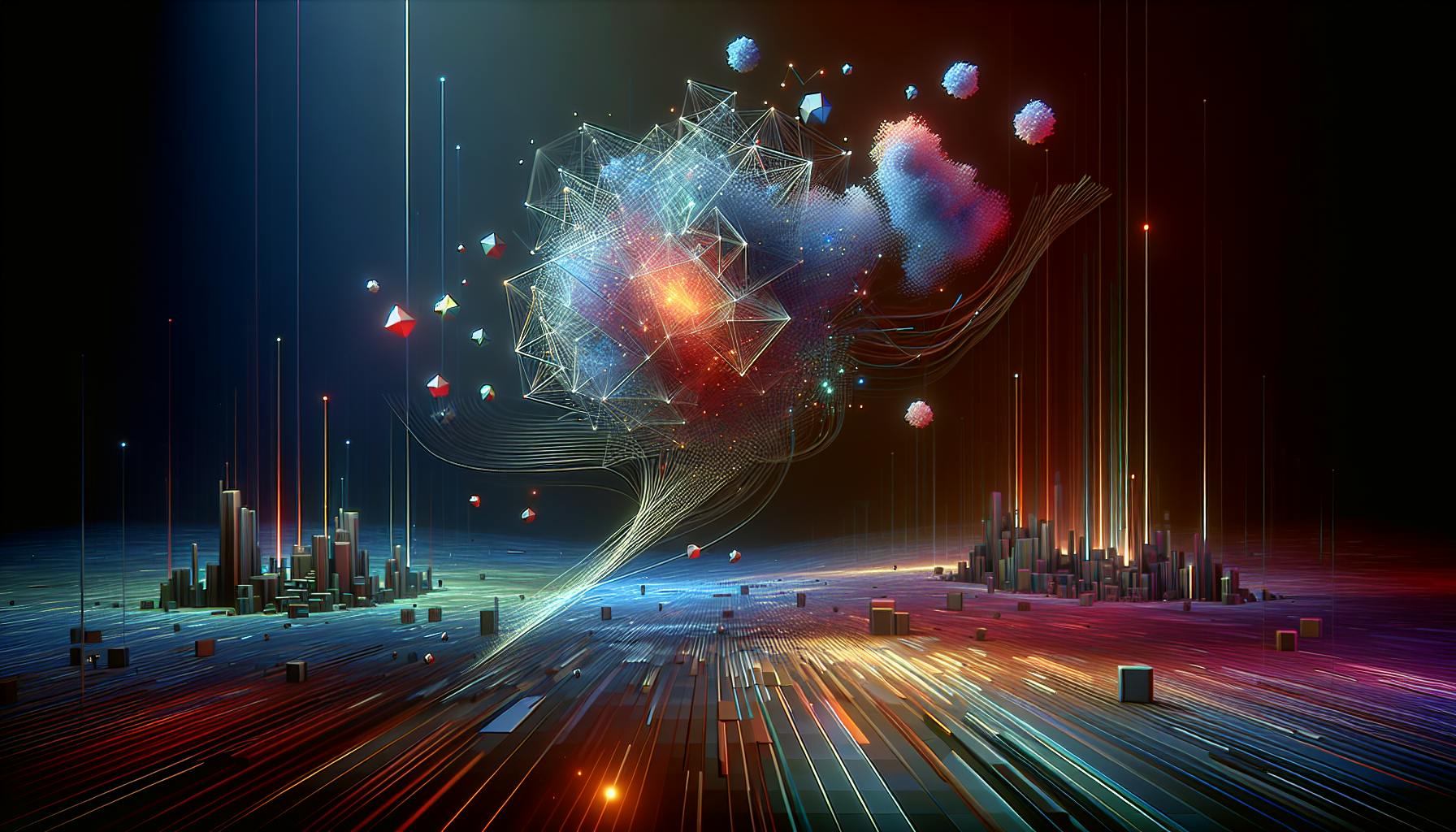
Learn the essentials of working with Angular arrays, from creating and modifying to transforming and sharing data between components. Explore HTML structure, JavaScript basics, and TypeScript features for efficient array management.
Understanding how to work with angular arrays is crucial for developers building web applications. This guide covers the essentials, from creating and initializing arrays to modifying and searching their elements. Here's a quick rundown:
- Creating and Initializing Arrays: Learn to declare, initialize, and access array elements.
- Adding and Removing Elements: Methods like
.push()
,.pop()
, and.splice()
help in modifying array contents. - Modifying and Searching Elements: Discover how to update array elements and search for specific items using methods like
.find()
and.includes()
. - Transforming Arrays: Use
.map()
,.filter()
, and.reduce()
to manipulate and derive new arrays. - Slicing and Concatenating Arrays: Learn to slice parts of arrays and concatenate multiple arrays into one.
- Angular Component Interaction: Understand how to share arrays between components using
@Input()
and@Output()
. - Best Practices: Tips on initializing, copying arrays correctly, and avoiding common pitfalls.
This guide aims to provide you with the foundational knowledge needed to effectively manage arrays in your Angular projects, making your app development process smoother and more efficient.
HTML
- Know how to structure a page with
<head>
and<body>
. - Be familiar with common tags like
<div>
,<span>
,<h1>
to<h6>
,<p>
,<ul>
,<ol>
, and<li>
. - Understand what attributes like
id
,class
,style
do. - Learn how to add links and images.
HTML is what you use to create the basic parts of a website that Angular can enhance.
JavaScript
- Learn about variables and types like strings, numbers, and booleans.
- Get the hang of using operators for math and comparisons.
- Understand how to make decisions with
if/else
and loop through data. - Practice writing functions to do tasks.
- Know how to group data and functions in objects.
- Learn about the
this
keyword and how to use it.
JavaScript is used to make web pages interactive and functional.
TypeScript
- Use static typing to make your code safer.
- Learn about interfaces to ensure parts of your code work together.
- Understand classes and how to use inheritance.
- Know when to use access modifiers like
public
andprivate
. - Get familiar with generics for writing flexible code.
TypeScript adds extra features to JavaScript, making it better for building big apps like those you create with Angular.
With a basic understanding of these areas, you'll be well-prepared to tackle arrays in Angular. Remember, learning these concepts takes time and practice, so be patient with yourself. It's always okay to go back to the basics when learning new things in Angular. Having a strong foundation makes learning more complex topics much easier.
Creating and Initializing Arrays
Arrays are super useful in Angular for keeping and working with lists of data in order. Let's dive into how to start off with arrays in TypeScript, which is what Angular uses.
Declaration
To tell TypeScript you're making an array, you just add []
after the type of stuff you'll put in the array, like this:
let numbers: number[] = []; // This is for numbers
let strings: string[] = []; // This is for text
let booleans: boolean[] = []; // This is for true/false values
Or, you can use a more general way with Array
:
let numbers: Array<number> = [];
Initialization
There are a couple of ways to fill your array right from the start:
Using square brackets:
let numbers = [1, 2, 3];
Using the new Array
way:
let numbers = new Array(1, 2, 3);
Making an empty array with a set size:
let numbers = new Array(3); // Makes an array with 3 empty spots
Accessing Elements
To get to an item in your array, use square brackets with the item's number (starting from 0):
let first = numbers[0];
To find out how many items are in your array, use .length
:
numbers.length; // 3
This is handy for getting the last item without counting:
let last = numbers[numbers.length - 1];
With these basics down, you're all set to use arrays in your Angular projects, making it easier to handle lists of data in your apps.
Adding and Removing Elements
Let's talk about how to put in and take out things from an array in Angular. Here are some simple ways to do it.
Adding Items
If you want to add something to the end of a list, use .push()
:
let fruits = ['Apple', 'Banana'];
fruits.push('Orange');
// Now, fruits is ['Apple', 'Banana', 'Orange']
But if you want to add something at the start, use .unshift()
:
let fruits = ['Apple', 'Banana'];
fruits.unshift('Strawberry');
// Now, fruits is ['Strawberry', 'Apple', 'Banana']
You can also put two lists together with the spread ...
operator:
let fruits = ['Apple', 'Banana'];
let citrus = ['Orange', 'Lemon'];
let produce = [...fruits, ...citrus];
// Now, produce is ['Apple', 'Banana', 'Orange', 'Lemon']
Removing Items
To take something off the end, use .pop()
:
let fruits = ['Apple', 'Banana', 'Orange'];
fruits.pop();
// Now, fruits is ['Apple', 'Banana']
To take something off the start, use .shift()
:
let fruits = ['Apple', 'Banana', 'Orange'];
fruits.shift();
// Now, fruits is ['Banana', 'Orange']
And to take out things from the middle, use .splice()
:
let fruits = ['Apple', 'Banana', 'Orange', 'Grape'];
fruits.splice(2, 1);
// Now, fruits is ['Apple', 'Banana', 'Grape']
The .splice()
method needs to know where to start and how many things to take out.
So, with these basic steps for adding and taking out stuff, you'll be great at changing lists in Angular in no time!
Modifying and Searching Elements
Changing arrays in Angular can be straightforward. Let's look at how you can do it:
Modifying Elements with Indexes
You can change an item in an array simply by using its position number (index):
let fruits = ['Apple', 'Banana', 'Orange'];
fruits[1] = 'Strawberry';
// Now fruits is ['Apple', 'Strawberry', 'Orange']
Just remember, counting starts at 0, so the first item is 0, the second is 1, and so on.
Using splice() to Modify Elements
The .splice()
method lets you add, remove, or replace elements:
let fruits = ['Apple', 'Banana', 'Orange'];
// Replace 1 element at index 1
fruits.splice(1, 1, 'Strawberry');
// Now fruits is ['Apple', 'Strawberry', 'Orange']
You tell it where to start, how many to take out, and what to put in.
Iterating Arrays with Loops
To change many items at once, you can use a loop:
let fruits = ['apple', 'banana', 'orange'];
for (let i = 0; i < fruits.length; i++) {
// Make the first letter big
fruits[i] = fruits[i][0].toUpperCase() + fruits[i].substr(1);
}
// Now fruits is ['Apple', 'Banana', 'Orange']
This goes through each item, making the first letter uppercase.
Searching Arrays
To find if an array has a certain item, you can use these methods:
Using includes():
let fruits = ['Apple', 'Banana', 'Orange'];
fruits.includes('Banana'); // true
Using indexOf():
let index = fruits.indexOf('Apple'); // 0
Using find():
let fruit = fruits.find(f => f === 'Orange'); // 'Orange'
So, you have many ways to change arrays and find items in Angular. Getting good at these methods will help you manage arrays better in your projects.
Transforming Arrays
Changing arrays in Angular is pretty straightforward and can really help you work with your data in different ways. Let's look at some common methods to transform arrays:
Map
The .map()
method goes through each item in your array, does something to it, and makes a new array with the results.
For instance, if you want to make all the fruit names uppercase:
let fruits = ['apple', 'banana', 'orange'];
let uppercasedFruits = fruits.map(fruit => fruit.toUpperCase());
// Now, uppercasedFruits is ['APPLE', 'BANANA', 'ORANGE']
Filter
The .filter()
method looks at each item and keeps only the ones that meet a certain condition, making a new array with them.
For example, to keep only numbers bigger than 5:
let numbers = [1, 5, 10, 15];
let bigNumbers = numbers.filter(n => n > 5);
// Now, bigNumbers is [10, 15]
Reduce
The .reduce()
method combines all items in an array into one value.
For example, to add all numbers together:
let numbers = [1, 5, 10];
let sum = numbers.reduce((prev, curr) => prev + curr);
// The total sum is now 16
sort()
The .sort()
method puts your items in order, either alphabetically or by number:
let fruits = ['Orange', 'Apple', 'Banana'];
fruits.sort();
// Now, fruits is ['Apple', 'Banana', 'Orange']
You can even tell it how to sort things exactly the way you want by giving it special instructions.
These methods are super helpful for changing your arrays in Angular to fit what you need for your apps. They're key tools for organizing and managing your data.
sbb-itb-bfaad5b
Slicing and Concatenating Arrays
Slicing and putting arrays together are useful tricks in Angular when you want to handle parts of arrays or merge them.
Slicing Arrays with slice()
The .slice()
method is like taking a piece from a cake without changing the rest of it. You just need to say where to start and end. It picks up everything from the start up to, but not including, the end point:
let fruits = ['Apple', 'Banana', 'Orange', 'Mango'];
let citrus = fruits.slice(2, 4);
// citrus now has ['Orange', 'Mango']
This method is handy for when you need to work with just a part of a big array.
Concatenating Arrays with concat()
When you want to stick arrays together, .concat()
is your friend:
let fruits = ['Apple', 'Banana'];
let vegetables = ['Asparagus', 'Broccoli'];
let produce = fruits.concat(vegetables);
// produce now has ['Apple', 'Banana', 'Asparagus', 'Broccoli']
Your original arrays stay the same, and you get a new one that's the combination of them.
And you can combine more than two arrays at once:
let array1 = [1, 2];
let array2 = [3, 4];
let array3 = [5, 6];
let combined = array1.concat(array2, array3);
// combined is now [1, 2, 3, 4, 5, 6]
So, slicing and putting arrays together are great for managing parts of arrays and joining them in your Angular projects.
Angular Component Interaction
Components are like the Lego pieces of Angular apps. A big part of using these pieces is making sure they can talk to each other, especially when it comes to sharing lists (arrays) of stuff between them. Here's how you can do that with some special tools in Angular called @Input()
and @Output()
.
Passing Arrays into a Child Component with @Input()
To send a list from a parent component down to a child, we use something called the @Input()
decorator:
// parent component
@Component({
// ...
})
export class ParentComponent {
fruits = ['Apple', 'Banana', 'Orange'];
}
// child component
@Component({
// ...
})
export class ChildComponent {
@Input() fruits: string[];
}
Then, in the parent's template, you connect the dots like this:
<app-child [fruits]="fruits"></app-child>
This way, the child gets the fruits
list from the parent.
Emitting Arrays from a Child Component with @Output()
To send data back up to the parent, we use the @Output()
decorator with an EventEmitter
:
// child component
@Component({
// ...
})
export class ChildComponent {
@Output() selectedFruits = new EventEmitter<string[]>();
someMethod() {
let selected = ['Apple', 'Orange'];
this.selectedFruits.emit(selected);
}
}
The parent can then catch this info:
// parent component
@Component({
// ...
})
export class ParentComponent {
fruits = ['Apple', 'Banana', 'Orange'];
onFruitSelected(selected: string[]) {
// do something
}
}
And in the template, it looks like this:
<app-child
[fruits]="fruits"
(selectedFruits)="onFruitSelected($event)">
</app-child>
So, using @Input()
and @Output()
, we can easily share lists of things like fruits between parent and child components.
Best Practices
When you're working with arrays in Angular, it's easy to slip up. Here's how to keep things smooth and avoid common traps:
Initialize Arrays Properly
Start off right by clearly stating what type of stuff your array will hold:
let fruits: string[] = [];
Or you can do it this way:
let fruits: Array<string> = [];
This keeps you from running into trouble later on.
Copy Arrays Correctly
If you just link two arrays like this, they'll actually be the same thing:
// Not the best idea
let fruits = ['Apple'];
let fruitsCopy = fruits;
Instead, do it like this to make a real copy:
// Much better
let fruits = ['Apple'];
let fruitsCopy = [...fruits];
Now, changing one won't affect the other.
Watch Out for Mutation Methods
Some methods like .push()
and .pop()
change the original array. To avoid unexpected issues:
- Copy the array first if you need the original untouched.
- Or use methods like
.concat()
or.slice()
that don't change the original.
Use Type Checks
With .push()
, you can accidentally add the wrong type of thing. To keep it safe:
class FruitBowl {
fruits: string[] = [];
addFruit(fruit: string) {
this.fruits.push(fruit);
}
}
This way, only the right type of items can be added.
Use Explicit Types with map(), filter(), reduce()
These methods can sometimes give you a mixed bag of types unless you're clear about what you want:
let fruits = ['Apple'];
let apples = fruits.filter(f => f === 'Apple'); // Now it's clear we only want strings
Keeping these tips in mind will help you work with arrays more effectively and avoid common mistakes.
Conclusion
Arrays in Angular help you organize and change lists of data in your apps. Knowing how to work with arrays means you can deal with complicated data and make your apps do cool stuff.
Here's a quick review of what we talked about:
-
Creating Arrays - To make a new array, write
let myArray: Type[] = []
orlet myArray: Array<Type> = []
. You can start with some data in it or leave it empty. -
Adding/Removing Items - Use
.push()
,.unshift()
,.pop
, and.shift()
to add or remove items from your list. -
Modifying Arrays - Change values using their position or remove items with splice. Use loops to update many items at once.
-
Searching Arrays - Find items with
.indexOf()
and.includes()
, or use.find()
for specific searches. -
Transforming Arrays - Change the shape of your data with
.map()
,.filter()
,.reduce()
, and.sort()
. This helps you prepare data for showing in your app. -
Slicing & Concatenating - Use
.slice()
to take parts of arrays or.concat()
to stick arrays together. -
Sharing Data - Send arrays from one component to another with
@Input()
and@Output()
. -
Best Practices - Stick to clear rules like typing your arrays and using methods that don't change the original array to avoid mistakes.
With these basics, you can use arrays to handle lots of data in your Angular apps. Arrays are key for showing, changing, and sharing data in web and mobile apps.
To learn more, check out the Angular guides on arrays and data binding. Try reading about common array manipulation and make some test apps to practice fetching and showing data from the internet.
As you get better at using arrays to move and change data between parts of your app, you'll find new ways to make your apps interactive and fast.
Related Questions
What would happen in the code if you did not use arrays?
Without arrays, we'd have to keep each item in its own variable and write a lot more code to work with them. This would make our code longer, harder to manage, and more likely to have mistakes.
Jan 1, 2024
Can you put variables in an array in JavaScript?
Yes, you can put variables in an array in JavaScript. Arrays are special kinds of objects, so they can hold variables of different types together.
What can you put in an array?
Arrays are meant to hold items of the same type, like numbers, strings, or objects. Once you decide what type of items your array will hold, all the items in that array should be of that type.
What is the use of an array in HTML?
Arrays aren't directly used in HTML. However, in JavaScript code that works with HTML, arrays help us store, organize, and change lists of data that we want to show on the web page.