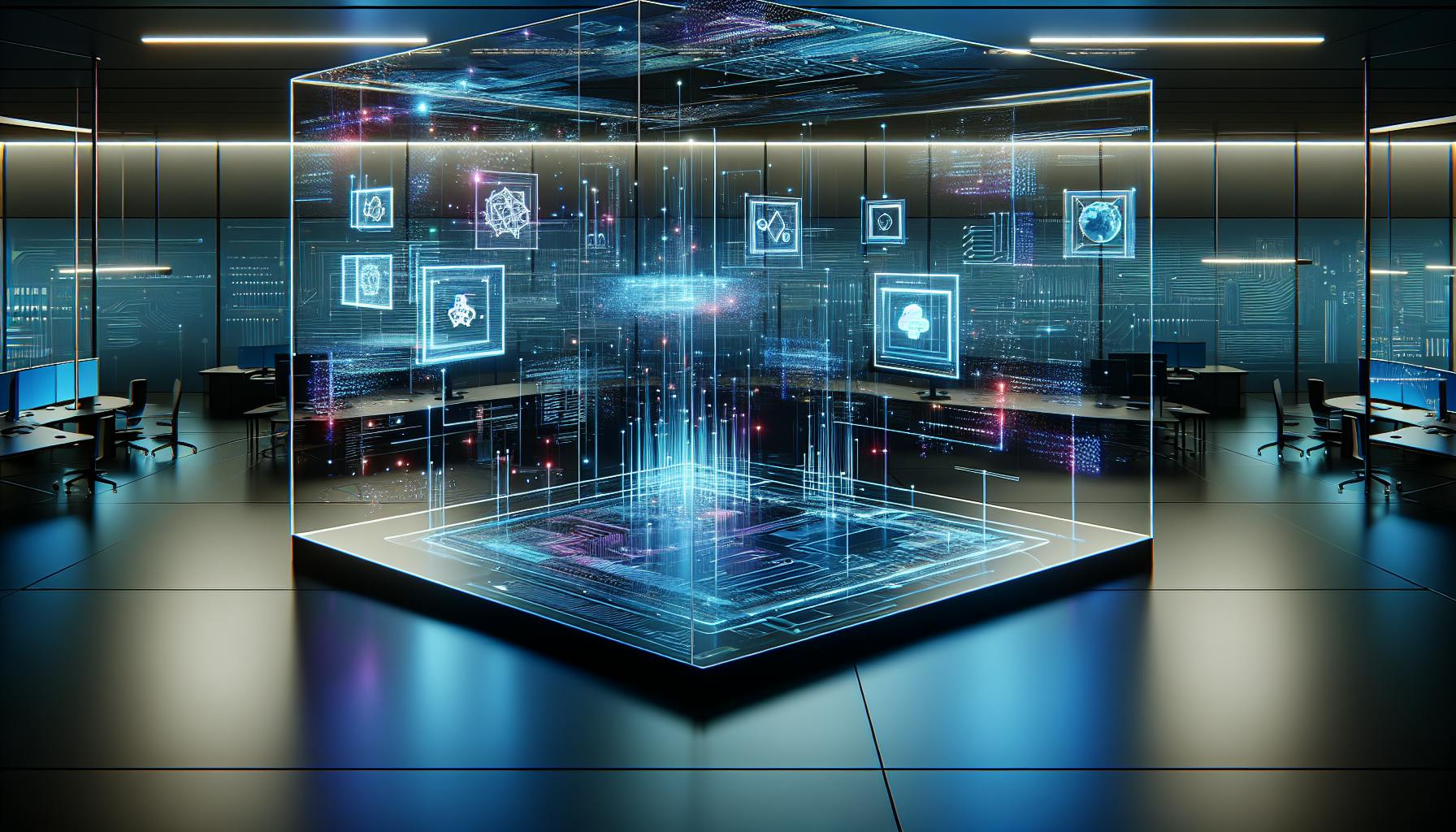
Explore advanced React topics for senior developers in 2024, including component patterns, React Hooks, state management, performance optimization, testing strategies, security practices, and future trends.
If you're eyeing a senior React developer position in 2024, you'll need to master not just the fundamentals like component handling, state management, and hooks, but also advanced concepts to tackle big React projects efficiently. This guide dives deep into what makes a senior React developer stand out:
- Advanced component patterns, such as Higher Order Components, Render Props, and Compound Components
- A deep dive into React Hooks, including custom hooks and performance optimization techniques like
useCallback
- State management tools comparison: Redux, MobX, Context API
- Performance enhancements through code splitting, lazy loading,
React.memo
, andPureComponent
- React ecosystem tools like TypeScript, GraphQL, Next.js
- Testing strategies spanning from unit to visual regression tests
- Security best practices for React applications
- Managing large-scale applications with smart code organization and efficient state management
- Keeping up with React's future trends like React Native, server-side rendering, and WebAssembly
This overview encapsulates the critical areas of focus for a senior React developer, highlighting the blend of technical prowess, best practices, and forward-thinking required to excel in 2024.
Advanced Component Patterns
React lets you create flexible and reusable UI components in several smart ways:
Higher Order Components (HOCs)
Think of HOCs as special functions that take a component and give it some extra features. Here's a quick example:
function withAuth(WrappedComponent) {
return class extends React.Component {
// custom auth logic
render() {
return <WrappedComponent {...this.props} />
}
}
}
// Usage
const AuthButton = withAuth(Button);
They're great for adding common features across different components, like user authentication.
Render Props
This pattern lets you pass a function as a prop that returns some UI. It's a handy way to share code:
<DataProvider render={data => (
<h1>Hello {data.name}</h1>
)}/>
It's all about sharing functionality in a flexible way.
Compound Components
This approach helps you build complex interfaces with simpler parts working together:
function FancyButton({ children }) {
return (
<button>
<StartDecoration/>
{children}
<EndDecoration/>
</button>
);
}
It's useful for keeping related components together.
React Hooks Deep Dive
Let's talk about some advanced ways to use React hooks:
Custom Hooks
With custom hooks, you can write your own hooks to share logic across components without needing HOCs or render props:
function useAuth() {
const [user, setUser] = useState(null);
// custom auth logic
return user;
}
useCallback
This hook helps you avoid unnecessary re-renders by remembering functions:
const memoizedCallback = useCallback(() => {
// callable logic
}, [])
useContext
This lets you use context values easily without having to pass them down through props:
const user = useContext(UserContext);
State Management in React
Here are some popular tools for managing state:
Library | Description | Use Case |
---|---|---|
Redux | Central store, immutable state, actions/reducers | Complex state flows |
MobX | Observable state, computed values | Decentralized state |
Context API | Built-in context sharing | Simple global state |
Each of these tools has its own pros and cons, depending on what you need for your project.
React Performance Optimization
Code Splitting and Lazy Loading
Code splitting and lazy loading are important for making React apps run faster, especially when they first start. The idea is to break your app into smaller pieces and only load the parts a user needs right when they need them.
Here are some simple tips:
- Use
React.lazy
andSuspense
to load components only when they're needed - Break your code into smaller pieces by routes or components with tools like Webpack
- Load parts of your app that aren't immediately seen (like stuff at the bottom of the page) later
- Get assets ready that you'll probably need soon
- Use
import()
to load modules without blocking the rest of your app - Load less important JS chunks after everything else is up and running
Lazy loading means your app doesn't load everything at once. This makes your app faster to start and use less data, which is especially good for mobile users.
By using smart loading strategies, your app feels quicker because pages load faster and moving around feels smoother since needed parts might already be loaded from before.
React.memo and PureComponent
React.memo
and React.PureComponent
help stop your app from doing extra work by only updating components when it really needs to.
// React.memo
const MyComponent = React.memo(function MyComponent(props) {
/* it won't rerender unless props change */
});
// React.PureComponent
class MyComponent extends React.PureComponent {
/* it won't rerender unless props or state change */
}
When to use them:
- Use
React.memo
for functions that don't change unless the props do - Use them for big parts of your app, like lists, that are slow to render
- They can help make your app run smoother by not updating everything all the time
Things to remember:
- They only check changes in a simple way, so they might not work well with complex data
- Sometimes you need to tell them how to compare things with
areEqual
React.memo
is usually easier for simple checks, but PureComponent
is there for class components.
Using these tools wisely can make your app run much better by not wasting time re-rendering stuff that hasn't changed.
React Ecosystem
Integration with Other Technologies
React often works alongside other tools and frameworks:
- TypeScript - This is JavaScript but with types. It helps catch mistakes early and keeps your code clean.
- GraphQL - A way to ask for data from your server. It lets you get exactly what you need in one go. Tools like Apollo Client make it easy to use with React.
- Next.js - A framework built on React that makes pages load faster and helps with SEO. It's great for projects that need to be fast and easily found on the web.
- Redux/MobX - These are tools for keeping track of your app's data. Redux has a single store for all your data, while MobX watches data and updates your app automatically.
Mixing React with these technologies needs a solid grasp of React basics to avoid common pitfalls. Tools like Next.js make things simpler, while TypeScript and GraphQL can make development smoother.
Testing Strategies for React Applications
Testing your React app well is key to making sure it works right. Here are some ways to do it:
- Unit Testing - Tests small parts of your app to find problems early. Tools like Jest and React Testing Library are good for this.
- Integration Testing - Checks how different parts of your app work together. You can simulate user actions with tools like Cypress.
- End-to-End (E2E) Testing - Tests the whole app as if a real user is using it. Tools like Selenium can automate this.
- Visual Regression Testing - Takes pictures of your UI and compares them over time to catch unexpected changes.
Using mocks for outside services, testing the logic behind your app, keeping an eye on test coverage, and testing before you launch can all help.
A mix of unit, integration, E2E, and visual tests can make your app more reliable and keep bugs at bay.
sbb-itb-bfaad5b
Advanced Topics
Security Practices in React Applications
When building React apps, it's important to keep them safe from common web dangers like XSS (where attackers inject harmful scripts), CSRF (where attackers trick users into doing things they didn't intend), and other injection attacks. Here's how to stay safe:
- Check and clean all user input. Use tools to make sure the data users send to your app is safe.
- Escape user output with specific libraries. This stops XSS by making sure any data shown to users doesn't include harmful scripts.
- Use HTTP security headers. Headers like Content-Security-Policy help prevent XSS and data injection.
-
Handle user sessions and tokens carefully:
- Use cookies that only send data over HTTPS
- Keep tokens in
localStorage
safely - Make tokens expire after a while
- Secure your API routes
- Keep secrets secret. Don't put sensitive info like keys in your code or git. Use environment variables instead.
- Limit what users can do based on their role with something called RBAC.
- Use Helmet for setting security headers easily.
Always check for vulnerabilities, update your code dependencies regularly, and follow good security habits everywhere in your app.
Handling Large-scale Applications
For big React projects, here are some tips:
- Break your code into small, reusable pieces. This makes your code easier to manage.
- Organize your files by features or routes. This helps keep related files together.
- Use Redux/MobX for shared state to avoid passing data through many layers. Use Context for simpler cases.
- Split your code and load parts as needed with React.lazy. This makes your app faster to load.
- Agree on how to do things like managing state or testing. This keeps everyone on the same page.
- Check types with TypeScript to catch bugs early.
- Automate testing to catch problems before they hit users.
- Set up CI/CD to automate tests and deployments.
- Keep an eye on performance and fix slowdowns.
- Write down how things should be done so everyone knows what to do.
With organized code, smart state management, and good habits, big React apps can be easier to handle.
React Future Trends
Here are some things that might be big for React soon:
- React Native getting more popular: Sharing code between web and mobile apps saves time.
- More TypeScript: It helps find bugs before your code runs.
- More server-side rendering: SSR makes your pages show up better in search results. Tools like Next.js help a lot.
- WebAssembly: This could make React apps run faster, almost like native apps.
- Component libraries: Tools like Storybook help manage and share UI pieces.
- Better testing tools: Expect easier ways to write and run tests.
- Concurrent React: This new feature could make apps run smoother by managing resources better.
React's way of building apps should keep it at the forefront, with improvements in mobile development, types, SSR, WebAssembly, testing, and performance.
Conclusion
React has really changed the game since it first came out, and it's always getting new updates and features. If you're aiming to be a top-notch React developer in 2024, it's super important to keep up with all the new stuff coming out.
Here are some simple tips to keep in mind:
- Understand React inside and out: Make sure you know the basics like how components work, but also get into the nitty-gritty of things like making your app run faster, testing it, and managing its state.
- Keep learning: Always check out the latest updates from React, try out new features before they're officially out, and keep playing around with new tools.
- Learn more than just React: Picking up skills in related areas like TypeScript, Next.js, and GraphQL can make you even more valuable.
- Watch for new trends: Keep an eye on things that are becoming more popular, like server-side rendering (SSR), WebAssembly, and new React features.
- Practice makes perfect: React is always changing, so the more you use it, the better you'll get.
- Help others learn: Sharing what you know by teaching, blogging, or helping out in the community not only helps others but also makes you better.
- Aim high: Don't just learn how to use React. Try to really understand how it works. This will make you a much stronger developer.
The React world is only going to keep growing. If you're willing to keep learning and sharing what you know, there are going to be a lot of exciting opportunities waiting for you. React's future is looking really bright!
Related Questions
How do I prepare for a senior React developer interview?
To get ready for a senior React developer interview, make sure you know the deeper parts of React like:
- How React updates what you see on the screen
- Managing complex data with tools like Redux and MobX
- Making your app run faster with tricks like remembering calculations, loading only what's needed, and splitting your code
- Different ways to test your app, from checking small parts to testing the whole thing
- Keeping your app safe from common attacks
- Building big apps in a smart way, so you can use parts again and everything stays organized
Also, go over basic programming concepts like how to organize data and solve problems. Look back at your React projects, and be ready to talk about tough spots you worked through.
What questions should I ask a React developer?
When talking to someone who might join your React team, consider asking:
- How do you make React apps run smoother?
- When would you pick React context over Redux?
- How have hooks changed the way components work?
- What's your approach to testing components?
- How do you manage data in big apps?
- What's your plan for starting a new React project?
- How do you make components that you can use again?
Asking about their past projects helps you understand how they solve real problems with React.
How do I prepare for a React technical interview?
Getting ready for a React technical interview means:
- Going through React's guides and making example apps
- Practicing with mock interviews on React and basic programming
- Brushing up on programming basics like organizing data and algorithms
- Learning the tougher parts of React like making your app efficient, testing, and managing data
- Looking back at your projects to talk about your coding decisions
- Learning about the company's technology and preparing for that
- Thinking of questions about how they build software and work as a team
Building things with React is the best way to get better. Focus on areas you're not as strong in.
What is component in React interview questions?
In React, a component is a piece of the screen that you can use over and over. It lets you split your app into smaller parts that can work together. Here are some examples:
<Header />
- Shows the top part of your app like the logo and menu<ProductList />
- Displays products<Footer />
- Shows the bottom part of your app like links
Components have:
- Props - Data you can give to a component so it knows what to show
- State - Data that can change inside the component
- Lifecycle Methods - Special functions that run at certain times
Components help organize your app by breaking it into smaller, reusable pieces.