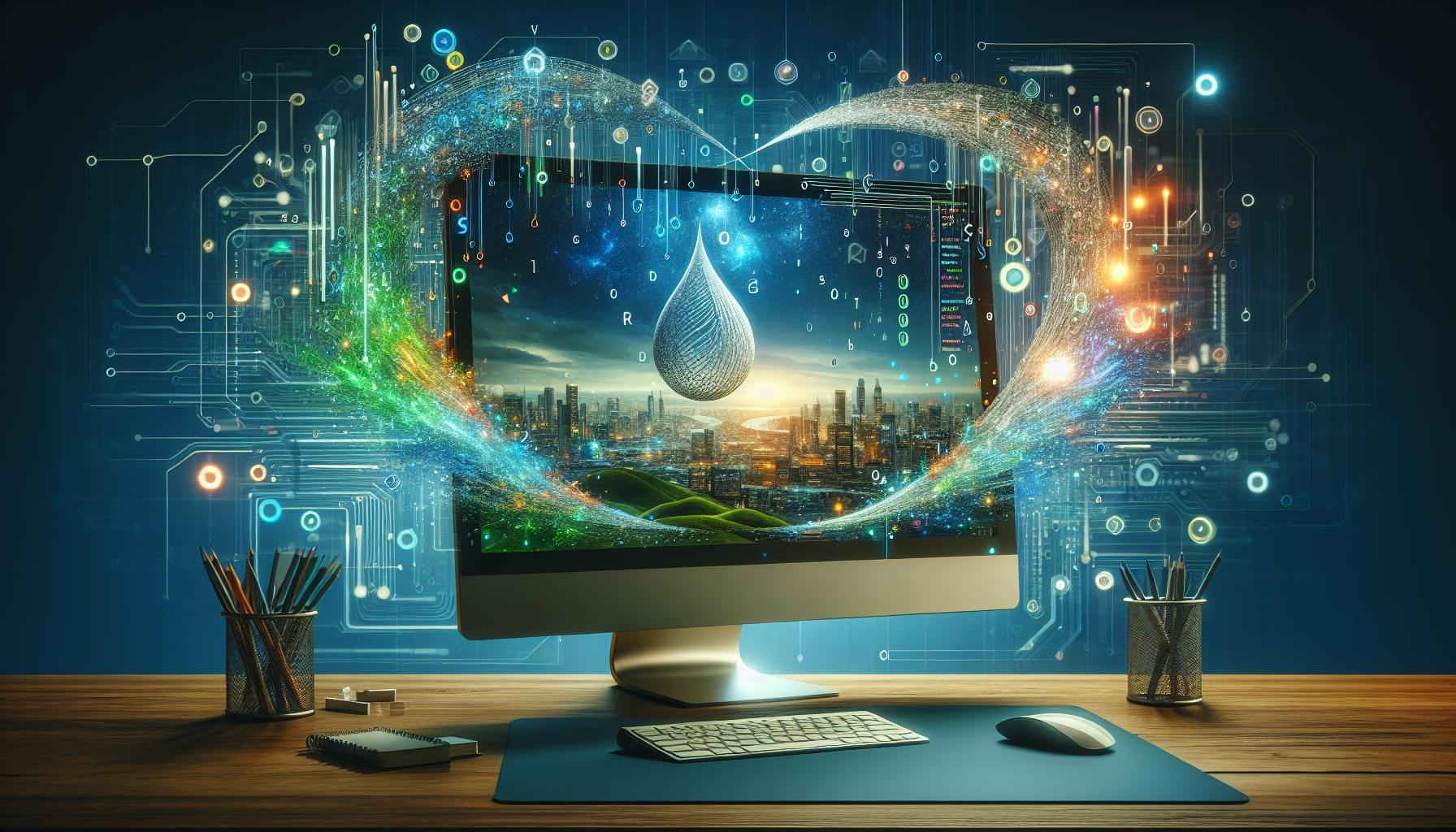
An introduction to Scala programming, covering the basics, features, advanced topics, and project setup. Learn about Scala's unique characteristics, functions, classes, collections, concurrency, and more.
If you're curious about Scala and why it's a popular choice for both beginners and seasoned developers, you've come to the right place. Scala is a unique programming language that combines the best of object-oriented and functional programming paradigms, offering a flexible and powerful platform for building scalable applications. Here's a quick overview of what we'll cover:
- What is Scala? An introduction to Scala, its creation, and its core principles.
- Getting Started with Scala: How to set up Scala on your computer and write your first program.
- Scala Basics: Learn about syntax, variables, data types, and control structures.
- Deep Dive into Functions and Classes: Exploring functions, including anonymous and nested functions, and understanding classes and objects.
- Advanced Scala Features: Pattern matching, Scala collections, and concurrency in Scala.
- Building Scala Projects: Tips on project setup, testing with ScalaTest, and running and packaging your Scala applications.
Whether you're completely new to programming or just new to Scala, this guide is crafted to build up your understanding from the basics to more complex concepts, using easy-to-follow examples and practical tips. Let's get started on this exciting journey into Scala programming!
What is Scala?
Scala was created in 2001 by Martin Odersky and his team in Switzerland. They wanted to make a language that:
- Combined object-oriented and functional programming
- Was statically typed but also easy to read and write
- Could handle concurrency (doing multiple things at once) and work well across different systems
- Was extendable and worked well with Java
Thanks to these goals, Scala is a very flexible language great for making scalable, high-performance systems and apps.
Some important things about Scala include:
- It's statically typed but doesn't feel like it
- It runs on the JVM (Java Virtual Machine)
- You can use Java code with it
- It supports both object-oriented and functional programming styles
- It has a concise and expressive way of writing
- It uses an advanced type system with type inference
- It focuses on immutable data structures and pattern matching
- It uses an actor-based model for dealing with concurrency
Who is this Guide For?
This guide is for anyone who's new to Scala or even programming in general. We'll start from the very beginning, so you don't need any prior knowledge.
We'll learn by doing, using simple code examples that you can try out yourself. We'll also share helpful tips and things to watch out for along the way.
If you're looking for a straightforward, practical introduction to Scala, you're in the right spot!
How this Guide is Structured
In this guide, we'll cover:
- Setting Up Scala - How to install Scala and write your first program
- Scala Basics - Learning about variables, data types, and how to use operators
- Control Structures - How to make decisions and repeat actions in your code
- Functions - Understanding functions and functional programming in Scala
- Classes & Objects - Learning about object-oriented programming
- Collections - Exploring Scala's collection library
We're aiming to keep things simple and focus on helping beginners. We want to make complex ideas easy to understand with clear examples.
Let's start coding!
Understanding Scala
The Evolution of Scala
Scala started in 2001 by Martin Odersky and his team in Switzerland. Odersky had worked on Java before and wanted to make a new language that mixed object-oriented (like Java) and functional programming.
Since its first release in 2003, Scala has gone through several updates:
- 2004 - Scala 2.0 came out with big changes to how it works.
- 2006 - Scala 2.1 added new features like traits and package objects.
- 2009 - Scala 2.8 made it faster and changed the standard library.
- 2014 - Scala 2.11 improved speed further.
- 2016 - Scala 2.12 made the language simpler and more modular.
Over the years, Scala has focused on being innovative but also stable. The 2016 update was a big deal because it made Scala better for businesses without losing its unique features.
Design Goals of Scala
Scala was made with some important goals:
- Integration - It works well with Java and uses the JVM infrastructure.
- Conciseness - It lets you say more with less code.
- Type safety - It keeps a strong check on types but is flexible.
- Object-oriented - It supports the object-oriented way of programming.
- Functional - It includes functional programming for better parallelism and scale.
- Scaleable - It's made to be fast and handle big systems.
These goals make Scala special. It combines object-oriented and functional programming on the JVM, making it great for big, fast systems.
Why Scala?
Scala has several advantages:
- Conciseness - You need less code compared to Java.
- Performance - Scala code can be as fast or faster than Java.
- Productivity - Its features make developers work faster.
- Functional capabilities - It's good for writing programs that do many things at once.
- Interoperability with Java - It can easily use Java libraries.
- Growing adoption - More companies are starting to use Scala.
In short, Scala lets developers write systems that are fast, safe, and scalable by combining the best of object-oriented and functional programming.
Scala in the Real World
Big companies use Scala for important systems:
- Twitter - Uses Scala for many parts of its platform.
- LinkedIn - Uses Scala for big data processing.
- Netflix - Has critical parts of its platform built on Scala.
- Apple - The Siri backend is written in Scala.
- Walmart - Uses Scala for its search engine and services.
- Zalando - Uses Scala in its architecture.
Scala's unique strengths make it a good choice for big systems that need to handle lots of data and users. More and more companies around the world are using it.
Setting up Scala
Installing Scala
To get Scala on your computer, you need to:
- Download Scala - Go to www.scala-lang.org and pick the latest version that works with your computer.
- Set up PATH variables - This lets you use Scala from anywhere on your computer. For Windows, you'll add the Scala folder path to your System variables. On Linux and macOS, you add it to your
.bashrc
or.zshrc
file. - Check if it's working - Open your terminal and type
scala -version
to see if Scala is ready to go.
Choosing an IDE
An IDE makes writing Scala code easier. Here are some good ones:
- IntelliJ IDEA - It's great for Scala and comes with smart code help, debugging, and more. There's a free version too.
- Eclipse - Free and open source, it supports Scala if you add the Scala IDE plugin. It helps with code suggestions, debugging, and other handy things.
- Ensime - Works with text editors like Vim, Emacs, Atom, etc., to give you IDE features for Scala.
Pick an IDE based on the Scala features and support you need. IntelliJ IDEA is a strong choice for Scala work.
Using Build Tools
Build tools like SBT and Maven help with compiling, testing, and managing your Scala projects.
-
SBT - Made for Scala and Java, it's good for compiling code, managing packages, running tests, and more.
-
Maven - Also works with Scala through a plugin and is great for project management and fits well into workflows.
SBT is highly recommended for Scala projects. We'll talk more about using SBT later.
Setting up a Hello World App
Let's make a simple program to check everything is working:
- Make a new folder for your project
- Make a file named
HelloWorld.scala
- Write this code inside:
object HelloWorld {
def main(args: Array[String]): Unit = {
println("Hello, World!")
}
}
- In the terminal, compile it with
scalac HelloWorld.scala
- Run it with
scala HelloWorld
You should see "Hello, World!" on your screen. Great job, your Scala setup is all good!
Scala Syntax Basics
Let's talk about how Scala is written and the basic rules it follows. Understanding these will help you start coding in Scala.
Syntax Conventions
Scala has a few simple rules about how code should look:
- Semicolons - You don't really need to use semicolons at the end of lines in Scala. It's up to you.
- Parentheses - Sometimes, when you call methods, you can skip the parentheses. Just make sure your code is still easy to read.
- Case sensitivity - Remember that Scala cares about uppercase and lowercase letters, so
myVar
andmyvar
are not the same. - Indentation - Keeping your code neatly indented makes it easier to read. The common practice is to use 2 spaces for each new level.
Sticking to these rules will help your code be clear and tidy.
Values and Variables
Scala has two types of variables - vals
and vars
:
- vals - These are constants that you set once and can't change. They're great for values that stay the same.
- vars - These are variables that you can change over time.
Example:
// A constant value
val language = "Scala"
// A variable we can change
var version = "2.12"
version = "2.13" // It's okay to change it
Scala is smart and can figure out what type a variable is, so you don't always have to say it. But you can if you want to be extra clear:
val language: String = "Scala"
Try to use vals
more than vars
to keep things simple and safe.
Basic Data Types
Scala has several simple types of data:
- Boolean - Just
true
orfalse
- Int - A whole number
- Double - A big, precise number
- String - A bunch of letters or words together
- Unit - This is like saying "nothing" or "void" in Java
For example:
val enabled: Boolean = true
val price: Int = 10
val tax: Double = 0.05
val name: String = "Scala"
val result: Unit = println("Hello!")
These are the building blocks for storing information in Scala.
Control Structures
Scala uses common patterns to make decisions or repeat actions:
If-else
val x = 10
if (x > 5) {
println("x is greater than 5")
} else {
println("x is less than or equal 5")
}
Match
val day = "Tuesday"
day match {
case "Monday" => println("start of week")
case "Friday" => println("end of week")
case _ => println("midweek day")
}
For loop
for (x <- 1 to 10) {
println(x)
}
Try/catch
try {
// This might cause an error
val x = 10/0
} catch {
case e: Exception => println("Error")
}
These structures help you add logic to your Scala programs, letting you handle more complex situations.
Functions in Scala
First-Class Functions
In Scala, you can treat functions just like any other piece of data. This means you can pass them around as if they were numbers or strings, use them as inputs for other functions, or even store them in variables. This makes it easy to build flexible and powerful programs.
Here are some examples:
// Assigning a function to a variable
val addNumbers = (x: Int, y: Int) => x + y
// A function that takes another function as an argument
def operate(f: (Int, Int) => Int, a: Int, b: Int): Int = {
f(a, b)
}
// Using the addNumbers function with operate
val result = operate(addNumbers, 10, 5) // Gives us 15
This approach helps make your code more modular and easy to reuse.
Anonymous Functions
Scala lets you write functions without names, which are called anonymous functions. They're handy for small tasks and can be used directly where they're needed.
Here's how you write one:
(param1: Type1, param2: Type2) => {
// What the function does
param1 + param2
}
You might use them for:
- Quick tasks inside other functions
- When you need a simple function and don't want to clutter your code with names
Example:
val numbers = List(1, 2, 3)
numbers.map(x => x * 2) // Turns it into List(2, 4, 6)
Anonymous functions keep things short and sweet.
Nested Functions
Scala allows you to write functions inside other functions. The inner ones can use the same variables as the outer ones, which can be really convenient.
def outer(input: Int) = {
val multiplier = 2
// Inner function
def inner(x: Int) = x * multiplier
inner(input) * 2
}
println(outer(5)) // Shows 20
This can be useful, but it's best to use it carefully to keep your code easy to understand.
Currying Functions
Currying is a fancy way of breaking down a function that takes several arguments into a series of functions that each take one argument.
For example:
// A normal function
def add(x: Int, y: Int) = x + y
// A curried version
def curriedAdd(x: Int)(y: Int) = x + y
// How to use it:
curriedAdd(5)(10) // Gives us 15
Currying can be great for making specialized functions and can make some types of function use easier.
Classes and Objects in Scala
Defining Classes
In Scala, you can make a class with the class
keyword, followed by the name you want to give your class and any initial info it needs. Inside the class, you can have fields (which are like variables) and methods (which are like functions).
Here's a simple example:
class Person(name: String, age: Int) {
// Fields
private var _name = name
private var _age = age
// Methods
def getName(): String = _name
def setName(newName: String) {
_name = newName
}
}
You can make some members private, which means they can only be used inside the class. Others can be public, meaning anyone can use them.
Creating Objects
To use a class, you make an instance of it, which is called an object. Here's how:
val person = new Person("John", 30)
You can then use the object's public methods:
person.getName() // Returns "John"
So, objects are a way to bundle together variables and functions that work on them.
Inheritance in Scala
Scala lets one class inherit from another using extends
. This means the new class gets all the methods and fields of the old one and can add or change some:
class Employee(name: String, salary: Double) extends Person(name) {
override def getName(): String = {
"Emp: " + super.getName()
}
}
You can also change the type of an object to another related type.
Traits as Interfaces
Traits in Scala are like a mix between classes and interfaces. They can have methods with code in them that other classes can inherit. You can mix multiple traits into a class.
For example:
trait Logger {
// Abstract method
def log(msg: String): Unit
// Concrete implementation
def info(msg: String): Unit = log("INFO: " + msg)
}
class ConsoleLogger extends Logger {
def log(msg: String) = println(msg)
}
Traits are a handy way to share code between classes.
sbb-itb-bfaad5b
Pattern Matching
Case Classes in Scala
Case classes in Scala are special kinds of classes that are perfect for when you want to keep things simple and not change them later. They're easy to break apart for checking what's inside, and Scala does a lot of the heavy lifting for you, like making equals
and hashCode
methods automatically.
Here's how you make one:
case class Person(name: String, age: Int)
And here's how you can use it with pattern matching:
val person = Person("John", 30)
person match {
case Person(n, a) => println(s"Name: $n, Age: $a")
}
// This will print "Name: John, Age: 30"
This way, you can easily get to the parts of the case class you're interested in.
Match Expressions
Match expressions in Scala are like supercharged if-else
statements. They let you compare a value against different patterns and do something based on the first one that matches:
x match {
case 1 => "One"
case 2 => "Two"
case _ => "Unknown" // This is the default case
}
For example, you can use them to check what's inside an option:
opt match {
case Some(x) => s"Found $x"
case None => "Empty"
}
Match expressions are really handy for controlling the flow of your program.
Pattern Guards
You can make your match expressions even smarter by adding if
conditions right inside the patterns. This is called using a guard:
item match {
case item: Item if item.price > 100 => "Expensive"
case _ => "Cheap"
}
Guards are great because they let you handle more specific situations without making your code messy.
Sealed Traits for Exhaustive Matching
Sealed traits in Scala help you make sure that when you're using match expressions, you've covered all possible cases. This is because they let the Scala compiler know all the types that might be involved ahead of time:
sealed trait Color
case object Red extends Color
case object Blue extends Color
val color: Color = Red
color match {
case Red => "Got Red"
case Blue => "Got Blue"
}
If you forget a case, the compiler will let you know, which helps prevent errors. Sealed traits, especially when used with case classes, make your code safer and more predictable.
Scala Collections
Scala comes with a lot of different types of collections. Think of collections as boxes where you can store and organize your data. Some boxes are fixed once you fill them up (immutable collections), and some allow you to add or remove items anytime (mutable collections).
Immutable vs. Mutable Collections
-
Immutable collections like
List
,Vector
,Set
, andMap
are like sealed boxes. Once you put something inside, you can't change it. This is great for when you have many people (threads) accessing the box at the same time because they can't mess up the contents. -
Mutable collections like
ArrayBuffer
,ArrayStack
,HashMap
are more like backpacks. You can take things out, put new things in, and rearrange them as you need. They're handy when you're working alone or when you're sure only one person at a time will access the backpack.
Choosing between sealed boxes and backpacks (immutable vs mutable collections) can help your code be safer or more flexible, depending on what you need.
Common Collection Types
Here are some of the most used Scala collection types:
-
List - A simple line of items, one after another. Great for when you need to look at things one by one.
-
Vector - Like a List, but better if you want to jump directly to an item in the middle.
-
Set - A group where each item is unique. No duplicates allowed.
-
Map - A collection where each item has a key and a value, like a dictionary.
-
Array - A flexible line of items that you can change anytime. It's stored in a way that makes it quick to work with.
Each type of collection is best for certain jobs.
Transforming Collections
Scala lets you easily change collections with operations like map
, filter
, flatMap
:
val numbers = List(1, 2, 3)
val doubled = numbers.map(x => x * 2) // Turns into List(2, 4, 6)
val even = numbers.filter(x => x % 2 == 0) // Turns into List(2)
You can also use methods like fold
, reduce
, and aggregate
to combine items in a collection in different ways.
Parallel Collections
Scala has special collections that can do many things at once, using more than one CPU core. This is great for quickly handling lots of data:
val numbers = (1 to 1000).toVector.par
numbers.map(_ + 1).reduce(_ + _) // This happens all at once, not one by one
This feature is a big help when you need to speed up data processing.
Concurrency in Scala
Asynchronous Programming with Futures
Scala has a feature called Futures that lets you run tasks in the background. Think of a Future as a placeholder for a result that hasn't happened yet. This way, your program can keep doing other stuff instead of just waiting around.
Here's how you might use a Future to get a webpage without stopping everything else:
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
val futureResult: Future[String] = Future {
// Code to fetch web page
val webpage = fetchWebpage("http://example.com")
webpage
}
// Continue doing other work here without stopping
println("Doing other work")
// Later, you can check if the result is ready
val result = futureResult.value // Waits here if the webpage isn't loaded yet
println(result)
The cool part is your program doesn't freeze while waiting for the Future
to finish. It can keep on moving with other tasks.
Promises for Completing Futures
While a Future
is like a promise for a result that will come later, a Promise
in Scala lets you decide when that future result is ready.
import scala.concurrent.{Future, Promise}
val promise: Promise[Int] = Promise()
val future: Future[Int] = promise.future
// Set the result of the Future
promise.success(10)
// Now the Future is complete
val result = future.value // gets the result 10
Promises let you control when a Future is considered complete, based on when the result is available.
Handling Timeouts
When dealing with Futures, sometimes you want to make sure they don't take forever. You can set a timeout to cancel a Future if it's taking too long:
val future = Future {
// some long task here
}
val result = future.withTimeout(2.seconds)
// Or provide an alternate result after a timeout
val altResult = future.withTimeout(2.seconds, "Timeout!")
Using timeouts can help make sure your Futures don't get stuck.
Composing Futures
You can also set up Futures to run one after another, passing results along:
val future1 = Future {
// Some task
}
val future2 = future1.map { result1 =>
// Uses result1 to do another task
}
val future3 = future2.map { result2 =>
// Uses result2 for more tasks
}
This way, you can organize tasks to run in a specific order without blocking your program.
Building Scala Projects
Project Setup using sbt
sbt (Scala Build Tool) is a popular tool for organizing Scala projects. Here's how to get started:
-
Project structure - Usually, your code goes in
src/main/scala
and tests insrc/test/scala
. If you have configuration files, put them insrc/main/resources
. -
Build definition - Your
build.sbt
file is where you tell sbt about your project's details like its name, version, and what libraries it needs. -
Dependencies - In
build.sbt
, you can list the libraries your project needs. sbt will download them for you. You might use ScalaTest for testing or Akka for building concurrent apps. -
Plugins - sbt supports extra tools through plugins, which you can add in
project/plugins.sbt
. These can help with formatting your code or building a single file from your project. -
Configuring tasks - You can set up custom actions in
build.sbt
for compiling, testing, or running your app.
sbt keeps project setup flexible yet straightforward.
Project Setup using Maven
For Maven users, here's a quick setup guide:
-
Add the Scala Maven plugin - This plugin helps Maven understand Scala. You'll need to add it in your project's build settings.
-
Configure the Maven POM file - This file holds your project's configuration, like which version of Scala you're using and where your source code is.
-
Manage dependencies - Just like sbt, Maven can automatically fetch the libraries your project needs.
-
Build assembly JARs - To bundle your project into one big file, use the Maven Assembly Plugin.
Maven takes a bit more setup but fits well with Java projects and offers a standard project layout.
Testing with ScalaTest
ScalaTest is a testing tool for Scala and Java. It lets you:
-
Pick a test style - ScalaTest offers different ways to organize tests, so you can choose what works best for you.
-
Make assertions - Check if your code behaves as expected with simple checks.
-
Use Matchers - For more readable tests, ScalaTest has special ways to write assertions.
-
Test concurrent code - It has features for testing code that runs at the same time or in the background.
You can easily add ScalaTest to your sbt or Maven project.
Running and Packaging
You can run your Scala app with sbt run
or make a JAR file to share or deploy:
-
Standalone JAR - Use sbt or Maven to create a JAR that includes your app and all its needed libraries.
-
Deployment - You can put this JAR on a server or a cloud platform to run it.
-
Containerization - Or, you can make a Docker image with your app for easy deployment anywhere Docker is supported.
Scala apps can be run in many ways, making them very flexible for different setups.
Conclusion
Summary
We've covered a lot in this guide, starting with the very basics and moving on to more complex stuff in Scala. Here's a quick recap:
- We talked about what Scala is, its background, why it was made, and who uses it.
- We went through how to get Scala set up on your computer and write a simple program.
- We covered the basics of Scala, like how to use variables, data types, and make decisions in your code.
- We dived into functions, showing different kinds, including ones without names and ones that can be passed around.
- We explored how to use classes and objects, inheritance, and traits for organizing your code.
- We looked at pattern matching, a cool way to check what's inside your data.
- We discussed Scala's collection types, both the kind you can't change (immutable) and the kind you can (mutable).
- We talked about how to work with collections, do things in parallel, and handle tasks that run at the same time.
- Lastly, we touched on how to set up and test your Scala projects with tools like sbt and ScalaTest.
Our goal was to give you a good starting point for writing Scala code.
Where Next?
Now that you've got the basics down, here are some next steps:
- Try making your own projects to practice what you've learned.
- Dive into specific tools and frameworks like Akka, Play, Spark, and others.
- Learn about more complex Scala features and functional programming.
- Read code written by others and check out style guides.
- Get involved in the Scala community through forums and events.
Scala opens up a lot of possibilities. We hope this guide has made you ready to explore them. Keep learning and enjoy coding!
Related Questions
Is Scala easy to learn for beginners?
Learning Scala can be a bit tough at first, especially if you're new to programming. It mixes two styles of programming - object-oriented (like Java) and functional programming, which can be a lot to take in. But don't worry! With some effort and time, beginners can definitely learn Scala. It helps to start with the basics and slowly move to more complex topics. Also, there are plenty of resources like online tutorials, books, and communities that can help you along the way.
Is Scala or Python easier to learn?
Python is often seen as easier for beginners. Its simpler and clearer way of writing code makes it a favorite for many who are just starting out. Python also has tons of learning materials and a big community ready to help. Scala, on the other hand, might take a bit more time to get used to because of its dual focus on object-oriented and functional programming. However, Scala is great for building big systems that need to scale. So, your choice might depend on what you want to do - start easy with Python or aim for building large systems with Scala.
What is the introduction of Scala programming?
Scala is a programming language that runs on the Java Virtual Machine (JVM) and brings together object-oriented and functional programming. It was made to improve on Java by making code shorter, more flexible, and easier to work with. Scala is designed to be efficient and can handle big projects really well. It's smart about types but doesn't make you spell everything out, thanks to something called type inference.
Is Scala a pure OOP language?
Scala isn't just focused on object-oriented programming (OOP). Yes, it treats everything as an object, but it also embraces functional programming. This means it includes features like treating functions as values, focusing on unchangeable data, and using pattern matching to deal with data more easily. While Scala works well with Java and supports an OOP approach, it's flexible and lets you use both object-oriented and functional programming styles.