a new string.
How to join an array of strings into a single string in JavaScript?
To turn an array of strings into one big string, just use the join()
method:
let strings = ["Hello", "world", "join", "method"];
let result = strings.join(" ");
// Result: "Hello world join method"
Here's how you do it:
- Start with an array full of strings
- Use
join()
on the array - Give
join()
a separator string (like a space) - It returns a single string with everything joined together
You can use any separator you want, like a comma or a dash, to connect the words.
How to split string and join in JavaScript?
To split a string into pieces and then join them back together, use split()
first, then join()
:
let text = "Hello world";
// Split into pieces
let textArr = text.split(" ");
// Join back into one string
let result = textArr.join("-");
// Result: "Hello-world"
Here's the step-by-step:
- Start with a string
- Use
split()
to break it into an array by choosing a spot to split (like a space) - Use
join()
to put the array back into a string, choosing a new spot (like a dash) to connect them
This is a neat trick to change how your string looks or to switch out separators.
How do you join array elements into a string?
To join elements of an array into a single string in JavaScript, you use the join()
method. You tell it what separator to use, and it puts that between each element as it combines them:
let fruits = ["apple", "banana", "orange"];
let str = fruits.join(" | ");
// Result: "apple | banana | orange"
Here are the steps:
- Make an array with your items
- Use
join()
on your array - Give
join()
a separator string (like " | ") - It turns your array items into one string
If you don't pick a separator, join()
will use a comma. But you can choose whatever you like to fit your needs.