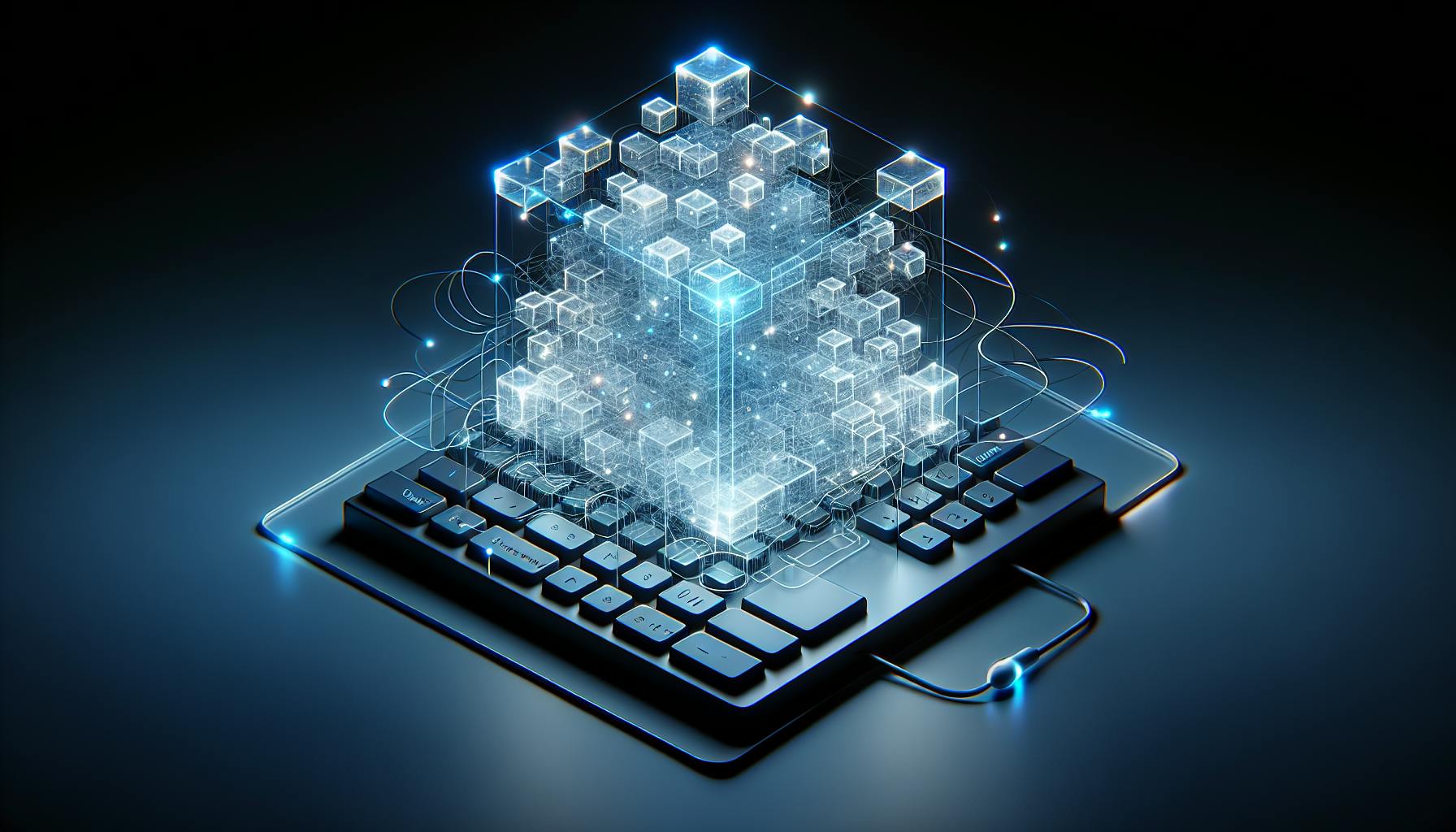
Learn about the essentials of using arrays, sets, maps, and weak maps in JavaScript for efficient data management. Explore real-world examples and best practices for developers.
If you're diving into JavaScript or looking to brush up on your skills, understanding collections—arrays, sets, maps, and weak maps—is essential. These tools are fundamental for organizing and managing data efficiently in any project. Here's a quick overview:
- Arrays help you store items in an ordered list.
- Sets ensure all elements are unique, perfect for eliminating duplicates.
- Maps allow for quick key-value pair storage and retrieval.
- WeakMaps offer a way to store key-value pairs with keys that are weakly referenced, which helps in memory management.
Using these collections effectively can lead to cleaner code, faster execution, and more manageable memory usage. This guide will take you through the essentials of using each type, with practical tips and real-world examples to help you apply these concepts in your projects.
Key Points:
- Understand the purpose and use of each collection type.
- Learn how to declare, initialize, and manipulate collections.
- Discover best practices and common pitfalls to avoid.
- Explore real-world applications to see how these collections can solve common programming challenges.
Whether you're creating a shopping cart in React or implementing a user profile search, mastering JavaScript collections will equip you with the skills to handle data more effectively in your web applications.
Why Collections Matter for Developers
Using JavaScript collections the right way helps you:
- Store and find data quickly
- Make changing data simpler
- Keep your code running fast
- Make your code easier to read
- Save on memory
- Avoid mistakes
For developers, being good at using things like filtering, mapping, and reducing with collections means you can write code that's easier to handle and grows better. Collections are at the heart of working with data in JavaScript. Learning them well is a big win for any developer.
Mastering Arrays
Arrays are super useful in JavaScript because they let you store and organize data in a way that keeps everything in order. Let's dive into how to make the most of arrays in your code.
Declaring and Initializing Arrays
Starting with arrays is easy. You can either use the Array
constructor or just square brackets:
let fruits = new Array('apple', 'banana', 'orange');
let veggies = ['tomato', 'onion', 'carrot'];
If you don't know what to put in an array yet, you can still set up one with a certain size:
let nums = new Array(5); // holds 5 undefined elements
To access or change items in an array, you use the index (where the item sits in the array):
fruits[0]; // 'apple'
fruits[1] = 'mango';
Arrays have a length
property that tells you how many items are in them.
Manipulating Array Elements
There are lots of tools for adding, removing, or changing items in arrays:
push
/pop
- add/remove items at the endunshift
/shift
- add/remove items at the beginningsplice
- add or remove items anywheremap
- change each item in some wayfilter
- keep only the items that meet a conditionreduce
- combine all items into oneconcat
- join arrays togetherslice
- take a part of the array
Here's a simple way to use map
:
let prices = [5, 10, 15];
let newPrices = prices.map(price => price * 2); // [10, 20, 30]
By using these tools together, you can do a lot with just a little bit of code.
Arrays vs Other Collections
Arrays are not the only way to store data. Sets and maps are also options:
- Sets - They make sure every item is different. Great for when you don't want duplicates.
- Maps - These are like dictionaries that pair keys with values, making it easy to find what you need.
So, when should you use arrays over sets or maps?
- Use arrays when the order of items is important, like making a list.
- Use sets when you want to make sure there are no repeats.
- Use maps when you need to find things quickly with a key.
In short, getting good at using arrays is key to working with data in JavaScript. It makes your code simpler and faster.
Leveraging Maps and Sets
Maps and sets are handy tools in JavaScript for keeping track of your data. Let's break down how to use them in a way that's easy to understand.
Introducing JavaScript Maps
Maps let you store pairs of keys and values. This is great for when you need to find something fast later on. Here's a simple way to start using them:
// Create a map
const fruitCalories = new Map();
// Add key-value pairs
fruitCalories.set('apple', 95);
fruitCalories.set('banana', 105);
// Get a value by key
const appleCals = fruitCalories.get('apple'); // 95
// Check if map has a certain key
if(fruitCalories.has('orange')) {
// ...
}
// Other handy things you can do
fruitCalories.size; // Tells you how many pairs are in the map
fruitCalories.clear(); // Removes everything from the map
Things to remember:
- You can use anything as keys or values
- Finding things by key is super quick
- Every key is unique
Maps are great for when you need quick access to your data and want to keep things tidy.
Understanding Sets
Sets are all about keeping values unique:
// Create a set
const ids = new Set();
// Add values
ids.add(1);
ids.add(2);
// Check if a value is in the set
ids.has(1); // true
// Remove a value
ids.delete(2);
// Other handy things you can do
ids.size; // Tells you how many values are in the set
ids.clear(); // Removes everything from the set
Things to remember:
- All values in a set are different from each other
- Looking things up is quick
- You can put any type of value in a set
Sets are perfect when you want to make sure there are no duplicates.
Maps vs Sets vs Objects
Collection | Use Case | Pros | Cons |
---|---|---|---|
Map | Lookup by key | Fast access | Can be memory intensive |
Set | Removing duplicates | Simple syntax | Limited functionality |
Object | Ad hoc data storage | Built-in feature | No duplicate keys |
So, in simple terms:
- Use maps when you need to find things quickly with a key
- Use sets when you want everything to be unique
- Use objects for basic data storage
Getting to know how to use maps and sets, along with arrays, gives you more control over your data and can make coding a lot smoother.
Advanced Collection Types
Introducing WeakMaps and WeakSets
WeakMaps and WeakSets are special versions of Maps and Sets in JavaScript. The big difference is they don't hold onto their items strongly. If no one else is using an item, it can be thrown away to free up space. This is great for keeping your app's memory clean.
Why you might use WeakMaps and WeakSets:
- To temporarily keep big stuff without worrying about memory waste.
- To attach extra info to objects without actually changing them.
- To keep track of things related to web page elements without stopping them from being cleaned up when not needed.
Just remember, items in WeakMaps and WeakSets can vanish when not used, so always check if they're there before you use them.
Implementing Keyed Collections
Maps, Sets, WeakMaps, and WeakSets help you organize your data smartly:
Fast Lookups
Finding stuff by its key is super quick, which is awesome for when you need to grab something fast, like in a cache or a lookup table.
// Map for keeping users with userIds
const userCache = new Map();
function getUser(id) {
return userCache.get(id);
}
Removing Duplicates
Sets make sure every item is different, perfect for when you're making lists or filters and don't want repeats.
const tags = new Set();
posts.forEach(post => {
post.tags.forEach(tag => tags.add(tag))
});
// Now, tags has a unique list of all tags
Metadata Storage
WeakMaps let you attach info to objects without changing the objects. This is handy for settings or temporary data.
const userMeta = new WeakMap();
function setUsername(user, name) {
userMeta.set(user, { username: name });
}
Choosing the right tool (map, set, etc.) for your data can make handling it a lot easier. Getting good at using these will help you manage data more effectively.
sbb-itb-bfaad5b
Collection Patterns and Best Practices
Choosing the Right Collection Type
When dealing with data in JavaScript, picking the right tool for the job is crucial for making your code work better. Here's a quick guide:
- Use arrays when the order matters, like for lists. They're good because you can quickly add or remove items from the end.
- Use sets when you want to make sure all your items are different and you don't care about the order. Sets are great for checking if something is already there.
- Use maps for when you need a quick way to match keys and values. They're perfect for things like caches.
- Use objects for simple data storage, but remember they're not as flexible as maps or sets for certain tasks.
- Use WeakMaps for when you want to link extra info to objects without messing with memory cleanup.
- Use WeakSets if you need to keep track of objects without stopping them from being cleaned up when not needed.
Choosing the right type of collection helps your program run more smoothly by using memory and processing power wisely.
Writing Optimal Collection Code
Here are some tips to make your collection code better:
- Size your arrays and sets right from the start to avoid making them bigger later, which can slow things down.
-
Try not to change collections too much in parts of your code that run a lot. Instead of adding or removing items, consider other ways like using
.concat
or.slice
. - Stick with unchangeable data when possible. This way, you won't accidentally mess up something elsewhere in your code.
- Spread syntax is a cool trick for changing one collection type to another easily.
- Pull apart items for use instead of reaching into them with indexes.
-
Chain commands like
.map().filter()
to keep your code neat and avoid extra variables.
Following these tips can make your code run faster because it uses less memory and power.
Common Collection Pitfalls
Watch out for these common mistakes with collections:
-
Changing arrays while using them can lead to weird results. If you need to change an array while going through it, make a copy first with
.slice()
. - Holding onto collections too long can cause memory leaks. Make sure you're not keeping collections around when you don't need them.
- Sharing data by accident can happen when you use arrays or objects. Using unchangeable data can help avoid this problem.
- Using objects as maps might seem easy, but real Maps are better because they handle different types of keys more effectively.
Avoiding these traps will help keep your code bug-free and running smoothly.
Applying Collections: Real-world Examples
React Shopping Cart
Let's talk about making a shopping cart in React. This is a cool way to use arrays and a thing called localStorage to keep track of what's in the cart, even if you close the page. Here's a simple plan:
- Keep a list (
products
array) with all the product info like name, price, and picture. Show these on the page using.map()
. - For the cart, have another list (
cartItems
) where each item hasproduct
andquantity
. Use this to show what's in the cart. - When someone adds or removes something from the cart, update
cartItems
and save it tolocalStorage
. - When the page loads, check
localStorage
to see if there's a cart already and use that.
This method keeps the important info in easy-to-use lists and uses localStorage for remembering the cart. It's a smart way to make the cart work well and easy to change.
User Profile Search
Making a search feature for user profiles can be made better with a Map.
- Get user data like
{id, name, email, avatar}
from an API. - Put this info into a Map, using
id
as the key. - For searching, take names into an array.
- Instead of going through the whole list every time, just look in the Map for names that match.
Why this is great:
- Finding users by ID in a Map is quicker than looking through a list.
- You don't have to go through all the user data each time someone searches.
- Keep user info in the Map so you don't have to fetch it again.
- Maps make it easy to add or change info later.
This example shows that Maps are really good for when you need to find stuff fast, making searches quicker and simpler.
Conclusion
Key Takeaways
- Understanding arrays, sets, maps, and weak maps is super important for writing good JavaScript code. These tools help you organize and manage data in smart ways.
- Arrays are great for keeping items in a specific order, like a list. Sets help you avoid repeating the same item. Maps let you quickly find data with a key, and weak maps are good for adding extra info without using too much memory.
- To make your code work better and avoid problems, it's important to pick the right tool for the job, keep your code simple, and be careful not to make common mistakes.
- Using these tools in real projects, like making a shopping cart or a search feature, shows how valuable they are for solving different problems.
Next Steps
If you want to get even better with these tools:
- Try making small apps that use arrays, sets, and maps in various ways.
- Check out MDN Web Docs for more info.
- Practice with coding challenges on Codewars that focus on using these tools.
- Join discussions on Stack Overflow to see how others use them.
Getting hands-on practice is a great way to learn more.
Additional Resources
- JavaScript Data Structures and Algorithms - Online Course
- How to Use Map, Filter and Reduce in JavaScript - Tutorial
- JavaScript — Map vs Object vs Set- Comparison Guide
- Mastering Data Structures in JavaScript - Book
These resources go deeper into how to use these tools and why they're useful.