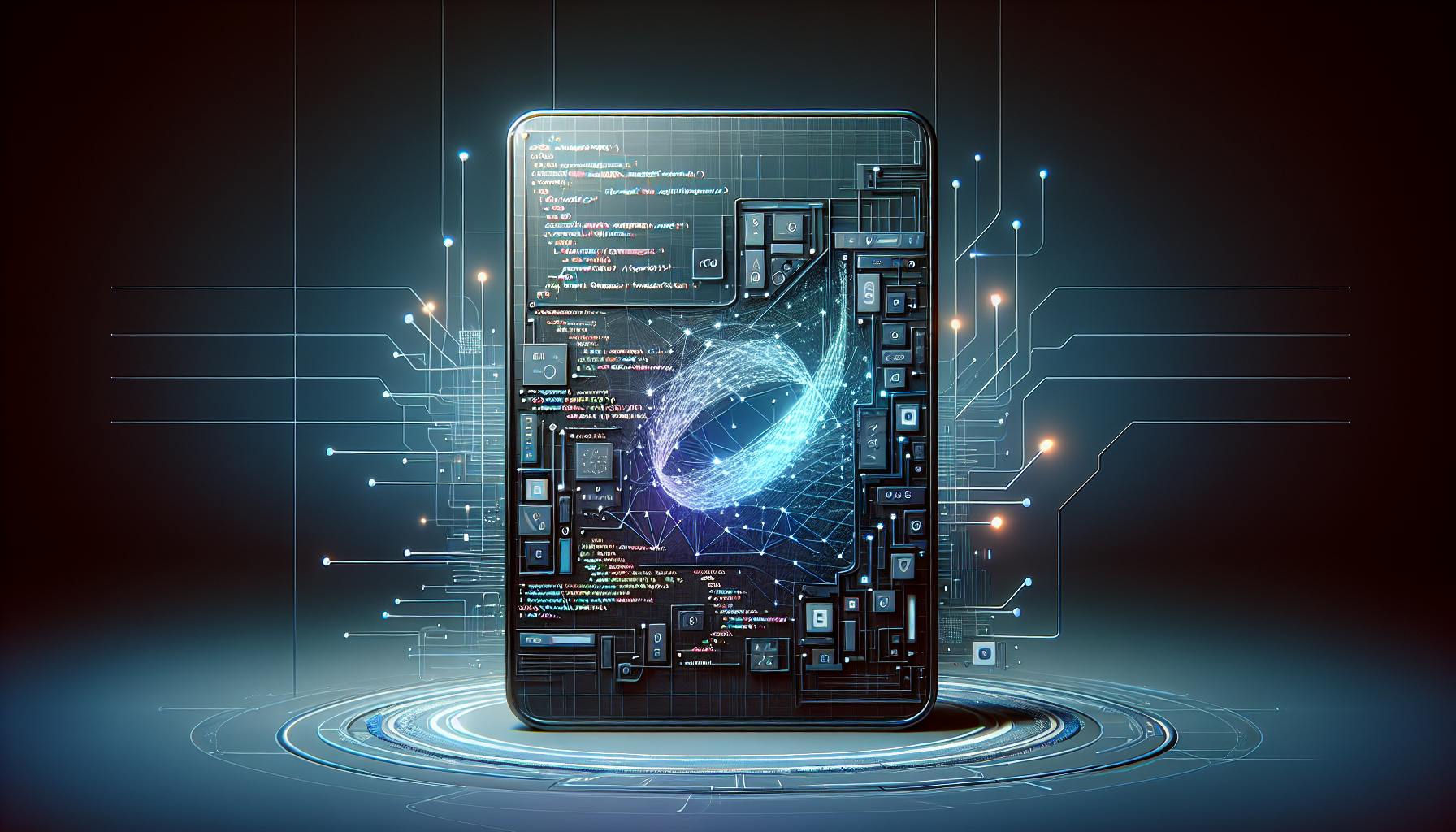
Key tips for Go developers transitioning to JavaScript, from syntax differences to setting up the environment. Learn about dynamic typing, asynchrony, error handling, and code organization.
Transitioning from Go to JavaScript can be a smooth journey with the right approach. Here are key tips to help Go developers adapt to JavaScript effectively:
- Understand the Syntax Differences: Variables, functions, conditionals, and loops have different syntax in JavaScript.
- Embrace the Dynamic Type System: Unlike Go's static typing, JavaScript uses dynamic typing, which can make development faster but requires caution.
- Set Up Your Environment: Install Node.js and familiarize yourself with npm commands for managing packages.
- Learn New Development Tools: Explore IDEs like Visual Studio Code and WebStorm, and utilize tools like Prettier and ESLint for code formatting and linting.
- Master Asynchrony and Error Handling: Get comfortable with JavaScript's async/await, promises, and try-catch for error handling.
- Organize Your Code: Adopt best practices for structuring your JavaScript projects effectively.
Additionally, continuously engage with learning resources such as online courses, books, and the JavaScript community to enhance your skills. Transitioning to JavaScript opens up a vast ecosystem of possibilities, so take it one step at a time, and enjoy the journey.
Syntax Comparison Table
Feature | Go Syntax | JavaScript Syntax |
---|---|---|
Variables | var name string |
let name = 'John' |
Functions | func add(x, y int) int { return x + y } |
function add(x, y) { return x + y; } |
Conditionals | if x > 10 { } else { } |
if (x > 10) { } else { } |
Loops | for i := 0; i < 10; i++ { } |
for (let i = 0; i < 10; i++) { } |
Imports | import \"fmt\" |
import lodash from 'lodash' |
When you look at Go and JavaScript, you'll see they have some things in common, like using curly braces and semi-colons. But, Go is stricter about how you write your code, especially with variables and spacing. JavaScript is more relaxed and lets you do things more freely.
Type Systems
Go is what's called a statically typed language. This means when you're writing your code, you have to say what type each piece of data is (like a number or text) before the program runs. This is good because it can catch mistakes early, help your tools suggest code, and make your program run faster. But, it also means you have to be very clear about your data types, and changing them can be a bit of work.
JavaScript is different. It's dynamically typed, so you don't have to declare the type of data upfront. This makes JavaScript super flexible and great for quickly trying out new ideas. However, it can also make finding mistakes harder because they only show up when the program is running. And sometimes, it's not easy to tell what type of data you're dealing with.
Some JavaScript developers use a tool called TypeScript to make their code more like Go's, with static types. This way, they get the best of both worlds: JavaScript's flexibility and the reliability of static typing. Each language's approach to types has its ups and downs, but knowing these differences can help you make a smooth shift from Go to JavaScript.
Setting Up Your JavaScript Environment
Installation and Tooling
Before you dive into JavaScript, you'll need to get Node.js on your computer. Node lets you run JavaScript not just in web browsers but pretty much anywhere.
After installing Node, you'll get a tool called npm for free. It lets you add other useful tools for JavaScript:
-
Code formatter: Prettier helps make your code look neat and consistent.
-
Linter: ESLint checks your code for mistakes and helps keep it clean.
-
Bundler: Webpack takes all your JavaScript pieces and packs them together, making them ready for the web.
Nodemon is another handy tool that refreshes your project automatically every time you make changes.
Here are some basic npm commands you'll likely use:
npm init -y // starts a new node project
npm install [package] // adds a new tool or library npm uninstall [package] // removes a tool or library
npm run [script] // runs a command you've set up in your project settings
IDEs and Debugging
Visual Studio Code is a free program where you can write and manage your JavaScript code. It's pretty popular and can do a lot, especially with the right add-ons for:
- Debugging in Chrome
- Using ESLint
- Running Prettier
- Testing with Jest Runner
These add-ons help you find and fix errors, keep your code looking clean, and do tests right in the program.
WebStorm is another option that's all about JavaScript. It comes with a lot of built-in tools for writing code, finding bugs, and more.
No matter which program you use, getting to know how to debug is key. Learning to pause your code and check it step by step can really help you understand what's going on and fix problems.
Mastering Key Differences
As you move from Go to JavaScript, understanding how they handle certain things differently will make things easier for you.
Asynchrony/Concurrency
Go and JavaScript manage tasks at the same time in different ways.
In Go, you have goroutines and channels. Think of goroutines like super light mini-teams that do tasks at the same time. Channels are like walkie-talkies for these mini-teams to talk to each other safely.
JavaScript does things one at a time but can still handle multiple tasks by setting them aside and coming back to them later. It uses things like callbacks, promises, and async/await to manage these tasks. Instead of stopping everything for a task, it waits for tasks (like loading a webpage) in the background.
So, Go creates mini-teams to work on tasks at the same time, while JavaScript takes turns on tasks without stopping the main work.
Error Handling
Go and JavaScript also deal with mistakes differently.
In Go, when something might go wrong, the function tells you by returning an error you can check.
file, err := os.Open("file.txt")
if err != nil {
// handle error
}
In JavaScript, you try to do something, and if it doesn't work, you catch the error right there.
try {
const file = fs.readFileSync('file.txt');
} catch (err) {
// handle error
}
So, in Go, you check if there's an error after trying something. In JavaScript, you plan ahead for what to do if something goes wrong.
Modules and Packages
Go uses packages, and JavaScript uses modules to organize code.
In Go, packages are like folders that hold your code, and you can share parts of it with other parts of your program.
JavaScript modules let you share code too, but they use import/export to do it. JavaScript has changed over time in how it handles modules.
So, moving from Go to JavaScript means getting used to how JavaScript shares code differently with its module system.
Understanding these differences will help a lot as you start using JavaScript. Each language has its own way of doing things, so focusing on these differences will help you adjust.
Best Practices for a Smooth Transition
Embrace the JavaScript Ecosystem
Jumping from Go to JavaScript might feel like a lot at first because there are so many tools and libraries. Here's how to get comfortable:
- Start using npm to add cool libraries to your projects. Check out Lodash, Express, and React for starters.
- Try different editors like VS Code, WebStorm, or Sublime Text to see which one you like best. A good editor can make coding easier.
- Keep up with the latest in JavaScript by following developers and groups on Twitter and Medium.
- When you're stuck, Stack Overflow and MDN Web Docs are great places to look for answers.
- Don't rush to learn everything. Get the basics down, then slowly add more tools as you need them.
JavaScript gives you lots of options to build things. Getting used to this can help you make the most of what JavaScript offers.
Mastering Asynchronous Coding
If you're used to Go, handling tasks that run at the same time in JavaScript might seem tricky. Here are some tips:
- Use async/await instead of callbacks. It makes your code easier to read.
- Keep your app running smoothly by doing heavy tasks in worker threads.
- When you have a bunch of tasks that need to happen one after the other, link promises together and use
.catch()
to handle any errors. - Learn how the event loop works. It helps JavaScript do many things at once without getting mixed up.
- Try using libraries like Async.js for tricky async tasks.
- Test your code well. When things run at the same time, it's easy for mistakes to happen.
Getting used to async programming takes time, but it'll get easier, especially with async/await.
Structuring JavaScript Projects
In Go, keeping your code organized is key. Here's how to do that in JavaScript:
- Put files together based on what they do (like all the login stuff in one place).
- Break your code into modules, so you can use the same code in different places.
- Name your files, variables, and classes in a way that makes sense and stick to it.
- Keep common code in utility files so you can use it everywhere without repeating yourself.
- Make a constants file for things that don't change, like settings, to avoid repeating them.
- Try to keep similar things at the same level in your project.
- Use JSDoc to explain what your modules and functions do. It makes it easier for others to understand your code.
Even though JavaScript lets you do things many ways, adding some structure makes your project easier to handle as it grows. Use what you know from Go to keep things tidy.
sbb-itb-bfaad5b
Continued Learning Resources
Online Courses and Tutorials
Here are some good online courses and tutorials to help you get better at JavaScript:
-
JavaScript Algorithms and Data Structures Masterclass on Udemy - This course covers both the basics and more complex topics like algorithms.
-
Modern JavaScript Tutorial on javascript.info - This is a step-by-step guide that takes you from the basics to more advanced stuff. It's interactive, so you can practice as you learn.
-
JavaScript 30 by Wes Bos - This course challenges you to build 30 small projects using JavaScript. It's great for hands-on learning.
-
FreeCodeCamp's JavaScript Course - This is a self-paced course with videos, coding challenges, and projects. It's free and you can get certifications.
-
Codecademy's Learn JavaScript Course - This course is interactive, with a built-in editor to try out code.
Essential Books
These books are great for learning more about JavaScript:
-
"Eloquent JavaScript" by Marijn Haverbeke - A free online book that covers from basic to advanced JavaScript with interactive examples.
-
"You Don't Know JS" by Kyle Simpson - A series of books that dive deep into how JavaScript works.
-
"JavaScript: The Definitive Guide" by David Flanagan - A detailed guide to JavaScript's features and best practices.
-
"JavaScript: The Good Parts" by Douglas Crockford - A short book focusing on the most important parts of JavaScript.
Community Support
Join the JavaScript community for more learning:
-
Stack Overflow JS Forum - A place to ask and answer JavaScript questions.
-
/r/JavaScript Subreddit - A community on Reddit where you can talk about JavaScript.
-
Dev.to JavaScript Tag - A site where you can read and write articles about JavaScript.
-
JS.coach Directory - A directory to find JavaScript libraries, tools, and more. It's great for finding new things to learn.
Taking part in courses, reading books, and connecting with the JavaScript community will help you improve your skills.
Conclusion
Switching from Go to JavaScript might seem tough at first. They're pretty different in how they handle things, like how they deal with types of data or do several tasks at once. But, if you focus on these differences and get the hang of JavaScript's way of doing things one after the other, it'll make things easier.
Here's some advice for a smooth move:
- Keep an open mind. Understand that JavaScript does things its own way. Don't expect it to be just like Go.
- Start with the basics. Get to know the simple stuff like syntax and setting up your environment before jumping into more complex areas. Make sure you're comfortable with the foundation.
- Focus on learning step-by-step. Really try to understand how JavaScript handles tasks that run at the same time, like promises and async/await. This is super important.
- Organize your code well. Use what you know from Go about keeping code organized to help manage your JavaScript projects, especially as they get bigger.
- Practice a lot. The more you code, the more familiar JavaScript will feel. Work on small projects to keep practicing what you're learning.
- Don't be shy to ask for help. JavaScript has a big community. If you're stuck or have questions, reach out. Always keep learning.
The main thing is to focus on the differences between JavaScript and Go and slowly get used to JavaScript's unique ways. Be patient, keep practicing, and use the resources out there. Soon, JavaScript will start to feel like a natural part of your skillset, not something completely new. The experience you have from Go will be really helpful as you learn to write good JavaScript code.
Related Questions
How to style transition in JavaScript?
To add a smooth change effect in JavaScript, you can use the transition
property like this:
const myElement = document.getElementById('myElement');
myElement.style.transition = 'background-color 1s ease';
document.body.appendChild(myElement);
myElement.addEventListener('click', () => {
myElement.style.backgroundColor = 'red';
});
This code makes the background color change smoothly when you click on the element. You can change the transition effect and timing as you like.
How to trigger transition using JavaScript?
To make an element change smoothly with JavaScript, you can switch a class name that has the transition styles. Like this:
const el = document.getElementById('myElement');
el.classList.add('transition');
el.classList.toggle('active');
You can also check and control the transition with getComputedStyle()
and getPropertyValue()
if you want to pause it or do more advanced stuff.
How do I become comfortable with JavaScript?
Here's how to get better at JavaScript:
-
Learn the basic parts like variables, functions, and loops well.
-
Keep practicing with online lessons or coding challenges. Doing it often helps you learn.
-
Once you're good with the basics, try out tools like React.
-
Join groups where developers hang out, like GitHub or Stack Overflow.
-
Make small projects by yourself to use what you've learned. Start simple.
-
It's okay if you don't get everything right away. Go step by step, and you'll get the hang of it.
How do you trigger transitions in CSS?
For CSS transitions, you need:
- Something to change, like the color or size of an element.
- A way to start the change, like moving your mouse over it or clicking.
For example:
button {
background: blue;
transition: background 0.3s ease;
}
button:hover {
background: red; /* This starts the change */
}
The mouse hover makes the button's color change, and the transition makes it smooth. You can also use JavaScript to switch classes and start these effects.