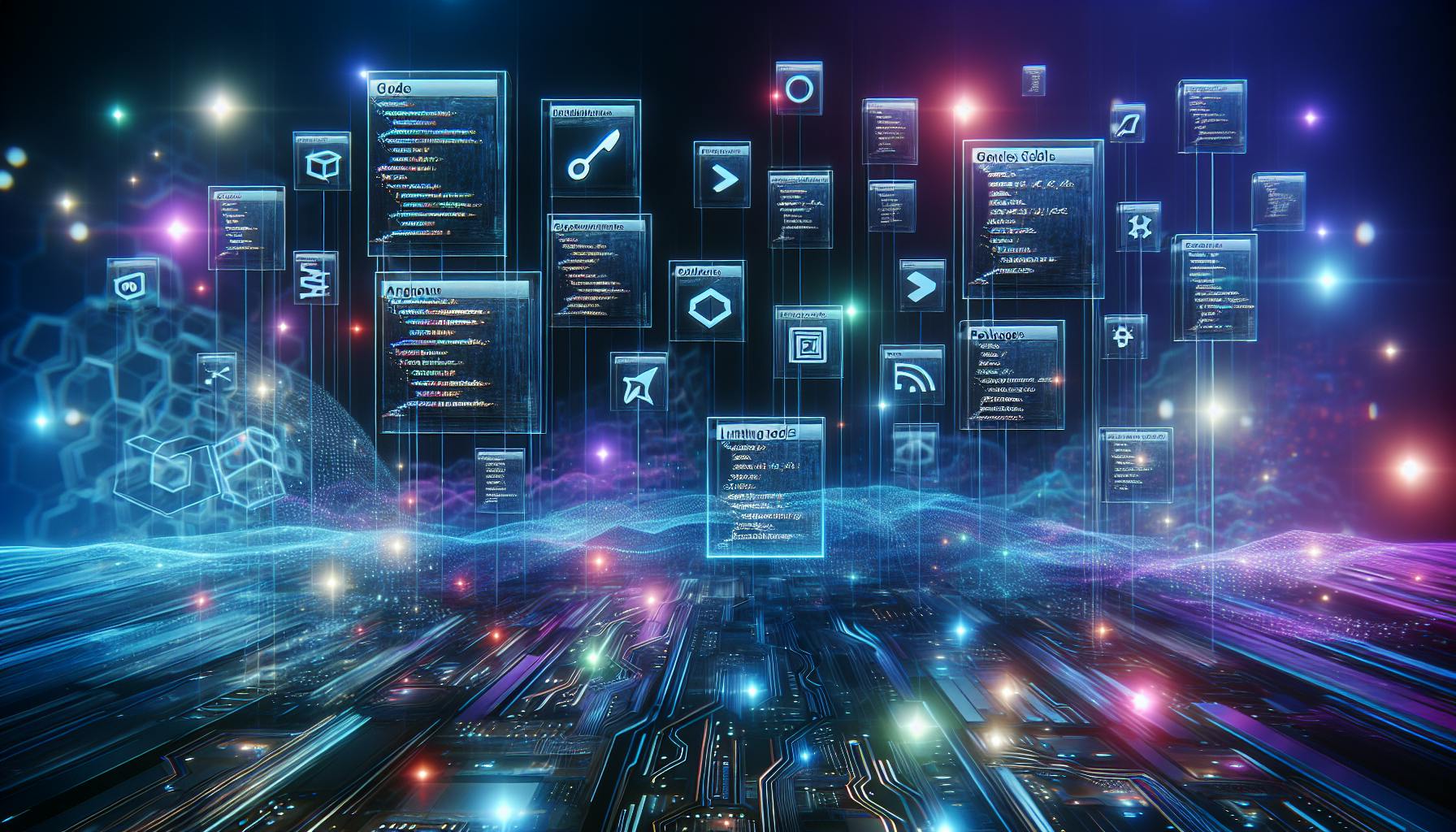
Learn how to use npm tsc for TypeScript projects. Set up your environment, compile code, enhance with ESLint and Prettier, and optimize for production. Dive into Node.js and npm setup, TypeScript editor plugins, and advanced usage with bundlers and transpilers.
If you're diving into TypeScript for your projects, you'll quickly encounter npm tsc
, the TypeScript compiler. Here's a straightforward guide to get you started:
- TypeScript transforms your code into JavaScript that browsers can understand.
- npm tsc is a tool that compiles TypeScript (.ts) files into JavaScript (.js) files.
- Setup: Install Node.js and npm, then add TypeScript to your project.
- Creating a Project: Initialize with
npm init
, then configure TypeScript withtsconfig.json
. - Compilation: Use npm scripts to compile your TypeScript files with
npm run compile
. - Watch Mode: Automatically recompile files on changes with
tsc -w
. - Linting and Formatting: Improve code quality with ESLint and make your code prettier with Prettier.
- Building for Production: Minify and bundle your code for faster loading times.
Remember, npm packages work seamlessly with TypeScript, giving you a robust ecosystem for your development needs. Whether you're a novice or looking to refine your build process, understanding npm tsc
is essential for working with TypeScript projects.
Node.js and npm
You'll need Node.js and npm installed on your computer. Here's how to get them:
- Go ahead and download the latest LTS version of Node.js. LTS stands for Long Term Support, which means it's stable and well-supported. When you install Node.js, npm comes with it.
- To make sure npm is up-to-date, run this command after installing Node.js:
npm install -g npm@latest
Node.js lets you run JavaScript on your computer, and npm helps you manage all the pieces of code you might need.
TypeScript Editor Plugins
To make coding with TypeScript easier, you'll want some plugins for your code editor:
- VS Code: Comes with TypeScript support already.
- Atom: Grab the atom-typescript plugin.
- Sublime: Install the TypeScript plugin.
These plugins help with things like making the code look nice, suggesting code as you type, and other helpful tools.
Once you've got Node.js, npm, and a plugin for your editor, you're all set to start using npm tsc to work on TypeScript projects on your computer. Next, we'll show you how to set up a simple project.
Setting up a TypeScript Project
Initializing npm project
To kick off a new project using Node.js and TypeScript, start by making a new folder and setting up npm inside it:
mkdir my-ts-project
cd my-ts-project
npm init -y
Running npm init
creates a package.json
file which is like a list for keeping track of all the code bits (dependencies) your project needs. The -y
option skips all the questions and fills in the defaults for you.
Then, add a .gitignore
file to keep certain files out of your Git history:
node_modules
dist
.parcel-cache
This step helps in skipping the Node modules folder, the folder where your final code (dist
) lives, and any cache from Parcel, a tool for bundling your code.
Installing TypeScript dependency
Now, you need to add TypeScript to your project. Do this by installing it as a development-only dependency:
npm install typescript --save-dev
The --save-dev
part means TypeScript is added under devDependencies
in your package.json
, indicating it's only for when you're working on your project, not for when it's actually running somewhere.
Creating tsconfig.json
To tell the TypeScript compiler how you want your code turned into JavaScript, you need a tsconfig.json
file:
npx tsc --init
This command makes a tsconfig.json
with standard settings. Here are a few settings you might want to tweak:
outDir
- tells where to put the JavaScript files after your TypeScript is compiledrootDir
- points to where to find your TypeScript filesstrict
- turns on strict rules for more secure codenoEmit
- stops the compiler from making JavaScript files (useful if you just want to check for errors)
With TypeScript ready and your tsconfig.json
set up, you're all set to start coding in TypeScript!
Compiling with npm tsc
Adding compile script
To make the TypeScript compiler (tsc) work using npm, you need to tweak your package.json
file a bit. Simply add a line under scripts
like this:
"scripts": {
"compile": "tsc"
}
This creates a quick way to run tsc. To compile your .ts files into .js, just type:
npm run compile
The tsc tool will check your tsconfig.json
for instructions on how to turn your TypeScript code into JavaScript.
Running compilation
Once you've set up your npm script, you can change your TypeScript into JavaScript by doing:
npm run compile
This process will:
- Look at all your .ts files
- Use the rules in your
tsconfig.json
to change them into .js files - Put the new .js files in the spot you picked in
tsconfig
(usually adist
folder)
For instance, index.ts
becomes dist/index.js
.
You'll use these JavaScript files on the web or on a server.
Watch mode
Tsc has a handy feature called watch mode that keeps an eye on your files and updates them as soon as you make changes:
tsc -w
With watch mode, every time you save a .ts file, tsc quickly updates it to JavaScript. This means you don't have to keep running npm run compile
over and over.
Watch mode stays on until you decide to stop it with Ctrl + C, making it easier to keep your project up to date.
Linting and Formatting Code
ESLint Setup
ESLint is a tool that helps you find and fix problems in your code. Setting it up for a TypeScript project is straightforward:
- First, you need to install ESLint and some TypeScript-specific tools:
npm install eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin --save-dev
- Next, create a
.eslintrc
file. This file will hold your rules. Here's a simple setup for TypeScript:
{
"parser": "@typescript-eslint/parser",
"plugins": ["@typescript-eslint"],
"extends": ["eslint:recommended", "plugin:@typescript-eslint/recommended"]
}
- You can change the rules in the
.eslintrc
file to make them fit what you want. The ESLint docs have a list of all the rules you can use. - To make it easy to check your code, add a command in
package.json
:
"scripts": {
"lint": "eslint . --ext .ts"
}
Now, ESLint will help you spot issues in your TypeScript files!
Prettier Setup
Prettier is a tool that makes your code look neat and consistent. Here's how to set it up:
- Install Prettier and some ESLint plugins for better integration:
npm install prettier eslint-config-prettier eslint-plugin-prettier --save-dev
- Make a
.prettierrc
file where you'll put your formatting preferences:
{
"printWidth": 80,
"tabWidth": 2,
"useTabs": false,
"semi": true,
"singleQuote": true,
"trailingComma": "es5",
"arrowParens": "avoid"
}
- Add another command in
package.json
so you can format your code easily:
"scripts": {
"format": "prettier --write ."
}
With Prettier, your code will always look good and be easy to read!
sbb-itb-bfaad5b
Building for Production
Minification and Bundling
When you're getting your TypeScript project ready for the real world, it's smart to make your files as small and efficient as possible. Here's how you can do that:
- Minifying your JavaScript: This means making your files smaller by getting rid of spaces, comments, and making names shorter. You can use a tool called Terser for this. Just add it to your project with:
npm install terser --save-dev
- Bundling your code: This means putting all your separate files into a few big ones, which makes them easier to handle. Tools like Webpack or Rollup.js are great for this job.
-
You can set up a
build
command in your npm scripts to do all this work for you, like so:
"scripts": {
"build": "tsc && terser ./dist -o ./dist && webpack"
}
Doing these steps makes your project load faster for your users by reducing the size and number of files they have to download.
Production Config
When you're ready to launch, it's a good idea to have a special setup for your TypeScript files that's all about being as efficient as possible. Here's what you can do:
-
Make a new TypeScript config file just for production, like
tsconfig.prod.json
, and point your output to a folder named./build
instead of./dist
. -
Turn on settings that make sure your code is super clean and error-free, like not creating files if there are errors (
"noEmitOnError"
), not making source maps ("sourceMap": false
), and doing extra checks ("noImplicitReturns"
,"noFallthroughCasesInSwitch"
). -
Also, tell it to remove comments from your final files by setting
"removeComments"
totrue
.
When it's time to build for production, just use this command:
tsc -p tsconfig.prod.json
Having a separate setup for when you're ready to go live helps make sure your app runs smoothly and quickly for your users.
Advanced Usage
Bundlers
Bundlers like Webpack and Rollup.js help get your TypeScript code ready for when it's live on the web. Here's how to make them work well with tsc:
-
Use
declaration
anddeclarationMap
in yourtsconfig.json
to create.d.ts
files. These files help bundlers understand the TypeScript types. -
Change
"module": "ESNext"
in yourtsconfig.json
. This tells tsc to make code that's compatible with ES6, which bundlers like more. -
If you're using Webpack, add
ts-loader
. This tells Webpack how to handle TypeScript files. -
For Rollup, add plugins like
@rollup/plugin-typescript
and@rollup/plugin-node-resolve
for dealing with TypeScript. -
Use
outDir
androotDir
intsconfig.json
to make sure tsc and your bundler agree on where files should go. - Turn on source maps to trace errors in your bundled code back to the original TypeScript files.
Transpilers
Transpilers like Babel and swc change new JavaScript back into older versions. Here's how to use them with tsc:
- Set
"target": "ESNext"
intsconfig.json
so tsc gives you the newest JavaScript. - Keep
"module": "ESNext"
to use modern JavaScript module syntax. - Have the transpiler work on the JavaScript that comes out of tsc, not the original TypeScript.
- Pick settings on your transpiler that match the old browsers or Node.js versions you need to support.
- Arrange your npm scripts to run tsc first, then send the output to the transpiler.
- Keep source maps on so you can see where errors in the transpiled JavaScript originally came from in TypeScript.
- Specifically for Babel, use
@babel/preset-typescript
to get it familiar with TypeScript.
The idea is to let tsc do its thing with the TypeScript, then have the transpiler make the JavaScript fit for older tech. This keeps things smooth and simple.
Conclusion
Using npm tsc to turn TypeScript code into JavaScript is pretty straightforward for those already familiar with JavaScript. Here are the main points to remember:
- The tsc tool, which comes with TypeScript when you use npm, changes your .ts files into regular JavaScript that web browsers can understand.
- You can set up commands in your package.json file, like
"compile": "tsc"
, to easily run the TypeScript compiler. - Handy features like watch mode and source maps make developing and debugging your TypeScript code easier.
- You can use extra tools like ESLint and Prettier, along with bundlers and transpilers, to make your project even better.
- When you're getting your app ready to show the world, you can use minification, bundling, and special compiler settings to make sure it's as fast and efficient as possible.
In short, npm tsc lets you use TypeScript in your projects without having to change how you work with Node.js and JavaScript. By tweaking a few settings, you can quickly switch your code to TypeScript and make your development process more efficient.
To go further, try out more advanced TypeScript features like interfaces and generics to make your app's design even stronger. The TypeScript community also offers lots of shared types and definitions to help make coding smoother.
As you get more used to npm tsc, consider looking into tools like Project References and Turborepo for managing big projects with lots of code, while keeping your build process quick and simple.
Related Questions
Do npm packages work with TypeScript?
Yes, npm packages can be used with TypeScript without any problems. If a package includes type definition files (with a .d.ts extension), TypeScript knows how to work with it, providing you with helpful features like autocomplete in your code editor.
What is the tsc for TypeScript?
The tsc stands for TypeScript compiler. It's a tool you run from the command line to turn your TypeScript code into regular JavaScript. This way, your code can run in both web browsers and on Node.js. The tsc is all about checking your code for errors and then translating it into a language that the web understands.
Is tsc deprecated?
No, the tsc isn't outdated or replaced. It comes with the TypeScript npm package, so when you install TypeScript using npm, you get tsc ready to use. You can run it directly using npx typescript
to access its features.
How to use tsc with npm?
To use tsc with npm, you can install TypeScript globally on your computer with npm install -g typescript
. This lets you use the tsc command from anywhere on your computer. If you prefer, you can install TypeScript just in your project, and then use npx to run tsc commands.