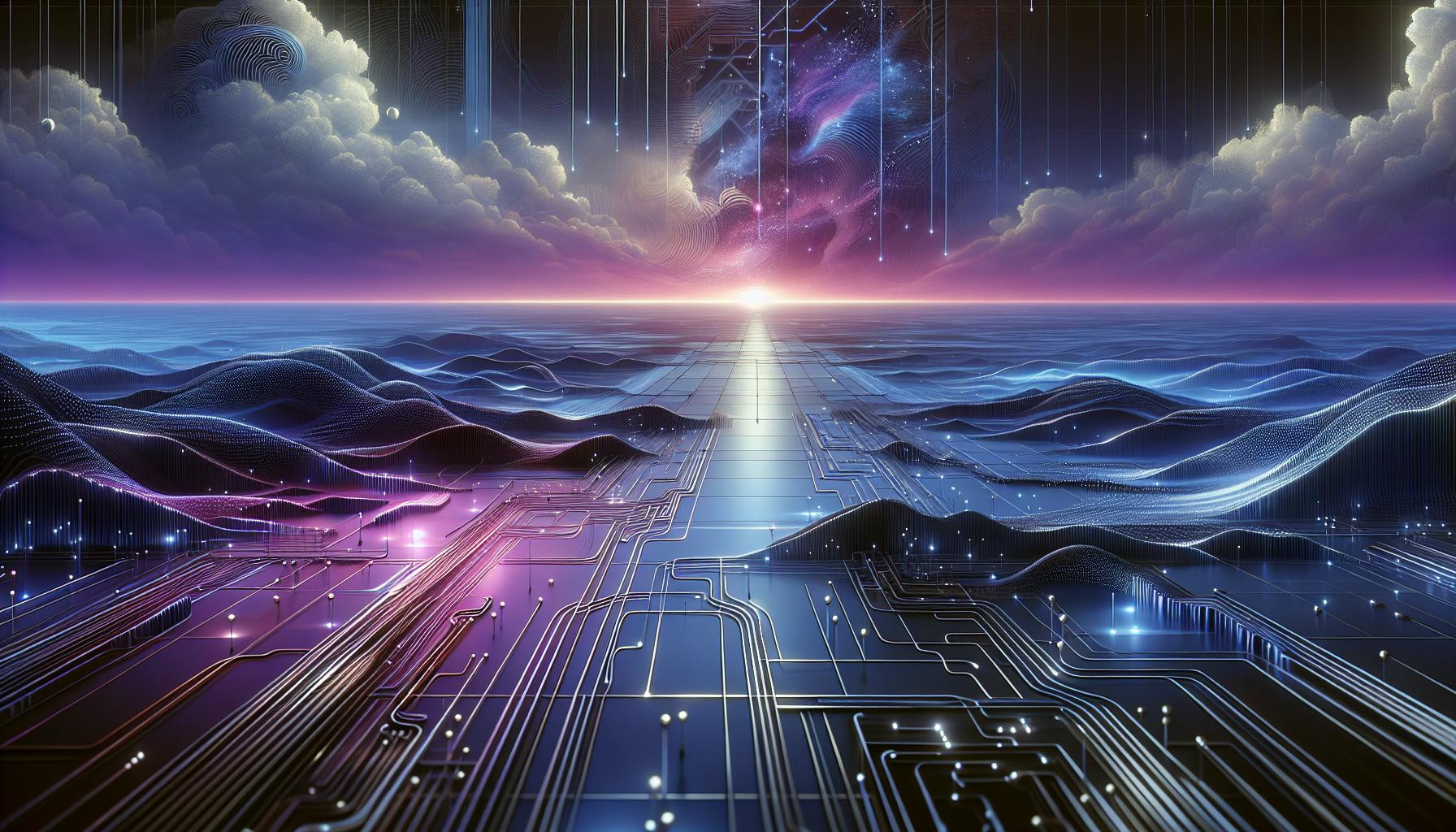
Learn about GraphQL extensions and how to implement them effectively to enhance your API capabilities. Explore schema, directive, and operation extensions, get started with Node.js and Apollo Server, and build custom features with ease.
GraphQL extensions are like add-ons or extra pages to a book, enhancing your GraphQL setup with new features without complicating the original structure. Here's a quick guide to understanding and implementing GraphQL extensions:
- Extensions let you add new details like additional fields to existing types in your schema, making your API more informative and flexible.
- Types of extensions include schema extensions for adding fields or types, directive extensions for custom instructions, and operation extensions for customizing tasks.
- Getting started requires familiarity with GraphQL basics, and setting up involves creating a Node.js project with Apollo Server.
- Implementing extensions involves configuring Apollo, working with local schemas, and possibly merging these with remote schemas for a comprehensive setup.
- Key features offered by tools like the Apollo GraphQL VS Code extension include intelligent autocomplete, inline errors, and easy navigation.
- Building custom extensions allows for adding new schema parts or authoring custom directives, enhancing your GraphQL API's functionality.
- Advanced topics cover data modeling techniques and the importance of creating shareable extension packages.
This guide aims to make your API more flexible and capable of evolving by adding new features seamlessly through GraphQL extensions.
What are GraphQL Extensions?
GraphQL extensions are bits of code that add new features to your GraphQL setup. They help you do things that GraphQL doesn't usually do on its own.
Here's what you can do with them:
- Add new types of data like Dates or JSON
- Create custom rules for things like checking if someone is allowed to see certain data
- Change how your setup acts when it runs different tasks
- Add more details to types you already have
Basically, they're like DIY kits for GraphQL that let you tweak it to fit what you need.
Types of Extensions
There are a few main kinds of GraphQL extensions you can use:
Schema Extensions
These let you change the structure of your GraphQL setup. For example, you can:
- Add more fields to types you already have
- Create new types of data
Directive Extensions
Directives are special instructions in GraphQL. With extensions, you can make your own. Some uses are:
- Checking if someone can access certain data
- Managing how data is stored or retrieved
Operation Extensions
These extensions let you tweak how GraphQL tasks (like asking for data or updating it) are done. Things you can do include:
- Keeping logs
- Tracking how fast things run
- Managing errors
In short, GraphQL extensions are your toolkit for making GraphQL work just the way you want. They let you add custom features to your setup, like changing the structure, making new rules, or tweaking how tasks are done.
Getting Started with GraphQL Extensions
Prerequisites
Before diving into GraphQL extensions, you'll need a few basics under your belt:
- A good grip on GraphQL basics like what schemas, types, fields, queries, and mutations are
- Node.js and npm ready to go on your computer
- Either Apollo Server set up or another GraphQL tool to work with
- A code editor, something like Visual Studio Code works great
It's important to be comfy with the main ideas of GraphQL and how to get a simple API up and running. Extensions are like the next step after you've got that down.
Environment Setup
Getting your setup ready for GraphQL extensions means:
- Starting a Node.js project
- Getting Apollo Server or a similar GraphQL tool installed
- Making a GraphQL schema
- Adding your extension code
Visual Studio Code is a solid choice for an editor, especially if you add the Apollo GraphQL extension. It makes things like checking your work and finding mistakes a lot easier.
Kick things off with npm init
and npm install apollo-server graphql
to get the basics installed. After that, lay out your initial schema and figure out your resolvers.
Next, bring your schema into the Apollo config file. This step helps the VS Code extension do its magic, like spotting errors early.
With these steps, you'll be all set to start crafting and using your own extensions!
Implementing Your First GraphQL Extension
Here’s a simple guide to making your first GraphQL extension to add more to your schema:
Setting Up an Apollo Config
First things first, you need to set up your Apollo config file so it knows about your schema:
- Get the Apollo CLI on your computer:
npm install -g apollo
- Sign in and pick a schema to work with:
apollo service:push --endpoint=ENDPOINT
- Make a config file:
apollo client:config
This will create a file named apollo.config.js
that has info about your schema and where it’s located.
To work with a schema that's on your own computer, just point the endpoint to your local GraphQL server’s address.
Working with Local Schemas
If you’re tweaking your schema right on your computer:
- Get your GraphQL server running (like with
npm run start
) - Set your Apollo config to point at
http://localhost:4000
- Start writing and testing your schema extensions locally
- Check out your changes in real-time using the GraphQL Playground
This setup lets you see updates fast as you work on your schema extensions.
Merging Remote and Local Schemas
When you’re working on your computer, Apollo takes care of combining your local schema changes with the remote schema automatically.
Your local updates are added to the remote schema you’ve got set up in Apollo config. This means you can work with a combined version of both schemas for your tasks.
So, you don’t need to worry about putting the two schemas together yourself—Apollo does that for you, letting your local changes show up right alongside the remote schema.
Key Features of GraphQL Extensions
Let's talk about some cool things that GraphQL extensions and tools like the Apollo GraphQL VS Code extension do to make your life easier:
Intelligent Autocomplete
Imagine your computer suggesting what to type next as you write code. That's what extensions do. They know your schema (the blueprint of your data) really well and suggest fields, arguments, and more as you type. This helps you write code faster and avoid mistakes like typing errors or using the wrong fields.
Inline Errors and Warnings
As you're writing queries (requests for data) and mutations (updates to data), the extension checks to see if you're doing it right. If there's a mistake, like a missing field or a wrong argument, it'll show you right there in your code editor. This means you can fix errors quickly and make sure everything matches up with your schema.
Navigation & Refactoring
Working with big projects can be tough, especially when you need to change or find something in your schema. The Apollo GraphQL extension helps you move around your project easily and make changes without messing things up.
It lets you quickly jump to where things are defined or see where they're used. If you need to rename something in your schema, the extension can update all the places that use it with just a few clicks. It also checks to make sure your changes won't break anything.
In short, these tools make working with GraphQL and building a schema, writing query resolvers, and managing local state a lot smoother. They help you avoid mistakes, navigate your project easily, and keep everything running smoothly.
sbb-itb-bfaad5b
Building Custom GraphQL Extensions
Registering Schema Extensions
To add new parts to your GraphQL setup, you can use something called schema extensions. This is like adding new chapters to a book without rewriting the whole thing. You can introduce new types, fields, and ways to get data (resolvers) easily.
Here's a simple way to do it using a tool called graphql-extensions
:
import { makeExecutableSchema } from 'graphql-tools';
import { schemaExtensions } from 'graphql-extensions';
const schema = makeExecutableSchema({
// ...original schema setup
});
schemaExtensions({
extensions: [
{
name: 'NewTypeExtension',
fields: {
NewType: {
newField: {
type: GraphQLString,
resolve: () => 'New field value!'
}
}
}
}
]
})(schema);
This code snippet adds a new part called newField
to an existing type. It's like saying, "Hey, let's add a new detail here." You can add more by including them in the extensions
list, making it a neat way to expand your setup.
Authoring Custom Directives
Directives are like special instructions in GraphQL that tell it how to do something during a query. You can create your own to control access, log activities, and more.
Here's how you can make a directive that logs activity:
import { SchemaDirectiveVisitor } from 'apollo-server';
class LoggingDirective extends SchemaDirectiveVisitor {
visitFieldDefinition(field) {
const { resolve = defaultFieldResolver } = field;
field.resolve = async function(...args) {
console.log(`Resolving field: ${field.name}`);
return resolve.apply(this, args);
}
}
}
To use this directive, you need to add it to your schema setup:
const schema = makeExecutableSchema({
directives: [LoggingDirective],
// ...
});
Now, you can use @logging
in your schema to start logging whenever a field is used:
type Query {
users: [User!]! @logging
}
This is a way to add special behaviors to your GraphQL setup, like keeping track of what's happening without changing your original code.
Advanced Topics
Let's dive into some more complex stuff about making your GraphQL setup even better:
Data Modeling Techniques
With GraphQL, you can organize your data in smart ways. Here's how to use some of the tools it gives you:
- Interfaces are like templates for groups of items that share common features. They're super handy when you have different things that should act the same way:
interface Searchable {
search(term: String!): [SearchResult!]!
}
type Users implements Searchable {
# ...
search(term: String!): [User!]!
}
type Products implements Searchable {
# ...
search(term: String!): [Product!]!
}
- Unions let you deal with items that could be one of several types. This is great for when you're not sure what kind of thing you'll get back:
union SearchResult = User | Product
- Type extensions let you add more info to existing items without messing up the original setup. This way, you can keep adding new stuff as you go:
type User {
id: ID!
name: String!
}
extend type User {
email: String!
}
Using these tricks, you can make your data setup really flexible and powerful.
Extension Packages & Reuse
You can make your own GraphQL extensions and share them with others. Here's how to do it:
- Write the extension and make sure it works well
- Get it ready to share by setting up the right files
- Put it up on the npm registry so others can find it
- Add it to your GraphQL projects just like any other package
Here are some tips for making good shareable extensions:
- Let people tweak them to fit their needs
- Pick clear names and stick to one main job per extension
- Explain how to use them well in your documentation
- Keep your version updates clear so people know what's changing
By making neat, useful extensions, you can help everyone build better GraphQL setups. It's all about making common tasks easier and sharing your solutions with the community.
Troubleshooting Guide
for GraphQL Extensions
Sometimes when you're working on adding new parts to your GraphQL setup, things don't go as planned. You might run into issues where parts of your setup clash, you mix up different types of data, or your custom functions (resolvers) aren't working right. Here's how to fix some common headaches:
Schema Conflicts
If you're trying to add something to a type but it's already there, you'll get an error. For example, adding an "email" field when one exists might cause:
Field "email" already exists on type "User".
Solutions:
- Make sure you're not adding something that's already there by checking your setup carefully.
- Use a unique name for your new fields, like "customEmail" instead of just "email".
- If you're adding a lot of new fields, consider grouping them under a new type, like "UserExtraInfo".
Type Mismatches
Sometimes you might use the wrong type for a field or an argument, leading to errors. For instance, using a string when you need a number might show:
Expected type Int!, found String.
Solutions:
- Double-check that the types of your fields match what you're expecting.
- If you need to change a type (like turning a string into a number), use functions that help with this, such as
parseInt()
.
Broken Resolvers
If your custom functions for fetching or changing data (resolvers) have errors or return unexpected results, you'll see errors related to resolvers.
Solutions:
- Protect your code from crashing by using try/catch blocks.
- To figure out what's going wrong, add logs to your code or use debugging tools to see the values you're working with.
- Start by returning simple, test data to make sure the basics are working before moving on to the real data.
Testing Locally
When you're developing on your computer, sometimes changes don't show up right away because they're not in sync with the main setup. This can make it hard to see if your new parts are working.
Solutions:
- Look at the Apollo server logs to see if your changes are syncing up.
- If things seem stuck, use the Command Palette to refresh your setup manually.
- Whenever possible, test using a local GraphQL server. This way, you can see your changes in real time.
In short, when adding new parts to your GraphQL setup, make sure you're not duplicating things, check your data types, handle errors in your custom functions, and keep your local testing environment in sync. With these tips, you can smooth out most issues you'll run into.
Conclusion
Think of GraphQL extensions as a smart way to add more features to your GraphQL setup as your needs grow. They make it easier to include new data or functions without messing up what you already have.
Here are the main points to remember:
- Keep things tidy. Extensions let you add new stuff without changing the original setup. This keeps your project clean and makes updates easier.
- Choose what you need. You can add just the features you want, making your setup flexible.
- Sharing helps everyone. When you make extensions that others can use, it helps the whole Apollo community. It's like sharing tools so everyone can build better stuff.
In short, learning how to use GraphQL extensions makes your API more flexible and capable of growing. Try making some extensions yourself. If you share them, you could help other developers too!
To get started, you might want to install the Apollo GraphQL extension for VS Code, connect to data sources, and practice writing query and mutation resolvers. Remember, Node.js and npm are your friends for setting everything up. And if you're looking to prove your skills, consider aiming for the Apollo Graph Developer - Associate Certification.