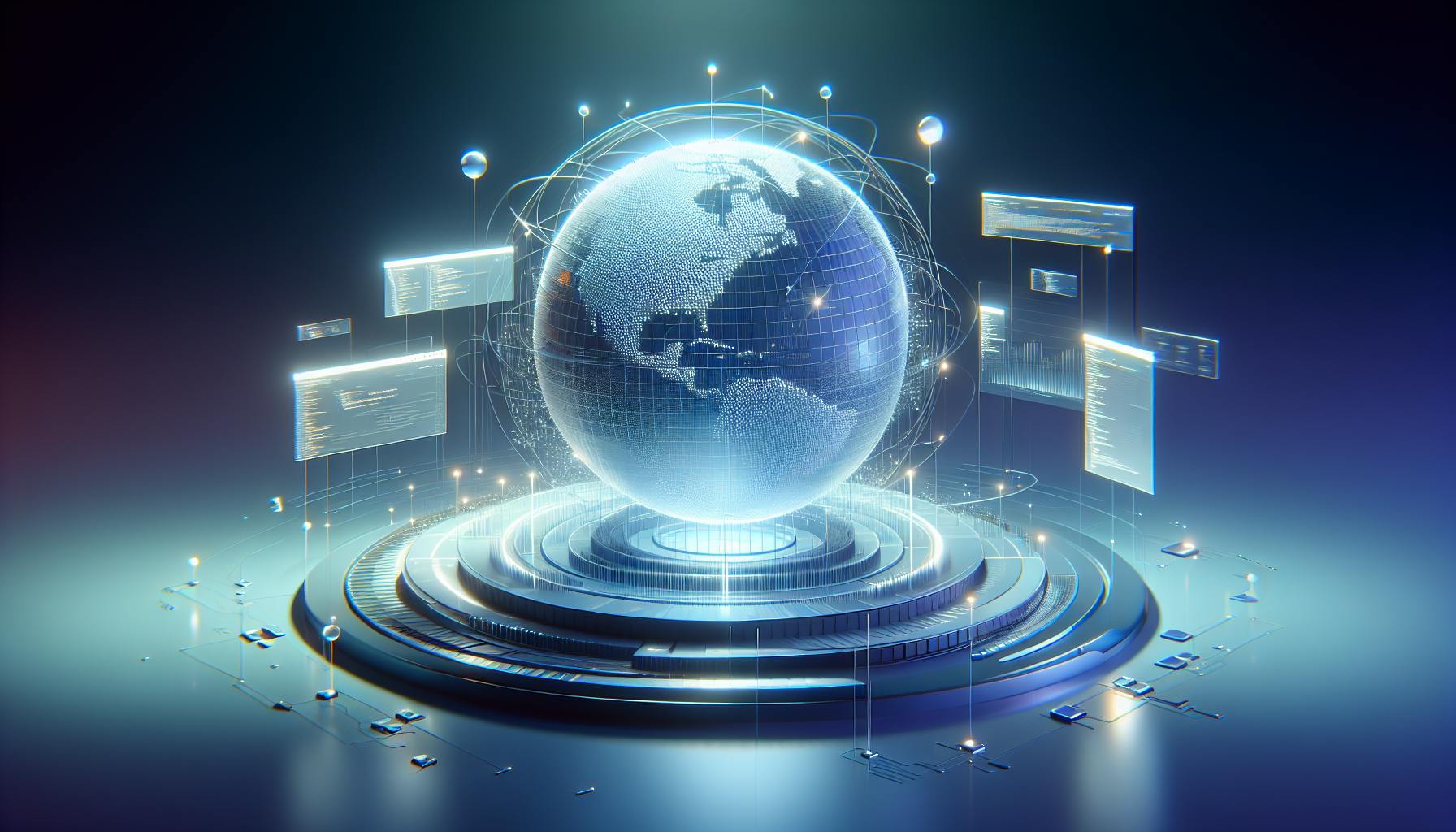
Learn how to interact effectively with Chrome's browser history as a developer. Discover key aspects like setting up permissions, using the history API, debugging techniques, and best practices.
If you're a developer working with Chrome extensions or web applications, understanding how to interact with browser history can offer valuable insights and enhance user experience. This guide covers key aspects of working with Chrome's browser history, including:
- Setting up the environment: Including enabling permissions in your
manifest.json
and ensuring user consent. - Using the history API: Learn how to add, remove, and query history items, and understand important concepts like
HistoryItem
andVisitItem
. - Debugging and advanced techniques: From searching history to analyzing visit patterns and handling large volumes of data.
- Best practices: Ensuring user privacy, secure data handling, and troubleshooting common issues.
By the end of this guide, you'll be equipped with the knowledge to leverage browser history chrome effectively, respecting user privacy and enhancing your projects.
Enabling Permissions
Some permissions are needed in your Chrome extension's manifest.json
file to work with browsing history:
- The
history
permission lets you use the history API - The
tabs
permission is for accessing the URL of the current tab - The
<all_urls>
host permission allows your extension to interact with any website the user visits
Here's an example of how to include these permissions:
"permissions": [
"history",
"tabs",
"<all_urls>"
]
Getting User Consent
You must ask users if it's okay to access their browsing history. When you ask for the history
permission, Chrome will show a message asking the user to allow or deny access.
To help users say yes, be clear about why you need this access and how it will help them. Being open and honest builds trust.
Using Incognito Mode
Remember, Chrome's Incognito Mode doesn't keep history. If your extension needs to remember websites, tell your users to turn off Incognito Mode for your extension.
Testing with DevTools
You can use DevTools to check your history features without messing up your own browsing history:
- Go to DevTools Settings > Preferences > Check "Allow in Incognito"
- Open an Incognito window to test your extension
- Close the window when done to clear any history
Getting ready to use Chrome's history features is easy if you know the steps. Always ask for permission clearly, and use the right settings to test your work. With everything set up, you can start making the most of browsing data.
Understanding the History API
The chrome.history
API is a tool for developers to work with the browser's history - that's the list of sites you've visited. It lets you do things like add or remove sites from this list, find out what sites have been visited, and look at details about these visits. Getting to know this API means you can make Chrome extensions that really understand and enhance how users browse the web.
Capabilities
Here's what you can do with the chrome.history
API:
- Adding and removing history items: With
addUrl()
anddeleteUrl()
, you can put new sites into the history or take out ones that shouldn't be there. - Querying history details: Use
search()
,getVisits()
, andgetVisitCount()
to find out which sites have been visited, when, and how many times. This is great for spotting trends in how the browser is used. - Analyzing visits: The API gives you details like how the user got to a site (like clicking a link or typing in the address) and when. This info can help you understand user habits better.
Key Concepts
To use the history API well, you need to know about:
- HistoryItem: This is info about a site that's been visited, like its URL, title, and how many times it's been visited.
- VisitItem: This gives more details about a specific visit to a site, including how the visit happened and when.
- TransitionType: This tells you what caused the visit, like clicking a link or using the back button. It's useful for figuring out why users visit certain sites.
- UrlDetails: When adding a site to the history, you can also include extra info like its title. This helps keep the history detailed and useful.
Understanding these parts of the API can help you make Chrome extensions that offer a more personalized browsing experience. It's all about getting to grips with the details and seeing how they can be used to improve how people use the web.
Using the API for Debugging
The chrome.history
API is great for developers who want to work with a user's browsing history in their browser extensions. It can help you find, add, or remove websites from a user's history list. Let's see how you can use it for different tasks.
Search History
You can use the search()
function to look through the history for specific websites or keywords. For example, if you want to find all the times someone visited 'stackoverflow' in the last week, you'd do something like this:
let oneWeekAgo = new Date().getTime() - 7 * 24 * 60 * 60 * 1000;
chrome.history.search({
text: 'stackoverflow',
startTime: oneWeekAgo
}, (historyItems) => {
// Here, you'd work with the history items found
});
This function gives you details like the website's URL, title, how many times it was visited, and when.
Add & Remove URLs
If you need to add a visit to a website to the history, use addUrl()
:
let url = 'https://github.com/dailydotdev';
let title = 'The Daily Dev';
chrome.history.addUrl({url: url, title: title}, () => {
console.log('URL added to history');
});
To remove visits, deleteUrl()
gets rid of all visits to a specific website:
let url = 'https://example.com';
chrome.history.deleteUrl({url: url}, () => {
console.log('Example.com visits deleted')
});
And if you want to clear out a specific time range, deleteRange()
does just that:
let oneWeekAgo = new Date().getTime() - 7 * 24 * 60 * 60 * 1000;
chrome.history.deleteRange({startTime: oneWeekAgo, endTime: Date.now()}, () => {
console.log('Last week of history deleted!');
});
Analyze Visits
To get more details about when and how a site was visited, use getVisits()
:
let url = 'https://github.com/dailydotdev';
chrome.history.getVisits({url: url}, (visitItems) => {
visitItems.forEach((visit) => {
console.log(visit.transitionType);
console.log(visit.visitTime);
});
});
This tells you the way the visit happened (like clicking a link) and when it happened.
With the history API, you can do a lot to work with and understand a user's browsing history. Whether it's for debugging, privacy, or just making your extension smarter, these tools are very useful.
Advanced Techniques
TransitionTypes
When you use the history API, it keeps track of how people get to different websites using something called transition types. This is really helpful when you're trying to figure out what people do on your site.
Here are a few important transition types:
"link"
: This means someone clicked on a link to get to a page. It's great for seeing how people move around your site."typed"
: This is when someone types the website's address directly into the browser. It shows you who really wants to visit your site."auto_bookmark"
: This happens when someone visits a page from their bookmarks. It's good for understanding which pages people like enough to save.
Here's a simple way to see these types:
chrome.history.getVisits({url: "https://github.com"}, (visits) => {
visits.forEach((visit) => {
console.log(visit.transition); // Shows the transition type
})
});
By looking at transition types, you can get a good idea of how people find and use your pages. Then, you can make your site better based on this info.
History Events
The history API lets you know when two main things happen with browsing history:
onVisited
: This is when someone goes to a new URL. It's perfect for doing things right away, like showing notifications.onVisitRemoved
: This happens when visits are taken out of the history. It's good for times when you need to clean up, like getting rid of cached images.
For example, to show a notification when someone visits GitHub:
chrome.history.onVisited.addListener((result) => {
if (result.url.includes("github")) {
chrome.notifications.create(/* ... */);
}
});
And if you need to clear cached images when a site's visits are deleted:
chrome.history.onVisitRemoved.addListener((removed) => {
clearCachedImages(removed.urls);
});
With these events, you can make extensions that react to what people do in their browsing history. It's a smart way to keep your extension up-to-date with how people use the web.
Integrating with Extensions
Making your Chrome extension work with browser history isn't too hard. Here's what you need to know:
Requesting Permissions
First up, if your extension wants to look at or change the browser's history, you need to ask for permission. In your extension's manifest.json
file, add:
"permissions": [
"history"
]
This lets your extension use the chrome.history
API. Remember to tell your users why you need this permission.
History API
With the chrome.history
API, your extension can do things like:
- Look up past browsing with
search()
- Add or take away history items using
addUrl()
anddeleteUrl()
- Know when new sites are visited with
onVisited
For instance, to do something when someone visits a GitHub page:
chrome.history.onVisited.addListener((result) => {
if (result.url.includes("github.com")) {
// Action when GitHub is visited
}
});
Content Scripts
You can also use content scripts to interact with browser history. This means you can add features right on the websites people visit.
For example, to show browsing history on GitHub:
// contentScript.js
chrome.history.search({text: "github.com"}, (history) => {
// Show history details for GitHub
});
Other APIs
Don't forget, you can mix the history API with others like tabs
, notifications
, and browsingData
. This way, you can delete history, manage browser tabs, or send notifications about visited sites.
Testing
When testing your extension, especially with private browsing data:
- Use test accounts and data that aren't personal
- Turn on your extension in Incognito mode to check it without messing with your own history
- Clear your cache and history often to keep things clean
By following these steps, you can make extensions that really understand and improve how users browse the web.
sbb-itb-bfaad5b
Best Practices
When you're adding browser history features to your Chrome extensions, it's key to think about asking for the right permissions, keeping user information safe, and handling data carefully. Here's how to do it right:
Permissions
- Only ask for the
"history"
permission when you really need it to access the browsing history. Don't ask for more than you need. - Be clear about why you need access to the history and what you'll do with it when you ask users for permission. This helps build trust.
Privacy Considerations
- Always get clear permission before using someone's browsing history.
- When storing data, it's safer to use codes or encryption instead of just writing it out.
- Make sure you're following privacy laws, especially for users in Europe (GDPR).
Secure Data Handling
- Avoid sending full browsing history to any outside servers. If you must send data, only send summaries or coded data.
- Keep any user data you store safe by encrypting it, not just keeping it in plain text.
- Make sure to delete any history data you have if someone uninstalls or turns off your extension.
Limit Access
- Use specific functions like
search()
that only get the information you need, rather than getting everything withgetVisits()
ordeleteAll()
. - Only look at the necessary parts of the history, not everything.
- When searching history, only look back a short, set amount of time instead of all of it.
Comparison Table
Here's a simple table to help you see what to avoid and what to do instead when working with the history API:
Pitfall | Best Practice |
---|---|
Using deleteAll() too much |
Use deleteUrl() and deleteRange() for specific deletes |
Asking for too many permissions | Just ask for the "history" permission |
Keeping full history | Use codes or encrypt data, and only keep necessary info |
Using plain text | Store data with encryption |
Sending data to servers | Keep stats local and coded |
Accessing everything | Use search() for specific needs |
No date limits on searches | Set a fixed time range |
By sticking to these guidelines, you can make extensions that are safe, respect privacy, and only use the history information that's really needed.
Troubleshooting
When you're working with Chrome's history in your extensions, you might run into some problems. Here's a quick guide on fixing common issues:
Permission Errors
If you see messages saying you can't use the history API, make sure of a few things:
- You've listed the
"history"
permission in yourmanifest.json
file. - If you're using other features like
tabs
, check those permissions too. - Make sure your extension actually asks people if it can use their browsing history.
Getting the permissions right is key to making everything work.
Search Isn't Working
If your search()
function isn't finding what you expect, double-check:
- Your search terms are correct.
- You're not looking over too long a time; try narrowing the search period.
- You're handling the results properly in your code.
Make sure you're searching wisely and managing the results correctly.
History Not Updating
If new sites aren't showing up in the history, it could be because:
- The user is in Incognito Mode, which doesn't save history.
- Your extension might be stuck on old data.
- The websites visited might not be loading correctly.
Ask users to switch off Incognito Mode for tests, and try clearing any old data.
Can't Delete History
If you're struggling to delete history items, ensure:
- You have the
"history"
permission. - You're using the correct URL or time frame with
deleteUrl()
ordeleteRange()
. - Your code correctly handles what happens after the delete action.
Check your delete commands and permissions closely. Start with deleting single items before moving to larger batches.
Handling Lots of History Data
Dealing with a ton of history data? Here's what to do:
- Only ask for the data you really need from the API.
- Use
search()
to narrow down the data you're getting. - Summarize the history data instead of showing every detail.
- Show just a part of the history and let users click through pages for more.
Limiting how much history you deal with at once can make things much easier to manage.
By carefully checking your setup and adjusting how you handle data, you can get past most issues with the history API. Keep users informed about any problems and use these tips to keep your history features running smoothly.
Conclusion
Chrome's browser history tools are like a treasure chest for developers. They help us understand what users do, find problems, and make things better. Here's a quick recap of what we talked about:
- The history API lets us peek into details like when someone visited a site and how they got there. This is super useful for figuring out how users move around.
- We can do stuff like search for specific visits, check out details, or clean up the history with special commands.
- We also talked about some fancy moves for tracking how users get to sites, responding to changes in the history, and dealing with a lot of data.
- When making Chrome extensions, we saw how to ask for the right permissions and use different parts of the API and scripts to add cool features.
- And we touched on the importance of being careful with permissions, keeping user data safe, and only accessing what we really need.
Using the history API is pretty powerful because it lets us see how people browse. But we have to be careful. It's important to think about privacy and make sure users are okay with us accessing their history. If we use this tool wisely and with the user's permission, it can help us make better apps. Just remember to always respect privacy and handle data the right way.
This guide is a starting point for diving into Chrome's history features. We've shared some tips and tricks, but also a reminder to think about the privacy of users. If we use these tools thoughtfully, they can help us understand our audience better. Just be sure to always ask users for permission and use the data responsibly.
Related Questions
Where do I find Chrome browser history?
- Open Chrome on your computer.
- Click on the three dots in the top right corner.
- Choose History.
- Select the items you want to delete from your history by checking the box next to them.
- Click on Delete at the top right.
- Confirm by clicking Remove.
How do I retrieve deleted history on Chrome?
- Open Chrome and check if your Google accounts are synced by going into settings.
- If they are synced, log out and then log back in to get your search history back.
- Look under Data & Privacy for Activity and Timeline.
- Click on My Activity to find your deleted history.
How do I see all my Google history?
- Go to your Google Account.
- Click on Data & privacy on the left.
- Under "History settings," select My Activity.
- Use the search bar and filters at the top to find specific activities.
How do I look at my browser history?
- On your Android, open the Chrome app.
- Tap on the three dots in the top right, then tap History.
- To visit a site from your history, just tap on it. To open it in a new tab, press and hold the entry, then tap More.