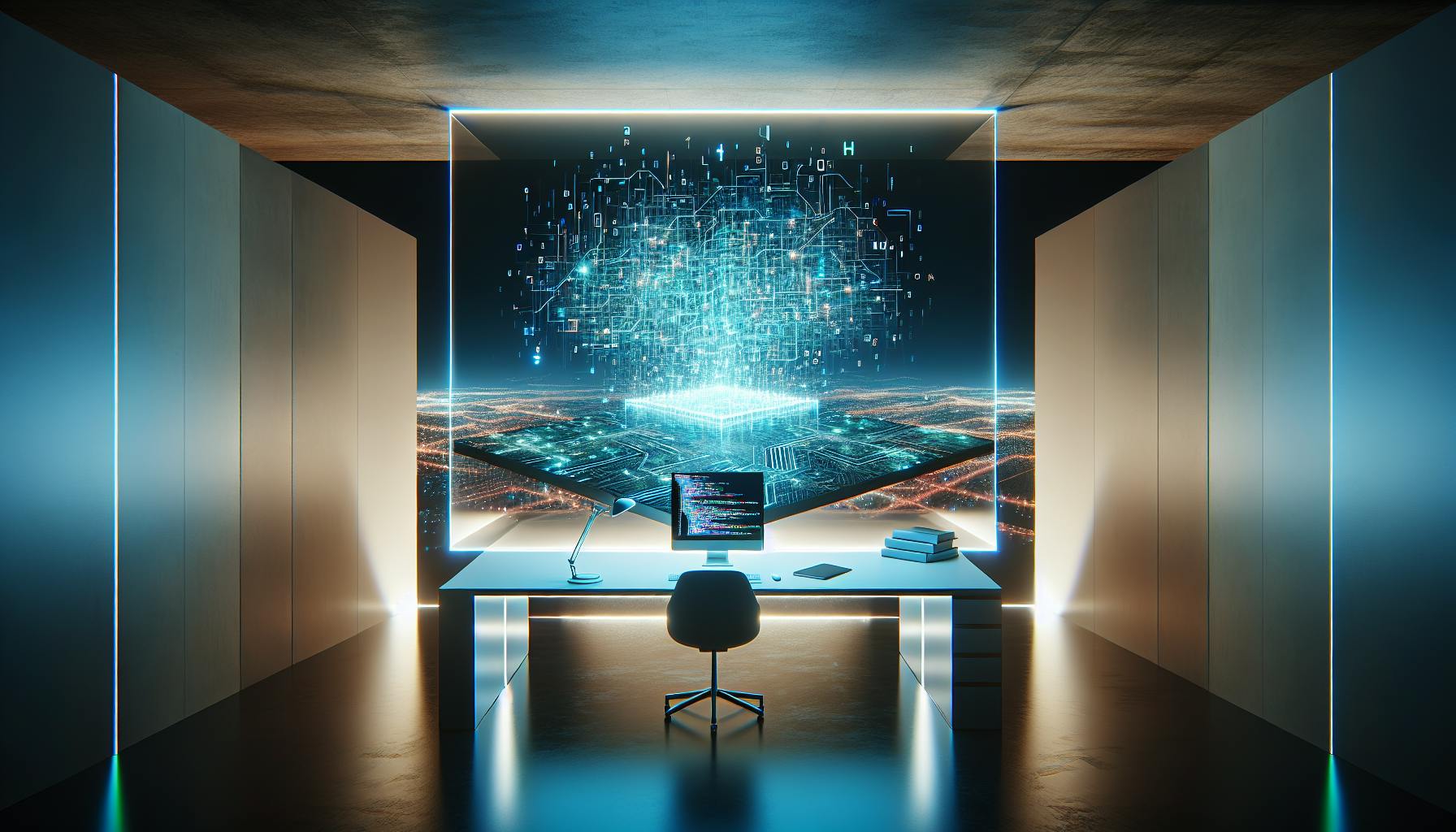
Learn how to populate arrays in JavaScript from basics to advanced techniques. Discover array creation, population methods, performance tips, best practices, real-world applications, and more.
Filling arrays in JavaScript is a fundamental skill for developers, enabling you to manage and manipulate data efficiently. Whether you're a beginner or looking to advance your knowledge, this guide covers everything from basic methods to more sophisticated techniques. Here's a quick overview:
- Basics of Arrays: Learn what arrays are and how to create them.
- Simple Methods: Populate arrays using array literal notation, loops, and methods like
.push()
and.unshift()
. - Advanced Techniques: Dive into
.fill()
,.from()
,.map()
, and the spread operator for more complex scenarios. - Special Patterns: Create sequential, multidimensional, and object arrays.
- Performance Tips: Understand how different methods impact performance.
- Best Practices: Avoid common mistakes and adopt best practices for efficient coding.
- Real-world Applications: Discover how arrays are used in tasks like managing API data and creating photo galleries.
This guide aims to make you proficient at populating arrays in JavaScript, equipping you with the knowledge to handle data in various ways.
What is an Array?
An array in JavaScript is like a big box where you can keep a bunch of different things together under one name. You can put stuff like words, numbers, or even other boxes inside, and each one has a special number so you can find it easily later.
Some important things to know about arrays:
- You can put lots of different things in them, like text, numbers, true or false values, and more.
- They start counting from 0. So the first item is at 0, the second at 1, and so on.
- You can change what's inside an array anytime, adding or taking things out, and it can grow or shrink as needed.
- It's really quick to get to any item if you know its number.
- Arrays come with handy tools like
push()
andpop()
to add or remove items easily.
Arrays are great for when you want to:
- Keep a bunch of related things together, like a shopping list or names of places to visit.
- Have a list of items to go through one by one, like photos in an album.
- Share a bunch of data between different parts of your program.
- Make simple 'to-do' or 'done' lists.
- Be more organized than having lots of separate variables for each thing.
Creating Arrays
There are two easy ways to make an array in JavaScript:
- Array Literal Notation - This is the usual way. You just list what you want in the array inside square brackets
[]
.
let fruits = ['Apple', 'Banana', 'Mango'];
- Array Constructor - This is another way, using a special command called the Array constructor with the
new
keyword.
let vehicles = new Array('Car', 'Bus', 'Truck');
Key Points on Array Creation:
- It's generally better to use the square brackets
[]
than the Array constructor. - You can start with an empty array and add things later.
- Using
Array(number)
creates an array with that many spots, but they're all empty to start with. - You can put arrays inside other arrays to make a multi-layered list.
Chapter 2: Basic Techniques to Populate Arrays
Filling up an array means putting items into it. Let's look at some simple ways to do this in JavaScript:
Method 1: Using Array Literal Notation
The simplest way to fill an array is by listing the items you want right when you make the array. This is called array literal notation:
let numbers = [1, 2, 3, 4, 5];
You just put the values you want between square brackets, separated by commas. This fills the array with these items from the start.
Some key points:
- You can put different types of items together, like text, numbers, or true/false values.
- The array's size is determined by how many items you put in.
- You can get to any item quickly if you know its position.
This method is perfect for when you have a clear list of items to add.
Method 2: Using a For Loop
Another way to fill an array is by using a for loop
:
let fruits = [];
for (let i = 0; i < 5; i++) {
fruits.push('Apple');
}
Here, we:
- Start with an empty array
fruits
- Use a for loop to run 5 times
- Add an 'Apple' to the array each time through the loop
This method lets you fill an array step by step.
Some key points about loops:
- You can add different items each time through the loop.
- Loops make it easy to create arrays that are as big as you need.
- You can use more complex rules inside the loop to decide what to add.
Loops are great for when you need to fill an array in a more controlled way.
Method 3: Using Array Methods (push, unshift)
You can also use built-in methods like .push()
and .unshift()
to add items:
let languages = [];
languages.push('JavaScript'); // Adds to the end
languages.unshift('Python'); // Adds to the start
.push()
puts elements at the end of an array.unshift()
puts elements at the start of an array
These methods change the original array.
Some key points:
- A simple way to add items without using a loop
- These methods are clear about what they do (adding to the array)
- You can use them one after another to add lots of items quickly
So, to sum up, use the literal method for a quick list of items, loops for more control, and methods like push/unshift for easy additions.
Chapter 3: Advanced Array Population Techniques
This chapter dives into some cooler ways to fill up arrays in JavaScript. We're moving past the simple stuff and looking at some powerful tools like fill()
, from()
, map()
, and the spread operator to make arrays do exactly what we want.
Method 1: Using the fill() Method
Imagine you have a box and you want everything in it to be the same thing, like all apples. The fill()
method does just that for arrays.
For example:
let array = new Array(5).fill(0);
// This makes [0, 0, 0, 0, 0]
This means you make an array with 5 spots, all filled with 0
.
Here's what to remember about fill()
:
- It can fill up the whole array with whatever you choose.
- You can start filling from a certain spot or stop before the end.
- It changes the array right away, putting in new stuff and getting rid of the old.
Method 2: Using the from() Method
Array.from()
is like a magic trick that turns things that aren't arrays into arrays. Like making an array from a string.
For example:
let str = "Hello";
let charArray = Array.from(str);
// Turns into ["H", "e", "l", "l", "o"]
What's cool about from()
:
- It works on things like strings and makes them into arrays.
- You can also change each item as you add it to the array.
- It's great for when you have things like a list of webpage elements (nodeLists) and you want them in an array.
Method 3: Using the map() Method
map()
is a way to go through an array and change each thing in it, one by one, using a special rule you set.
For example:
let numbers = [1, 2, 3];
let doubled = numbers.map(x => x * 2);
// Now doubled is [2, 4, 6]
Here's the deal with map()
:
- It goes through each item, applies your rule, and makes a new array with the results.
- The original array stays the same.
- It's super useful for changing data or getting it ready to show.
Method 4: Using Spread Operator
The spread operator ...
lets you take all the items from one array and spread them out into another.
For example:
let arr1 = [1, 2, 3];
let arr2 = [...arr1, 4, 5];
// arr2 is now [1, 2, 3, 4, 5]
What to know about the spread operator:
- It's a quick way to get all elements from one array into another.
- It works with other things that can be spread out, not just arrays.
- Great for mixing arrays together or copying them.
So, fill()
is awesome for making everything the same, from()
changes non-arrays into arrays, map()
lets you change each item in an array, and the spread operator helps combine or copy arrays.
Chapter 4: Creating Arrays with Specific Patterns
Creating Sequential Arrays
You can make arrays that follow a simple number order using a couple of smart ways:
Array.from()
The Array.from()
method helps you create an array that goes in order. For example:
let sequence = Array.from({length: 5}, (_, i) => i);
// Returns [0, 1, 2, 3, 4]
This method counts from 0 up to the length you want, adding each number to the array.
Spread Operator
Another way is using the spread syntax with Array()
to create number sequences:
let numbers = [...Array(5).keys()];
// Returns [0, 1, 2, 3, 4]
This method spreads out the indexes into an array.
Key Points:
- Great for making lists of numbers in order
- Allows you to create sequences easily
- Can be used with other array methods
Creating Multidimensional Arrays
For arrays within arrays:
let matrix = [[1, 2], [3, 4], [5, 6]];
This creates a 2D array. You can make arrays with more dimensions too.
To get items from these arrays:
let firstSubArray = matrix[0];
// Returns [1, 2]
let item = matrix[1][0];
// Returns 3
Key Points:
- Good for organizing related data
- Use multiple indexes to access items
- Easy to loop through all the values
Creating Arrays of Objects
Arrays can hold objects too:
let people = [
{name: "Alice"},
{name: "Bob"}
];
To get to the object properties:
let firstPerson = people[0];
console.log(firstPerson.name); // Prints "Alice"
Why Use Objects in Arrays?
- Helps keep complex data organized
- Makes it easy to work with and access data
- Offers more options than simple arrays
Key Points:
- You can store objects, not just simple items
- Great for dealing with complex information
- Lets you access data through properties
Chapter 5: Performance Considerations
When you're filling up arrays in JavaScript, it's smart to think about how your choices affect how fast your code runs and how smoothly it works. Picking the right way to add or take out items can make your code work better.
In this chapter, we'll look at some common ways to work with arrays and see which ones are quicker or use less memory. Knowing this helps you choose the best method for what you need.
Comparing push/pop versus shift/unshift
There are a couple of ways to add items to or remove them from an array:
.push()
and.pop()
- for adding or removing at the end.unshift()
and.shift()
- for adding or removing at the start
But which is quicker? Let's compare:
Operation | push/pop | unshift/shift |
---|---|---|
Add item | Very fast O(1) | Slow O(n) |
Remove item | Very fast O(1) | Slow O(n) |
Explanation:
.push()/pop()
let you add or take away items at the end of an array quickly, no matter how many items are in the array..unshift()/shift()
do the same at the start of an array. But, every time you do this, all the other items need to move one spot. This takes longer the more items you have.
Conclusions:
- It's usually better to use
.push()/pop()
because they're faster for adding or removing items one by one. - Choose
.unshift()/shift()
only if you need to work at the start of the array.
So, adding and taking away items is much quicker at the end than at the start of an array. Knowing this can help you make your code run more efficiently.
sbb-itb-bfaad5b
Chapter 6: Best Practices and Common Mistakes
When you're working with arrays in JavaScript, it's smart to stick to some rules and try to avoid certain slip-ups. This can help your programs run better, keep away bugs, and make your code neater. Here are some key tips:
Use Literal Notation
It's generally better to make arrays using square brackets instead of using the Array constructor:
// Good
const arr = [];
// Avoid
const arr = new Array();
Using square brackets is easier and quicker.
Check Array Length
Before adding items to an array, make sure there's enough space:
if(arr.length < 10) {
arr.push(item);
}
This helps you avoid errors from the array getting too full.
Copy Arrays Properly
If you want to make a copy of an array, don't just assign it to a new variable:
const arr2 = arr1; // This doesn't actually make a copy
Instead, use methods like Array.from(), slice(), or the spread operator:
const arr2 = Array.from(arr1);
const arr2 = arr1.slice();
const arr2 = [...arr1];
These methods create a new array that's separate from the original.
Avoid Index Gaps
When taking items out, use methods like .pop()
, .shift()
, or .splice()
instead of deleting by index. This prevents empty spots:
// Leaves empty spots at index 2
delete arr[2];
// Removes items without leaving empty spots
arr.splice(2, 1);
Empty spots can cause problems and make your array behave weirdly.
Handle Sparse Arrays
Arrays with empty spots are called "sparse arrays". Some array methods might skip these empty spots, which can mess things up. To clean out the gaps, use .filter(Boolean)
:
sparseArr = sparseArr.filter(Boolean);
This makes your array neat and tidy, so everything works as expected.
By sticking to these tips, you can avoid common mistakes, boost performance, and make your code cleaner. The biggest mistakes usually involve not copying arrays correctly, leaving gaps, or not dealing with sparse arrays right. Steering clear of these issues will make your coding life much easier.
Chapter 7: Real-world Applications
Using arrays is super important when building websites. Here are some everyday ways they come in handy:
Storing API Data
When we get data from an API, it's usually in JSON format. We can turn this data into arrays to make it easier to work with in JavaScript:
// Get data from API
const apiData = getData();
// Turn it into an array
const dataArray = Array.from(apiData);
Creating Photo Galleries
For photo galleries, we keep the image files in an array. This helps us set up the gallery on the page:
const images = [
"img1.jpg",
"img2.jpg",
//...
];
displayGallery(images);
Generating Tables of Data
We use tables to organize info. By using a 2D array (an array of arrays), we can easily create tables for websites:
const data = [
[ "Product", "Price" ],
[ "Apples", 2.99 ],
[ "Oranges", 3.50 ],
];
buildTable(data);
Managing Todo Lists
Todo list apps are all about adding, checking off, and removing tasks. We store these tasks in an array and change it as needed:
let todos = [];
function addTodo(todo) {
todos.push(todo);
}
function completeTodo(index) {
todos[index].completed = true;
}
Paginating Content
To split content across different pages, we use arrays to hold the data for each page. This makes it easy to show the right page's content:
const itemsPerPage = 10;
const content = [
page1Data,
page2Data,
//...
]
These examples show how arrays can help us sort and show data in different web development projects. The techniques we've talked about are key to making these tasks easier.
Conclusion
Filling up arrays with data is a key skill for anyone coding in JavaScript. This guide has shown you many ways to do this, from the simple to the more complex.
Starting with basics like using square brackets or loops, and adding items with .push()
or .unshift()
, gives you a straightforward way to build your arrays piece by piece.
Then, we moved on to more advanced tricks that came with ES6, like the .fill()
, .from()
, .map()
methods, and using the spread operator. These tools offer more control, letting you turn things that aren't arrays into arrays, change data in specific ways, or merge arrays together smoothly.
We also covered how to organize data in more complex ways with multi-dimensional arrays or arrays that hold objects. Plus, we talked about how to make your code run faster by choosing the right methods for adding or removing items.
Real-life uses for arrays include working with data from the internet, setting up photo galleries, making tables, managing lists of tasks, and splitting up content into pages. These are all important for making websites.
The main point is to know what each method does best and use the right one for your task. Trying out these different ways to work with arrays in your projects is a great idea.
By understanding the basics and getting creative with the newer tools, you'll be able to use arrays in JavaScript like a pro. So go ahead, start filling those arrays, and create something cool!
Appendix: Further Reading
If you're keen to dive deeper into JavaScript arrays, here are some easy-to-follow resources that could be really helpful:
Articles and Tutorials
- JavaScript Array Handbook by Flavio Copes - This guide is packed with everything you need to know about arrays, including how to use different methods, sort items, and much more. It's written in a way that's easy to understand.
- Modern Ways of Filling Arrays in JavaScript by Preethi Kasireddy - This article talks about some of the newer ways to work with arrays, like using the
fill()
,from()
, andmap()
methods. It's a great read if you want to learn some cool tricks. - Multi-dimensional Arrays in JavaScript - This piece breaks down how to work with arrays that have more than one level, using clear examples to show you how it's done.
Video Tutorials
-
JavaScript Array Superpowers - A series of short videos that show off what you can do with advanced array methods. It's like a quick workout for your coding skills.
-
Array Cardio by Wes Bos - A set of engaging exercises to help you get better at using arrays. It's a fun way to practice.
-
Arrays and Objects in JavaScript by Programming with Mosh - A longer tutorial that covers both arrays and how they can hold objects. It's really informative and easy to follow.
Documentation
-
MDN Arrays Reference - This is the go-to place for everything about arrays. It's detailed and has everything you need.
-
Arrays on JavaScript.info - Another excellent resource for learning about arrays. It comes with lots of examples to help you understand better.
These resources are great for getting a stronger grip on working with arrays. The best way to get better is by trying out what you learn in your own projects.
Related Questions
How to dynamically populate array in JavaScript?
To add items to arrays on the go in JavaScript, you can use methods like:
Array.from()
- This turns something like a string into an array.fill()
- This fills the array with the same value everywhere.map()
- This changes each item in an existing array into something new and puts those into a new array.push()/pop()
- These let you add or remove items from the end of the array.
For instance:
// Using Array.from()
let arr = Array.from("hello");
// Using fill()
let filled = new Array(3).fill(0);
// Using map()
let mapped = [1, 2, 3].map(x => x * 2);
// Using push()
let arr = [];
arr.push("new value");
These methods help you fill arrays without having to list all the values from the start.
How to populate array data in JavaScript?
To put data into arrays in JavaScript, you can:
- Use array literal notation:
let fruits = ["Apple", "Banana", "Orange"];
- Use loops:
let nums = [];
for (let i = 0; i < 5; i++) {
nums.push(i);
}
- Use methods like
.fill()
,.from()
:
let arr = new Array(5).fill(0);
let arr2 = Array.from("text");
- Use the spread syntax:
let arr1 = [1, 2, 3];
let arr2 = [...arr1, 4, 5];
Choose the method that fits your need - literal notation for simple lists, loops for adding items one by one, and spread syntax for combining arrays.
What is the fastest way to fill an array in JavaScript?
The .fill()
method is super quick for filling arrays in JavaScript. Like this:
let arr = new Array(100000).fill(0);
This creates a big array and fills it with zeros really fast.
Key things about .fill()
:
- It's quicker than using loops or
.map()
- Made for filling big arrays fast
- Only puts in the same value everywhere
So if you need a large array filled with the same thing, .fill()
is your go-to for speed.
How to populate an array with another array in JavaScript?
To put one array's items into another array, you can:
Use the spread syntax:
let arr1 = [1, 2, 3];
let arr2 = [...arr1, 4, 5];
Use concat():
let arr1 = [1, 2, 3];
let arr2 = arr1.concat([4, 5]);
Use slice():
let arr1 = [1, 2, 3];
let arr2 = arr1.slice();
Use map():
let arr1 = [1, 2, 3];
let arr2 = arr1.map(x => x);
The spread syntax is the easiest and most flexible way. concat()
and slice()
are also good for adding arrays together.