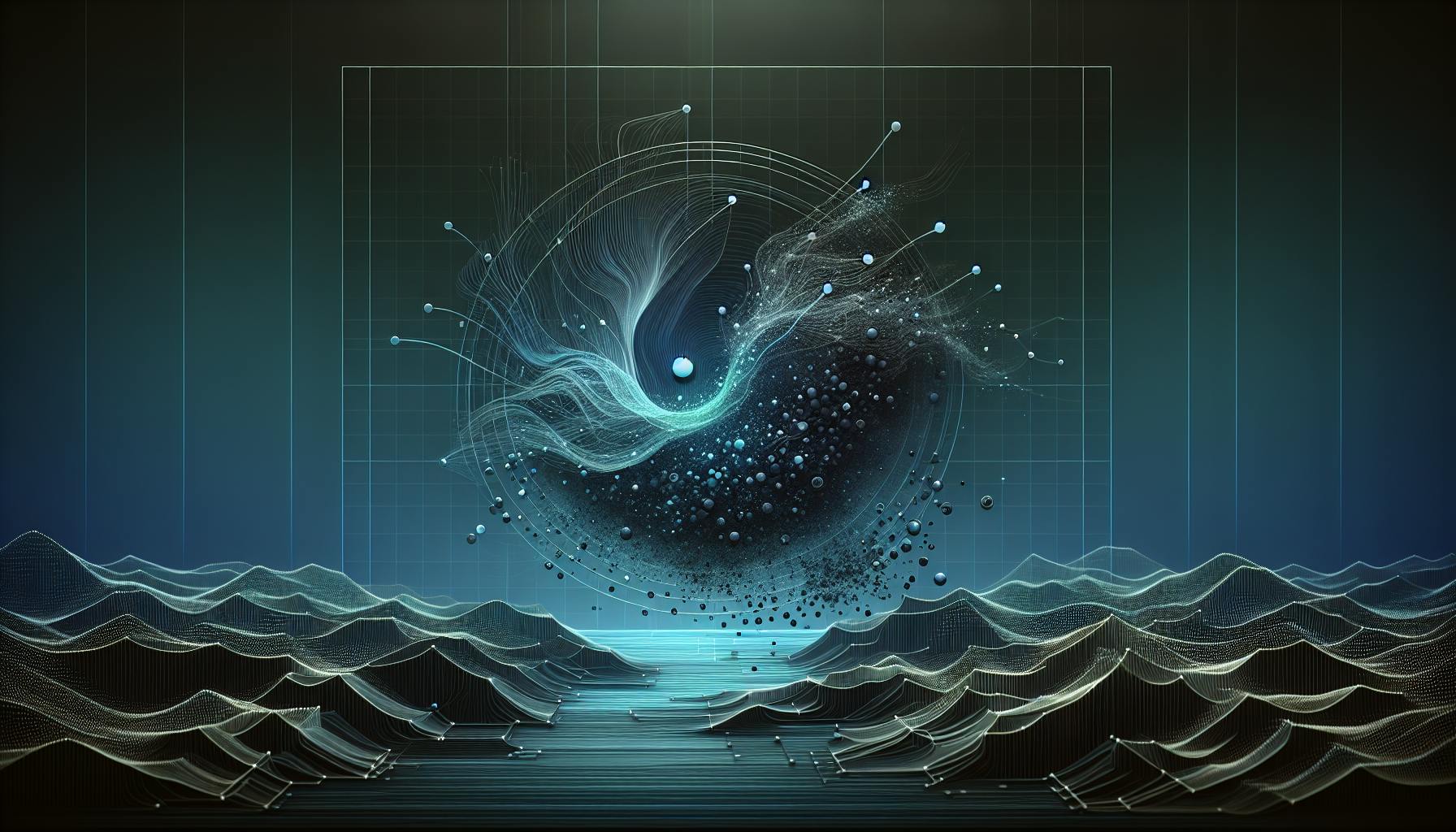
Learn about Async GraphQL basics for beginners, including speed, efficiency, setting up your environment, implementing an Async GraphQL server, and tackling advanced topics for optimized performance.
Async GraphQL revolutionizes data fetching in web apps, offering speed, efficiency, and the ability to handle multiple requests concurrently. This guide introduces you to the basics of Async GraphQL, covering its advantages like better resource use and simpler code, and guiding you through setting up your environment, implementing an Async GraphQL server, and tackling advanced topics for optimized performance. Whether you're new to GraphQL or looking to upgrade your skills, this guide provides a comprehensive overview of Async GraphQL's core concepts, practical applications, and best practices for secure, scalable, and fast web applications.
- Speed and Efficiency: Handles multiple requests at once, improving performance.
- Resource Use: More efficient, allowing servers to manage more users simultaneously.
- Simpler Code: Async operations lead to cleaner, more maintainable code.
- Setting Up: Choose the right tools and languages like Rust, JavaScript, or Go.
- Implementing: A step-by-step guide to creating an Async GraphQL server.
- Advanced Topics: Tips on database access, security, real-time updates, and performance analysis.
This guide is designed to help beginners grasp the essentials of Async GraphQL, enabling the development of faster, more efficient web applications.
What is GraphQL?
Imagine you could ask a server for exactly what data you want and get it in one go. That's what GraphQL does. Here's why it's cool:
- It lets you ask for specific information in one request, so you don't have to keep asking for bits and pieces. This means less waiting.
- The server has a set of rules (or a type system) that helps make sure your requests make sense. This keeps things running smoothly.
- The way you ask for data looks a bit like a shopping list, which makes it easy to understand.
- Tools like GraphiQL make it even easier by helping you write your requests with suggestions and highlighting.
In short, GraphQL makes getting data simpler and more efficient, saving time and hassle.
Why Use GraphQL?
There are a bunch of reasons why GraphQL is great for getting data:
- Less back and forth - You can get all the data you need in one request, and you only get what you ask for. This makes everything faster and cleaner.
- Great tools - Because everything is set up in a specific way, there are helpful tools that make writing and checking your requests easier.
- Works with everything - GraphQL isn't picky about what programming language or database you're using. This makes it super versatile.
- Easy updates - You can add new features to your API without messing up what's already there. This means your app or website can grow and change without big headaches.
- Easier to figure out - The set of rules (schema) acts like a guide, making it easier to understand how to get the data you need. Plus, the tools available make exploring different parts a breeze.
Overall, GraphQL takes away a lot of the pain of getting data for your app or website. It's designed to make things faster, more efficient, and simpler for everyone involved.
The Shift to Asynchronous Operations
Asynchronous operations are becoming a big deal in making websites today. They let websites do many things at once instead of one by one, which makes them faster and able to handle more people without slowing down. This part will look at the difference between the usual way of doing things (synchronous) and the new, faster way (asynchronous), focusing on how they perform and how complicated they are.
Synchronous vs. Asynchronous GraphQL
Metric | Synchronous GraphQL | Asynchronous GraphQL |
---|---|---|
Throughput | Lower (handles requests one by one) | Higher (handles many requests at the same time) |
Latency | Higher (requests have to wait) | Lower (requests are handled right away) |
Resource Use | Less efficient (waits while working on requests) | More efficient (keeps working while handling requests) |
Complexity | Simpler (things happen one after another) | More complex (has to manage many things at once) |
The usual way, or synchronous GraphQL, deals with requests one at a time. This can make things slow because requests have to wait their turn.
Asynchronous GraphQL, on the other hand, can do lots of things at the same time without waiting. This means it can handle more people faster, uses resources better, but it's a bit trickier to manage because there's a lot going on at once.
Core Concepts of Async GraphQL
Here are some basic ideas you need to know about Async GraphQL:
- Non-Blocking Operations - It does things in the background without stopping the main work. This means more things can happen at the same time.
- Promises - These are like IOUs for operations that will finish later. They help avoid a mess of callbacks.
- Futures - Similar to promises, but with extra features like being able to cancel them.
- Event Loop - Keeps track of what's waiting to be done and takes care of them when they're ready. It helps keep everything running smoothly together.
Understanding these ideas is important to use Async GraphQL well. Building your website around these fast, simultaneous actions can make it much faster and able to handle more visitors. However, it does make the code a bit more complicated, so you need to plan how to handle all these simultaneous actions carefully.
Setting Up Your Environment for Async GraphQL
To start making apps with Async GraphQL, you'll need to pick the right programming languages, frameworks, libraries, and tools. Here's a simple guide to help you choose:
Languages
Rust is a top pick for working with Async GraphQL because it's really good at handling tasks at the same time without causing problems. It's also great for writing clear and efficient code. Other languages you might consider are:
- JavaScript/TypeScript - These are very popular and flexible, with good support for asynchronous operations.
- Go - Known for its built-in ability to handle multiple tasks at once.
- Elixir - A language that's really good at doing many things at once because of its functional approach.
Frameworks
For Rust users, Actix and Axum are two web frameworks that work well with Async GraphQL. If you're using other languages, here are some alternatives:
- ASP.NET Core for C# users offers good support for GraphQL.
- Apollo Server is a good choice for those using Node.js with JavaScript or TypeScript.
Libraries
- async-graphql is a Rust library specifically for Async GraphQL.
- Apollo is a set of tools for both the server and client side in JavaScript.
- Sangria is a library for Scala developers working with GraphQL.
IDEs
- Visual Studio Code has great support for Rust and JavaScript.
- JetBrains Rider is packed with features for .NET developers.
- IntelliJ IDEA supports Scala and Java and has a plugin for GraphQL.
Other Tools
- GraphiQL and GraphQL Playground are tools for testing your GraphQL queries.
- Apollo Studio helps manage your GraphQL setup in the cloud.
- OpenTelemetry is useful for finding and fixing issues by tracking how data moves through your app.
Combining Rust with Actix or Axum for the backend and using Apollo for GraphQL on the Node.js and the front end is a strong setup. It lets you use Rust's strengths in handling asynchronous operations while also taking advantage of JavaScript's vast ecosystem. The recommended IDEs and tools will help you write, debug, and keep an eye on how well your app is running.
With these tools and languages, you'll be all set to build fast and efficient apps using Async GraphQL. If you have any questions, feel free to ask!
A Step-by-Step Guide to Implementing Async GraphQL
Quickstart Guide
Here's a simple way to get your Async GraphQL server working quickly using Rust:
- First, add some necessary pieces to your project:
[dependencies]
async-graphql = "2.0"
tokio = { version = "1", features = ["macros"]}
- Next, lay out your GraphQL setup - this includes types, queries, and mutations:
struct QueryRoot;
#[Object]
impl QueryRoot {
async fn hello(&self) -> &str {
"Hello world!"
}
}
- Now, make an Async GraphQL schema instance:
let schema = Schema::build(QueryRoot, EmptyMutation, EmptySubscription)
.data(context)
.finish();
- Set up a Warp server to handle requests:
let route = warp::post()
.and(warp::path("graphql"))
.and(warp::graphql(schema.clone()));
warp::serve(route)
.run(([127, 0, 0, 1], 3030))
.await;
- Send a query and see the JSON response:
query {
hello
}
// Result:
{
"data": {
"hello": "Hello world!"
}
}
This is how you set up a basic Async GraphQL server with Rust. It's ready to handle queries and send back JSON responses.
Testing Your Async GraphQL Application
Here's how to check if your Async GraphQL application is working right:
Unit Testing Resolvers
- Use fake data and contexts in tests
- Make sure the returns match what your schema says
- Check how it deals with errors
#[tokio::test]
async fn resolver_hello_world() {
let resolver = QueryRoot;
let result = resolver.hello(&None).await;
assert_eq!(result, "Hello world!");
}
Integration Testing
- Start a test version of your GraphQL server
- Send some test queries and mutations
- Make sure the answers you get are what you expect
let test_server = serve_graphql(schema);
let client = Client::new(test_server.url());
let res = client.query(hello_query).await?;
assert_eq!(res.data.hello, "Hello world!");
Schema Validation
- Use tools like valico to check your schema
- Look at field types, arguments, and connections
- Make sure changes to your schema don't mess up what's already working
Testing your Async GraphQL app properly is key to making sure it works well. This includes checking individual parts, how they work together, and that changes don't cause problems.
sbb-itb-bfaad5b
Advanced Topics in Async GraphQL
Async GraphQL can do some really cool things, but it also comes with its own set of challenges. Let's dive into some more complex areas you'll come across as you start building bigger and better Async GraphQL apps.
Efficient Database Access and Caching
When you're dealing with lots of queries at the same time, your database needs to keep up. Here are a few tips to help:
- Use async database drivers like
sqlx
ortokio-postgres
if you're using Rust. These help avoid delays by managing connections well. - Think about using a graph database like Neo4j. It keeps data in memory for quick access.
- DataLoader can help by grouping similar data requests together and remembering previous fetches.
- Setting up Redis can help remember query results, so you don't have to ask the database every time.
Securing Your API
Keeping your API safe is super important:
- Use JWT tokens for authentication instead of sessions.
- Make a custom Authorize directive to check if users can access certain data.
- Prevent users from asking for too much data at once by limiting query depth and complexity.
- Use rate limiting based on API keys to stop misuse.
Real-Time Updates with Subscriptions
Subscriptions keep your users updated in real-time:
- Use a PubSub system like Redis or Apache Kafka to send out updates.
- Your schema needs to explain what kinds of updates can happen.
- Handle authentication tokens during the WebSocket handshake.
- Make sure your usual HTTP session logic works with WebSockets too.
Analyzing and Improving Performance
More requests mean you need to watch out for slow spots:
- Look for slow resolver functions and figure out why they're lagging.
- Use tools like Apollo tracing extension or OpenTelemetry to find problems.
- Test your app under heavy load with tools like k6 or Artillery.
- Keep an eye on CPU, memory, and database usage to find bottlenecks.
When you're working with Async GraphQL, thinking about database speed, keeping things secure, updating users in real-time, and checking on performance are all key. Planning for these things from the start helps everything run smoothly as your app grows.
Conclusion
Async GraphQL makes apps faster and more efficient but also brings new challenges. Here's a quick summary and some advice for making your apps better.
The Benefits of Going Async
Speed and Efficiency
With Async GraphQL, your app can handle many users at once, making everything run smoother and quicker. It's like having a super-efficient server that doesn't waste time or space.
Scalability
Your app can grow and still stay fast. You won't need to keep adding more servers because one server can take on more work.
Simpler Code
Using async libraries, especially with languages like Rust and JavaScript, makes your code easier to understand. It's all thanks to tools like futures and promises that help manage the work.
Tips for Smooth Development
Plan Ahead
Think about which parts of your app will use async and design it with that in mind.
Test Thoroughly
Make sure to test your app in different ways - check each part, see how the whole system works together, and make sure any changes don't cause problems.
Monitor Performance
Use tools to keep an eye on how your app is doing. This helps you find any slow spots before they get in the way.
Take Security Seriously
Start with good security - make sure only the right people can get in, limit how much data can be asked for at once, and control how often someone can ask for data.
With careful planning, testing, and keeping an eye on how things are running, you can create awesome Async GraphQL apps that are quick, efficient, and safe. Async GraphQL really changes the game for modern apps if you do it right!
Let me know if you have any questions. I'm here to help!
Related Questions
What is async in GraphQL?
Async in GraphQL means that the parts of the server that get data can do so without stopping everything else. This means the server can do more things at once, making it faster and more efficient. For instance, a part of the server can ask for information from a database and move on to other tasks while waiting for that information.
What is GraphQL for beginners?
GraphQL is a special way to ask for data from a server. It lets you ask for exactly what you need, no more, no less. It uses a system of types to describe the data, which helps make things more organized and efficient. If you're just starting, you begin by setting up how your data is structured (your schema) and how to get that data (your resolvers), then you can start asking for data.
What is @KEY in GraphQL?
The @key directive in GraphQL is used to tell which field(s) in a type are unique, like an ID. This helps make sure each piece of data is unique and not duplicated. For example, using @key(fields: "id")
means the id
field is what makes that piece of data unique.
How to implement GraphQL in Python?
Here's a simple way to use GraphQL with Python:
- Get a GraphQL library like Graphene.
- Set up your data types and overall structure (schema).
- Make resolvers to get the data you need.
- Use a web server like Flask to run everything.
- Make a special spot (endpoint) where you can ask for data.
- Start sending requests to get data and handle the answers.
After setting it up, you can work on making it better by testing and finding ways to improve.