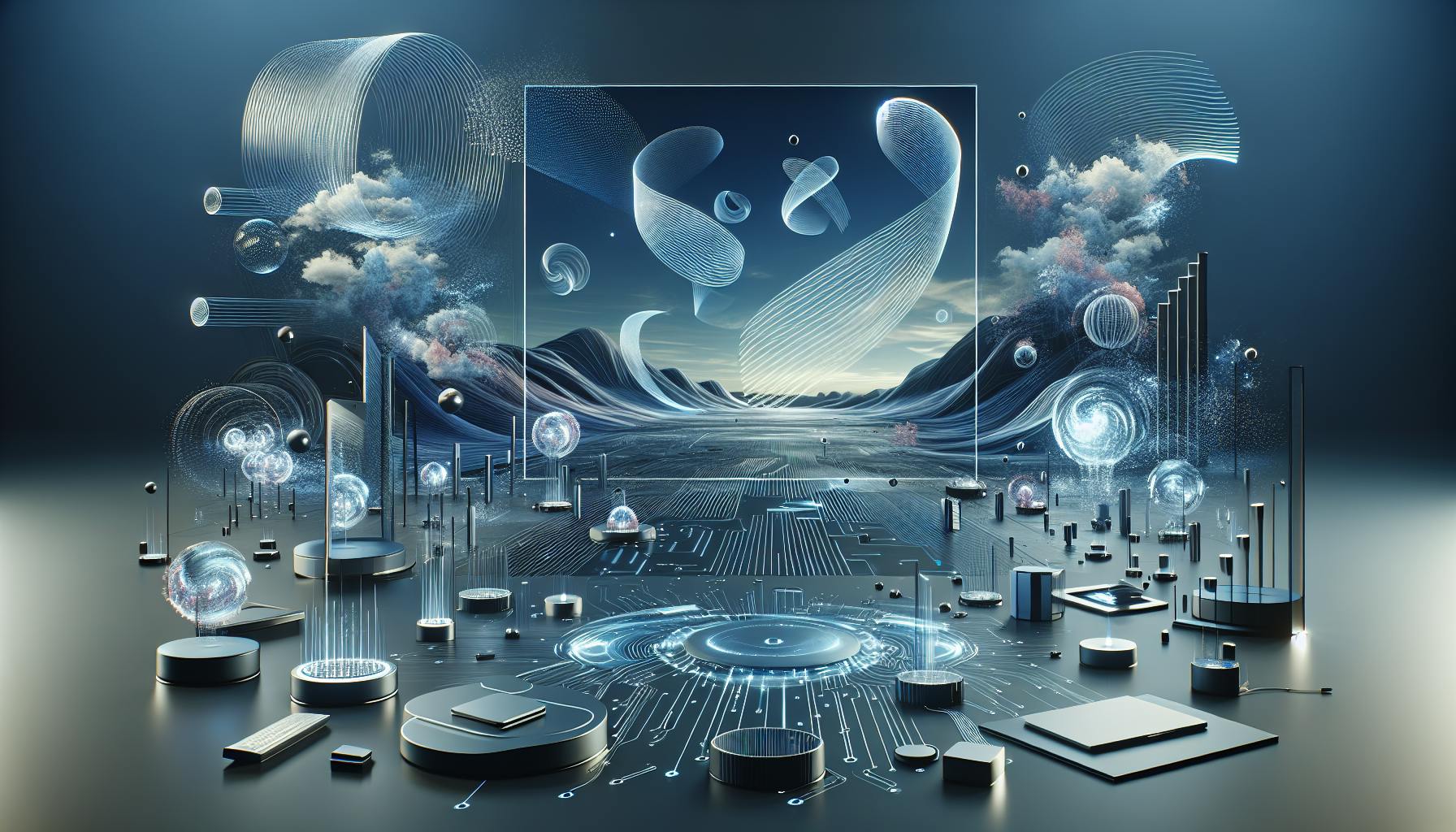
Enhance your JavaScript development environment with best practices for coding, collaboration, and tool selection. Learn about runtime options, package managers, version control, coding standards, and more.
Creating an effective environment for JavaScript development is key to improving productivity, collaboration, and the overall quality of your projects. This guide covers the essentials, from choosing the right tools to best practices in coding and teamwork. Here's a quick overview:
- Runtime Options: Node.js, Deno, and web browsers.
- Package Managers: npm, Yarn, and pnpm.
- Development Tools: Code editors like Visual Studio Code, WebStorm, and Sublime Text.
- Version Control: Practices around using Git effectively.
- Coding Standards: Importance of style guides and linting.
- Modularization: Benefits of component-based architecture.
- Security: Managing environment variables and secrets safely.
- Dependency Management: Keeping your project's dependencies in check.
- Building and Bundling: Tools like Webpack and Vite for optimizing your code.
- Testing and Debugging: Writing testable code and efficient debugging techniques.
- Performance Optimization: Tips for improving JavaScript performance.
- Collaboration and Workflow: Tools and practices for effective teamwork.
- Continuous Integration and Deployment (CI/CD): Automating tests and deployments for smoother workflows.
Whether you're new to JavaScript or an experienced developer, setting up a conducive environment and following these practices can significantly enhance your work.
1. Understanding the Basics
When we talk about setting up an environment for JavaScript, we're looking at three main parts:
- Runtime - This is where your JavaScript code runs. Common choices are Node.js for server-side projects, Deno, and web browsers for client-side scripts.
- Package Manager - These tools help you add and manage extra bits of code (libraries or frameworks) from other people. npm, Yarn, and pnpm are popular ones.
- Development Tools - This includes stuff like code editors (where you write your code), tools to check your code for mistakes (like ESLint), and others that make coding easier. Visual Studio Code is a favorite editor for many.
Choosing the right tools from each category depends on what you're working on and what you like. Knowing what each part does helps you pick the best ones for your project.
2. Choosing the Right IDE
An IDE, or code editor, can make coding a lot smoother by helping you write, test, and debug your code. Here are some good options for JavaScript:
IDE | Pros | Cons |
---|---|---|
Visual Studio Code | Lots of add-ons, great for TypeScript, quick | Might be too much for beginners |
WebStorm | Made for web development, really smart at understanding code | Costs money, uses a lot of computer resources |
Sublime Text | Quick and light, easy to use | Not as many features without adding plugins |
Think about what you need from an IDE. Do you want something simple or with all the bells and whistles? Are you working alone or with a team? Testing out a few can help you find the best one for you.
3. Version Control Systems
Version control, like Git, lets you and others work on code together without mixing it up by keeping track of changes. Here are some good habits:
- Write clear messages when you save changes
- Use branches for working on new stuff or fixing bugs
- Review changes with your team before adding them to the main project
- Mark big updates clearly
- Keep secret keys out of your project!
Using version control right makes working on projects smoother and keeps everyone on the same page. Platforms like GitHub or GitLab add extra features like letting you control who can see your code, keeping track of issues, and automating some tasks.
Core Practices
4. Coding Standards and Style Guides
It's important to follow certain rules and guidelines when writing code to make sure it's easy to read, update, and work on with others. These rules help with things like naming variables and organizing your code.
Why this matters:
- Easy to Read - If everyone uses the same style, it's simpler to understand the code.
- Teamwork - It helps prevent arguments and mix-ups about how to write code.
- Easy to Update - If your code follows a standard, it's easier to change things later.
- Sharing - Code that follows common rules is easier to share with others.
Some well-known guides are from Airbnb, Google, and Standard. It's good to pick one as a team. Tools like ESLint can help make sure everyone sticks to the rules.
5. Linting and Formatting
Linting is when you use a tool to check your code for mistakes or parts that don't match the style guide you're following. Formatting is about arranging your code neatly.
They help by:
- Pointing out possible errors
- Highlighting when you're not following the style guide
- Fixing messy code
- Making your code look nice
- Sometimes, fixing problems for you
Tools like ESLint and Prettier work with most code editors and can really help improve your code. It's best if everyone on the team uses these tools the same way to keep things consistent.
6. Modularization and Component-Based Architecture
Writing your code in separate, small pieces can make it easier to handle. This approach lets you reuse parts of your code, makes it simpler to fix problems, and helps when you want to add more features.
Why it's good:
- Reuse - You can use the same piece of code in different places.
- Easier to Fix - If something goes wrong, it's easier to find and fix the problem.
- Grow Your Project - Adding new features is simpler.
- Work Together - It's easier for people to work on different parts at the same time.
How to do it:
- Split your code into small, focused parts.
- Make each part independent and straightforward.
- Make sure the parts can work together smoothly.
- Use libraries like React that are all about building with small, reusable pieces.
Starting with a plan for how to organize your code can save you a lot of trouble later on.
Advanced Configuration
7. Environment Variables and Security
Keeping secret info like API keys or passwords safe is really important. Here's how to do it right:
- Use
.env
files to keep your secret info. This way, it doesn't get shared by accident. Make sure.env
is listed in your.gitignore
file so it doesn't go online. - When your project is live (in production), add these secrets directly on your hosting service, not in your code.
- If you're working with others, consider using a secure tool like Vault by HashiCorp to share secrets safely.
- If you can, encrypt these secrets using tools like AWS Key Management Service.
- Make sure only the people who really need to see these secrets can access them.
- Change your keys and passwords from time to time to keep things extra secure.
Following these steps helps keep your project's secret info safe from leaks.
8. Dependency Management
Handling your project's external code pieces (dependencies) well is key to avoiding headaches. Here are some smart moves:
-
Use tools like npm or Yarn to keep track of all the external code your project uses. They make adding and updating these pieces easier.
-
In your
package.json
, lock down the versions of your dependencies to avoid surprises from updates. -
Regularly run
npm audit
oryarn audit
to spot and fix security issues in your dependencies. -
Think twice before adding a new dependency. More dependencies mean more potential problems down the road.
-
Keep development and production dependencies separate to keep your live project light.
-
Look into a dependency's popularity and how well it's maintained before adding it. This can save you trouble later.
Organizing your dependencies well now can save you a lot of time fixing things later.
9. Building and Bundling
JavaScript tools like bundlers help make your code ready for the web, making it faster and more compatible.
Why use a bundler?
- They shrink and clean up your code to make it smaller.
- They change new JavaScript into a form that works on older browsers.
- They gather and combine all your code and its dependencies into a few files.
- They help your website load faster by doing things like caching.
Some good tools are:
- Webpack: Great for all kinds of projects
- Parcel: Easy to use if you're not looking for lots of settings
- Rollup: Good at getting rid of code you're not using
- Vite: Super fast, especially for new web projects
These tools might take a bit to set up, but they make your development process much smoother. Setting them up to compress, cache, and convert your code can make your website run faster and let you use the latest JavaScript features.
Testing and Debugging
10. Writing Testable Code
When you write code that's easy to test right from the start, checking your project for errors becomes a lot simpler. Try these ideas:
- Make functions small and to the point - Smaller functions are simpler to check.
- Stay away from side effects - Your functions should do their job without messing with anything outside.
- Use dependency injection - This means giving a function what it needs instead of letting it get it on its own. This way, you can use fake versions for testing.
- Keep to what's shared - Only use functions that are meant to be used by others, not the private ones inside modules.
- Define object shapes with interfaces - This helps you test using any object that looks right.
Tools like Jest, Mocha, Ava, and Jasmine are great for making testing easier. Starting tests early can save you a lot of trouble later on.
11. Debugging Techniques
Finding bugs is part of coding. Here's how to deal with them:
-
Logging - Print out values to see what's going on and spot issues.
-
Use 'debugger;' - Putting
debugger;
in your code pauses it so you can look closer. -
Use debugging tools - Browsers and IDEs like Visual Studio Code have tools to help you find and fix problems.
-
Talk it through - Explaining your code out loud can help you find where you went wrong.
-
Narrow it down - Use comments or logs to figure out where the issue might be.
-
Check your understanding - Make sure you really know how things are supposed to work. Confirm each part step by step.
-
Get a second opinion - Sometimes, just showing your code to someone else can help find the bug.
With a little patience and the right approach, you can solve even the hardest problems.
sbb-itb-bfaad5b
Performance Optimization
12. Optimizing JavaScript Performance
To make your JavaScript run faster and smoother, focus on writing better code, loading resources smartly, and making sure things render well on the screen. Here are some straightforward tips:
Optimize Code Execution
-
Turn on strict mode to catch mistakes early. Just add "use strict"; at the beginning of your JS files.
-
When declaring variables, use
const
andlet
instead ofvar
to avoid accidentally creating global variables. -
Instead of using loops, try array methods like
.map()
and.filter()
for quicker operations. -
Keep heavy tasks like big calculations outside loops or functions that run a lot.
-
If a function runs too often (like when scrolling), use
setTimeout()
or_lodash.throttle
to slow it down a bit. -
Store data you use a lot in variables or objects so you don't have to look it up every time.
Improve Resource Loading
-
Make your JavaScript, CSS, and HTML files smaller for production by minifying them.
-
Turn on gzip compression to make these files even smaller.
-
Use a CDN to spread your files across servers worldwide for quicker downloads.
-
Lazy load images and other content that's not immediately visible to make the first page load faster.
-
Use
<link rel="preload">
to tell browsers to load important stuff first.
Optimize Rendering
-
For visual changes, use
requestAnimationFrame()
to match the screen's refresh rate better than with timeouts. -
Use Web Workers for long JavaScript tasks to keep the user interface smooth.
-
Avoid causing the page to repaint or reflow too often. Try to make all your DOM changes at once.
-
Use
lodash
to debounce scroll and resize events, which helps prevent too much rendering.
Follow these simple steps, and your JavaScript will run more efficiently, making your site feel quicker and more responsive. Keep an eye on performance with tools like DevTools and Lighthouse.
Collaboration and Workflow
13. Effective Collaboration in Teams
Working together well is crucial for any JavaScript team. It's important to pick tools and habits that make talking to each other easier, make work flow smoothly, and help avoid stepping on each other's toes.
Communication Tools
- Slack/Discord: Good for quick chats and questions. Works well with other tools.
- Zoom/Meet: Lets remote teams have face-to-face talks. You can record meetings to watch later.
- Twist/Threads: Keeps talks organized by topic or project. Great for chatting when you're not all online at the same time.
- GitHub/GitLab: Where you keep your code and use tools to hand out tasks and talk over ideas.
Workflow Tools
- Git: Keep changes small and clear. Use details in your update messages. Make separate branches for big updates.
- Husky/Lint-Staged: Automatically makes sure your code looks good and works before any changes are made final.
- Semantic Release: Handles version numbers and change logs automatically when you add to the main project. No more last-minute rush to get releases ready.
Best Practices
- Have a quick 15-minute meeting every day so the team knows what's going on.
- Use wikis for writing down how things are done, style guides, and project details.
- Look at each other's code to learn and make sure it's good.
- Decide on how to write and format code to avoid mix-ups.
With the right tools and a bit of planning on how to talk to each other, teams can work together better and build cooler stuff faster.
14. Continuous Integration and Deployment
CI/CD is a way to make sure new features are added smoothly and safely by using automatic tests and updates.
Key Practices
- Automated Testing: Checks your code for problems every time you make changes. Jest, Mocha, and Cypress are some tools people use.
- Build Verification: Makes sure your app can be built without errors every time you update. Helps catch problems early.
- Static Analysis: Tools like ESLint help find issues with code quality and consistency.
- Code Review: Having someone else look at changes before they're final helps keep things high quality and spreads know-how.
Popular CI/CD Platforms
- GitHub Actions: Works well with GitHub to run checks when code is updated.
- CircleCI: Lets you set up workflows easily with a lot of options for common tools.
- Travis CI: A well-known platform with a lot of support from users.
Tips for Success
- Start with small, less important updates first.
- Keep an eye on updates and set alerts to catch any issues.
- Write down how things are done so anyone can fix or change workflows.
- Use feature flags and small test rollouts for big updates.
With CI/CD, developers can do more, spend less time on manual work, and safely add new things quickly.
Conclusion
Getting your JavaScript setup right might take a bit of work at the beginning, but it really helps make things easier and better in the long run. Here's what you should remember from this guide to make a great environment for working on JavaScript projects.
- Pick the main tools you need, like where your JavaScript will run, how you'll manage extra code, and where you'll write your code. Go for ones that work well together.
- Agree on coding rules with your team and use tools like ESLint and Prettier to keep your code clean and easy to read. This makes working together and understanding each other's code much simpler.
- Break down your code into smaller parts that can work on their own. This helps make your code easy to use again, easy to fix, and easy to add to.
- Be smart about using external code in your project, keep important information safe, and make sure the way you put together your code for the final product is efficient.
- Design your code so it's easy to test from the start. Add in testing steps that catch problems early. This saves a lot of time fixing bugs later.
- Use smart tricks to make your website or app work smoothly and quickly for users. Simple steps can make a big difference in how fast and nicely your project works.
- Make sure your team can talk easily, work well together, and use automation to make common tasks quicker. Planning this part can really help everyone get more done.
While setting up a good JavaScript environment takes some effort, it's a great investment. With the right setup, you can spend more time creating and less time fixing problems. It's definitely worth it for making your work better and your life easier.
Related Questions
Where is the best place to practice JavaScript?
If you want to get better at JavaScript, here are some cool websites to try:
- JSChallenger: Offers interactive challenges and tutorials.
- Codewars: Solve coding problems and learn from other developers.
- Exercism: Practice with exercises and get feedback.
- A Smarter Way to Learn JavaScript: Uses interactive lessons.
- W3resource: Has exercises and quizzes on the basics.
- Edabit: Features quick challenges to solve.
- JS Hero: Learn by playing through a game.
These sites give you a hands-on way to improve your JavaScript skills with fun lessons and challenges.
What environment for JavaScript?
JavaScript can run in two main places:
- Browser environment: This is where JavaScript works in your web browser, letting you interact with web pages.
- Node.js environment: Here, JavaScript runs on a server, not in your browser. It's used to build things like web servers.
So, you can use JavaScript for both making websites interactive and for server-side stuff with Node.js.
What IDE should I use for JavaScript?
IntelliJ IDEA is a great choice for working with JavaScript. It's packed with features to help you code better, like:
- Helps with writing and refactoring code
- Supports lots of JavaScript tools and frameworks
- Has built-in tools for debugging and testing
- Lets you customize a lot
Other good options include Visual Studio Code, WebStorm, and Sublime Text. The best one for you depends on what you need. But IntelliJ IDEA is a top pick for its strong JavaScript support.
How to do best practice of JavaScript?
Here are some tips for writing good JavaScript code:
- Stick to a code style guide like Airbnb or StandardJS.
- Name things clearly.
- Use
const
andlet
instead ofvar
. - Write comments for tricky code parts, but keep them short.
- Don't use JavaScript for something you can do with CSS.
- Use tools like ESLint to help you write better code.
- Break your code into small, focused functions.
- Use
try...catch
to handle errors smoothly.
These practices help make your code easier to read and work with. They're good habits that make coding with others smoother, too.