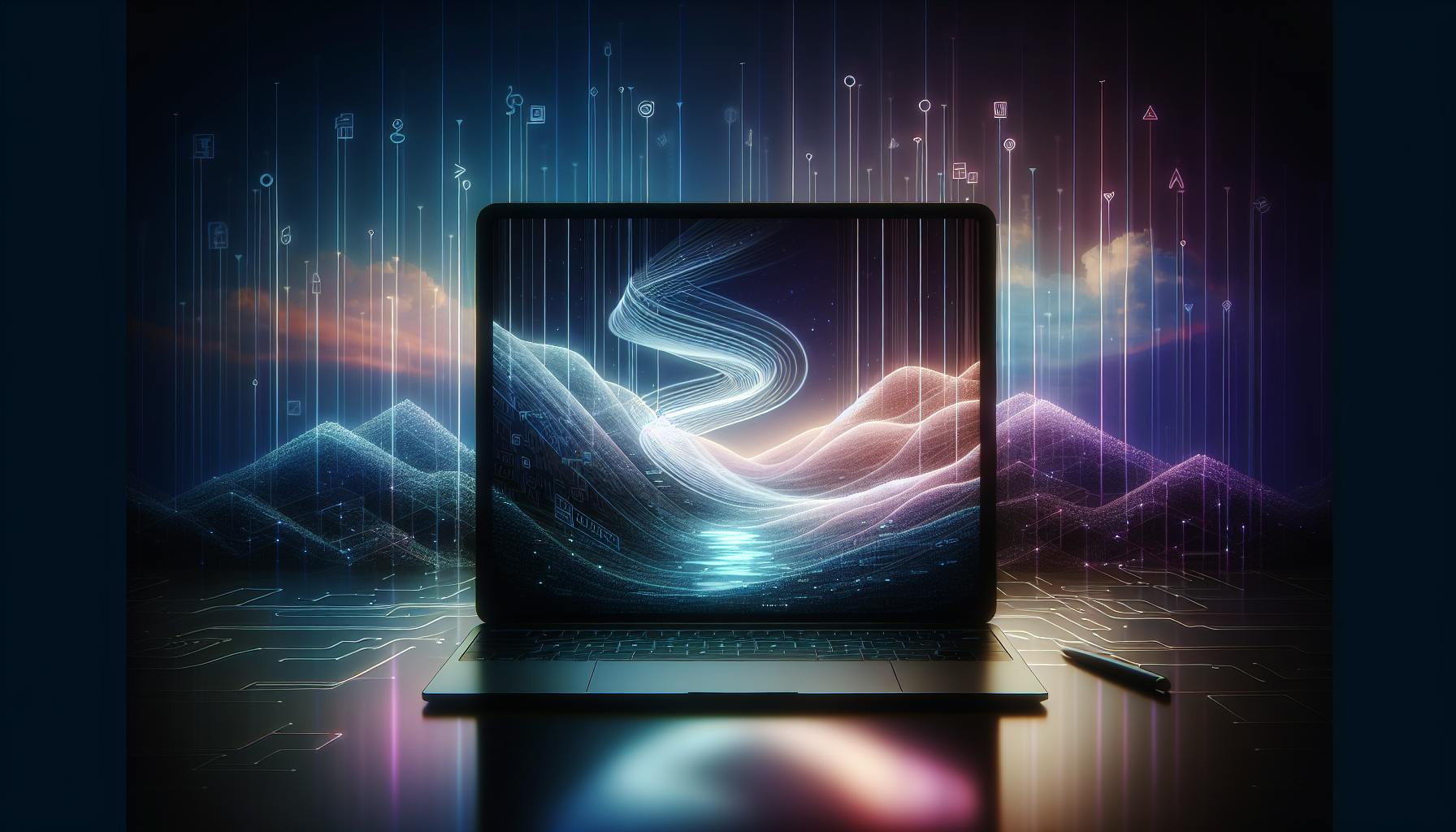
A comprehensive guide to getting started with Svelte, including setting up a development environment, creating components, managing state and events, exploring advanced concepts, and best practices. Learn how Svelte differs from other frameworks and the benefits it offers.
Svelte is a modern tool for building fast and efficient web applications. Unlike traditional frameworks that rely on a virtual DOM, Svelte compiles your code to highly optimized vanilla JavaScript at build time. This guide introduces you to the basics of Svelte, demonstrating how it differs from other frameworks, and walks you through setting up your development environment, creating components, and managing state and events. You'll also get a sneak peek into SvelteKit for more advanced projects and best practices for working with Svelte. Whether you're a beginner or looking to expand your web development toolkit, this guide has something for you.
- Learn what Svelte is and how it differs from other frameworks.
- Set up your Svelte development environment.
- Understand the structure and syntax of Svelte components.
- Manage state and events within your app.
- Explore advanced concepts and SvelteKit for building complex applications.
- Follow best practices for coding and organizing your Svelte projects.
Svelte offers a unique approach to web development, emphasizing performance and developer experience. With its compiler-based architecture, Svelte ensures your applications are lightweight and fast, providing an excellent foundation for both learning and building modern web applications.
Key Features
Here are some cool things about Svelte:
- Reactivity - Svelte automatically updates parts of your app when the data changes, so you don't have to do it yourself.
- Scoped styles - The styles you write in a Svelte component only apply to that component. This means you won't accidentally mess up styles in other parts of your app.
- Transitions - Svelte makes it easy to add smooth animations when things in your app change or move around.
- Accessibility - Svelte helps make your app more accessible to people with disabilities by adding special attributes where needed.
Benefits
Why you might like using Svelte:
- Performance - Apps made with Svelte run really well because there's no extra baggage from using a framework.
- Bundle size - Svelte apps can be smaller than others because they don't include unnecessary code.
- Intuitive syntax - Writing components in Svelte feels natural because it builds on HTML.
- Developer experience - Svelte makes coding more pleasant with features like error messages that help you catch mistakes early.
Svelte's Compiler
Svelte uses a special tool called a compiler to turn your .svelte
files into simple JavaScript. Here's what makes it special:
- Ahead-of-time compilation - Svelte gets your code ready before your app even starts, which helps it run faster.
- Code generation - The code Svelte generates is clean and simple, doing exactly what's needed without extra fluff.
- Tree shaking - Svelte only includes the parts you use in your app, so there's no wasted space.
- Type checking - Using TypeScript, Svelte checks your code for mistakes, making it more reliable.
Setting Up a Svelte Development Environment
Prerequisites
Before diving into Svelte, make sure you have these ready:
- Node.js - Since Svelte is all about building apps with JavaScript, you need Node.js (version 12.22 or newer) to run JavaScript on your computer, not just in a web browser.
- npm or pnpm - These are tools that let you add Svelte and other useful stuff to your project. npm comes with Node.js, but you can get pnpm by itself if you prefer.
- Editor/IDE - Any text editor works for writing Svelte code, but using one like VS Code that understands Svelte can make your life easier.
Project Setup
Getting started with a Svelte project is straightforward. Here's a simple way using npm
:
npm init svelte@latest my-svelte-app
This command creates a new folder named my-svelte-app
with everything set up for a Svelte project.
Or, you can use a tool called degit to copy the official Svelte starter template:
npx degit sveltejs/template my-svelte-app
cd my-svelte-app
npm install
This starter template has some example code to look at.
Next, run npm install
to add any needed libraries, and npm run dev
to start a development server. Now, as you change your Svelte files, your app will update and show the changes in your web browser automatically.
Understanding Svelte Components
Svelte components are built with three key parts:
<script>
The <script>
part is where you write JavaScript code for the component. Here, you can do things like:
- Set up variables with
let
- Make props (properties) with
export let
- Bring in other components
- Use lifecycle functions (code that runs at specific times)
- Write functions and define variables
For instance:
<script>
// Setting up a variable
let count = 0;
// Making a prop
export let name;
// Bringing in another component
import Child from './Child.svelte';
// Lifecycle function example
onMount(() => {
// This runs when the component is first shown
})
// A simple function
function handleClick() {
count += 1;
}
</script>
Markup
The markup part is basically your HTML. It's where you decide how your component looks and behaves. Svelte adds some cool features to HTML like:
- Using
{curly braces}
for dynamic content - If/Else conditions with
#if / #else
- Loops with
#each
- Handling events with
on:event
- Using
<slot>
for placeholders - Two-way data binding with
bind:
Example:
<!-- Dynamic content -->
<h1>Hello {name}!</h1>
<!-- If/Else condition -->
{#if count > 0}
<p>{count} clicks so far</p>
{:else}
<p>No clicks yet</p>
{/if}
<!-- Loop -->
{#each items as item}
<li>{item.name}</li>
{/each}
<!-- Handling an event -->
<button on:click={handleClick}>
Click me
</button>
<!-- Placeholder -->
<slot></slot>
<!-- Two-way data binding -->
<input bind:value={name}>
<style>
The <style>
part is for CSS, which styles your component. These styles only affect this component, preventing any mix-ups with the rest of your app.
Example:
<style>
button {
/* These styles are just for this button */
}
</style>
Svelte components automatically update when you change variables or props, thanks to Svelte's smart coding. This means your app can react quickly to changes without needing extra code.
Managing State and Events
Svelte makes it easy to keep track of information and respond to things users do, like clicking a button.
Local State
To keep track of information inside a component, just use let
:
<script>
let count = 0;
</script>
This makes a variable count
that will automatically update in your component whenever it changes.
For instance, to increase count
when a button is clicked:
<script>
let count = 0;
function handleClick() {
count += 1;
}
</script>
<button on:click={handleClick}>
Clicked {count} {count === 1 ? 'time' : 'times'}
</button>
The button's text will change on its own as count
goes up.
Handling Events
To make something happen when a user does something, like clicking, use things like on:click
. You tell it which function to run:
<button on:click={handleClick}>
Click me
</button>
You can handle all sorts of user actions like click
, input
, and mouseover
.
In your function, Svelte lets you use event
to get more info:
function handleClick(event) {
console.log(event.clientX); // Shows where the cursor was on the x-axis
}
Component Events
Components can send out their own events with createEventDispatcher
:
<script>
import { createEventDispatcher } from 'svelte';
const dispatch = createEventDispatcher();
function shout() {
dispatch('shout', {
message: 'Hello!'
});
}
</script>
Other components can listen to these events with on:shout
. This is how components talk to each other.
Stores
For information that needs to be shared across components, Svelte has something called stores. There are two main types: readable stores for just looking at the information, and writable stores for when you need to change the information from different places.
Advanced Concepts
State Management with Stores
When your Svelte app starts to get bigger, you'll find that you need a way to share information between different parts of your app. This is where Svelte's concept of stores comes into play.
Think of stores as a common space where any part of your app can pick up or leave information. There are mainly two types:
- Readable stores - These are like bulletin boards where components can see the latest updates, perfect for displaying things like user settings or data from the internet.
- Writable stores - These are more interactive. Components can not only see the information but also change it. This is great for things that need to be updated often, like the items in a shopping cart.
To start using a store, you just need to set it up like this:
import { writable } from 'svelte/store';
export const count = writable(0); // Starting point
And here's how you use it in your components:
import { count } from './stores.js';
function increment() {
$count += 1;
}
The cool part is, any component using the store will automatically get the updates.
There are also other types of stores, like derived
that can calculate new values based on other stores, and tweened
for adding smooth animations to changes.
Lifecycle Functions
Svelte components have special functions for different times in their life:
onMount
- When the component first shows uponDestroy
- Just before the component goes awaybeforeUpdate
andafterUpdate
- Right before and after it updates
For example, you can use onMount
to grab data when the component first appears:
onMount(async () => {
const response = await fetch('/api/data');
data = await response.json();
});
And onDestroy
to clean up, like stopping a timer:
onDestroy(() => {
clearInterval(timer);
});
These functions help you manage what happens at different stages.
Dynamic Components
If you want to switch between different parts of your app, you can use <svelte:component>
with a variable to decide which part to show:
let currentTab = Tab1;
<svelte:component this={currentTab}>
Changing currentTab
will switch the component being displayed.
Slots are another way to insert changing content into a fixed structure, like tabs switching content but keeping the same outer look:
<Tab>
<p slot="content">I am the Tab 1 content</p>
</Tab>
<Tab>
<p slot="content">I am the Tab 2 content</p>
</Tab>
The <Tab>
component can show different content dynamically. This is handy for parts of your app where the content changes but the structure stays the same.
Using these advanced features in Svelte can really help make your apps more dynamic and interactive.
Building a Sample App
In this part, we're going to make a simple app for keeping track of tasks using Svelte. This will help us see how Svelte's parts work together.
Project Setup
First, we'll create a new Svelte project and add some tools we need:
npm init svelte@latest svelte-tasks
cd svelte-tasks
npm install date-fns uuid
We're using date-fns to handle dates and times, and uuid to create unique IDs for each task.
Components
Our app will have three parts:
App.svelte
- This is where we manage everything.TaskForm.svelte
- A place to enter new tasks.TaskList.svelte
- Where we show all the tasks.
In App.svelte
, we use a writable store to keep track of tasks:
import { writable } from 'svelte/store';
export const tasks = writable([]);
In TaskForm
, we let the app know when a new task is added:
import { createEventDispatcher } from 'svelte';
const dispatch = createEventDispatcher();
function addTask() {
dispatch('add', {
// Details for the new task
});
}
And App
updates the list of tasks when it gets this info:
import { tasks } from './stores.js';
function handleAdd(event) {
$tasks = [...$tasks, event.detail];
}
TaskList
keeps an eye on the tasks to show them:
import { tasks } from './stores.js';
$: filteredTasks = $tasks.filter(/*...*/);
Features
Here are some cool things we could add to our app:
- Mark tasks as done or not done
- Remove tasks
- Sort or filter tasks
- Keep track of dates and times
- Use local storage to remember tasks
- Add animations and transitions for a nicer look
And there you go! With components, stores, and events, we can create a neat task tracking app with Svelte.
sbb-itb-bfaad5b
Introducing SvelteKit
SvelteKit is a tool that helps you make websites and web apps. It's built on Svelte, which we talked about before, but it adds more features so you can do more things, like making your website work better for search engines and helping pages load faster.
Here’s what SvelteKit offers:
Core Features
- With SvelteKit, your web pages can be prepared on the server before they get to your browser. This means they can load faster and be more friendly to search engines.
- It can work on different platforms like Vercel, Netlify, and regular servers, thanks to its flexible setup.
Routing
- SvelteKit uses the files and folders in your project to figure out the web page structure. This makes setting up new pages easy.
- It can handle all sorts of web page setups, including ones that change based on the link or have multiple layers.
Code Splitting
- It breaks down your code into smaller parts so your website only loads what it needs for each page. This helps pages load much faster.
Developer Experience
- Offers quick updates to your work as you make changes, so you can see what you're doing right away.
- Comes with built-in support for TypeScript and makes it easy to use styles like Sass.
Plugins & Extensibility
- You can add custom features to your development setup. For example, checking your code quality or adding support for content management systems.
- There are over 50 community-made plugins that add all sorts of useful features.
SvelteKit is great for making more complex websites and web apps because it lets you use Svelte in a way that's easy for search engines to read and quick for users to load. It simplifies a lot of the technical stuff, making it easier to get your site up and running.
Best Practices
Here are some easy-to-follow tips for working with Svelte:
Folder Structure
- Keep all your Svelte code in a
src
folder. - Group components by their use or which part of the site they belong to.
- Use a
stores
folder for your stores. - Place images and other static files in a
public
orstatic
folder.
Naming
- For component files, use names like
Header.svelte
. - Stick to
PascalCase
for naming components. - Name stores with a simple format like
tasks.js
. - When naming CSS classes, go with
kebab-case
.
Formatting
- Use a 2-space indent for nested elements.
- Arrange your code with
<script>
, then the HTML markup, and<style>
last. - Keep a blank line between each section for clarity.
- Use tools like Prettier to help keep your code neat.
Components
- Aim to make components that you can use in many places, can be easily adjusted, and are simple.
- Try not to make components too big; smaller is usually better.
- When a component does something, use events to communicate that upwards.
- Use lifecycle functions wisely to manage what happens when components load, update, or go away.
State
- For data that needs to be shared, use stores.
- Keep component-specific data within those components.
- Give stores and events names that say what they do.
Testing
- Consider writing tests before you write your actual code.
- Test components and stores individually.
- Use snapshot tests to catch changes in how your app looks.
- For testing pages that are rendered on the server, tools like Playwright are handy.
Build
- Let SvelteKit take care of getting your project ready for people to use.
- Pick the right tools (adapters) based on where your project will live.
- Turn on options to make your project files smaller and load faster.
Following these simple steps can help you work better with Svelte and SvelteKit. Keeping your code organized, easy to read, and well-tested makes a big difference. And remember, keeping your components small and your project structure clear is key!
Where To Go From Here
Now that you've started with Svelte, there are many ways to keep learning and growing. Here's a guide on what you could do next:
Learn Advanced Svelte
- The official Svelte documentation is a great place to explore more about animations, how to render your app on the server, and how to test your code. They have lots of helpful examples.
- For more challenging projects, look up some advanced tutorials like making a news app or visualizing data with D3.
- You can also find lots of code samples and components on the Svelte REPL.
Join the Community
- The Svelte Discord is a friendly space where you can talk to other developers and ask questions.
- Stay updated with what’s happening in Svelte by following Svelte on Twitter or searching for the #sveltejs hashtag.
- Svelte Society offers more learning resources like courses and articles from other developers.
- Look for a Svelte meetup near you or online to meet other people interested in Svelte.
Put Your Skills to Use
- Try building a bigger app with SvelteKit, and maybe use Node.js for the server side of things.
- Explore creating mobile apps, desktop apps, or browser extensions with Svelte Native.
- If you’re feeling confident, you could even contribute to improving Svelte by helping with its code or documentation.
- And don’t forget to share what you make! Writing tutorials, making videos, or just showing off your projects can help other people learn too.
The Svelte community is always growing and adding new features. So, keep exploring and stay involved!
Conclusion
Svelte is a great tool for making websites and apps that work really well and don't slow down your computer. Let's go over why Svelte is so good:
Performance
- Svelte makes your app's code small and fast right from the start.
- It doesn't use a virtual DOM, which means it uses less computer memory and is quicker when you change something.
- It only includes the code you actually use, so your app isn't weighed down by extra stuff.
Developer Experience
- The way you write code for Svelte is straightforward and easy to pick up.
- It gives you helpful error messages, so you can fix problems before they get big.
- Cool features like styles that only apply to parts of your app, smooth animations, and a simple way to manage data make it fun to use.
Features
- Svelte automatically updates your app when data changes, which saves you time.
- You can write CSS right in your components, and it won't mess up styles in other parts of your app.
- It has a great set of tools for adding animations and dealing with user interactions.
- SvelteKit offers a smooth way to build and organize your app, making things like creating new pages a breeze.
Ecosystem
- There's a big community of Svelte users who make plugins, help out, and create guides.
- Svelte works well with other tools you might already be using, like TypeScript, ESLint, and Prettier.
- You can use Svelte to build more than just web apps. It's also great for server-side projects with Node.js.
The best way to get better at Svelte is by making actual projects with it. Here are some ideas:
- A blog or a website for a business that's easy to update with SvelteKit
- A web app that works offline and can send you notifications
- A dashboard for keeping an eye on data or stats
- A game where multiple players can interact in real-time
There's a lot you can do with Svelte. Try making something and see how it goes. And if you do, share what you've made with others who are learning too!
Related Questions
Is Svelte good for beginners?
Yes, Svelte is great for people who are just starting out. It's easier to learn than many other tools because it uses simple code that looks a lot like the basic HTML, CSS, and JavaScript you might already know. Svelte also takes care of tricky parts for you, like making sure styles don't clash and updating your app automatically when data changes. This makes it less daunting for newcomers to get into web development.
What is the easiest way to get started with Svelte?
The simplest way to try out Svelte is by using the Svelte REPL. This online tool lets you write Svelte code and see what it does right away, without having to set anything up on your computer. If you want to build a real project, you can start by running npm init svelte@latest
in your terminal. This sets up a new project with everything you need to start coding.
Does Svelte have a future?
Yes, Svelte is becoming more popular and has a lot of potential. It's different because it makes your app run faster by doing a lot of work before the app even starts. SvelteKit, a tool based on Svelte, also helps with making websites that load quickly and are easy to find on search engines. As the web keeps evolving, Svelte's focus on making things fast and simple will likely attract more developers.
What are the cons of Svelte?
Since Svelte is newer, it doesn't have as big a community or as many extra tools and libraries as some other frameworks. This means you might not find as many resources, tutorials, or community support. However, the community is growing, and many people find that Svelte's easy-to-understand approach makes up for these gaps.